## 六、组件切换
### 6.1、方式一:使用v-if判断
Vue.component(“login”,{
template: `
Vue.component(“register”,{
template: `
const app = new Vue({
el: “#myDiv”,
data: {
isLogin: true
},
methods: {
login(){
this.isLogin = true;
},
register(){
this.isLogin = false;
}
}
});
### 6.2、方式二:is实现
Vue.component(“login”,{
template: `
Vue.component(“register”,{
template: `
const app = new Vue({
el: “#myDiv”,
data: {
componentName: “login”
}
});
## 七、父子组件
### 7.1、初步认识父子组件的定义
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 1、定义子组件
const children = Vue.extend({
template: `
这个子组件
` });// 2、定义父组件
const father = Vue.extend({
template: `
这个是父组件
const app = new Vue({
el: “#myDiv”,
components: {
father
}
});
### 7.2、父组件向子组件传递数据
#### 7.2.0、问题案例
**解决思路可以这样:在父组件中,可以在使用子组件的时候,通过属性绑定的方式,把需要传递给子组件的数据以属性绑定的形式传递到子组件内部,这样的话,子组件内部就可以接收到了。**
**方式:通过props向子组件传递数据**
#### 7.2.1、形式一
**字符串数组,数组中的字符串就是传递时的名称。**
说明:让Vue的实例作为父组件,之后再定义一个子组件
<template id="myTemplate">
<div>
<p v-for="item in subaddresses">
{{item}}
</p>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 注册组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: [“subaddresses”]
});
const app = new Vue({
el: “#myDiv”,
data: {
fatherAddresses: [
‘河南林州’,
“陕西西安”,
“浙江杭州”
]
}
});
**父组件要向子组件传递数据,总结如下:**
* 第一步:
在父组件去应用子组件的时候,去动态的绑定一个属性。
`subusername`这个属性就是自定义的一个属性名称,目的是向子组件中传递数据的这么一个名称。而`username`就是父组件的`data`中的数据。
* 第二步:
自定义属性做好之后,子组件还不能直接使用,还需要接收。即:把父组件传递过来的`subusername`属性,需要在子组件的`props`数组中定义,注意是一个字符串的形式,这样的话,子组件才能使用这个数据。
也可以这么说:**组件中所有的props中定义的数据,都是通过父组件传递给子组件的。**
* 第三步:
子组件在`template`中使用子组件`props`定义的名称,就可以使用数据了。
**说明几个细节问题:**
* 对于子组件来说,也可以有自己的`data`属性,也就是说子组件可以有自己的数据,而`data`中的数据并不是通过父组件传递过来的,而是子组件自身所独有的,常用的方式是:子组件可以通过调用ajax请求数据,之后把查询出来的数据填充到`data`属性中。而子组件的`props`属性一定是从父组件传递过来的。
* `data`属性中的数据都是可读可更改的,而`props`属性中的数据是只读的。
#### 7.2.2、形式二
**对象,对象可以设置传递时的类型,也可以设置默认值**
<template id="myTemplate">
<div>
<p v-for="item in subaddresses">
{{item}}
</p>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 注册组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subaddresses: Array // 对象,可以指定类型
}
});
const app = new Vue({
el: “#myDiv”,
data: {
fatherAddresses: [
‘河南林州’,
“陕西西安”,
“浙江杭州”
]
}
});
* 指定默认值:基本类型
<template id="myTemplate">
<div>
{{subusername}}
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 注册组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subusername: {
type: String,
default: “Spring”
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
fatherUsername: “HelloWorld”
}
});
* 指定默认值:对象或者数组
<template id="myTemplate">
<div>
<p v-for="item in subaddresses">{{item}}</p>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 注册组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subaddresses: {
type: Array,
default: function(){
return [‘HelloWorld’ , “Spring”]; // 对象或数组默认值的话要用函数
}
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
fatherAddresses: [
“河南林州”,
“浙江杭州”
]
}
});
* 指定是否必传
<template id="myTemplate">
<div>
{{subusername}}
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 注册组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subusername: {
type: String,
required: true // 表示必传
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
fatherUsername: “HelloWorld”
}
});
#### 7.2.3、写法上的改进:驼峰
<template id="myTemplate">
<div>
<p v-for="item in subAddresses">{{item}}</p> // 拿数据依然是驼峰法方式
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 注册组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subAddresses: { // 驼峰命名
type: Array,
default: function(){
return [‘HelloWorld’ , “Spring”];
}
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
fatherAddresses: [
“河南林州”,
“浙江杭州”,
“云南大理”
]
}
});
### 7.3、子组件向父组件传递数据
**方式:通过自定义事件向父组件发送消息**
// 定义子组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
data: function(){
return {
person: {
username: ‘我是子组件HelloWorld’
}
}
},
methods: {
subCompClick(){
// 点击之后,子组件发射一个自定义的事件
this.$emit(“fn”,this.person);
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
username: “HelloWorld”
},
methods: {
fatherFn(obj){
console.log(obj);
}
}
});
**解决的思路是这样的:既然我们能做到父组件的data数据传递到子组件中,我们就可以实现将父组件的方法传递到子组件中。【实际上,虽然我们现在的确是在讲子组件向父组件传递数据,如果要按照这种思维方式去实现代码的话,不太好理解,我个人更推荐这种理解方式,即:现在不考虑子组件向父组件传递数据,我们就一律看做是:父组件向子组件传递数据,如果按照这种方式来去理解的话,写代码就会顺畅很多,通过此种方式去理解代码,写着写着就自然而然的就成了子组件向父组件传递数据了。】**==
**子组件向父组件传递数据/父组件向子组件传递方法,步骤总结如下:**
* 第一步
在父组件中定义一个方法。本案例中Vue实例作为父组件,自定义的方法是`fatherFn` 。
* 第二步
在应用子组件的标签上,去动态绑定一个事件。本案例中的事件是`fn`。
<my-component @fn=“fatherFn”>
代码的含义:就相当于是将父组件中的`fatherFn`方法的引用传递给子组件的`fn`事件函数。此时该`fn`函数肯定是需要在某个时刻要用到的。
* 第三步
既然我们是子组件向父组件传递数据,那么肯定是在子组件中做了一些操作,然后将数据传递给父组件。本案例中,在子组件`template`中有一个`button`按钮,该按钮的作用就是当点击的时候,向父组件传递数据。并且为该按钮绑定了一个事件,属于该按钮的事件,是`subCompClick` 。
* 第四步
在子组件中的`methods`属性中去定义`subCompClick`事件,当点击按钮的时候就会触发该事件,那么在
`subCompClick`事件中,操作是:调用`$emit`方法去发射我们的`fn`事件,并且通过该方法传递数据,而我们知道,我们的`fn`其实就是我们第一步骤中的`fatherFn` 。 然后在`fatherFn`函数中可以通过参数的形式去接收子组件传递的数据。
<template id="myTemplate">
<div>
<a href=""
v-for=“item in categories”
@click.prevent=“aClick(item)”>
{{item.name}}
<script src="../js/vue.js"></script>
<script type="text/javascript">
// 子组件
Vue.component(“myComponent”,{
template: “#myTemplate”,
data: function(){
return {
categories: [
{id: 1, name: “动作片”},
{id: 2, name: “爱情片”},
{id: 3, name: “悬疑片”},
{id: 4, name: “悬疑片”}
]
};
},
methods: {
aClick(item){
// 点击之后,子组件发射一个自定义的事件
this.$emit(“get-list-click”,item);
}
}
});
const app = new Vue({
el: “#myDiv”,
methods: {
fatherHandle(item){
console.info(“接收的信息是:” ,item);
}
}
});
### 7.4、案例
**该案例:子组件向父组件传数据综合运用。该案例是我们二期jQuery的一个案例:发表评论的案例。**
#### 7.4.1、第一步:先把数据给模拟出来
评论人: {{ item.user }} 内容: {{ item.content }} 发表日期: {{ item.pubDate }}
<script src="../js/vue.js"></script>
<script type="text/javascript">
const app = new Vue({
el: “#myDiv”,
data: {
list: [
{id: 1, user: ‘HelloWorld’, content: ‘美好的一天’, pubDate: new Date()},
{id: 2, user: ‘Spring’, content: ‘很高兴’, pubDate: new Date()}
]
}
});
#### 7.4.2、第二步:定义子组件,发表评论
<my-component></my-component>
<p v-for="item in list" :key="item.id">
评论人: {{ item.user }} 内容: {{ item.content }} 发表日期: {{ item.pubDate }}
</p>
</div>
<template id="myTemplate">
<div>
评论id: <input type="text"> <br/>
评论人: <input type="text"> <br/>
评论内容:<textarea></textarea><br/>
<input type="button" value="提交">
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
});
const app = new Vue({
el: “#myDiv”,
data: {
list: [
{id: 1, user: ‘HelloWorld’, content: ‘美好的一天’, pubDate: new Date()},
{id: 2, user: ‘Spring’, content: ‘很高兴’, pubDate: new Date()}
]
}
});
#### 7.4.3、第三步:点击发表评论
* 为表单控件绑定v-model,目的是获取数据
* 绑定的数据需要定义在子组件template的data中
* 为发表按钮提供单击事件
<my-component @fn="fatherFn"></my-component>
<p v-for="item in list" :key="item.id">
评论人: {{ item.user }} 内容: {{ item.content }} 发表日期: {{ item.pubDate }}
</p>
</div>
<template id="myTemplate">
<div>
评论id: <input type="text" v-model="id"> <br/>
评论人: <input type="text" v-model="user"> <br/>
评论内容:<textarea v-model="content"></textarea><br/>
<input type="button" value="发表" @click="publish">
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
data:function(){
return {id: ‘’ , user: ‘’ , content: ‘’}
},
methods: {
publish: function(){
// 发表评论业务处理
// 1、构造一个评论数据对象
let comment = {id: this.id, user: this.user , content: this.content, pubDate: new Date()};
// 2、需要将创建好的评论数据保存到父组件中的data中,涉及到了子组件向父组件传值
// >> 子组件发射一个自定义的事件
this.$emit(“fn”,comment);
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
list: [
{id: 1, user: ‘HelloWorld’, content: ‘美好的一天’, pubDate: new Date()},
{id: 2, user: ‘Spring’, content: ‘很高兴’, pubDate: new Date()}
]
},
methods: {
fatherFn(comment){
// 刚刚新增评论就插入到数组的头部
this.list.unshift(comment);
}
}
});
## 八、综合案例
**说明:该案例体现了父子组件相互传递数据。**
### 8.1、第一步:实现父组件数据传递给子组件
<template id="myTemplate">
<div>
通过props属性获取到的父组件中的数据:{{subusername}}---{{subage}}
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subusername: String,
subage: Number
}
});
const app = new Vue({
el: “#myDiv”,
data: {
username: ‘HelloWorld’,
age: 12
}
});
**说明:通过案例可以发现,子组件的确可以使用到了父组件中的数据。子组件可以使用props中的数据并显示到页面上,不过props中的数据是从父组件中获取的,是只读数据。**
### 8.2、第二步:看一个细节问题
在子组件中,定义两个`input`,实现双向绑定,绑定到了`props`属性的`subusername`和`subage`这两个属性,看下实验效果。
<template id="myTemplate">
<div>
通过props属性获取到的父组件中的数据:{{subusername}}---{{subage}} <br/>
双向绑定props属性中的subusername: <input type="text" v-model="subusername"><br/>
双向绑定props属性中的subage: <input type="text" v-model="subage"><br/>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subusername: String,
subage: Number
}
});
const app = new Vue({
el: “#myDiv”,
data: {
username: ‘HelloWorld’,
age: 12
}
});
**通过实验发现,该案例是有问题的,控制台会报错。**
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-UlBrOQmL-1690870417750)(/005.png)]
**结论:根据提示,避免去直接修改props属性中的数据,因为props属性中的数据都是通过父组件传递过来的,是只读的,避免去覆盖。取而代之的是,可以使用data和computed计算属性。**
### 8.3、第三步:使用data属性
<template id="myTemplate">
<div>
通过props属性获取到的父组件中的数据:{{subusername}}---{{subage}} <br/>
子组件data属性中的数据:{{data_subusername}}---{{data_subage}} <br/>
双向绑定data属性中的subusername: <input type="text" v-model="data\_subusername"><br/>
双向绑定data属性中的subage: <input type="text" v-model="data\_subage"><br/>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subusername: String,
subage: Number
},
data: function(){
return {
data_subusername: this.subusername,
data_subage: this.subage
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
username: ‘HelloWorld’,
age: 12
}
});
**使用data问题解决。但是还有一个需求就是:如果我想改变子组件中的文本框的数据,也想同步修改到父组件,让父组件也实现同步更新。这样的话,就涉及到了子组件向父组件传递数据,需要使用到自定义事件。**
### 8.4、第四步、给子组件的数据设置侦听器
需求:要想实现子组件的数据可以传递到父组件,也就是说子组件中的data数据如果发生了改变,那么父组件也可以感知到,则需要发射自定义事件来解决。此时,可以在子组件中为data中的属性设置侦听器来实现,当属性发生了修改,立马侦听到之后,再发送事件。
Vue.component(“myComponent”,{
template: “#myTemplate”,
props: {
subusername: String,
subage: Number
},
data: function(){
return {
data_subusername: this.subusername,
data_subage: this.subage
}
},
watch: {
data_subusername(newValue){
// 发生了修改,发送事件
this.KaTeX parse error: Expected 'EOF', got '}' at position 38: …e",newValue); }̲, data\_subage…emit(“subchangeage”,newValue);
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
username: ‘HelloWorld’,
age: 12
},
methods: {
fatherChangeUsername(value){
this.username = value;
},
fatherChangeAge(value){
this.age = parseInt(value);
}
}
});
## 九、父组件直接访问子组件
**使用方式:`$children`或者是`$refs`**
### 9.1、方式一:$children
Vue.component(“myComponent”,{
template: “#myTemplate”,
data: function(){
return {
username: “HelloWorld”
}
},
methods: {
info(){
console.info(“子组件的用户名:” + this.username);
}
}
});
const app = new Vue({
el: “#myDiv”,
methods: {
handleClick(){
// 单击父组件的按钮,执行该函数,目的是访问子组件中的数据和调用子组件中的方法
console.info(this.
c
h
i
l
d
r
e
n
)
;
/
/
返回的结果是一个数组
f
o
r
(
l
e
t
c
o
m
p
o
f
t
h
i
s
.
children); // 返回的结果是一个数组 for (let comp of this.
children);//返回的结果是一个数组for(letcompofthis.children){
console.info(comp.username);
comp.info();
}
}
}
});
**总结:此种方式使用不多,要获取具体的子组件还需要通过下标的方式来去获取,非常不方便。**
### 9.2、方式二:$refs
Vue.component(“myComponent”,{
template: “#myTemplate”,
data: function(){
return {
username: “HelloWorld”
}
},
methods: {
info(){
console.info(“子组件的用户名:” + this.username);
}
}
});
const app = new Vue({
el: “#myDiv”,
methods: {
handleClick(){
// 单击父组件的按钮,执行该函数,目的是访问子组件中的数据和调用子组件中的方法
console.log(this.
r
e
f
s
.
c
o
m
p
1.
u
s
e
r
n
a
m
e
)
;
t
h
i
s
.
refs.comp1.username); this.
refs.comp1.username);this.refs.comp1.info();
console.log(this.
r
e
f
s
.
c
o
m
p
2.
u
s
e
r
n
a
m
e
)
;
t
h
i
s
.
refs.comp2.username); this.
refs.comp2.username);this.refs.comp2.info();
}
}
});
**总结:这种方式类似于给组件起了一个id值,通过id的方式直接获取到了某个组件。通常,通过`$refs`来去获取Dom元素。**
HelloWorld
Spring
const app = new Vue({
el: “#myDiv”,
methods: {
handleClick(){
console.log(this.
r
e
f
s
.
p
1.
i
n
n
e
r
T
e
x
t
)
;
c
o
n
s
o
l
e
.
l
o
g
(
t
h
i
s
.
refs.p1.innerText); console.log(this.
refs.p1.innerText);console.log(this.refs.p2.innerText);
}
}
});
## 十、子组件直接访问父组件和根组件
**访问父组件使用方式:`$parent`**
**访问根组件使用方式:`$root`,也就是访问的Vue实例这个根组件**
<template id="myTemplate">
<div>
这个是子组件
<input type="button" value="访问父组件" @click="subHandleClick">
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
methods: {
subHandleClick(){
// 访问父组件
console.log(this.
p
a
r
e
n
t
.
m
e
s
s
a
g
e
)
;
t
h
i
s
.
parent.message); this.
parent.message);this.parent.fatherInfo();
// 访问根组件
console.log(this.
r
o
o
t
.
m
e
s
s
a
g
e
)
;
t
h
i
s
.
root.message); this.
root.message);this.root.fatherInfo();
}
}
});
const app = new Vue({
el: “#myDiv”,
data: {
message: ‘Spring’
},
methods: {
fatherInfo(){
console.info(“父组件的信息:” + this.message);
}
}
});
## 十一、插槽
### 11.1、说明
插槽,其实就相当于占位符。它在组件中给你的HTML模板占了一个位置,让你来传入一些东西。决定将所携带的内容,插入到指定的某个位置,从而使模板分块,具有模块化的特质和更大的重用性。
**插槽显不显示、怎样显示是由父组件来控制的,而插槽在哪里显示就由子组件来进行控制。**
**适用场景:是那些可以将多个组件看做一个整体,这个整体会被复用。但其中的一些部分内容不固定。**
### 11.2、HelloWorld案例
**需求:我现在有一个div,是一个子组件,里面有公共的代码,就是p标签,但是这个子组件在不同页面上所展示的效果还是有细微区别的,可能A页面是一个button按钮,B页面可能是一个p标签。**
#### 11.2.1、简单案例
这个是p标签
<template id="myTemplate">
<div>
<p>我是子组件</p>
<slot></slot> // 相当于是占位符
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
});
const app = new Vue({
el: “#myDiv”
});
#### 11.2.2、改进:为插槽指定默认内容
这个是p标签
<template id="myTemplate">
<div>
<p>我是子组件</p>
<slot>
<button>这个是按钮,为插槽指定默认内容</button>
</slot>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
});
const app = new Vue({
el: “#myDiv”
});
**总结:该案例是在`slot`插槽中设置了一个`button`按钮,相当于是一个默认值,那么此时,在使用该组件的时候,可以不传入了,那么就用默认值`button`,如果传入了,则就用指定的传入Dom模板。**
### 11.3、具名插槽
说明:如果在一个组件内有多个插槽,如何为指定的插槽填充内容呢,此时就需要为每个插槽提供一个名字,这种插槽就叫做具名插槽。
#### 11.3.1、问题案例
此处是P标签
Tomcat
我是子组件
HelloWorld
**总结:会发现,组件中的`slot`插槽中的内容都被`button`和`p`所替代了。**
#### 11.3.2、解决方案一
此处是P标签
<template id="myTemplate">
<div>
<slot name="slot1"><p>Tomcat</p></slot>
<p>我是子组件</p>
<slot name="slot2"><p>HelloWorld</p></slot>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
});
const app = new Vue({
el: “#myDiv”
});
#### 11.3.3、 解决方案二
此处是P标签
<template id="myTemplate">
<div>
<slot name="slot1"><p>Tomcat</p></slot>
<p>我是子组件</p>
<slot name="slot2"><p>HelloWorld</p></slot>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component(“myComponent”,{
template: “#myTemplate”,
});
const app = new Vue({
el: “#myDiv”
});
### 最后
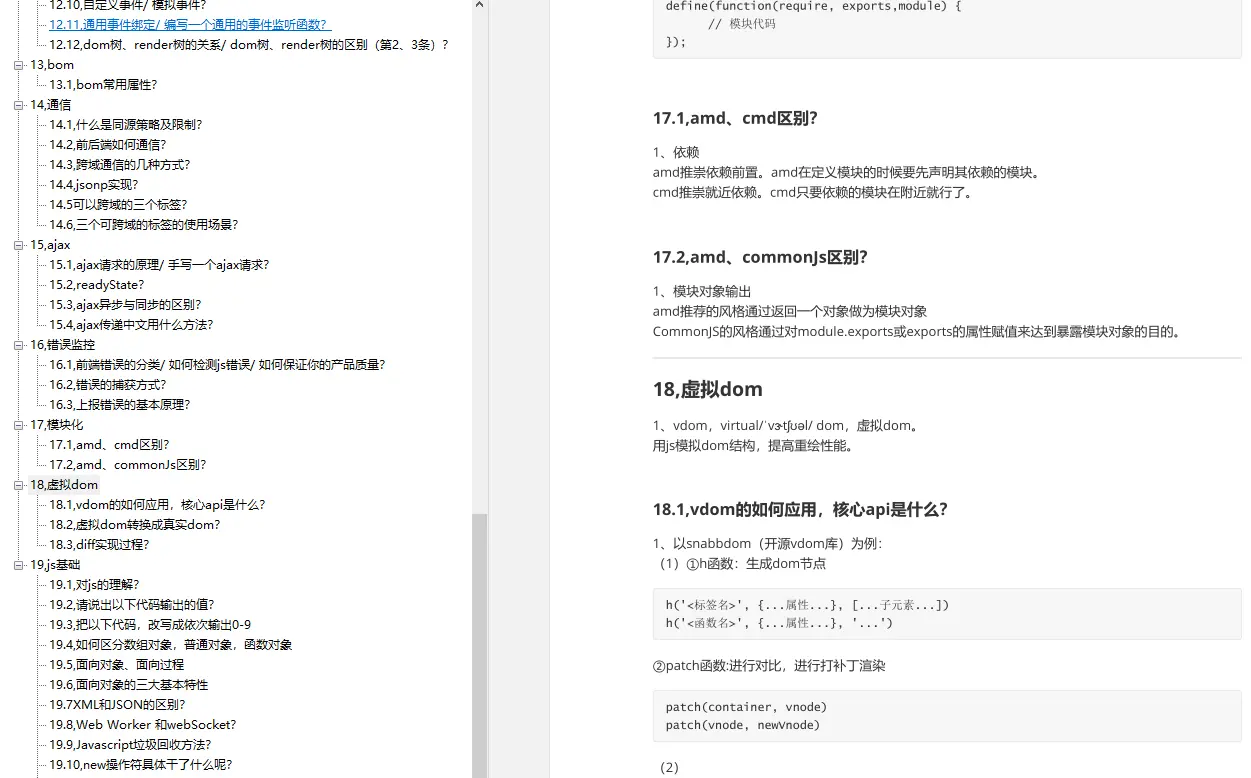
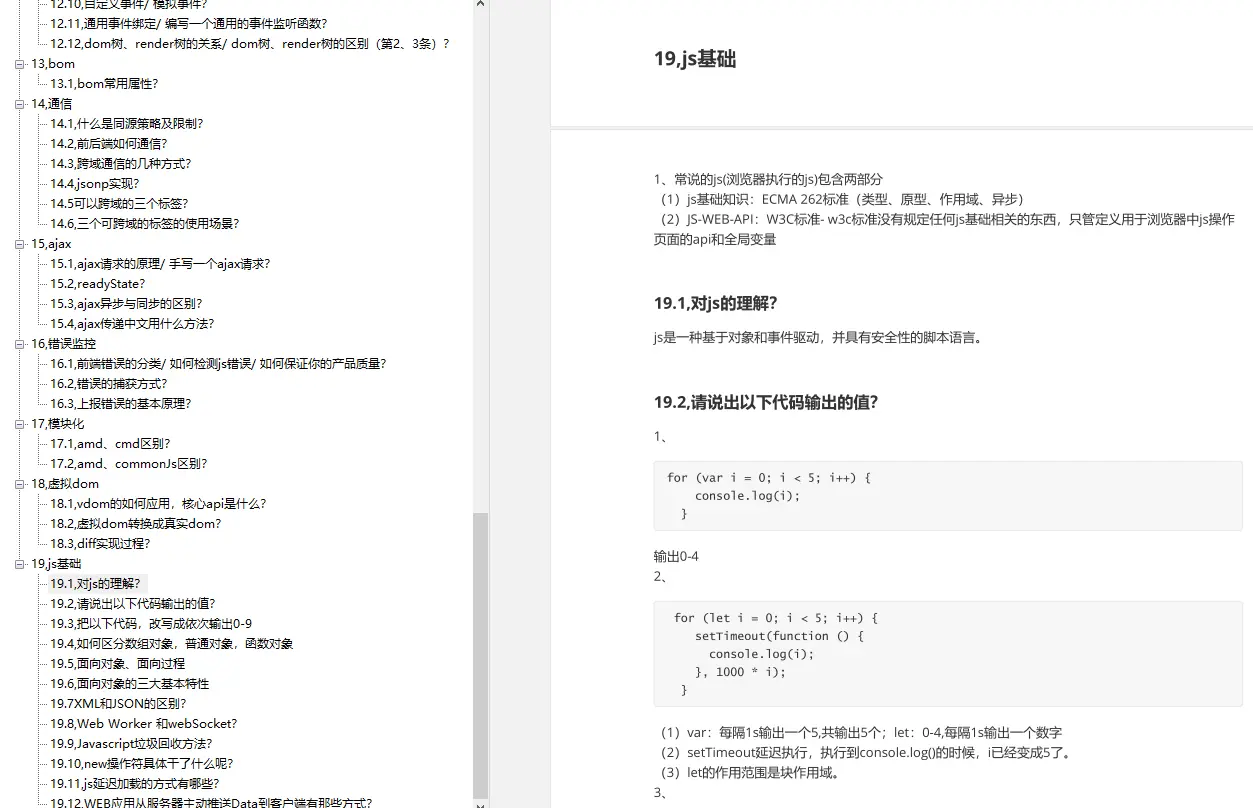
>由于文档内容过多,为了避免影响到大家的阅读体验,在此只以截图展示部分内容
>
dy>
<div id="myDiv">
<my-component>
<button slot="slot1">此处替换为按钮</button>
<p>此处是P标签</p>
</my-component>
</div>
<template id="myTemplate">
<div>
<slot name="slot1"><p>Tomcat</p></slot>
<p>我是子组件</p>
<slot name="slot2"><p>HelloWorld</p></slot>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component("myComponent",{
template: "#myTemplate",
});
const app = new Vue({
el: "#myDiv"
});
</script>
</body>
11.3.3、 解决方案二
<body>
<div id="myDiv">
<my-component>
<template v-slot:slot1>
<button>此处替换为按钮</button>
</template>
<p>此处是P标签</p>
</my-component>
</div>
<template id="myTemplate">
<div>
<slot name="slot1"><p>Tomcat</p></slot>
<p>我是子组件</p>
<slot name="slot2"><p>HelloWorld</p></slot>
</div>
</template>
<script src="../js/vue.js"></script>
<script type="text/javascript">
Vue.component("myComponent",{
template: "#myTemplate",
});
const app = new Vue({
el: "#myDiv"
});
</script>
</body>
最后
[外链图片转存中…(img-TBINGBta-1718864360648)]
[外链图片转存中…(img-AoMf3wqp-1718864360650)]
由于文档内容过多,为了避免影响到大家的阅读体验,在此只以截图展示部分内容