网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
System.out.println("this is my test");
}
}
实际效果如下,
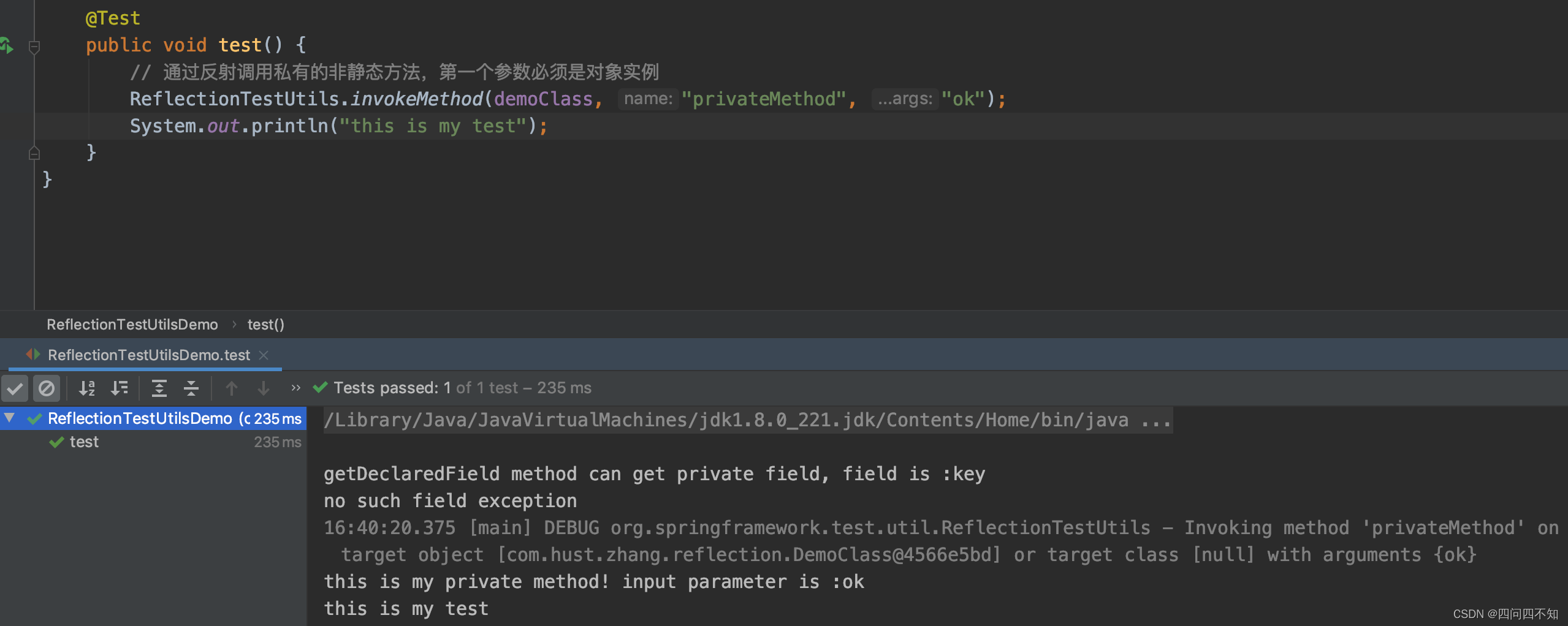
注意Class类的getDeclaredField才可以获取私有成员变量,getField方法只能获取公有成员变量。
## 代码解析
直接看org.springframework.test.util.ReflectionTestUtils类下面的invokeMethod方法,如下,
首先对目标对象断言不为空,只有对象不为null才会继续执行invokeMethod方法。
@Nullable
public static <T> T invokeMethod(Object target, String name, Object... args) {
Assert.notNull(target, "Target object must not be null");
return invokeMethod(target, (Class)null, name, args);
}
invokeMethod的重载方法步骤如下,
* 1)判断目标对象或对象类不为null,且传进来的方法命不为空;
* 2)创建MethodInvoker实例对象,并把目标对象或目标类,目标方法,参数等信息set到MethodInvoker对象中;
* 3)调用MethodInvoker的prepare方法,这样后续可以多次直接调用反射获取的方法;
* 4)调用MethodInvoker的invoke方法,执行方法。
@Nullable
public static T invokeMethod(@Nullable Object targetObject, @Nullable Class<?> targetClass, String name, Object… args) {
Assert.isTrue(targetObject != null || targetClass != null, “Either ‘targetObject’ or ‘targetClass’ for the method must be specified”);
Assert.hasText(name, “Method name must not be empty”);
try {
MethodInvoker methodInvoker = new MethodInvoker();
methodInvoker.setTargetObject(targetObject);
if (targetClass != null) {
methodInvoker.setTargetClass(targetClass);
}
methodInvoker.setTargetMethod(name);
methodInvoker.setArguments(args);
methodInvoker.prepare();
if (logger.isDebugEnabled()) {
logger.debug(String.format("Invoking method '%s' on %s or %s with arguments %s", name, safeToString(targetObject), safeToString(targetClass), ObjectUtils.nullSafeToString(args)));
}
return methodInvoker.invoke();
} catch (Exception var5) {
ReflectionUtils.handleReflectionException(var5);
throw new IllegalStateException("Should never get here");
}
}
下面看看prepare方法做了什么,
* 1)判断MethodInvoker对象传入的静态方法名是否不为空;
* 2)拿到MethodInvoker对象传入的目标对象和目标方法,并断言不为空;
* 3)获取参数集合和参数的类型;
* 4)通过目标类的getMethod方法找父类方法或接口方法,找不到则通过MethodInvoker对象的findMatchingMethod方法,再找不到则抛异常;
public void prepare() throws ClassNotFoundException, NoSuchMethodException {
if (this.staticMethod != null) {
int lastDotIndex = this.staticMethod.lastIndexOf(‘.’);
if (lastDotIndex == -1 || lastDotIndex == this.staticMethod.length()) {
throw new IllegalArgumentException(
"staticMethod must be a fully qualified class plus method name: " +
“e.g. ‘example.MyExampleClass.myExampleMethod’”);
}
String className = this.staticMethod.substring(0, lastDotIndex);
String methodName = this.staticMethod.substring(lastDotIndex + 1);
this.targetClass = resolveClassName(className);
this.targetMethod = methodName;
}
Class<?> targetClass = getTargetClass();
String targetMethod = getTargetMethod();
Assert.notNull(targetClass, "Either 'targetClass' or 'targetObject' is required");
Assert.notNull(targetMethod, "Property 'targetMethod' is required");
Object[] arguments = getArguments();
Class<?>[] argTypes = new Class<?>[arguments.length];
for (int i = 0; i < arguments.length; ++i) {
argTypes[i] = (arguments[i] != null ? arguments[i].getClass() : Object.class);
}
// Try to get the exact method first.
try {
this.methodObject = targetClass.getMethod(targetMethod, argTypes);
}
catch (NoSuchMethodException ex) {
// Just rethrow exception if we can't get any match.
this.methodObject = findMatchingMethod();
if (this.methodObject == null) {
throw ex;
}
}
}
继续往下挖,看看targetClass.getMethod怎么获取方法对象的,
* 1)检查是否允许客户端访问成员;
* 2)从getMethod0方法中去找父类方法或接口方法;
* 3)找不到则抛出NoSuchMethodException异常,找到则返回方法;
@CallerSensitive
public Method getMethod(String name, Class<?>... parameterTypes)
throws NoSuchMethodException, SecurityException {
checkMemberAccess(Member.PUBLIC, Reflection.getCallerClass(), true);
Method method = getMethod0(name, parameterTypes, true);
if (method == null) {
throw new NoSuchMethodException(getName() + "." + name + argumentTypesToString(parameterTypes));
}
return method;
}
MethodInvoker对象的findMatchingMethod方法,
* 1)获取目标方法、参数、参数长度;
* 2)获取目标类,断言不为null,并通过getAllDeclaredMethods方法获取目标类的所有方法;
* 3)遍历目标类中的所有方法,找到匹配的方法;
@Nullable
protected Method findMatchingMethod() {
String targetMethod = getTargetMethod();
Object[] arguments = getArguments();
int argCount = arguments.length;
Class<?> targetClass = getTargetClass();
Assert.state(targetClass != null, "No target class set");
Method[] candidates = ReflectionUtils.getAllDeclaredMethods(targetClass);
int minTypeDiffWeight = Integer.MAX_VALUE;
Method matchingMethod = null;
for (Method candidate : candidates) {
if (candidate.getName().equals(targetMethod)) {
if (candidate.getParameterCount() == argCount) {
Class<?>[] paramTypes = candidate.getParameterTypes();
int typeDiffWeight = getTypeDifferenceWeight(paramTypes, arguments);
if (typeDiffWeight < minTypeDiffWeight) {
minTypeDiffWeight = typeDiffWeight;
matchingMethod = candidate;
}
}
}
}
return matchingMethod;
}
再看方法的调用是如何实现的,找到MethodInvoker对象的invoke方法,
1)获取目标对象和提前准备好的方法;
2)如果目标对象为null或者目标方法是静态方法则抛出IllegalArgumentException异常;
3)使给定的非静态方法可访问setAccessible=true;
4)调用目标方法Method的invoke方法;
@Nullable
public Object invoke() throws InvocationTargetException, IllegalAccessException {
// In the static case, target will simply be {@code null}.
Object targetObject = getTargetObject();
Method preparedMethod = getPreparedMethod();
if (targetObject == null && !Modifier.isStatic(preparedMethod.getModifiers())) {
throw new IllegalArgumentException("Target method must not be non-static without a target");
}
ReflectionUtils.makeAccessible(preparedMethod);
return preparedMethod.invoke(targetObject, getArguments());
}
Method的invoke方法如下,
* 1)如果是覆写的方法,调用Reflection.getCallerClass()的native方法获取调用者的类,并对调用者的类和目标对象进行检查;
* 2)获取MethodAccessor对象,如果当前Method没有,则在根节点root不为null时从根节点获取MethodAccessorImpl对象,否则调用反射工厂的newMethodAccessor;
* 3)调用MethodAccessorImpl对象(MethodAccessor接口的实现类)的invoke方法;
@CallerSensitive
public Object invoke(Object obj, Object... args)
throws IllegalAccessException, IllegalArgumentException,
InvocationTargetException
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
6)]
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!