app:layout_constraintHorizontal_bias=“1.0”
app:layout_constraintStart_toStartOf=“parent”
app:layout_constraintTop_toTopOf=“parent”
app:layout_constraintVertical_bias=“0.0”
app:srcCompat=“@drawable/image” />
<Button
android:id=“@+id/convert_button”
android:layout_width=“363dp”
android:layout_height=“63dp”
android:text=“CONVERT”
app:layout_constraintBottom_toBottomOf=“parent”
app:layout_constraintEnd_toEndOf=“parent”
app:layout_constraintStart_toStartOf=“parent”
app:layout_constraintTop_toTopOf=“parent” />
<TextView
android:id=“@+id/result_text”
android:layout_width=“348dp”
android:layout_height=“121dp”
android:text=“TextView”
android:textAlignment=“center”
android:textSize=“34sp”
app:layout_constraintBottom_toBottomOf=“parent”
app:layout_constraintEnd_toEndOf=“parent”
app:layout_constraintHorizontal_bias=“0.38”
app:layout_constraintStart_toStartOf=“parent”
app:layout_constraintTop_toTopOf=“parent”
app:layout_constraintVertical_bias=“0.693” />
// 按钮的转换操作
converbutton.setOnClickListener((v) ->{
String input = edittext.getText().toString();
double value = Double.parseDouble(input);
double result = value * miToFT;
resulttext.setText(String.format(“%.2f英尺”,result));
resulttext.setVisibility(View.VISIBLE);
});
---
**基本效果图**
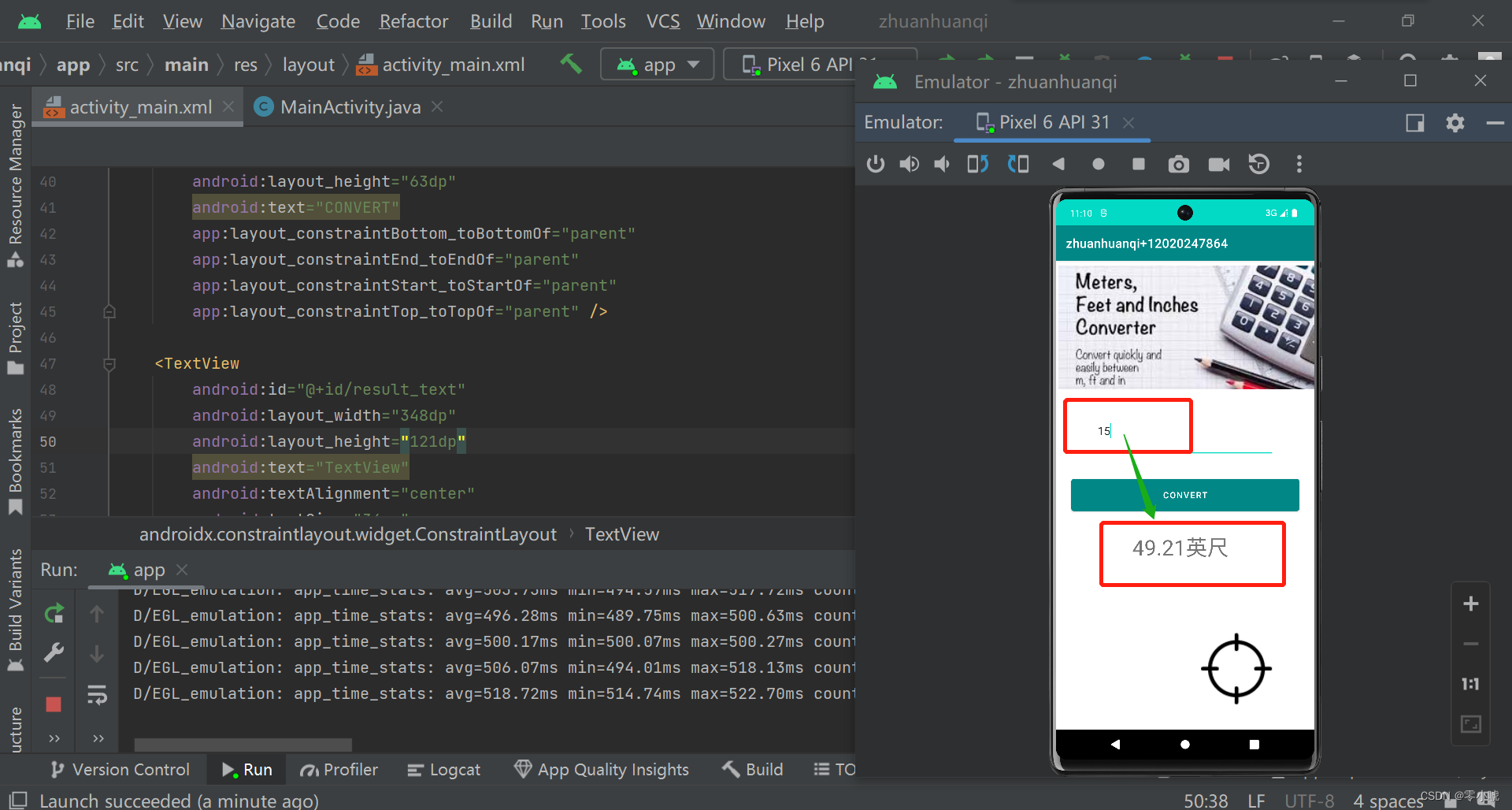
`说明`:实验要求完成的部分基本完成,只是简单的实现单位的转换功能,右下角的那个图片属于拓展部分的,适用于通过点击图片实现为页面添加背景颜色的功能,下面就简单说一下怎么实现这一功能吧。
---
**拓展部分简单分析**
如果需求是文本图片下方显示一段红色的固定背景,在用户点击图片时,背景不需要变化,可以采用以下步骤实现:
* `在原有的项目基础上,添加一个ImageView和一个TextView控件。选定需要带有红色背景的TextView,将其位置设置在ImageView的下方。`
* `在Java代码中,为TextView控件设置背景颜色。`
* `将TextView的背景颜色设置为红色,以显示红色的背景。`
---
**相关代码的实现**
<TextView
android:id=“@+id/result_text”
android:layout_width=“348dp”
android:layout_height=“121dp”
android:text=“TextView”
android:textAlignment=“center”
android:textSize=“34sp”
app:layout_constraintBottom_toBottomOf=“parent”
app:layout_constraintEnd_toEndOf=“parent”
app:layout_constraintHorizontal_bias=“0.38”
app:layout_constraintStart_toStartOf=“parent”
app:layout_constraintTop_toTopOf=“parent”
app:layout_constraintVertical_bias=“0.693” />
<ImageView
android:id=“@+id/imageView3”
android:layout_width=“200dp”
android:layout_height=“141dp”
app:layout_constraintBottom_toBottomOf=“parent”
app:layout_constraintEnd_toEndOf=“parent”
app:layout_constraintHorizontal_bias=“0.886”
app:layout_constraintStart_toStartOf=“parent”
app:layout_constraintTop_toTopOf=“@+id/imageView2”
app:layout_constraintVertical_bias=“0.957”
app:srcCompat=“@drawable/image1” />
imageView.setOnClickListener((V)->{
int color = Color.argb(128,255,0,0);
mainview.setBackgroundColor(color);
});
`代码说明`:找到需要设置背景色的控件,然后使用Color.argb方法生成一个Argb颜色值,其中`第一个参数128指定了颜色的透明度为50%`(255是不透明,0是全透明),`后面三个参数分别为红、绿、蓝的颜色值`。`最后使用setBackgroundColor方法将颜色值设置为控件的背景色`即可,需要注意的是,不同的设备可能会有不同的透明度支持,因此需要进行实测调试,以保证透明效果符合需求。
---
**基本实现效果图**
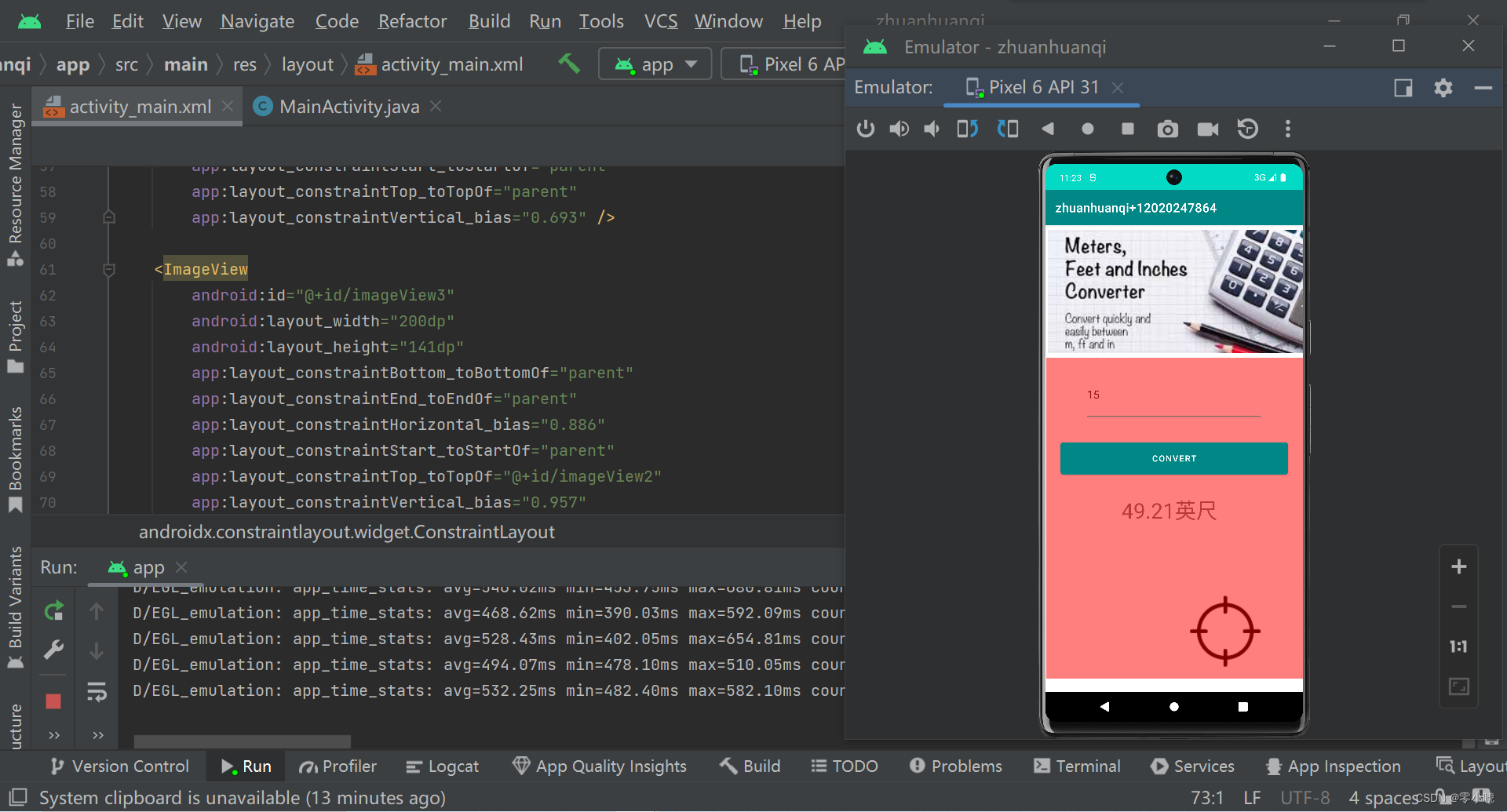
---
`再次申明本次实验是自己的Android Studio实验作业,题目出自帅帅老师,上面的只是部分核心代码的实现,很简单,因为自己也是第一次学习Android Studio,本身Java基础就不怎么好,上面的代码仅供参考,基本的bug已经修复,运行是没有问题的。有问题或者代码还有可以改进的地方可以一起学习交流,欢迎大佬指正~`
---
**bug问题1描述**
>
> 上面的实现过程中有一个问题不知道大家有没有发现,这个也是我调试多次后发现这个地方可以改进一下,就是那个输入文本框中的内容,在用户每次输入数值的时候,都必须将里面的提示内容全部清除掉才可以进行输入新的内容,所以显得很麻烦,理想的状态应该是用户通过点击输入文本框的时候,就会自动将里面的提示内容隐藏掉,直接输入新的内容。
>
>
>
---
**修改bug2的过程**
<EditText
android:id=“@+id/edit_text”
android:layout_width=“286dp”
android:layout_height=“85dp”
android:ems=“10”
android:inputType=“text”
android:hint=“Enter meters here”
app:layout_constraintBottom_toBottomOf=“parent”
app:layout_constraintEnd_toEndOf=“parent”
app:layout_constraintHorizontal_bias=“0.497”
app:layout_constraintStart_toStartOf=“parent”
app:layout_constraintTop_toTopOf=“parent”
app:layout_constraintVertical_bias=“0.346” />
`说明`:其中,`android:hint属性表示提示文本的内容`,可以根据需求自行修改。此外,`android:inputType属性指定了EditText控件的输入类型`。在上述代码中,输入类型设置为text,表示接受任意文本输入。可以根据需求设置对应的输入类型,例如数字、日期、邮箱等。当用户点击EditText控件时,提示文本会自动隐藏,用户可以直接在输入框内输入内容。需要注意的是,在设置EditText控件的输入类型时,可能会受到输入法的影响,不同的输入法可能会有不同的输入效果。为避免输入错误,建议在需要输入特定类型的文本时,通过设置输入类型及相关的输入限制,来确保输入的正确性。
---
**bug问题2描述**
>
> 前一个问题就是一个简单的小问题,大不了用户删除内容重新进行修改就可以了,但是还有一个致命的问题就是在输入文本框中,当用户输入直接的数字,运行是没有问题的,但是当用户输入12米或者13m的时候,程序就会闪崩,既然提前注意到了,下面是自己尝试修改的过程。
>
>
>
---
**修改bug2的过程**
converbutton.setOnClickListener((v) ->{
String input = edittext.getText().toString();
String regex = "\\d+(\\.\\d+)?";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(input);
while (matcher.find()) {
String numberStr = matcher.group();
double number = Double.parseDouble(numberStr);
double result = number \* miToFT;
resulttext.setText(String.format("%.2f英尺", result));
resulttext.setVisibility(View.VISIBLE);
最后
由于题目很多整理答案的工作量太大,所以仅限于提供知识点,详细的很多问题和参考答案我都整理成了 PDF文件
esulttext.setVisibility(View.VISIBLE);
最后
由于题目很多整理答案的工作量太大,所以仅限于提供知识点,详细的很多问题和参考答案我都整理成了 PDF文件
[外链图片转存中…(img-rR0XTalZ-1719206700022)]
[外链图片转存中…(img-bKygKRQO-1719206700022)]
[外链图片转存中…(img-vWOWpM3w-1719206700023)]