starttls:
enable: true
required: true
#### **4. 定义发送邮件的服务类**
**定义邮件发送接口IMailService**
package com.yyg.boot.mail;
/**
-
@Author 一一哥Sun
-
@Date Created in 2020/4/20
-
@Description 封装一个发邮件的接口,方便后边直接调用.
*/
public interface IMailService {/**
- 发送文本邮件
- @param to 收件人
- @param subject 主题
- @param content 内容
*/
void sendSimpleMail(String to, String subject, String content);
/**
- 发送HTML邮件
- @param to 收件人
- @param subject 主题
- @param content 内容
*/
void sendHtmlMail(String to, String subject, String content);
/**
- 发送带附件的邮件
- @param to 收件人
- @param subject 主题
- @param content 内容
- @param filePath 附件
*/
void sendAttachmentsMail(String to, String subject, String content, String filePath);
/**
- 发送模板邮件
- @param to 收件人
- @param subject 主题
- @param fileName 邮件模板文件名称
- @param model 邮件数据载体
*/
void sendModelMail(String to, String subject, String fileName, Object model);
}
**定义邮件发送实现类IMailServiceImpl**
package com.yyg.boot.mail.impl;
import com.yyg.boot.mail.IMailService;
import freemarker.template.Configuration;
import freemarker.template.Template;
import freemarker.template.TemplateException;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.FileSystemResource;
import org.springframework.mail.SimpleMailMessage;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import org.springframework.ui.freemarker.FreeMarkerTemplateUtils;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import java.io.IOException;
import java.util.Objects;
/**
-
@Author 一一哥Sun
-
@Date Created in 2020/4/20
-
@Description Description
*/
@Slf4j
@Service
public class IMailServiceImpl implements IMailService {/**
- Spring Boot 提供了一个发送邮件的简单抽象,使用的是下面这个接口,这里直接注入即可使用
*/
@Autowired
private JavaMailSender mailSender;
@Autowired
private Configuration configuration;/**
- 配置文件中我的qq邮箱
*/
@Value(“${spring.mail.from}”)
private String from;
/**
- 简单文本邮件
- @param to 收件人
- @param subject 主题
- @param content 内容
*/
@Override
public void sendSimpleMail(String to, String subject, String content) {
//创建SimpleMailMessage对象
SimpleMailMessage message = new SimpleMailMessage();
//邮件发送人
message.setFrom(from);
//邮件接收人
message.setTo(to);
//邮件主题
message.setSubject(subject);
//邮件内容
message.setText(content);
//发送邮件
mailSender.send(message);
}
/**
- html邮件
- @param to 收件人
- @param subject 主题
- @param content 内容
*/
@Override
public void sendHtmlMail(String to, String subject, String content) {
//获取MimeMessage对象
MimeMessage message = mailSender.createMimeMessage();
MimeMessageHelper messageHelper;
try {
messageHelper = new MimeMessageHelper(message, true);
//邮件发送人
messageHelper.setFrom(from);
//邮件接收人
messageHelper.setTo(to);
//邮件主题
message.setSubject(subject);
//邮件内容,html格式
messageHelper.setText(content, true);
//发送
mailSender.send(message);
//日志信息
log.info(“邮件已经发送…”);
} catch (MessagingException e) {
log.error(“发送邮件时发生异常!”, e);
}
}
/**
-
带附件的邮件
-
@param to 收件人
-
@param subject 主题
-
@param content 内容
-
@param filePath 附件
*/
@Override
public void sendAttachmentsMail(String to, String subject, String content, String filePath) {
MimeMessage message = mailSender.createMimeMessage();
try {
MimeMessageHelper helper = new MimeMessageHelper(message, true);
helper.setFrom(from);
helper.setTo(to);
helper.setSubject(subject);
helper.setText(content, true);//FileSystemResource file = new FileSystemResource(new File(filePath)); ClassPathResource resource = new ClassPathResource(filePath); FileSystemResource file = new FileSystemResource(resource.getFile()); helper.addAttachment(Objects.requireNonNull(file.getFilename()), file); //可以同时添加多个附件,只需要在这里直接添加第2,第3...附件就行了. //helper.addAttachment(fileName2, file2); mailSender.send(message); //日志信息 log.info("邮件已经发送...");
} catch (MessagingException e) {
log.error(“发送邮件时发生异常!”, e);
} catch (IOException e) {
e.printStackTrace();
log.error(“发送邮件时发生异常!”, e);
}
}
@Override
public void sendModelMail(String to, String subject, String fileName, Object model) {
MimeMessage mimeMessage = mailSender.createMimeMessage();
try {
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, true);
helper.setFrom(from);
helper.setTo(to);
helper.setSubject(subject);Template template = configuration.getTemplate(fileName); String html = FreeMarkerTemplateUtils.processTemplateIntoString(template, model); helper.setText(html, true); mailSender.send(mimeMessage); //日志信息 log.info("邮件已经发送..."); } catch (MessagingException e) { log.error("发送邮件时发生异常!", e); } catch (TemplateException e) { e.printStackTrace(); log.error("发送邮件时发生异常!", e); } catch (IOException e) { e.printStackTrace(); }
}
- Spring Boot 提供了一个发送邮件的简单抽象,使用的是下面这个接口,这里直接注入即可使用
}
#### **5. 定义实体类**
package com.yyg.boot.entity;
import lombok.Data;
import java.util.Date;
/**
-
@Author 一一哥Sun
-
@Date Created in 2020/4/20
-
@Description Description
*/
@Data
public class Employee {/**
- 员工编号
*/
private Long id;
/**
- 员工名称
*/
private String username;
/**
- 合同期限
*/
private Integer contractTerm;
/**
- 员工薪水
*/
private Double salary;
/**
- 合同起始日期
*/
private Date beginContract;
/**
- 合同截至日期
*/
private Date endContract;
/**
- 部门名称
*/
private String departmentName;
/**
- 职位名称
*/
private String posName;
- 员工编号
}
#### **6. 创建入口类**
package com.yyg.boot;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
/**
-
@Author 一一哥Sun
-
@Date Created in 2020/4/20
-
@Description Description
*/
@SpringBootApplication
public class MailApplication {public static void main(String[] args){
SpringApplication.run(MailApplication.class,args);
}
}
#### **7. 创建Controller接口**
**7.1 定义发送简单邮件的接口方法:**
@GetMapping(“/simple”)
public String sendSimpleMail() {
iMailService.sendSimpleMail(“收件箱@qq.com”, “邮件标题”, “邮件内容…机密”);
return “success”;
}
**启动程序进行测试**
邮件发送成功:
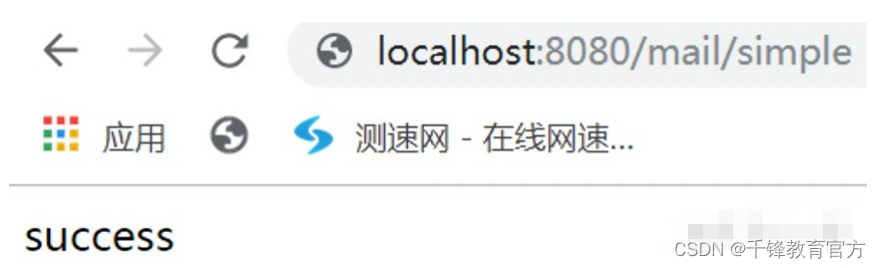
去目标邮箱的收件箱中进行查看,可以看到如下邮件内容,说明邮件发送成功!
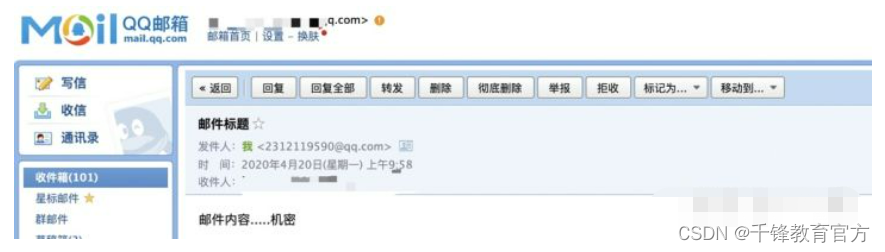
**7.2定义发送html格式邮件的接口方法:**
@GetMapping(“/html”)
public String sendHtmlMail() {
iMailService.sendHtmlMail(“收件箱@qq.com”, “邮件主题”, “
邮件主题
邮件内容
”);return “success”;
}
邮件发送成功:
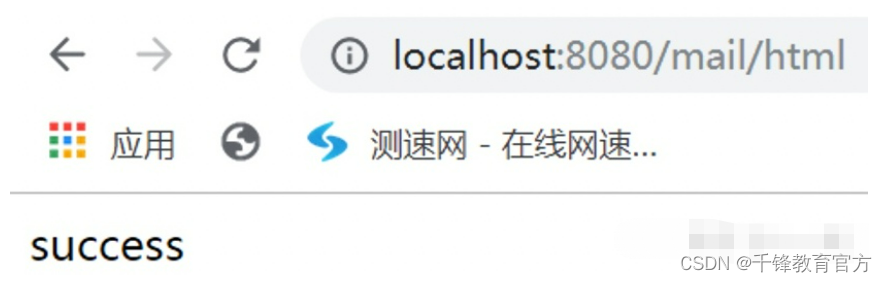
去目标邮箱的收件箱中进行查看,可以看到如下邮件内容,说明邮件发送成功!
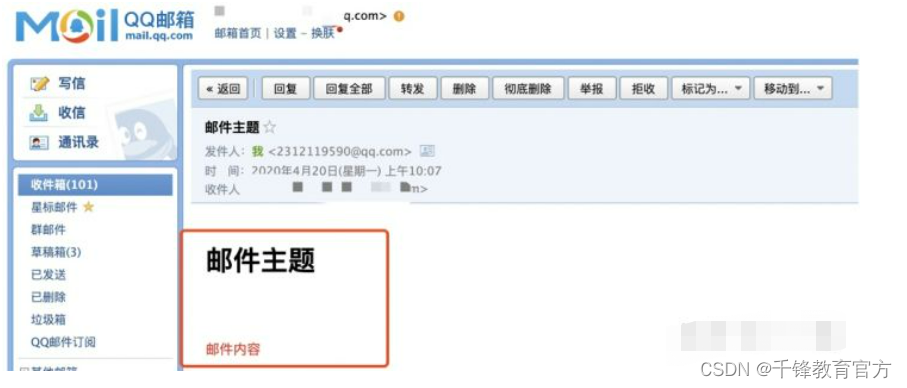
**7.3创建发送带附件的邮件接口方法:**
@GetMapping(“/attachment”)
public String sendAttachmentMail() {
iMailService.sendAttachmentsMail(“收件箱@qq.com”, “主题:带附件的邮件”, “有附件的邮件,不要错过哦…”, “static/touxiang.png”);
return “success”;
}
#### **注意:**
我这里是把附件直接放到了项目的resource/static目录下了,我们也可以存放到桌面等位置。
邮件发送成功:
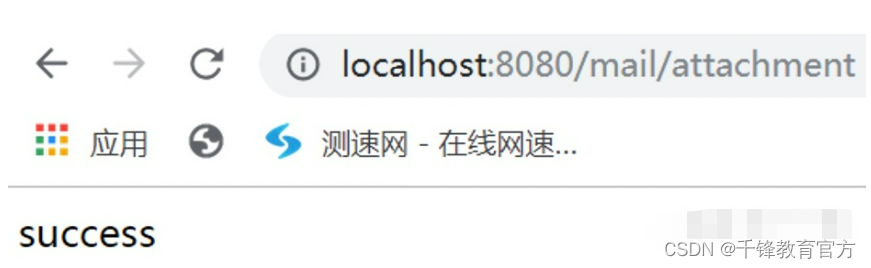
然后去目标邮箱的收件箱中进行查看,可以看到如下邮件内容,说明邮件发送成功!
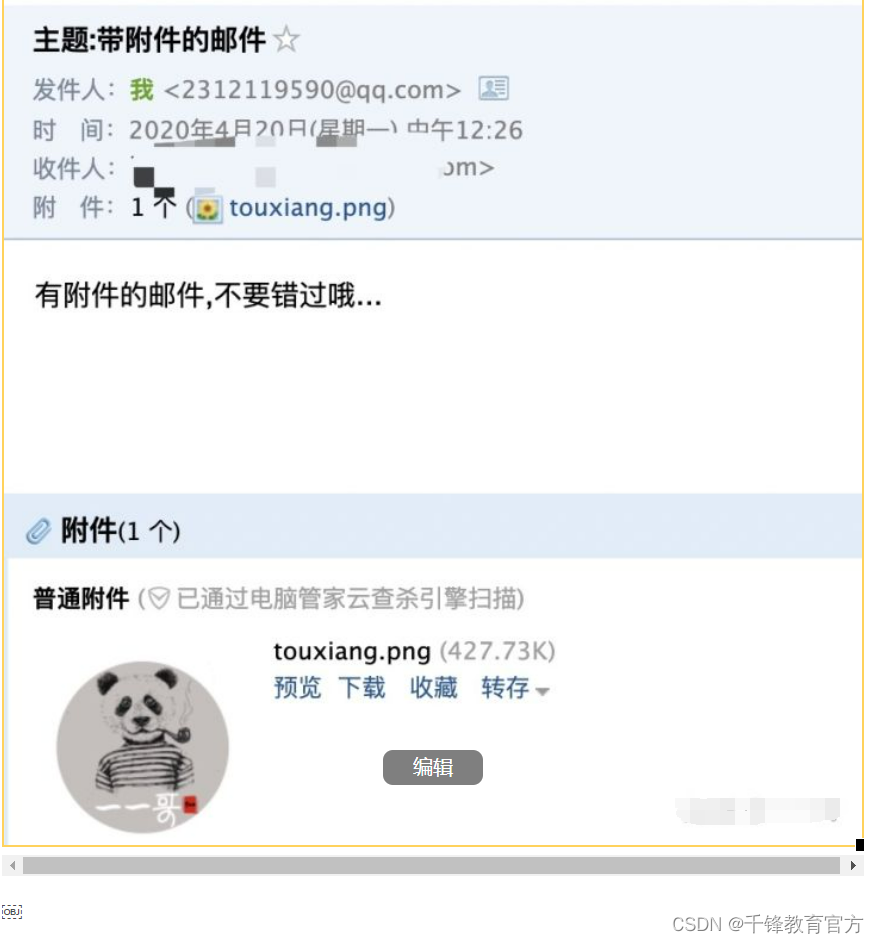
**7.4创建发送模板邮件的接口方法:**
首先利用FreeMarker创建页面模板。
${username}--你好,欢迎加入XXX大家庭!您的入职信息如下:
工号 | ${id} |
合同期限 | ${contractTerm}年 |
员工薪资 | ${salary}/月(美元) |
合同起始日期 | ${beginContract?string("yyyy-MM-dd")} |
合同截至日期 | ${endContract?string("yyyy-MM-dd")} |
所属部门 | ${departmentName} |
职位 | ${posName} |
希望在未来的日子里,携手共进!
```别忘了在application.yml文件中对freemarker进行配置:
### 最后
**文章中涉及到的知识点我都已经整理成了资料,录制了视频供大家下载学习,诚意满满,希望可以帮助在这个行业发展的朋友,在论坛博客等地方少花些时间找资料,把有限的时间,真正花在学习上,所以我把这些资料,分享出来。相信对于已经工作和遇到技术瓶颈的朋友们,在这份资料中一定都有你需要的内容。**