}
}
这样的服务器代码很简单,理论上也是正确的,但是在实现的环境中却很糟糕,因为它每次只能处理一个请求,如果每个请求的耗时过长,后面的请求会长时间等待或者超时错误。
于是乎,出现了下面这种写法:
###### 2、每个请求分配一个线程的服务器
package com.zhy.concurrency.executors;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
/**
-
为每个请求分配一个线程
-
@author zhy
*/
public class TaskPreThreadServer
{
public static void main(String[] args) throws IOException
{
ServerSocket server = new ServerSocket(8811);
while (true)
{
final Socket client = server.accept();
new Thread()
{
public void run()
{
handleReq(client);
};
}.start();
}
}
protected static void handleReq(Socket client)
{
}
}
为每一个请求开辟一个线程,首先我得承认我也经常用这样的写法~但是我们还是要吐槽下这样写法的不足:
a、线程的生命周期的开销还是相当高的,大量的线程的创建将消耗大量的计算机资源
b、可创建线程的数量存在一个限制值(这个值由平台觉得,且受很多因素的制约),如果超过这个限制,可能会报OOM错误
c、在一定范围内,增加线程可以提高系统吞吐量,但是超过了这个范围,就物极必反了,只会降低程序的执行速度。
所以我们要继续改进我们的服务器代码,Executor提供了非常方便的方式:
###### 3、基于Executor的服务器
package com.zhy.concurrency.executors;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.concurrent.Executor;
import java.util.concurrent.Executors;
/**
-
基于Executor的服务器
-
@author zhy
*/
public class TaskExecutionServer
{
private static final int THREAD_COUNT = 100;
private static final Executor exec = Executors
.newFixedThreadPool(THREAD_COUNT);
public static void main(String[] args) throws IOException
{
ServerSocket server = new ServerSocket(9911);
while (true)
{
final Socket client = server.accept();
Runnable task = new Runnable()
{
@Override
public void run()
{
handleReq(client);
}
};
exec.execute(task);
}
}
protected static void handleReq(Socket client)
{
}
}
创建一个固定长度的线程池,既解决了单线程的阻塞问题,也解决了无限创建线程带来的内存消耗过多等问题。
###### 4、Executors的API介绍
# Spring全套教学资料
**Spring是Java程序员的《葵花宝典》,其中提供的各种大招,能简化我们的开发,大大提升开发效率!目前99%的公司使用了Spring,大家可以去各大招聘网站看一下,Spring算是必备技能,所以一定要掌握。**
**目录:**
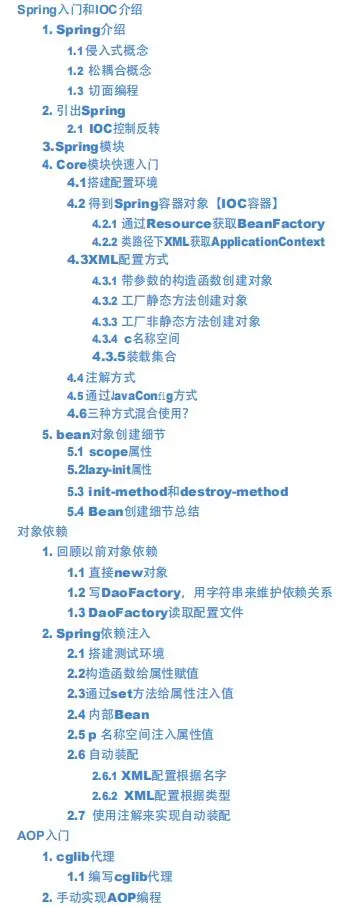
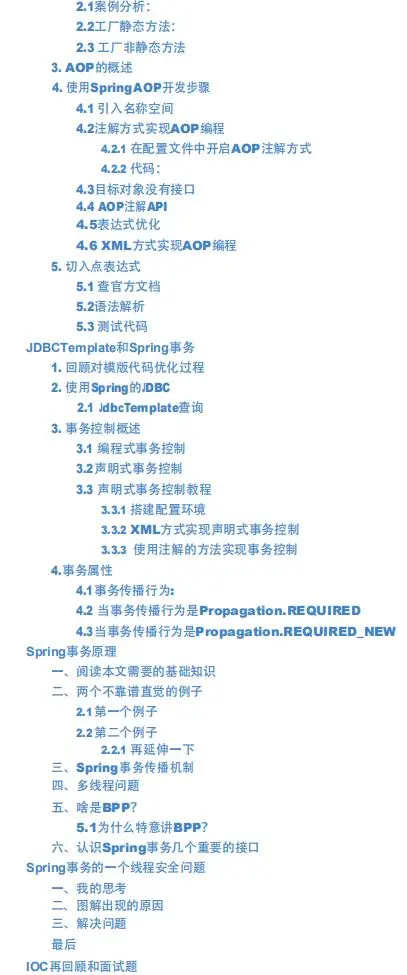
**部分内容:**
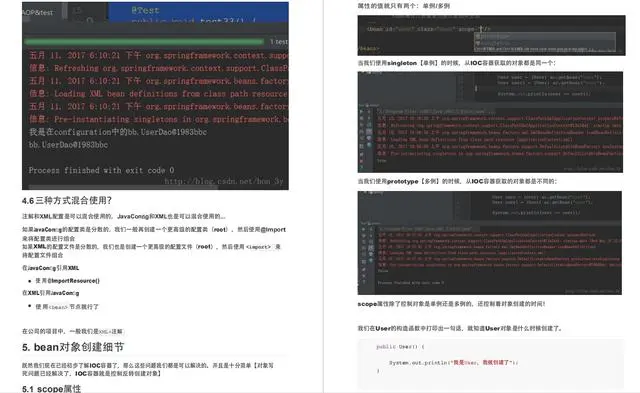
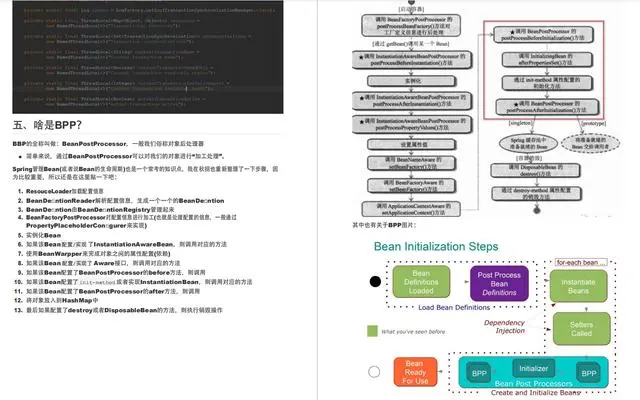
# Spring源码
* 第一部分 Spring 概述
* 第二部分 核心思想
* 第三部分 手写实现 IoC 和 AOP(自定义Spring框架)
* 第四部分 Spring IOC 高级应用
基础特性
高级特性
* 第五部分 Spring IOC源码深度剖析
设计优雅
设计模式
注意:原则、方法和技巧
* 第六部分 Spring AOP 应用
声明事务控制
* 第七部分 Spring AOP源码深度剖析
必要的笔记、必要的图、通俗易懂的语言化解知识难点
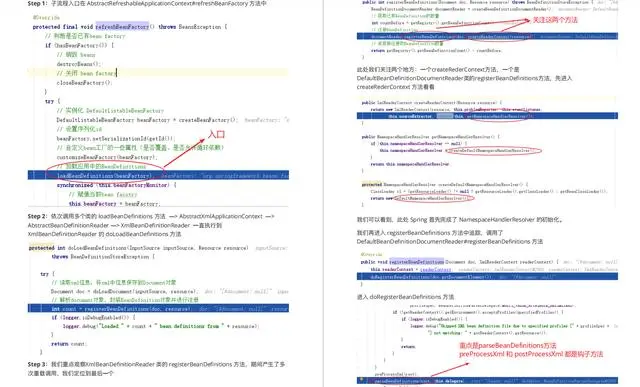
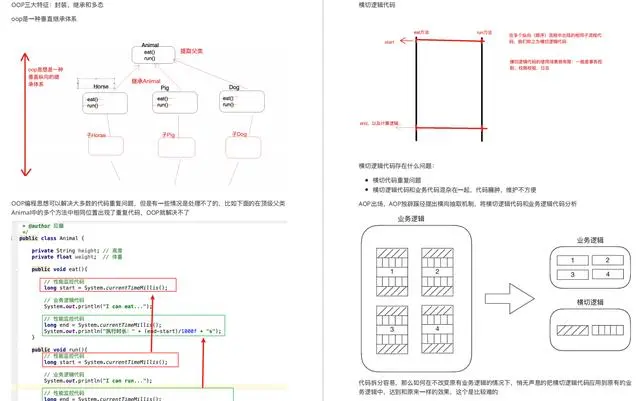
# 脚手框架:SpringBoot技术
> 它的目标是简化Spring应用和服务的创建、开发与部署,简化了配置文件,使用嵌入式web服务器,含有诸多开箱即用的微服务功能,可以和spring cloud联合部署。
>
> Spring Boot的核心思想是约定大于配置,应用只需要很少的配置即可,简化了应用开发模式。
* SpringBoot入门
* 配置文件
* 日志
* Web开发
* Docker
* SpringBoot与数据访问
* 启动配置原理
* 自定义starter
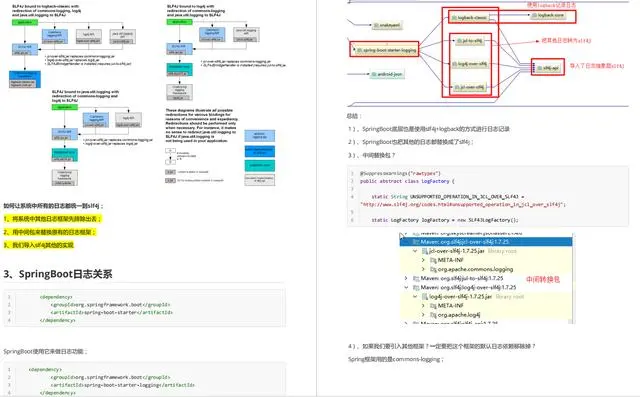
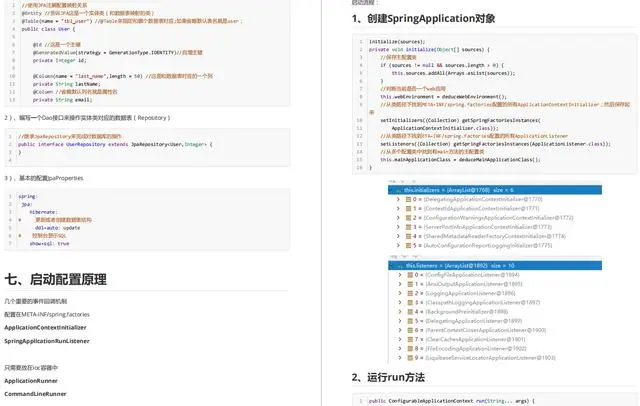
# 微服务架构:Spring Cloud Alibaba
> 同 Spring Cloud 一样,Spring Cloud Alibaba 也是一套微服务解决方案,包含开发分布式应用微服务的必需组件,方便开发者通过 Spring Cloud 编程模型轻松使用这些组件来开发分布式应用服务。
* 微服务架构介绍
* Spring Cloud Alibaba介绍
* 微服务环境搭建
* 服务治理
* 服务容错
* 服务网关
* 链路追踪
* ZipKin集成及数据持久化
* 消息驱动
* 短信服务
* Nacos Confifig—服务配置
* Seata—分布式事务
* Dubbo—rpc通信
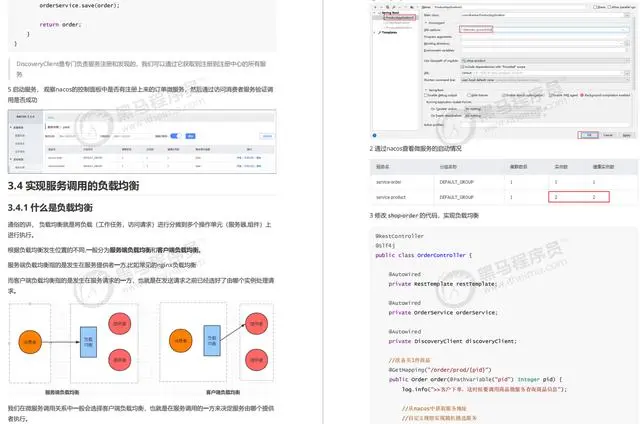
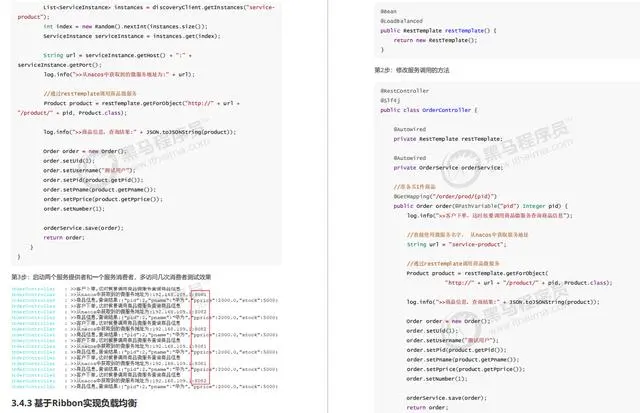
# Spring MVC
**目录:**
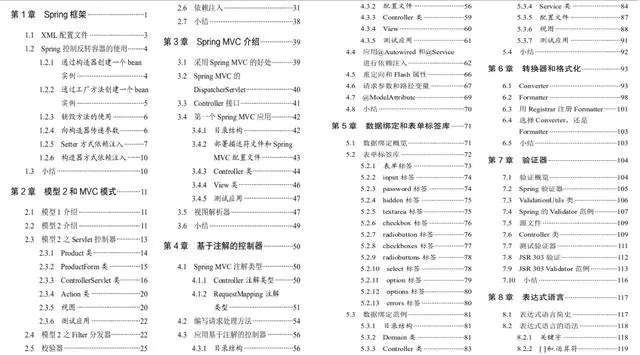
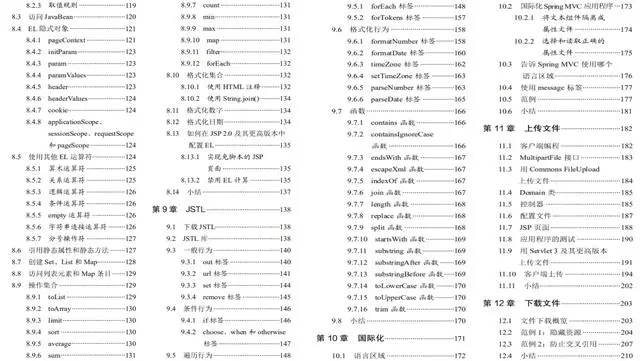
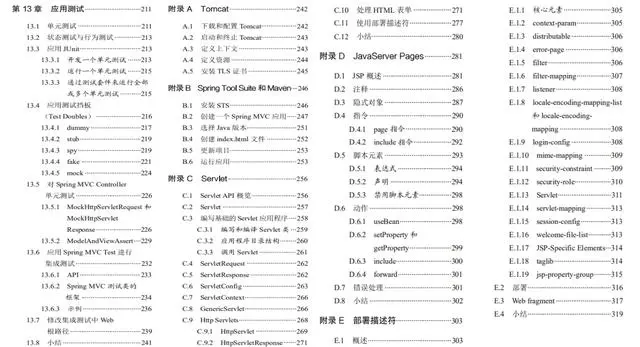
**部分内容:**
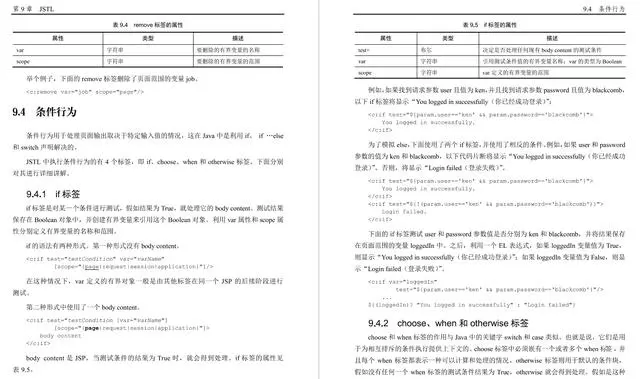
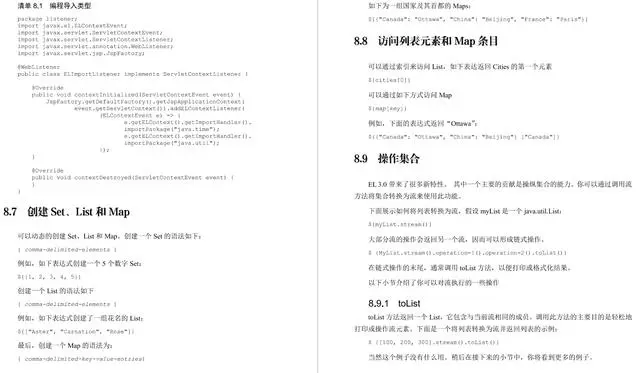
> **找小编(vip1024c)领取**
.(img-JjKVzbnN-1721631981539)]
# Spring MVC
**目录:**
[外链图片转存中...(img-Mfiv5nWs-1721631981540)]
[外链图片转存中...(img-Mb7kKNF2-1721631981540)]
[外链图片转存中...(img-7VF2ipew-1721631981540)]
**部分内容:**
[外链图片转存中...(img-uDvzvwuM-1721631981541)]
[外链图片转存中...(img-h3OTLoY7-1721631981541)]
> **找小编(vip1024c)领取**