项目结构
为什么需要多条件搜索
答:因为在数据量过大时,展示的数据太多,这对用户造成很不好的操作,想要拿到一条数据,却不知如何下手,难道去数据库里找吗?
于是多条件搜索,多个条件并到一块,来显示符合条件的数据记录,这大大的提高的用户的操作体验,所以说,使用多条件搜索对用户友好,提高的程序的可维护性。
为什么需要分页
答:因为数据量过大展示的数据过多,一打开页面,让一次性展示出来这对服务器来说是个灾难,所以以分页展示来减轻服务器的压力,同时方便了操作,多条件搜索与分页结合可以说是完美搭配,大大提示程序的操作感,提高查询数据的速度。
商品的多条件搜索与分页
-
数据表
-
分页
-
多条件查询
======================================================================
/*
SQLyog Ultimate v12.09 (64 bit)
MySQL - 5.7.24
*********************************************************************
*/
/*!40101 SET NAMES utf8 */;
CREATE TABLE `t_goods` (
`order_id` int(11) NOT NULL AUTO_INCREMENT,
`goods_name` varchar(32) COLLATE utf8mb4_unicode_ci NOT NULL,
`goods_price` decimal(10,3) DEFAULT NULL,
`goods_addr` varchar(32) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
PRIMARY KEY (`order_id`)
) ENGINE=InnoDB AUTO_INCREMENT=12 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('1','华为手机','2999.000','中国大陆');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('2','苹果手机','5999.000','美国');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('3','小米手机','1999.000','中国大陆');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('4','三星手机','3999.000','韩国');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('5','苹果','1.000','中国大陆');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('6','香蕉','3.000','菲律宾');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('7','桃','1.000','中国大陆');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('8','DELL电脑','5999.000','美国');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('9','联想电脑','4999.000','中国大陆');
insert into `t_goods` (`order_id`, `goods_name`, `goods_price`, `goods_addr`) values('10','苹果电脑','9999.000','美国');
=====================================================================
分页思路:
1.在展示商品页面写上前一页与后一页,这里我用的是组件,bootstarp
2.在servlet页面调用service层page方法,传入参数为当期页,每页的固定记录数,返回分页对象
3.service实现类调用dao层page方法返回list商品集合,调用getCount方法获取当期商品的总记录数,构造PageInfo对象将截取到的商品集合,总记录数,当前页,每页的固定数传入
4.PageInfo类中初始化参数,计算共多少页,修正当前页,如果小于1,固定为1,如果大于当前页,哪就为当前页,计算前一页与后一页,与修正当前页思路相同。
PageInfo类
package com.wanshi.bean;
import java.util.List;
public class PageInfo {
private List goodsList;
private Integer pageNumb;
private Integer pageSize;
private Integer pageCount;
private Integer rowCount;
private Integer prevPageNumb;
private Integer nextPageNumb;
public PageInfo(List<Goods> goodsList, Integer rowCount, Integer pageNumb, Integer pageSize) {
// TODO Auto-generated constructor stub
//先将商品集合,商品总记录数,每页的记录数赋值
this.goodsList = goodsList;
this.rowCount = rowCount;
this.pageSize = pageSize;
//计算共有几页
this.pageCount = this.rowCount / this.pageSize;
//如果页数不够,那就再补上一页
if (this.rowCount % this.pageSize != 0) {
this.pageCount ++;
}
//修正当前页
this.pageNumb = pageNumb;
if (this.pageNumb < 1) {
this.pageNumb = 1;
}
if (this.pageNumb > this.pageCount) {
this.pageNumb = this.pageCount;
}
//前一页
this.prevPageNumb = this.pageNumb - 1;
if (this.prevPageNumb < 1) {
this.prevPageNumb = 1;
}
//后一页
this.nextPageNumb = this.pageNumb + 1;
if (this.nextPageNumb > this.pageCount) {
this.nextPageNumb = this.pageCount;
}
}
public List getGoodsList() {
return goodsList;
}
public Integer getPageNumb() {
return pageNumb;
}
public Integer getPageSize() {
return pageSize;
}
public Integer getRowCount() {
return rowCount;
}
public Integer getPrevPageNumb() {
return prevPageNumb;
}
public Integer getNextPageNumb() {
return nextPageNumb;
}
public Integer getPageCount() {
return pageCount;
}
}
========================================================================
1.获取要查询的条件,作为参数传入page方法,
2.传入dao层后,dao层实现类判断不为null时,搜索查询该条件的记录
具体代码如下
GoodsDaoImpl实现类
package com.wanshi.dao.impl;
import java.util.List;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.BeanListHandler;
import org.apache.commons.dbutils.handlers.ScalarHandler;
import org.apache.commons.lang.math.NumberUtils;
import com.alibaba.druid.util.StringUtils;
import com.wanshi.bean.Goods;
import com.wanshi.dao.GoodsDao;
public class GoodsDaoImpl extends BaseDao implements GoodsDao{
@Override
public List<Goods> queryAllGoods() throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "select * from t_goods";
List<Goods> goodsList = qur.query(sql, new BeanListHandler<Goods>(Goods.class));
return goodsList;
}
public String strSql(String goodsName, String strMinPrice, String strMaxPrice, String goodsAddr) {
String sql = "";
if (!StringUtils.isEmpty(goodsName)) {
sql += " and goods_name like '%"+goodsName+"%'";
}
if ((!StringUtils.isEmpty(strMinPrice) && NumberUtils.isNumber(strMinPrice))
&& (!StringUtils.isEmpty(strMaxPrice) && NumberUtils.isNumber(strMaxPrice))) {
sql += "and goods_price between "+Double.valueOf(strMinPrice)+" and "+Double.valueOf(strMaxPrice)+" ";
}
if (!StringUtils.isEmpty(goodsAddr)) {
sql += "and goods_addr like '%"+goodsAddr+"%'";
}
return sql;
}
@Override
public List<Goods> page(Integer pageNumb, Integer pageSize, String goodsName, String strMinPrice, String strMaxPrice, String goodsAddr) throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "select * from t_goods where 1 = 1 ";
sql += strSql(goodsName, strMinPrice, strMaxPrice, goodsAddr);
sql += " limit ?, ?";
return qur.query(sql, new BeanListHandler<Goods>(Goods.class), (pageNumb - 1) * pageSize, pageSize);
}
@Override
public Integer getCount(String goodsName, String strMinPrice, String strMaxPrice, String goodsAddr) throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "select count(1) from t_goods where 1 = 1 ";
sql += strSql(goodsName, strMinPrice, strMaxPrice, goodsAddr);
Long count = qur.query(sql, new ScalarHandler<Long>());
System.out.println(sql);
System.out.println(count);
return count.intValue();
}
### 最后
**这份清华大牛整理的进大厂必备的redis视频、面试题和技术文档**
祝大家早日进入大厂,拿到满意的薪资和职级~~~加油!!
感谢大家的支持!!
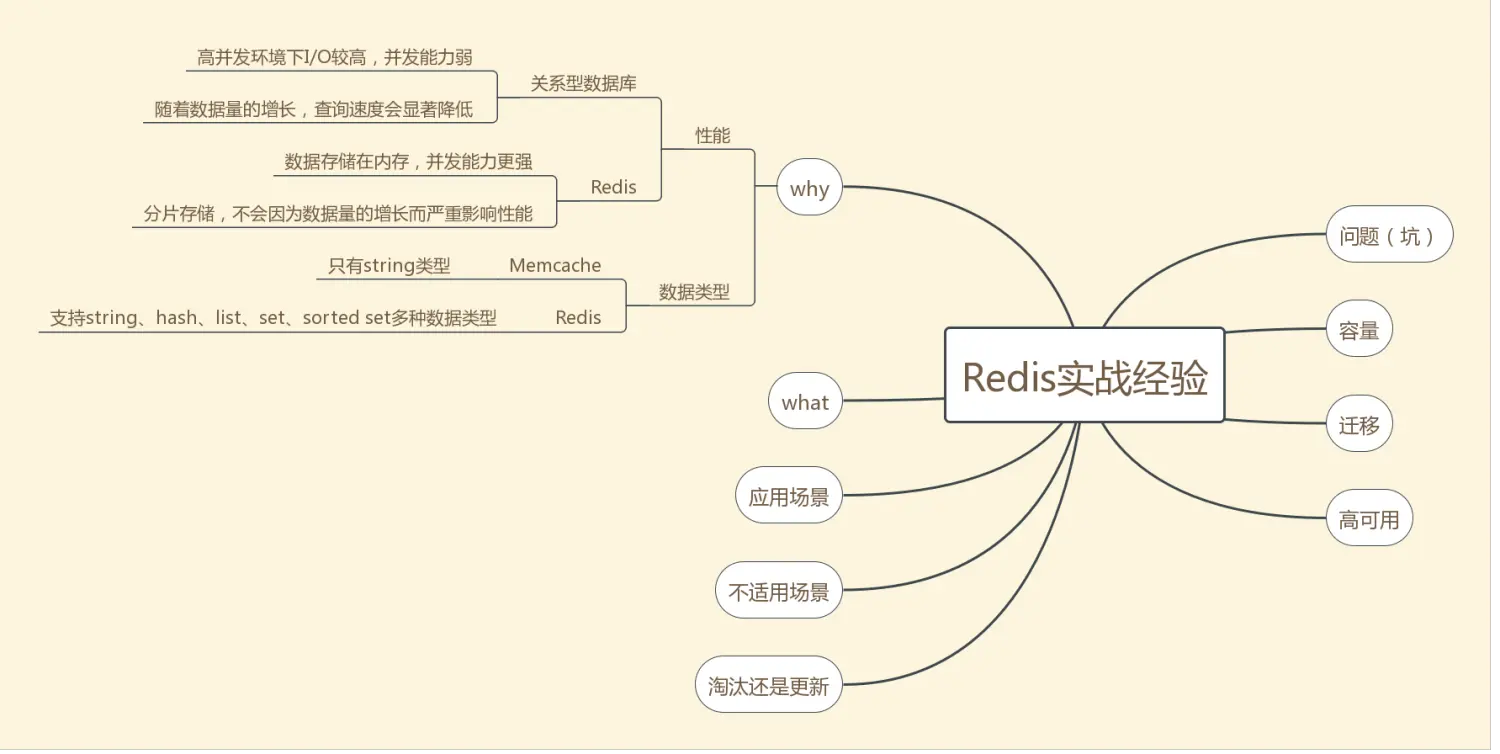
了解详情https://docs.qq.com/doc/DSmxTbFJ1cmN1R2dB
t_goods where 1 = 1 ";
sql += strSql(goodsName, strMinPrice, strMaxPrice, goodsAddr);
Long count = qur.query(sql, new ScalarHandler<Long>());
System.out.println(sql);
System.out.println(count);
return count.intValue();
}
### 最后
**这份清华大牛整理的进大厂必备的redis视频、面试题和技术文档**
祝大家早日进入大厂,拿到满意的薪资和职级~~~加油!!
感谢大家的支持!!
[外链图片转存中...(img-K8dnyat4-1724422923744)]
了解详情https://docs.qq.com/doc/DSmxTbFJ1cmN1R2dB