React Hooks是React 16.8版本中引⼊的新特性,它允许你在不编写class的情况下使⽤state以及其他的React特性。Hooks提供了⼀种新的函数式编程的⽅式来使⽤React组件的状态和⽣命周期性。
以下是⼀些常⽤的React Hooks以及它们的作⽤:
1.
useState
◦
作⽤:⽤于在函数组件中添加和管理状态。它返回⼀个状态变量和⼀个更新该状态的函数。
◦
示例:
import React, { useState } from 'react';
function Example() {
// 声明⼀个新的状态变量,我们称之为 "count"
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
2.
useEffect
◦
作⽤:在函数组件中执⾏副作⽤操作(如数据获取、订阅或⼿动更改DOM)。与
componentDidMount
、
componentDidUpdate
和
componentWillUnmount
具有相
似的⽤途,但将它们合并为了⼀个统⼀的API。
◦
示例:
import React, { useState, useEffect } from 'react';
function Example() {
const [count, setCount] = useState(0);
// 类似于 componentDidMount 和 componentDidUpdate:
useEffect(() => {
// 使⽤浏览器的 API 更新⻚⾯标题
document.title = `You clicked ${count} times`;
});
// ...
}
3.
useContext
◦
作⽤:在函数组件中访问和使⽤React的Context。它接收⼀个Context对象,并返回该Context
的当前值。
◦
⽰例:
import React, { useContext } from 'react';
import MyContext from './MyContext';
function MyComponent() {
const value = useContext(MyContext);
// ...
}
4.
useReducer
◦
作⽤:⼀个替代
useState
的Hook,它接收⼀个reducer函数来更新状态,并返回当前的
state以及⼀个dispatch⽅法来触发状态的更新。这在处理更复杂的状态逻辑时特别有⽤。
◦
⽰例:
import React, { useReducer } from 'react';
function initialCount(initialCount) {
return { count: initialCount };
}
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialCount(0));
return (
<>
Count: {state.count}
<button onClick={() => dispatch({ type: 'increment' })}>
+
</button>
<button onClick={() => dispatch({ type: 'decrement' })}>
-
</button>
</>
);
}
以上都是React Hooks中常⽤的⼀些,它们使得函数组件具备了与类组件相同甚⾄更强⼤的功能,同时保持了函数组件的简洁和可读性。通过使⽤Hooks,你可以在函数组件中管理状态、执⾏副作⽤操
作、访问Context等,从⽽提⾼了组件的复⽤性和灵活性。
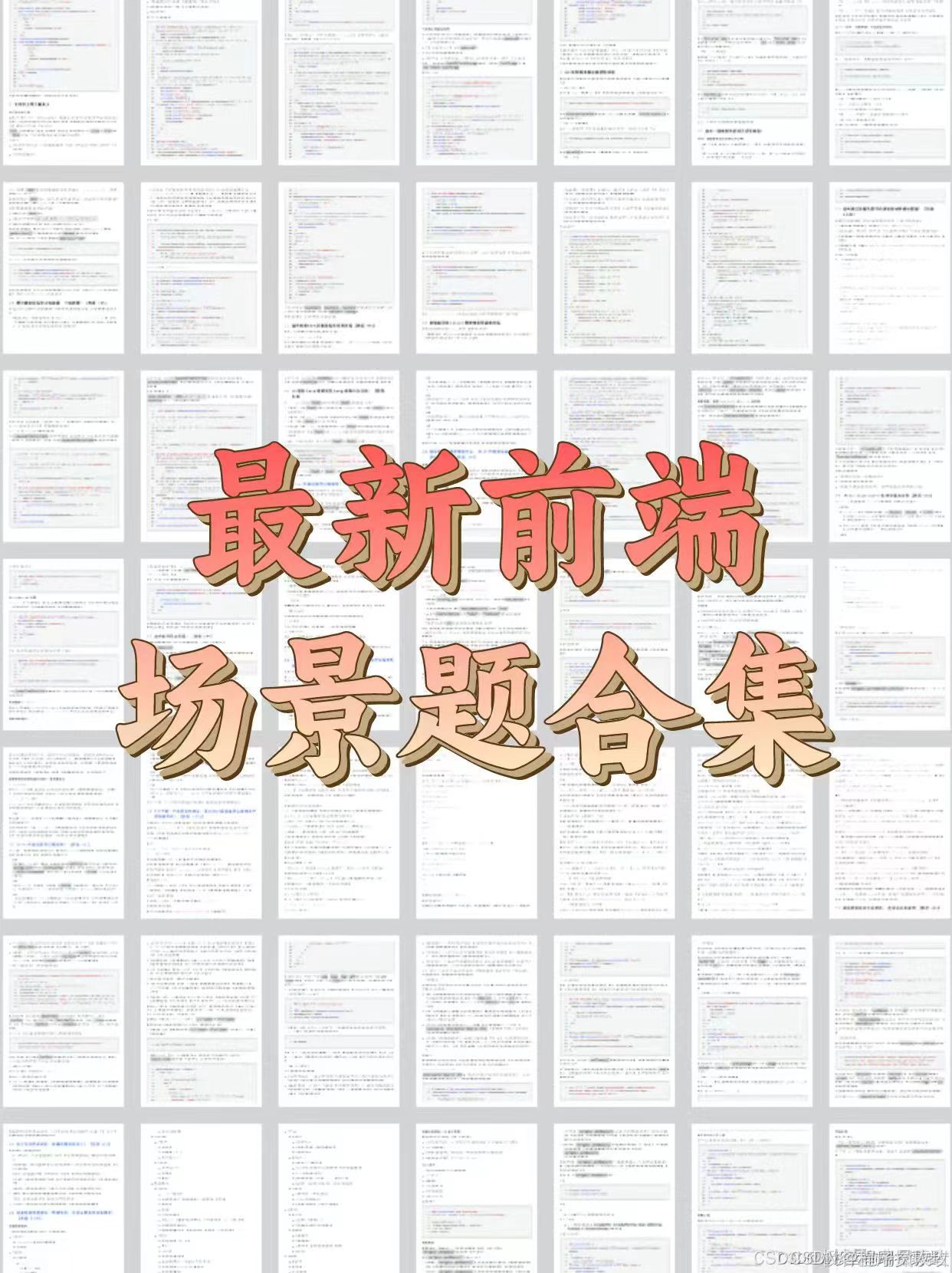
更多前端面试题 需要的同学转发本文+关注+【点击此处】即可获取!加油复习