相信有很多这样的兄弟,学了 Vue3 的各种 API 和新特性,但公司项目依然使用的是 Vue2 ,也不知道自己的水平能否上手 Vue3 项目。其实你学的是零碎的知识点,缺少真实的使用场景。
今天就把实战过程中遇到的十个场景分享给大家,结合尤大大推荐的 <script setup>
,希望你能从 Vue2 丝滑过渡到 Vue3!
场景一:父子组件数据传递
父组件数据传递到子组件
Vue3 中父组件同样是通过属性传递数据,但子组件接受数据的方式和 Vue2 不同。在 <script setup>
中,props 需要使用 defineProps()
这个宏函数来进行声明,它的参数和 Vue2 props 选项的值是一样的。
<!-- 父组件 -->
<script setup>
import ChildView from './ChildView.vue'
</script>
<template>
<ChildView some-prop="parent message" />
</template>
复制代码
<!-- 子组件 -->
<script setup>
const props = defineProps({
someProp: {
type: String,
required: true
}
})
console.log(props.someProp) // parent message
</script>
<template>
<!-- 使用 someProp 或 props.someProp -->
<div>{{ someProp }}</div>
<div>{{ props.someProp }}</div>
</template>
复制代码
注意:defineProps
、defineEmits
、 defineExpose
和 withDefaults
这四个宏函数只能在 <script setup>
中使用。他们不需要导入,会随着 <script setup>
的处理过程中一起被编译。
子组件数据传递到父组件
Vue2 中子组件数据传递到父组件,通常是使用 $emit
触发一个自定义事件来进行传递。但 $emit
无法在 <script setup>
中使用,这时候我们需要使用 defineEmits()
:
<!-- 子组件 -->
<script setup>
const emit = defineEmits(['someEvent'])
function onClick() {
emit('someEvent', 'child message')
}
</script>
<template>
<button @click="onClick">点击</button>
</template>
复制代码
<!-- 父组件 -->
<script setup>
import ChildView from './ChildView.vue'
function someEvent(value) {
console.log(value) // child message
}
</script>
<template>
<ChildView @some-event="someEvent" />
</template>
复制代码
父组件使用子组件数据
在 <script setup>
中,组件的属性和方法默认都是私有的。父组件无法访问到子组件中的任何东西,除非子组件通过 defineExpose
显式的暴露出去:
<!-- 子组件 -->
<script setup>
import { ref } from 'vue'
const msg = ref('hello vue3!')
function change() {
msg.value = 'hi vue3!'
console.log(msg.value)
}
// 属性或方法必须暴露出去,父组件才能使用
defineExpose({ msg, change })
</script>
复制代码
<!-- 父组件 -->
<script setup>
import ChildView from './ChildView.vue'
import { ref, onMounted } from 'vue'
const child = ref(null)
onMounted(() => {
console.log(child.value.msg) // hello vue3!
child.value.change() // hi vue3!
})
</script>
<template>
<ChildView ref="child"></ChildView>
</template>
复制代码
场景二:组件之间双向绑定
大家都知道 Vue2 中组件的双向绑定采用的是 v-model
或 .snyc
修饰符,两种写法多少显得有点重复,于是在 Vue3 中合成了一种。Vue3 统一使用 v-model
进行处理,并且可以和多个数据进行绑定,如 v-model:foo
、v-model:bar
。
v-model
等价于 :model-value="someValue"
和 @update:model-value="someValue = $event"
v-model:foo
等价于 :foo="someValue"
和 @update:foo="someValue = $event"
下面就是一个父子组件之间双向绑定的例子:
<!-- 父组件 -->
<script setup>
import ChildView from './ChildView.vue'
import { ref } from 'vue'
const msg = ref('hello vue3!')
</script>
<template>
<ChildView v-model="msg" />
</template>
复制代码
<!-- 子组件 -->
<script setup>
defineProps(['modelValue'])
const emit = defineEmits(['update:modelValue'])
</script>
<template>
<div @click="emit('update:modelValue', 'hi vue3!')">{{ modelValue }}</div>
</template>
复制代码
子组件可以结合 input
使用:
<!-- 子组件 -->
<script setup>
defineProps(['modelValue'])
const emit = defineEmits(['update:modelValue'])
</script>
<template>
<input :value="modelValue" @input="emit('update:modelValue', $event.target.value)" />
</template>
复制代码
如果你觉得上面的模板比较繁琐,也可以结合 computed
一起使用:
<!-- 子组件 -->
<script setup>
import { computed } from 'vue'
const props = defineProps(['modelValue'])
const emit = defineEmits(['update:modelValue'])
const newValue = computed({
get() {
return props.modelValue
},
set(value) {
emit('update:modelValue', value)
}
})
</script>
<template>
<input v-model="newValue" />
</template>
复制代码
场景三:路由跳转,获取路由参数
在 Vue2 中我们通常是使用 this.$router
或 this.$route
来进行路由的跳转和参数获取,但在 <script-setup>
中,是这些方法无法使用的。我们可以使用 vue-router
提供的 useRouter
方法,来进行路由跳转:
<script setup>
import { useRouter } from 'vue-router'
const router = useRouter()
function onClick() {
router.push({
path: '/about',
query: {
msg: 'hello vue3!'
}
})
}
</script>
复制代码
当我们要获取路由参数时,可以使用 vue-router
提供的 useRoute
方法:
<script setup>
import { useRoute } from 'vue-router'
const route = useRoute()
console.log(route.query.msg) // hello vue3!
</script>
复制代码
场景四:获取上下文对象
Vue3 的 setup
中无法使用 this
这个上下文对象。可能刚接触 Vue3 的兄弟会有点懵,我想使用 this
上的属性和方法应该怎么办呢。虽然不推荐这样使用,但依然可以通过 getCurrentInstance
方法获取上下文对象:
<script setup>
import { getCurrentInstance } from 'vue'
// 以下两种方法都可以获取到上下文对象
const { ctx } = getCurrentInstance()
const { proxy } = getCurrentInstance()
</script>
复制代码
这样我们就可以使用 $parent
、$refs
等,干自己想干的事情了,下面是我打印出来的 ctx
的完整属性。
注意:ctx
只能在开发环境使用,生成环境为 undefined 。 推荐使用 proxy
,在开发环境和生产环境都可以使用。
场景五:插槽的使用
在 Vue2 的中一般是通过 slot
属性指定模板的位置,通过 slot-scope
获取作用域插槽的数据,如:
<!-- 父组件 -->
<script setup>
import ChildView from './ChildView.vue'
</script>
<template>
<div>parent<div>
<ChildView>
<template slot="content" slot-scope="{ msg }">
<div>{{ msg }}</div>
</template>
</ChildView>
</template>
复制代码
<!-- 子组件 -->
<template>
<div>child</div>
<slot name="content" msg="hello vue3!"></slot>
</template>
复制代码
在 Vue3 中则是通过 v-slot
这个指令来指定模板的位置,同时获取作用域插槽的数据,如:
<!-- 父组件 -->
<script setup>
import ChildView from './ChildView.vue'
</script>
<template>
<div>parent</div>
<ChildView>
<template v-slot:content="{ msg }">
<div>{{ msg }}</div>
</template>
</ChildView>
</template>
<!-- ChildView 也可以简写为: -->
<ChildView>
<template #content="{ msg }">
<div>{{ msg }}</div>
</template>
</ChildView>
复制代码
<!-- 子组件 -->
<template>
<div>child</div>
<slot name="content" msg="hello vue3!"></slot>
</template>
复制代码
注意:v-slot
在 Vue2 中也可以使用,但必须是 Vue2.6+ 的版本。
场景六:缓存路由组件
缓存一般的动态组件,Vue3 和 Vue2 的用法是一样的,都是使用 KeepAlive
包裹 Component
。但缓存路由组件,Vue3 需要结合插槽一起使用:
// Vue2 中缓存路由组件
<KeepAlive>
<RouterView />
</KeepAlive>
复制代码
// Vue3 中缓存路由组件
<RouterView v-slot="{ Component }">
<KeepAlive>
<Component :is="Component"></Component>
</KeepAlive>
</RouterView>
复制代码
一个持续存在的组件可以通过 onActivated()
和 onDeactivated()
两个生命周期钩子注入相应的逻辑:
<script setup>
import { onActivated, onDeactivated } from 'vue'
onActivated(() => {
// 调用时机为首次挂载
// 以及每次从缓存中被重新插入时
})
onDeactivated(() => {
// 调用时机为从 DOM 上移除、进入缓存
// 以及组件卸载时
})
</script>
复制代码
场景七:逻辑复用
Vue2 中逻辑复用主要是采用 mixin
,但 mixin
会使数据来源不明,同时会引起命名冲突。所以 Vue3 更推荐的是全新的 Composition Api
。
下面是鼠标跟踪的例子,我们可以把逻辑提取出来:
// mouse.js
import { ref, onMounted, onUnmounted } from 'vue'
// 按照惯例,组合式函数名以 use 开头
export function useMouse() {
// 组合式函数管理的数据
const x = ref(0)
const y = ref(0)
function update(event) {
x.value = event.pageX
y.value = event.pageY
}
### 最后前端到底应该怎么学才好?
如果你打算靠自己摸索自学,那么你首先要了解学习前端的基本大纲,这是你将要学习的主要内容,理解以及掌握好这些内容,便可以找到一份初级的前端开发工作。你还需要有一套完整的前端学习教程,作为初学者最好的方式就是看视频教程学习,初学者容易理解接受。
不要选择买书学习,这样的方式没有几个人能学会,基本都是看不下去书,也看不懂书。如果喜欢看书的学弟,可以买一些经典的书籍作为辅助即可,主要还是以看教程为主。每天抽出固定几个小时学习,做好长期学习的准备。学习编程并不是每天光看视频,你学习编程最重要的目的是为了编写软件产品,提供给大众使用,所以用手写出代码实现功能才是我们要做的事情。
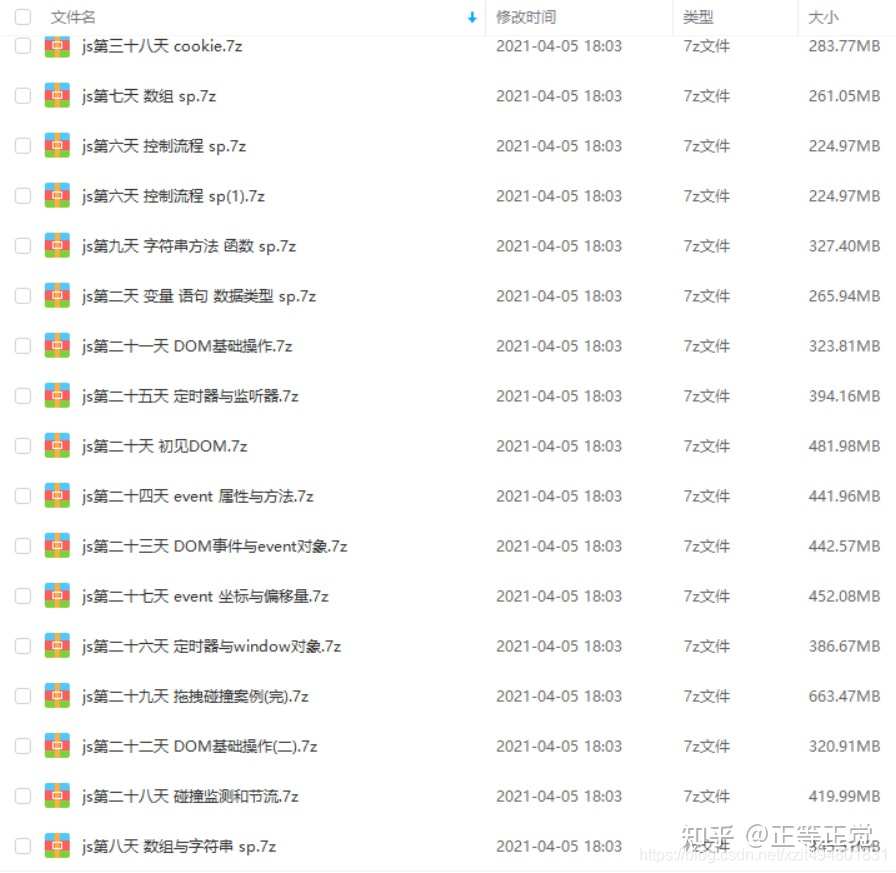
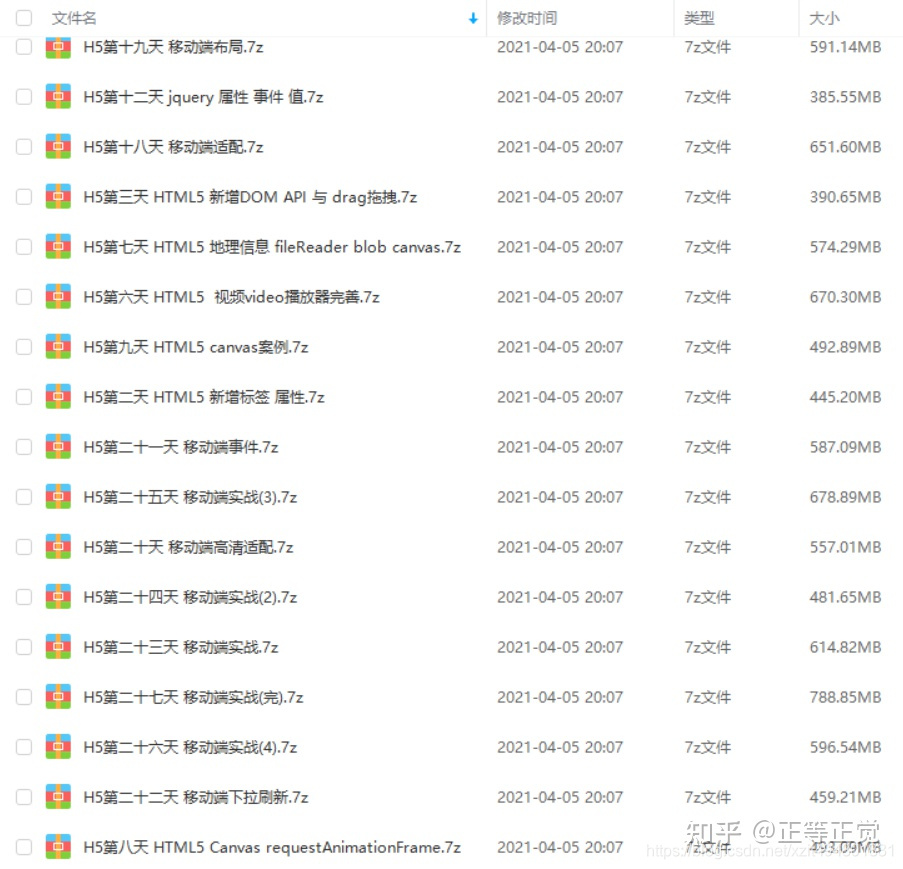
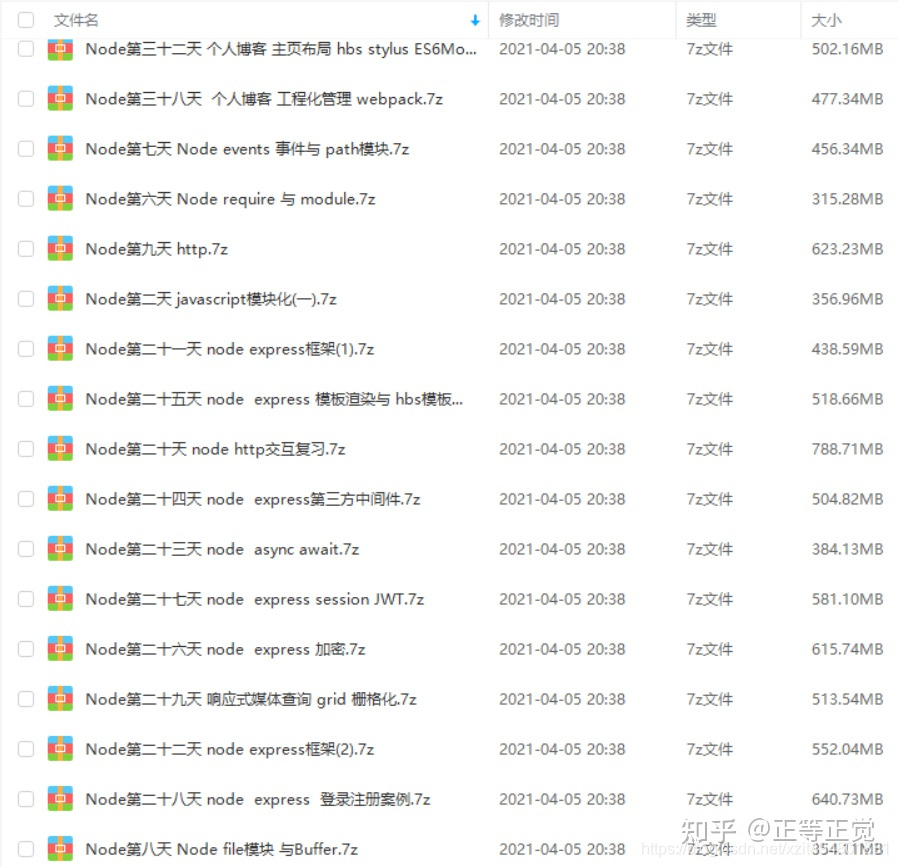
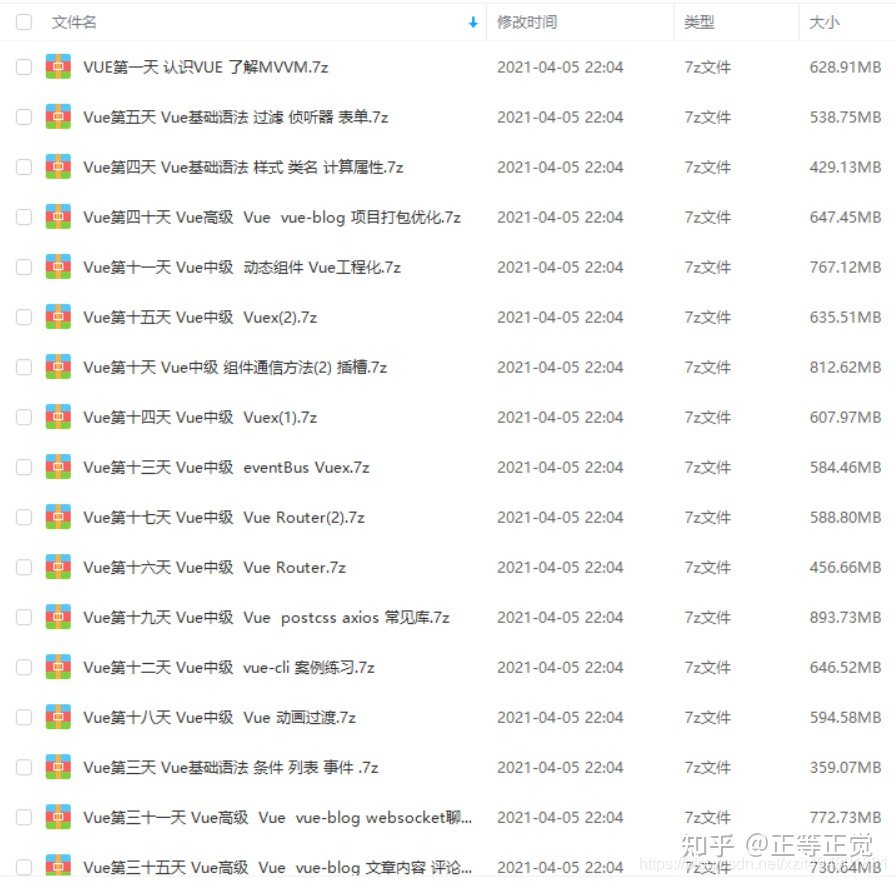
参考docs.qq.com/doc/DSmRnRGxvUkxTREhO