print(s[3]) #‘l’
print(s[-3]) #‘l’
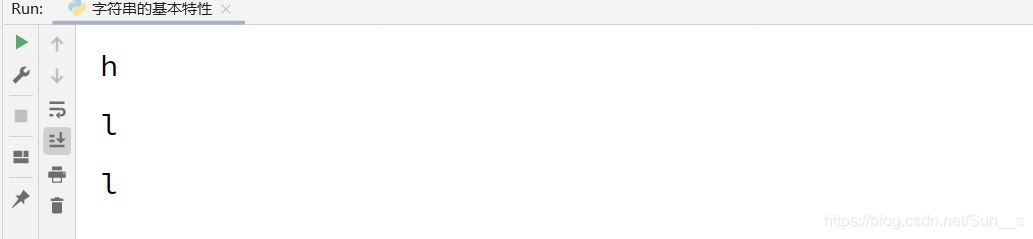
### 4.切片
4.切片
“”"
切片:切出一部分的内容
s[start🔚step]
s[:end]:
s[start:]:
“”"
s = ‘hello world’
print(s[1:3]) # 从1开始到3-1结束
print(s[:3]) # 从0开始到3-1 结束
print(s[:5]) # 前五个字符
print(s[3:]) # 从第3个开始,到结束所有字符
print(s[::]) # 拷贝字符串 拿出所有字符串
print(s[::-1]) #倒序
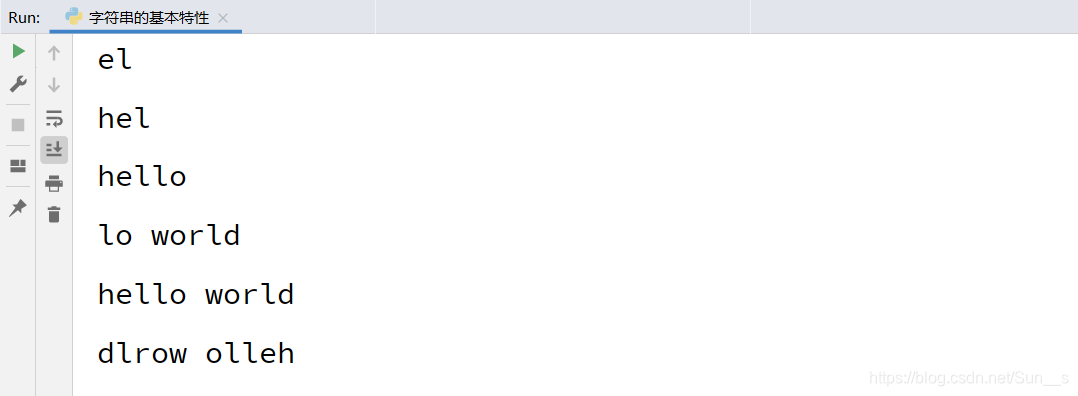
### 5. 可迭代对象for循环访问
5. 可迭代对象for循环访问
s = ‘hello’
count = 0
for item in s:
count +=1
print(f’第{count}字符是{item}')
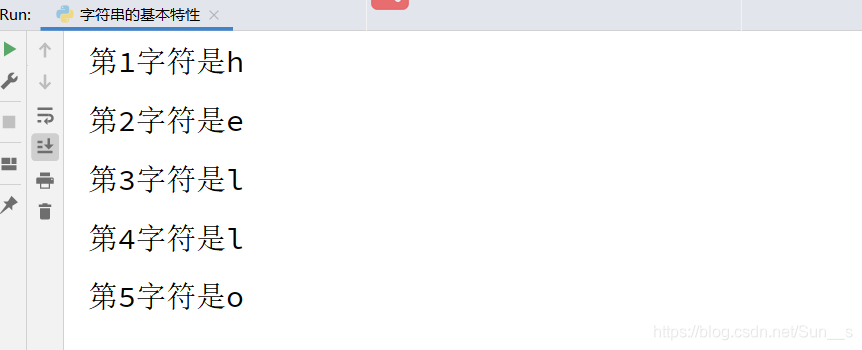
练习:
用户输入一个字符串,判断这个字符串是否为回文字符串。
“”"
代码需求:
用户输入一个字符串,判断这个字符串是否为回文字符串。
“”"
s = input(“输入字符串:”)
result = “回文字符串” if s == s[::-1] else “不是回文字符串”
print(s + result)
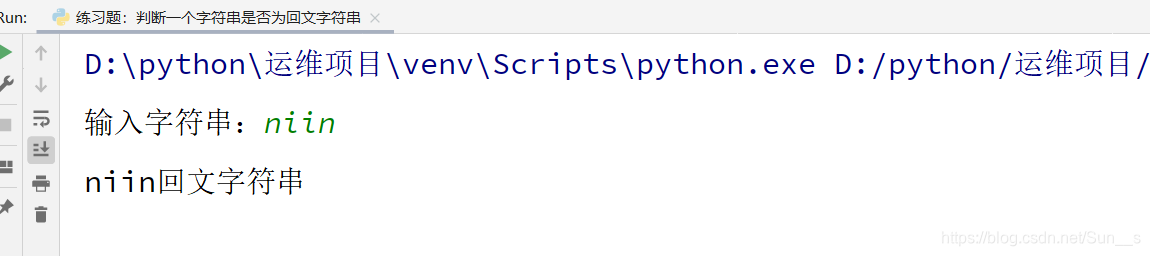
## 三、字符串的内建方法
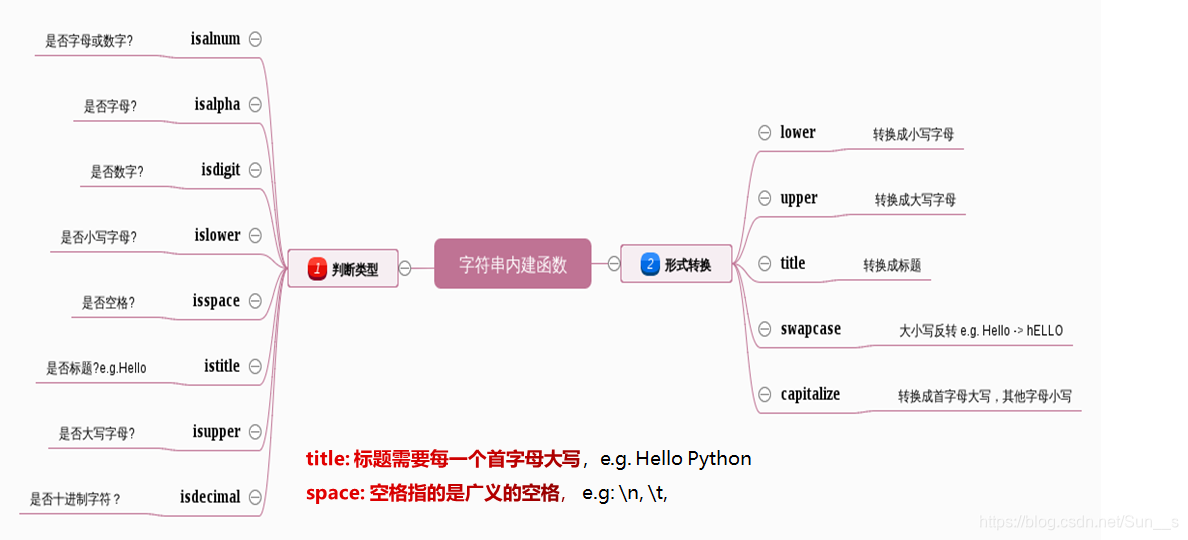
### 1.字符串的判断和转换
下面我们演示三种 是否字母或数字? 是否数字? 是否大写字母?
s = “helloworld”
print(s.isalnum()) ##True
print(s.isdigit()) ##False
print(s.isupper()) ##False
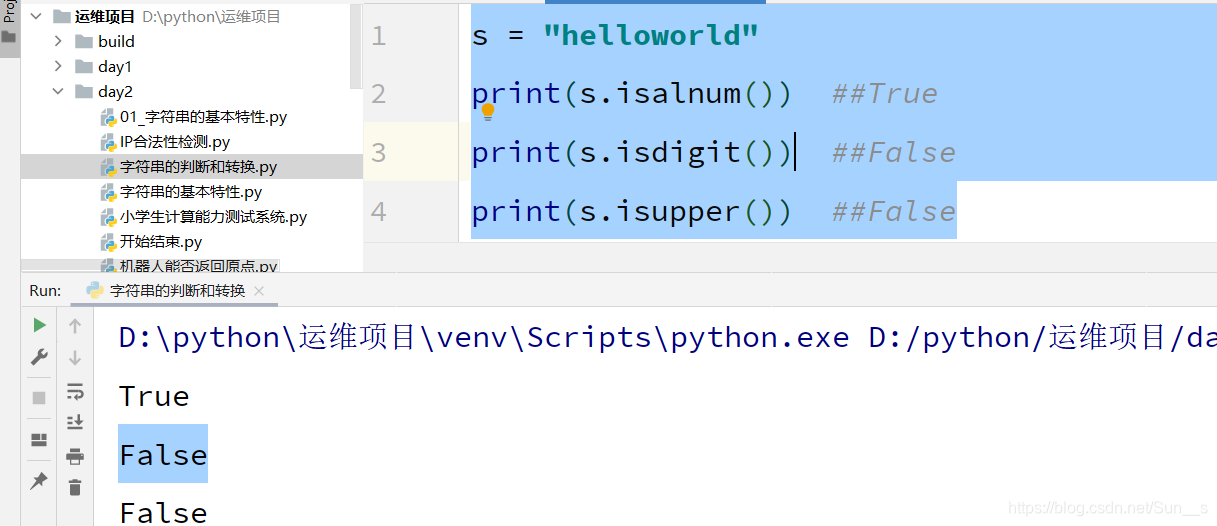
### 2.类型转换
2.类型转换
print(‘hello’.upper()) ##HELLO
print(‘HELLO’.lower()) ## hello
print(‘HELLO world’.title()) ## Hello World
print(‘HELLO world’.capitalize()) ## Hello world
print(‘HELLO world’.swapcase()) ##hello WORLD
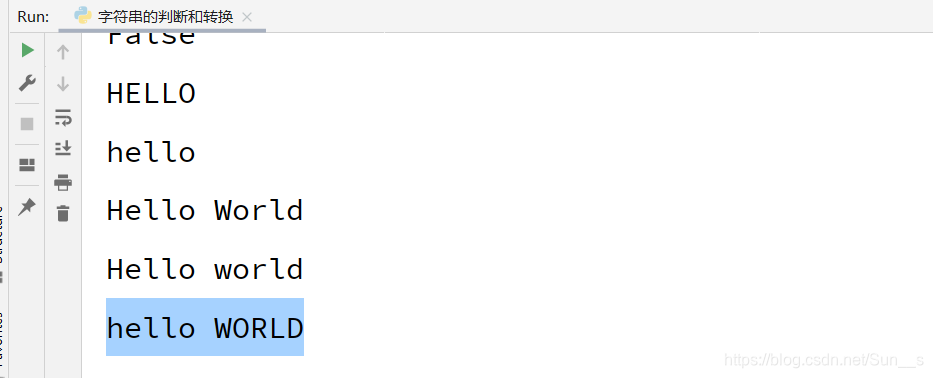
实例:
需求:用户输入Y或者y都继续执行代码
yum install httpd
choice = input (‘是否继续安装程序(y|Y):’)
if choice.lower() == ‘y’:
print(“正在安装程序…”)正在安装程序

### 3.字符串开头和结尾的判断

startswith
url = ‘http://www.baidu.com’
if url.startswith(‘http’):
print(f’{url}是一个正确的网址,可以爬取网站’)
endswith:
常用场景:判断文件类型
filename = ‘sun.png’
if filename.endswith(‘.png’):
print(f’{filename} 是图片文件’)
elif filename.endswith(‘.mp3’):
print(f’{filename}是音乐文件’)
else:
print(f’{filename}是未知文件’)
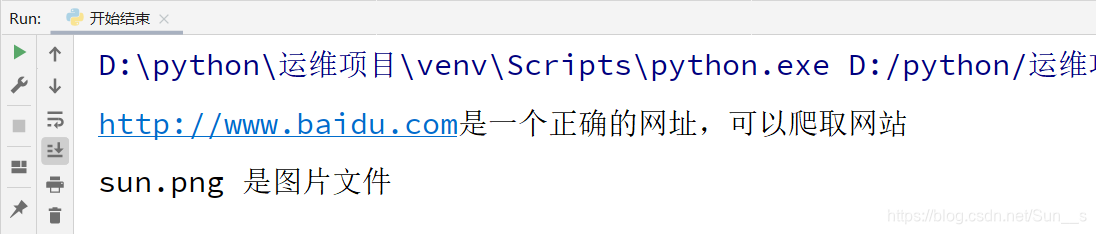
#pycharm常用的快捷键:
#如何查看方法的源代码和解释说明: ctrl键按住,
#鼠标移动到你想要查看方法的位置,点击即可进入源码及方法说明
### 4.字符串的数据清洗
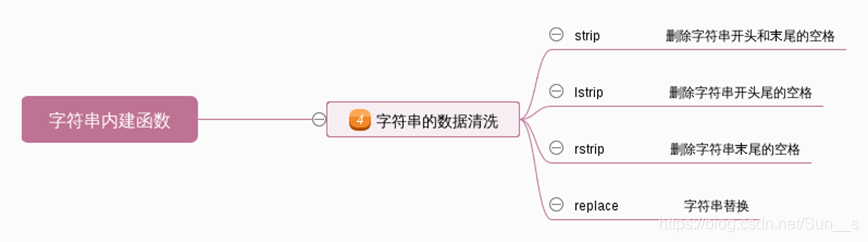
数据清洗的思路:
* lstrip: 删除字符串左边的空格(指广义的空格: \n, \t, ’ ')
* rstrip: 删除字符串右边的空格(指广义的空格: \n, \t, ’ ')
* strip: 删除字符串左边和右边的空格(指广义的空格: \n, \t, ’ ')
* replace: 替换函数, 删除中间的空格, 将空格替换为空。replace(" ", )
s = ’ hello’
print(s)
print(s.lstrip())
s = 'hello ’
print(s.rstrip())
s = ’ hello ’
print(s.strip())
s = ‘hel lo’
print(s.replace(" “,”"))
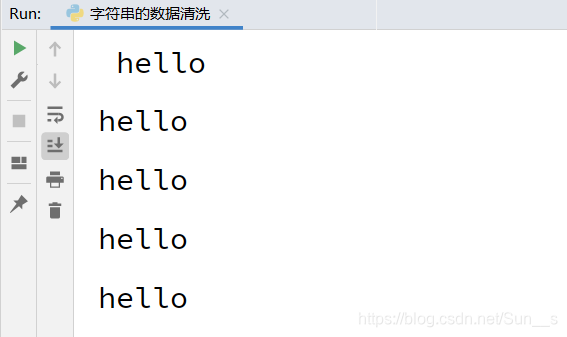
### 5.字符串的位置调整
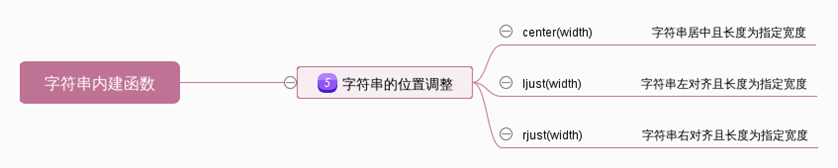
print(“学生管理系统”.center(50))
print(“学生管理系统”.center(50,“*”))
print(“学生管理系统”.center(50,“-”))
print(“学生管理系统”.ljust(50,“*”))
print(“学生管理系统”.rjust(50,“*”))
效果查看:
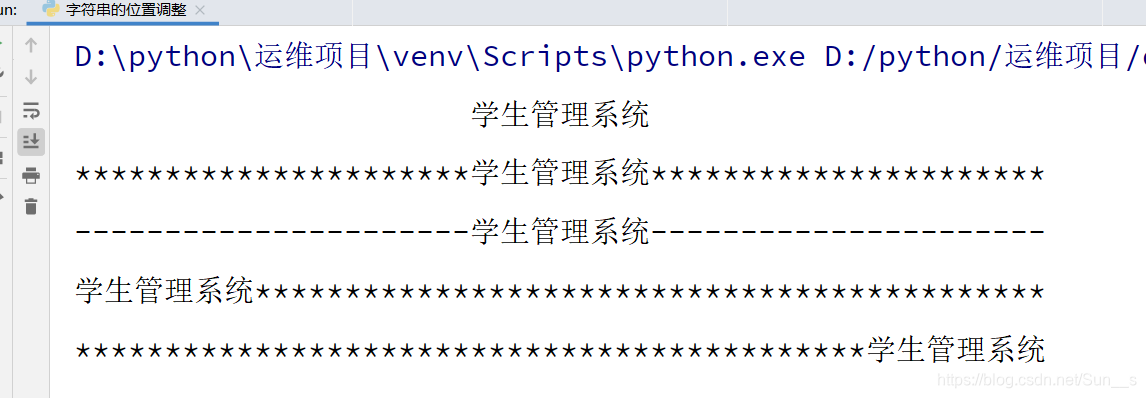
### 6.字符串的搜索和统计

s = “hello world”
print(s.find(“llo”))
print(s.index(“llo”))
print(s.find(“xxx”))
print(s.index(“xxx”))
find如果找到子串, 则返回子串开始的索引位置。 否则返回-1
index如果找到子串,则返回子串开始的索引位置。否则报错(抛出异常).
print(s.count(“l”)) ## 判断字符出现次数
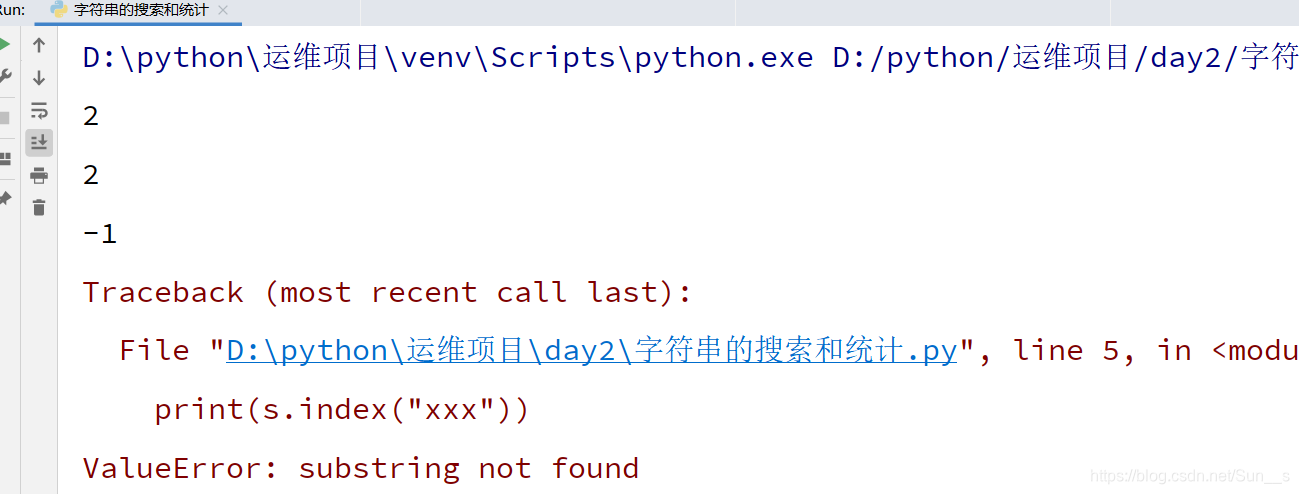
### 6.字符串的分离和拼接
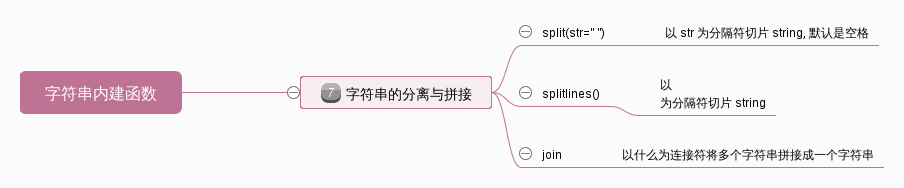
ip = ‘172.25.254.100’
print(ip.split(‘.’))
items = ip.split(‘.’)
print(‘-’.join(items))


**感谢每一个认真阅读我文章的人,看着粉丝一路的上涨和关注,礼尚往来总是要有的:**
① 2000多本Python电子书(主流和经典的书籍应该都有了)
② Python标准库资料(最全中文版)
③ 项目源码(四五十个有趣且经典的练手项目及源码)
④ Python基础入门、爬虫、web开发、大数据分析方面的视频(适合小白学习)
⑤ Python学习路线图(告别不入流的学习)