ThreadTask()
{
}
//将数据与处理方式打包在一起
void setTask(int data, handler_t handler)
{
_data = data;
_handler = handler;
}
//执行任务函数
void run()
{
return \_handler(_data);
}
private:
int _data;//任务中处理的数据
handler_t _handler;//处理任务方式
};
//线程池类
class ThreadPool
{
public:
ThreadPool(int thr_max = MAX_THREAD)
:_thr_max(thr_max)
{
pthread_mutex_init(&_mutex, NULL);
pthread_cond_init(&_cond, NULL);
for (int i = 0; i < _thr_max; i++)
{
pthread_t tid;
int ret = pthread_create(&tid, NULL, thr_start, this);
if (ret != 0)
{
printf(“thread create error\n”);
exit(-1);
}
}
}
~ThreadPool()
{
pthread_mutex_destroy(&_mutex);
pthread_cond_destroy(&_cond);
}
bool taskPush(ThreadTask &task)
{
pthread_mutex_lock(&_mutex);
_queue.push(task);
pthread_mutex_unlock(&_mutex);
pthread_cond_signal(&_cond);
return true;
}
//类的成员函数,有默认的隐藏参数this指针
//置为static,没有this指针,
static void *thr_start(void *arg)
{
ThreadPool *p = (ThreadPool*)arg;
while (1)
{
pthread_mutex_lock(&p->_mutex);
while (p->_queue.empty())
{
pthread_cond_wait(&p->_cond, &p->_mutex);
}
ThreadTask task;
task =p-> _queue.front();
p->_queue.pop();
pthread_mutex_unlock(&p->_mutex);
task.run();//任务的处理要放在解锁之外
}
return NULL;
}
private:
int _thr_max;//线程池中线程的最大数量
queue _queue;//任务缓冲队列
pthread_mutex_t _mutex; //保护队列操作的互斥量
pthread_cond_t _cond; //实现从队列中获取结点的同步条件变量
};
main.cpp
//main.cpp
#include <unistd.h>
#include “threadpool.hpp”
//处理方法1
void test_func(int data)
{
int sec = (data % 3) +1;
printf(“tid:%p – get data:%d, sleep:%d\n”, pthread_self(), data, sec);
sleep(sec);
}
//处理方法2
void tmp_func(int data)
{
printf(“tid:%p – tmp_func\n”, pthread_self());
sleep(1);
}
int main()
{
ThreadPool pool;
for (int i = 0; i < 10; i++)
{
ThreadTask task;
if (i % 2 == 0)
{
task.setTask(i, test_func);
}
else
{
task.setTask(i, tmp_func);
}
pool.taskPush(task);
}
sleep(1000);
return 0;
}
运行结果:线程池最多有5个线程,标注的每种颜色对应的是同一个线程,这样子就能完成通过几个线程,完成多个任务,而不是多个线程完成多个任务。创建和销毁的时间开销也节省了不少
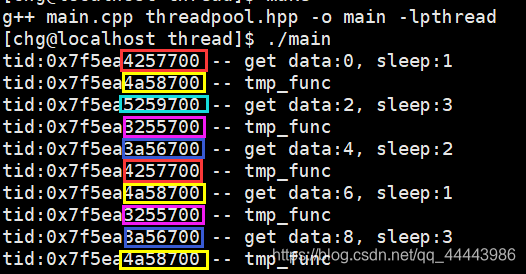