自定义 Tree 树形控件背景
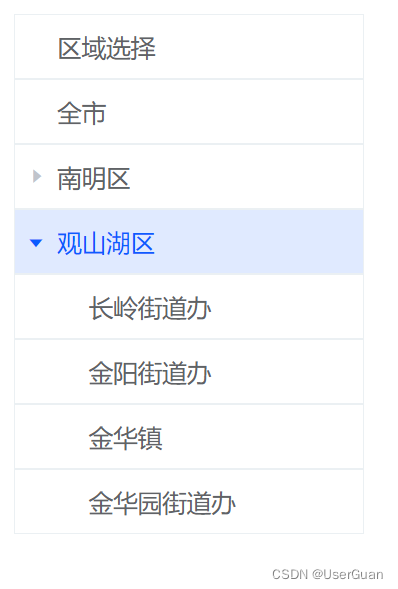
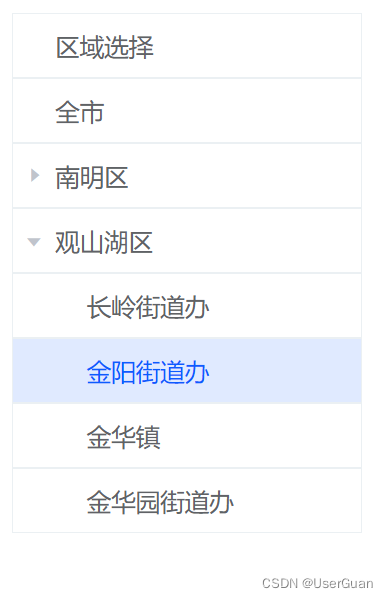
在 template 中
<div class="tree-box">
<el-tree :data="treeList" :props="defaultProps" accordion @node-click="handleNodeClick" />
</div>
在 script 中
treeList: [
{ id: "-1", label: "区域选择" },
{ id: "0", label: "全市" },
{
id: "1",
label: "南明区",
children: [{ id: "1-1", label: "花果园" }]
},
{
id: "2",
label: "观山湖区",
children: [
{ id: "2-1", label: "长岭街道办" },
{ id: "2-2", label: "金阳街道办" },
{ id: "2-3", label: "金华镇" },
{ id: "2-4", label: "金华园街道办" }
]
}
],
defaultProps: { children: "children", label: "label" },
handleNodeClick(e) {
console.log(e.id);
},
在 style 中
.tree-box {
width: 200px;
}
.el-tree-node__content {
padding: 5px 0;
border: 1px solid #ebf0f3;
}
.el-tree-node.is-current > .el-tree-node__content .el-tree-node__expand-icon {
color: #0c5cff;
}
.el-tree-node__content:hover {
color: #0c5cff;
background: #e0eaff;
}
.el-tree-node.is-current > .el-tree-node__content {
color: #0c5cff;
background-color: #e0eaff;
}
.el-tree-node__expand-icon.is-leaf {
color: transparent !important;
}
Tree 树形菜单组件
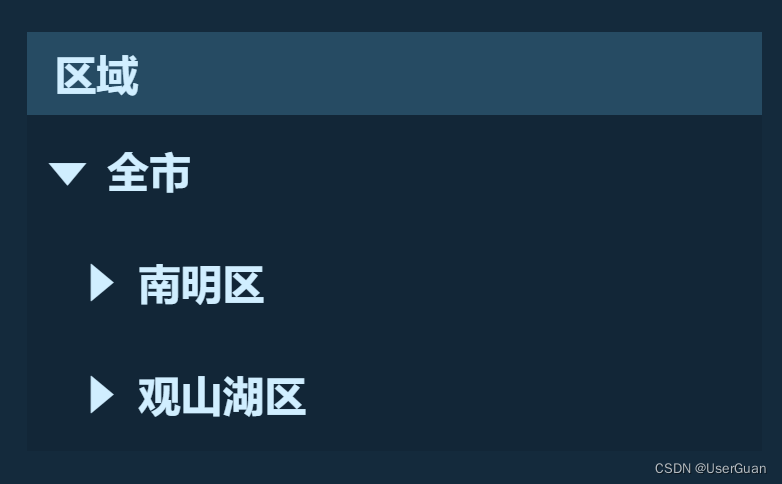
在 template 中
<tree-menu
key="menu1"
high-prefix="max"
high="1039"
title="区域"
:tree-list="areaList"
:default-expanded-keys="['0']"
@nodeClick="areaChoose"
/>
在 script 中
/* 树形菜单 */
areaList: [
{
id: "0",
label: "全市",
children: [
{
id: "1",
label: "南明区",
children: [{id: "1-1", label: "花果园"}]
},
{
id: "2",
label: "观山湖区",
children: [
{id: "2-1", label: "长岭街道办"},
{id: "2-2", label: "金阳街道办"},
{id: "2-3", label: "金华镇"},
{id: "2-4", label: "金华园街道办"}
]
}
]
},
], // 区域
/* 树形菜单 */
areaChoose(e) {
// 区域菜单
console.log(e)
},
组件源码
<!-- 树形菜单 -->
<template>
<div class="tree-box box-roll-vertical" :style="`${highPrefixCalc(highPrefix)}height: ${high}px`">
<div class="top-tip">{{ title }}</div>
<el-tree
:data="treeList"
:props="treeProps"
:node-key="nodeKey"
:default-expand-all="defaultExpandedKeys.length > 0 ? false : defaultExpandAll"
:default-expanded-keys="defaultExpandedKeys"
:accordion="accordion"
@node-click="handleNodeClick"
/>
</div>
</template>
<script>
export default {
props: {
/** 菜单最大高度
* 默认:324,单位px
*/
high: {
type: [Number, String],
default: 324
},
/** 高度前缀
* 默认:"",可取值:max(最大高度),min(最小高度)
*/
highPrefix: {
type: String,
default: ''
},
/** 顶部标题
* 默认:""
*/
title: {
type: String,
default: ""
},
/** 配置选项
* 默认:{children: "children", label: "label"}
*/
treeProps: {
type: Object,
default: () => ({children: "children", label: "label"})
},
/** 每个树节点用来作为唯一标识的属性,整棵树应该是唯一的(默认展开的节点的 key 的数组中的 key 值就是 nodeKey 这个值)
* 默认:id
*/
nodeKey: {
type: String,
default: "id"
},
/** 是否默认展开所有节点
* 默认:false
*/
defaultExpandAll: {
type: Boolean,
default: false
},
/** 默认展开的节点的 key 的数组
* 默认:[]
*/
defaultExpandedKeys: {
type: Array,
default: () => ([])
},
/** 是否每次只打开一个同级树节点展开
* 默认:true
*/
accordion: {
type: Boolean,
default: true
},
/** 展示的数据
* 默认:[]
*/
treeList: {
type: Array,
default: () => ([])
}
},
data() {
return {}
},
methods: {
/** 高度前缀计算
* @param e
*/
highPrefixCalc(e) {
let result = ''
if (e === 'max' || e === 'min') result = `${e}-`
return result
},
/** 节点被点击时的回调
* @param e 返回值
*/
handleNodeClick(e) {
this.$emit("nodeClick", e)
}
}
}
</script>
<style lang="scss" scoped>
$baseColor: #D0EEFF; // 字体颜色
$baseBg: rgba(16, 33, 48, 0.4); // 背景颜色
$selectedBg: rgba(16, 33, 48, 0.4); // 选中时的背景颜色
.tree-box {
width: 420px;
.top-tip {
color: $baseColor;
font-size: 24px;
padding: 8px 16px;
background: rgba(112, 204, 255, 0.2);
backdrop-filter: blur(10px);
font-weight: bold;
}
.el-tree {
background: $baseBg;
}
::v-deep .el-tree-node__expand-icon {
font-size: 34px;
color: $baseColor;
}
::v-deep .el-tree-node__expand-icon.is-leaf {
color: transparent;
}
::v-deep .el-tree-node__content {
height: 64px;
backdrop-filter: blur(10px);
}
::v-deep .el-tree-node:focus > .el-tree-node__content,
::v-deep .el-tree-node__content:hover {
background: $selectedBg;
}
::v-deep .el-tree-node__label {
color: $baseColor;
font-size: 24px;
font-weight: bold;
}
}
/* 上下滚动条 */
.box-roll-vertical {
overflow-y: auto;
overflow-x: hidden;
}
.box-roll-vertical::-webkit-scrollbar {
width: 2px;
}
.box-roll-vertical::-webkit-scrollbar-track,
.box-roll-vertical::-webkit-scrollbar-thumb:hover {
background: #f2f2f2;
}
.box-roll-vertical::-webkit-scrollbar-thumb,
.box-roll-vertical::-webkit-scrollbar-thumb:active {
background: rgba(0, 0, 0, 0.2);
}
</style>