JS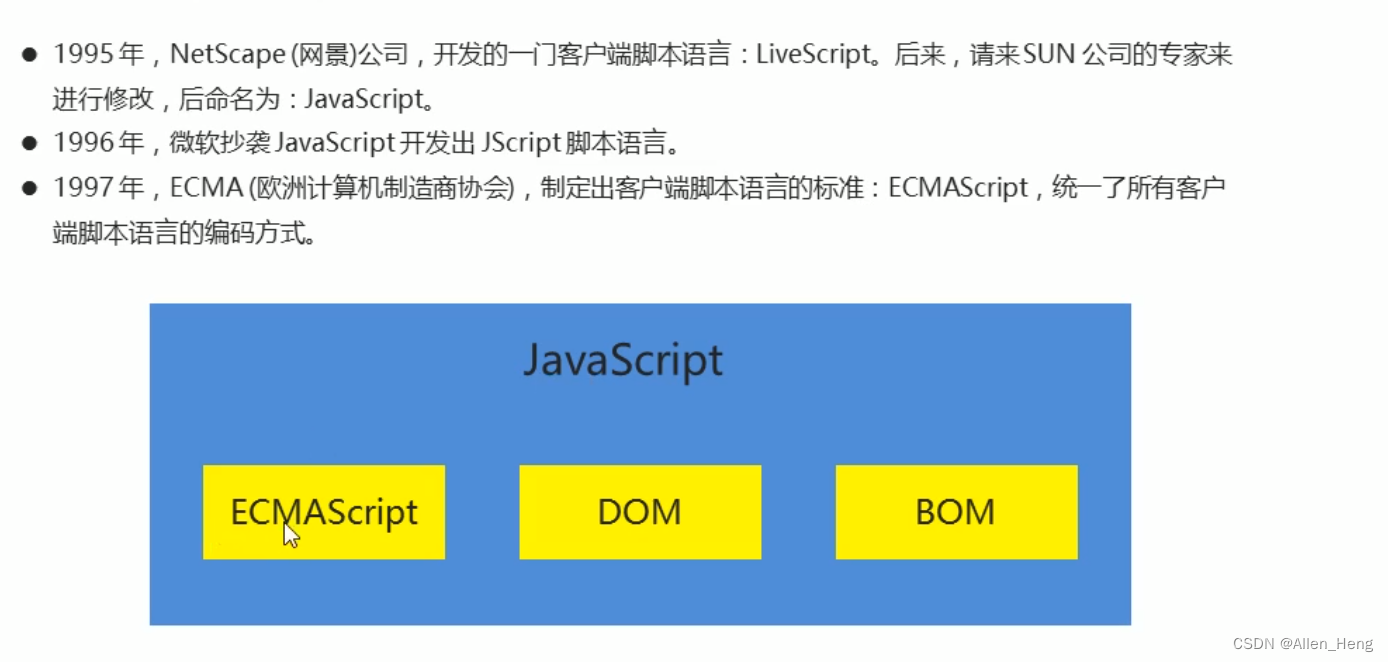

环境搭建
基本语法:
注释:
// 单行注释
/* 多行注释 */
输入输出语句
// 输入输出语句
document.getElementById("btn").onclick = function(){
// 输入语句
var message = prompt("输入信息")
var insure = confirm("是否确认?")
// 控制台打印日志欲绝
console.log(insure+"确认结果为");
// 警告框弹出语句
alert("打印日志呀!~~~"+message);
// 页面写出信息语句
document.write(message+"<br>");
document.write("是你输入的信息");
}
变量常量
let变量 作用在{} 内 变量 作用在全局 常量只能被赋值一次 var相同不过可以重复定义
// 变量和常量
document.getElementById("btn2").onclick = function(){
// 常量不能声明在局部代码块里 且不能改变其值
const v3= "ccc";
{
// 局部变量
let v1 = "aaa";
// 全局变量
v2 = "bbb";
// 常量
// 局部变量只能在 他所在的最近{} 中使用
document.write(v1+"<br>");
}
document.write(v2+"<br>");
document.write(v3+"<br>");
}
数据类型
// 原始数据类型
document.getElementById("btn3").onclick = function(){
// 常量不能声明在局部代码块里 且不能改变其值
document.write(typeof("aaa")+"<br>"); // String
var bbb = null;
document.write(typeof(bbb)+"<br>"); // 返回object 是js原始错误
document.write(typeof(1)+"<br>"); // int
document.write(typeof(1n)+"<br>"); // bingInt
document.write(typeof(true)+"<br>"); // boolean
var cc ;
document.write(typeof(cc)+"<br>"); // undifind
var ddd =[1,2,3,4,5]
document.write(typeof(ddd)+"<br>"); // object
fa(); // 调用匿名函数
}
typeof(goUpTime) === 'undefined' 判断变量是否被定义
算数运算符
比较运算符:
// 运算符
document.getElementById("btn4").onclick = function(){
// 常量不能声明在局部代码块里 且不能改变其值
var a ="10";
document.write(a+"5"+"<br>"); // String + string 字符串拼接
document.write(a+5+"<br>"); // String + number 字符串拼接
document.write(a-5+"<br>"); // String - number string-》int 计算
document.write(a*5+"<br>"); // String * number string-》int 计算
document.write(a/5+"<br>"); // String / number NaN2
document.write(a%3+"<br>"); // String % number string-》int 计算
var b= 10;
document.write((a==b )+"<br>"); // String -> int 比较值
document.write((a===b )+"<br>"); // 会比较数据类型 和值
}
typeof(goUpTime) === 'undefined' 判断变量是否为未定义
逻辑运算符
流程控制语句
if switch
for while do while
都同java
三元运算符
// 流程控制语句 注意 字符串比较的是有 不能连比 例如 1<month<5 要写成 1<month && month<5
document.getElementById("btn5").onclick = function(){
var month = prompt("请输入一个月份");
var session2 = 0<month && month<13 ? "数据合法" :"输入数据不合法";
document.write(session2 +"<br>")
var session;
var sessionSwitch;
if(3<=month && month<=5){
session = "春季";
}else if(6<=month && month<=8){
session = "夏季";
}else if(9<=month && month<=11){
session = "秋季";
}else if(1<=month && month<=2 || month ==12 ){
session = "冬季";
}else{
session="输入有误";
}
document.write(session+"if 语句 <br>")
var month2 =parseInt(month) ;
switch(month2){
case 3:
case 4:
case 5: sessionSwitch ="春季";
break;
case 6:
case 7:
case 8: sessionSwitch ="夏季";
break;
case 9:
case 10:
case 11: sessionSwitch ="秋季";
break;
case 12:
case 1:
case 2: sessionSwitch ="冬季";
break;
default: sessionSwitch ="输入有误";
break;
}
document.write(sessionSwitch+"switch语句 <br>")
}
// 循环语句
document.getElementById("btn6").onclick = function(){
var dddArray =[1,2,3,4,5]
for (var index = 0; index < dddArray.length; index++) {
var element = dddArray[index];
document.write(element+"<br>")
}
document.write("for 循环 <br>")
var index = 0;
while (index<dddArray.length) {
document.write(dddArray[index]+"<br>")
index++;
}
document.write("while循环<br>")
index = 0 ;
do {
document.write(dddArray[index]+"<br>")
index++;
} while (index<dddArray.length);
document.write("do while循环<br>")
}
数组
/ 数组操作
document.getElementById("btn7").onclick = function(){
var aaaArray =[1,2,3,4,5]
var dddArray =[...aaaArray] // 使用 ... 对数组赋值 注意这里是赋值 不是赋地址值
dddArray[dddArray.length] = 10; // 数组长度可变 索引位置只能是数组的长度 超过了不可以
printArray(aaaArray,"数组添加");
printArray(aaaArray,"数组复制");
var cccArray =[6,7,8,9,10];
var cccArray = [...cccArray,...aaaArray]; // 两个数组合并为一个数组
printArray(cccArray,"数组合并");
var fffarray = splitArray("," ,"aaa,bbb,ccc" ,"ddd,eee,fff"); // 将字符串切割为字符数组
printArray(fffarray,"字符串切割为数组");
}
function testArray(){
console.log("Array:");
arr =[1,2,3,7,4,5,6];
console.log(arr.push(8)); // 输出的是新增到尾部的值
console.log(arr.pop()); // 输出的是去除的尾部元素的值
console.log(arr.shift(8)); // 输出的是去除的首部元素
console.log(arr.includes(8));
console.log(arr.sort());
console.log(arr.reverse(8));
}
函数
// 有返回值 和 可变参 的函数 根据传入的 标识 和 字符串数组 切割字符串
function splitArray(splitFloge,...str){
var tatalstr =""; // 注意此处没有初始化 后面字符串的值会是 undifend
for (let index = 0; index < str.length; index++) {
tatalstr= tatalstr+str[index];
}
return tatalstr.split(splitFloge);
}
// 函数/方法 遍历数组 打印信息
function printArray(arrayTemplate,message){
for (var index = 0; index < arrayTemplate.length; index++) {
var element = arrayTemplate[index];
document.write(element+"<br>")
}
document.write(message+"<br>")
}
this可以指当前元素对象( onclick ="fun(this)")
DOM
Element
获取元素
// Element 元素进行操作 注意elements 获取的是数组对象
document.getElementsByName("btn8")[0].onclick = function (){
var getById = document.getElementById("div1");
console.log("根据id获取元素"+getById);
var getByTagName = document.getElementsByTagName("div");
console.log("根据标签名获取元素"+getByTagName.length);
getDivId(getByTagName);
var getByName = document.getElementsByName("div2");
console.log("根据元素名称获取元素"+getByName.length);
var getByClassName = document.getElementsByClassName("div3");
console.log("根据class属性获取元素"+getByClassName.length);
var getParent = getById.parentElement;
console.log("根据字标签获取父标签元素"+getParent);
}
function getDivId(elements){
for (let index = 0; index < elements.length; index++) {
let element = elements[index];
console.log("元素的id值为:"+element.getAttribute("id"));
}
}
元素增删改
// Element 对元素进行增删改操作
document.getElementById("btn9").onclick = function (){
// 创建元素
createOpt = document.createElement("option")
createOpt.innerText="青岛";
selectDoc = document.getElementById("changeOpt")
// 添加元素
selectDoc.appendChild(createOpt);
// 删除元素
optDel = document.getElementById("optDel");
selectDoc.removeChild(optDel);
// 更改元素
optOld = document.getElementById("optChange");
newOpt = document.createElement("option")
newOpt.innerText="烟台";
selectDoc.replaceChild(newOpt,optOld);
}
Attribute属性操作
// 更改元素属性 样式
document.getElementById("btn10").onclick=function(){
taga = document.getElementsByTagName("a")[0];
taga.setAttribute("href","https://youngchan.com")
console.log("href标签的属性值为"+taga.getAttribute("href"))
taga.setAttribute("href","https://www.youngchan2.com");
taga.removeAttribute("title");
taga.style.fontSize="20px";
taga.className="classA";
}
createTextNode(创建文本元素)
文本操作
// 更改文本内容
document.getElementById("btn11").onclick=function(){
tagb = document.getElementsByTagName("b")[0];
tagb.innerText="innerText";
tagb2 = document.getElementsByTagName("b")[1];
tagb.innerHtml="<a href='hahhah'>innerHtml</a>";
}
documentElement.value; 获取表单信息 documentElement.innerHtml 获取文本信息
事件:
对象:
// 自定义对象
class Person{
constructor(name ,age){
this.name = name;
this.age = age;
}
say() {
console.log(this.name+","+this.age);
}
}
// 继承
class student extends Person{
constructor(name ,age ,score){
super(name,age);
this.score = score;
}
// 方法会被重写
say() {
console.log(this.name+","+this.age+","+this.score);
}
}
// 字面量定义和使用
var weather = {
oDate : "",
city :"济南",
isSUn : "晴",
wendu : "2-5",
shidu : "20%",
say : function(){
console.log(this.oDate+","+this.city+","+this.isSUn+","+this.wendu+","+this.shidu);
return ;
}
}
// json类使用 Date
document.getElementById("btn12").onclick=function(){
var stu = new student("小小",29,98);
stu.say();
weather.oDate = Date(2022,11,3,17,28);
weather.say();
// 对象转json
weatherJson = JSON.stringify(weather)
console.log(weatherJson);
// Json转对象 注意转为对象后没有对象的方法
weatherJson =weatherJson.replace("晴","多云");
var todayWeather = JSON.parse(weatherJson)
console.log(todayWeather.isSUn);
}
内置对象 (工具类)
// 转int 转float
function testNumber(){
console.log("Number:");
console.log(Number.parseInt("111aaa")); // 遇到字符串停止
console.log(Number.parseInt("aa111aaa")); // NaN
console.log(Number.parseInt("1"));
console.log(Number.parseFloat("1.11"));
}
function testMath(){
console.log("Math:");
console.log(Math.ceil(1.1));
console.log(Math.floor(1.1));
console.log(Math.round(1.4));
console.log(Math.round(1.5));
console.log(Math.pow(2,3));
console.log(Math.random());
}
Date 注意使用date 需要new
// 注意date 的使用 需要new 对象
function testDate(){
console.log("Date:");
// 三种构造
var aDate = new Date(2022,11,3,17,28);
console.log(new Date());
console.log(new Date(3600));
// 方法
console.log(aDate.toLocaleString());
console.log(aDate.getTime());
console.log(aDate.getFullYear());
console.log(aDate.getMonth()+1);
console.log(aDate.getDate());
console.log(aDate.getMinutes());
console.log(aDate.getSeconds());
}
function testString(){
console.log("String:");
aString = String("haha");
bString = "今天天气好晴朗";
console.log(bString.length);
console.log(bString.charAt(0));
console.log(bString.indexOf("天"));
console.log(bString.lastIndexOf("天"));
console.log(aString.substring(0,3));
console.log(aString.split("a")); // 最后出现空串
console.log(aString.replace("a","e")); // 只更改第一个
}
function testArray(){
console.log("Array:");
arr =[1,2,3,7,4,5,6];
console.log(arr.push(8)); // 输出的是新增到尾部的值
console.log(arr.pop()); // 输出的是去除的尾部元素的值
console.log(arr.shift(8)); // 输出的是去除的首部元素
console.log(arr.includes(8));
console.log(arr.sort());
console.log(arr.reverse(8));
}
function testset(){
console.log("Set:");
s = new Set();
s.add("a");
s.add("b");
s.add("b");// 同值 添加不进去
s.add("c");
s.delete("a");
skey = s.keys(); // 获取迭代器对象
for (let index = 0; index < s.size; index++) {
console.log(skey.next().value); // 迭代器对象获取值
}
}
function testMap(){
console.log("Map:");
mMap = new Map();
mMap.set("a","1");
mMap.set("b","2");
mMap.set("b","3");// 同key 修改值
mMap.set("c","4");
console.log(mMap.get("b"));
mEntries = mMap.entries() // 获取entry 对象
for (let index = 0; index < mMap.size; index++) {
console.log(mEntries.next().value); // entry 对象获取值
}
}
// 字面量定义和使用
var weather = {
oDate : "",
city :"济南",
isSUn : "晴",
wendu : "2-5",
shidu : "20%",
say : function(){
console.log(this.oDate+","+this.city+","+this.isSUn+","+this.wendu+","+this.shidu);
return ;
}
}
// json类使用 Date
document.getElementById("btn12").onclick=function(){
var stu = new student("小小",29,98);
stu.say();
weather.oDate = Date(2022,11,3,17,28);
weather.say();
// 对象转json
weatherJson = JSON.stringify(weather)
console.log(weatherJson);
// Json转对象 注意转对象后会无法使用对象的方法
weatherJson =weatherJson.replace("晴","多云");
var todayWeather = JSON.parse(weatherJson)
console.log(todayWeather.isSUn);
}
表单校验
return true 提交 false 不提交
BOM
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>定时跳转</title>
<script>
function changeSecond() {
var num = 5;
var docSpan = document.getElementById("second");
num = Number.parseInt(docSpan.textContent);
num--;
docSpan.innerText = num;
// var number = document.createTextNode(num);
// docSpan.appendChild(number);
if (num <= 0) {
location.href = "demo1.html";
}
}
window.onload = function () {
window.setInterval("changeSecond()", 1000);
}
</script>
</head>
<body>
<span id="second" style="color: green;"> 5</span>后跳转页面
</body>
</html>
封装:
案例代码 1 . 登录验证
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>登录验证</title>
</head>
<body>
<div style="text-align: center;">
<form id="myForm" method="get" autocomplete="false" action="demo1.html">
<input type="text" id="userName1" placeholder="请输入用户名" />
<br> <input type="password" id="password1" placeholder="请输入密码">
<br> <input type="submit" id="mySubmit" value="提交">
</form>
</div>
</body>
<script>
// onsubmit 和submit
document.getElementById("myForm").onsubmit = function () {
userName = document.getElementById("userName1").value;
password = document.getElementById("password1").value;
petten1 = /^[a-z0-9_-]{3,16}$/
petten2 = /^[a-z0-9_-]{6,18}$/;
if (!petten1.test(userName)) {
alert("用户名不合规")
return false;
}
if (!petten2.test(password)) {
alert("密码不合规")
return false;
}
return true;
}
</script>
</html>
滚动图片 定时器 + 按钮 版
<!DOCTYPE html>
<html lang="en">
<head>
<!-- <script src="js/my.js"/> -->
<meta charset="UTF-8">
<!-- <meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0"> -->
<title>JavaScript</title>
<style>
.classA {
color: aqua;
}
</style>
</head>
<body>
<button id='btn' name="btn"> 输入输出</button>
<button id='btn2' name="btn2"> 变量常量</button>
<button id='btn3' name="btn3"> 数据类型</button>
<button id='btn4' name="btn4"> 运算符</button>
<button id='btn5' name="btn5"> 流程控制</button>
<button id='btn6' name="btn6"> 循环</button>
<button id='btn7' name="btn7"> 数组</button>
<br> <br>
<div id='div1'>
<button id='btn8' name="btn8"> 获取div 信息</button>
<div name='div2' id="div2"></div>
<div name='div2' id="div3"></div>
<div class='div3' id="div4"></div>
<div class='div3' id="div5"></div>
</div>
<br><br>
<button id='btn9' name="btn9"> 更改元素 信息</button>
<select id="changeOpt">
<option>更改元素中元素</option>
<option id="optChange">请选择</option>
<option>济南</option>
<option>济宁</option>
<option>泰安</option>
<option id="optDel">需要删除的</option>
</select>
<br><br>
<button id='btn10' name="btn10"> 更改元素属性</button>
<a title="原始title信息"> 更改元素属性</a>
<button id='btn11' name="btn11"> 更改元素文本内容</button>
<b> text更改元素文本内容</b>
<b> html更改元素文本内容</b><br><br>
<button id='btn12' name="btn12"> 自定义类 转json 输出控制台</button>
<br>
<br>
<button id='btn13' name="btn13">工具类</button>
<br>
<br>图片 点击或定时 轮播
<br>
事件:<button id="up">上一张</button>
<img id="imgId" src="img/1.jpg" style="width: 600px;height: 300px;">
<button id="next">下一张</button>
</body>
<script src="js/my.js"></script>
</html>
// 对图片轮播的实现
const imgUrlArray = ["img/1.jpg","img/2.jpg","img/3.jpg","img/4.jpg","img/5.jpg","img/6.jpg","img/7.jpg"];
// 绑定点击事件
document.getElementById("up").onclick = upImg;
document.getElementById("next").onclick = nextImg;
// 向上切换图片
function upImg(){
var imgDoc = document.getElementById("imgId");
var imgUrl = imgDoc.getAttribute("src");
var i =imgUrlArray.length-2;
for (let index = 0; index < imgUrlArray.length; index++) {
if(imgUrlArray[index] == imgUrl){
i = index;
}
}
if(i == (imgUrlArray.length-1)){
document.getElementById("next").removeAttribute("disabled");
}
if(i == 1){
// 按钮隐藏 按钮 失效
document.getElementById("up").style.display="none" ;
// document.getElementById("up").setAttribute("disabled",true);
// 开启向下自动轮播
goNext();
}
imgDoc.setAttribute("src",imgUrlArray[i-1]);
}
// 向下切换图片
function nextImg(){
var imgDoc = document.getElementById("imgId");
var imgUrl = imgDoc.getAttribute("src");
var j =1;
for (let index = 0; index < imgUrlArray.length; index++) {
if(imgUrlArray[index] == imgUrl){
j = index;
}
}
if(j == 0){
// 按钮 显示 // 按钮开启
document.getElementById("up").style.display="" ;
// document.getElementById("up").removeAttribute("disabled");
}
if(j == imgUrlArray.length-2){
document.getElementById("next").setAttribute("disabled",true);
// 开启向上自动轮播
goUp();
}
imgDoc.setAttribute("src",imgUrlArray[j+1]);
}
// 定时任务 向下切换图片
function goNext(){
if(typeof(goUpTime) === 'undefined'){
}else{
clearInterval(goUpTime);
}
gonextTime = window.setInterval("nextImg()",3000);
}
// 定时任务向上切换图片
function goUp(){
if(typeof(gonextTime) === 'undefined'){
}else{
clearInterval(gonextTime);
}
goUpTime = window.setInterval("upImg()",3000);
}
// 定时任务开启图片轮播
window.onload = function(){
document.getElementById("up").style.display="none" ;
var start = window.setTimeout("goNext()",1000)
}