一、需求
有一只羊,姓名,年龄,id
复制10只属性相同的羊
二、传统方式
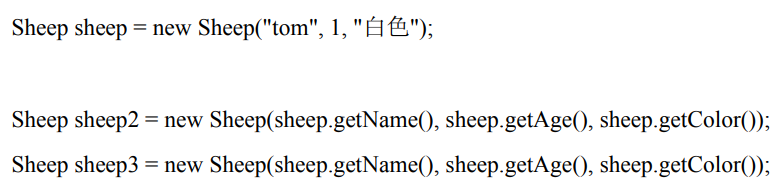
优点:易于理解
缺点:创建的对象复杂,效率低
改进:Java中所有的类都是Object的子类,使用Object中提供的clone方法,实现Cloneable接口
三、原型模式
步骤一 重写Sheep的clone方法
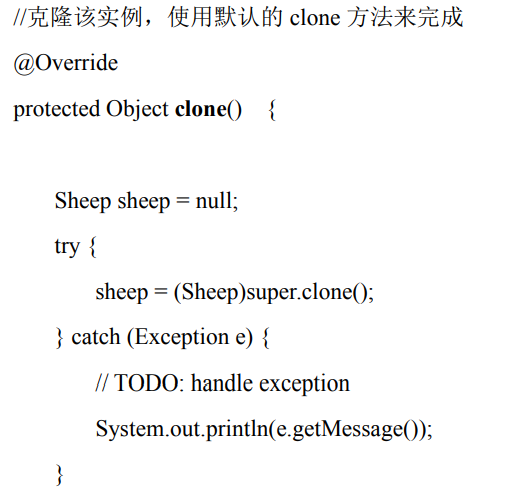
步骤二 调用Sheep的clone方法
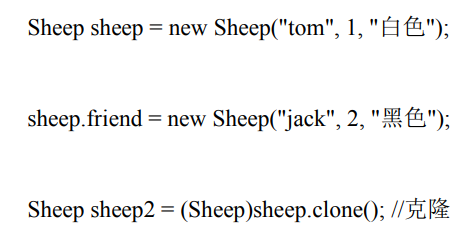
四、Spring中使用
Spring中bean创建
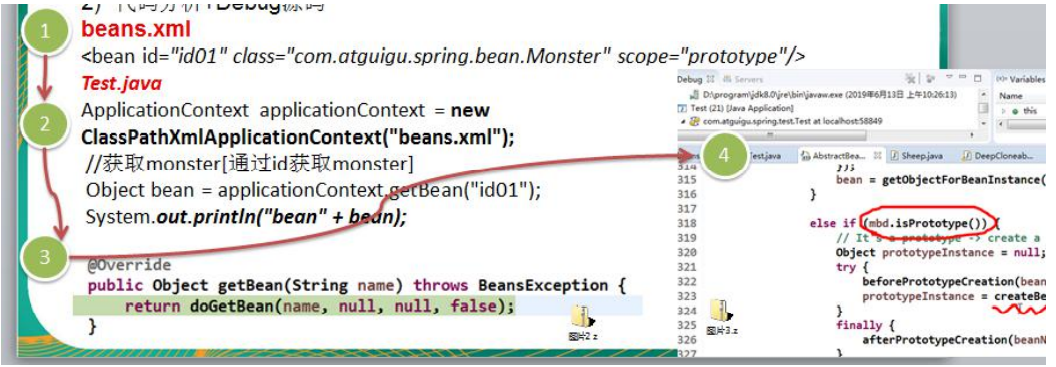
五、深拷贝、浅拷贝
引用对象
深拷贝:复制一份一样的对象 hashCode(objA) != hashCode(objB)
浅拷贝: 引用对象地址相同,修改objA 也会影响 objB
思考:如果Sheep中存在引用对象,拷贝后,是深拷贝还是浅拷贝
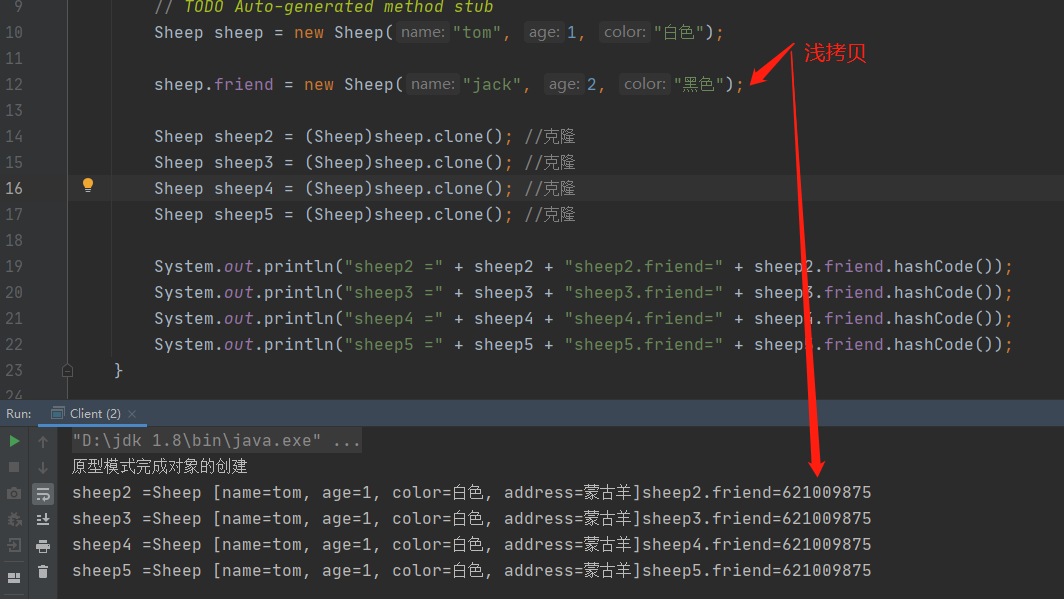
如何完成super.clone中的深拷贝?
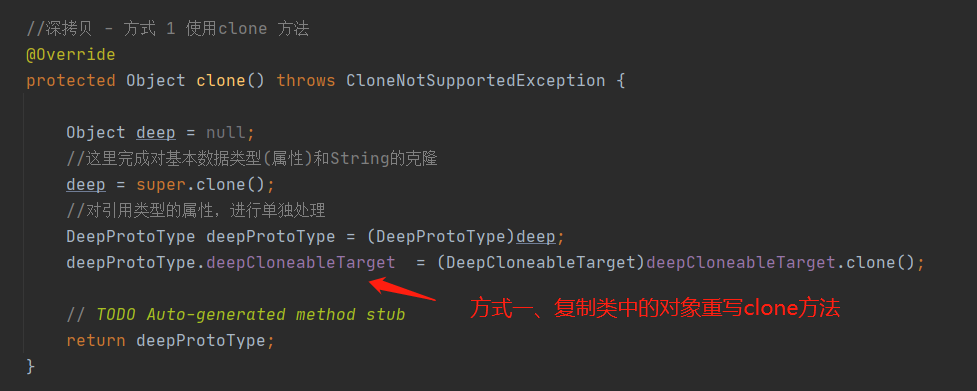
方式二、使用序列化
//深拷贝 - 方式2 通过对象的序列化实现 (推荐
public Object deepClone() {
//创建流对象
ByteArrayOutputStream bos = null;
ObjectOutputStream oos = null;
ByteArrayInputStream bis = null;
ObjectInputStream ois = null;
try {
//序列化
bos = new ByteArrayOutputStream();
oos = new ObjectOutputStream(bos);
oos.writeObject(this); //当前这个对象以对象流的方式输出
//反序列化
bis = new ByteArrayInputStream(bos.toByteArray());
ois = new ObjectInputStream(bis);
DeepProtoType copyObj = (DeepProtoType)ois.readObject();
return copyObj;
} catch (Exception e) {
// TODO: handle exception
e.printStackTrace();
return null;
} finally {
//关闭流
try {
bos.close();
oos.close();
bis.close();
ois.close();
} catch (Exception e2) {
// TODO: handle exception
System.out.println(e2.getMessage());
}
}
}