C++ Boost regex 文档(翻译)(Reference进行中) Copyright ? John Maddock 1998-2003 译者:nirvana C++ Boost regex 文档(翻译)(Reference进行中) Overview Configuration and setup Installation Borland C++ Builder Microsoft Visual C++ GNU G++(2.95 and 3.x) Sun Forte Compiler 6.1 Other compilers (building with bjam) Reference Types syntax_option_type error_type match_flag_type class regex_error class regex_traits class template basic_regex class template sub_match class template match_results Algorithms regex_match regex_search regex_replace Iterators regex_iterator regex_token_iterator Typedefs regex [ = basic_regex ] wregex [ = basic_regex ] cmatch [ = match_results ] wcmatch [ = match_results ] smatch [ = match_results ] wsmatch [ = match_results ] cregex_iterator [ = regex_iterator] wcregex_iterator [ = regex_iterator] sregex_iterator [ = regex_iterator] wsregex_iterator [ = regex_iterator] cregex_token_iterator [ = regex_token_iterator] wcregex_token_iterator [ = regex_token_iterator] sregex_token_iterator [ = regex_token_iterator] wsregex_token_iterator [ = regex_token_iterator] Misc. POSIX API Compatibility Functions Partial matches Regular Expression Syntax Format String Syntax Understanding Captures Deprecated interfaces class regbase class template reg_expression Algorithm regex_grep Algorithm regex_format Algorithm regex_merge Algorithm regex_split class RegEx FAQ Appendix Implementation Thread Safety Localisation Examples Headers Redistributables and Library Names Standards Conformance History Performance Contacts and Acknowledgements Overview 正则表达式是一种模式匹配的形式,经常用于文本处理;很多用户熟悉Unix下的grep,sed和awk,以及perl语言,它们都大量应用了正则表达式。传统的C++用户要操作正则表达式,只能使用POSIX C API,regex++提供了这些API,但那并不表明那是使用regex库的最好方法。regex++能够处理宽字符串或是以类似于sed或perl的方式进行搜索和替换,这是传统的C函数库们做不到的。 类boost::basic_regex是库中的关键类;它代表一个机器可读的正则表达式,并且非常类似于std::basic_string的模型,可以把它看作一个增强字符串,一个真正的正则表达式所需的状态机。象std::basic_string一样,它有两个typedef,这几乎总是这个类被引用的意义: namespace boost{ template <class charT, class traits = regex_traits<charT>, class Allocator = std::allocator<charT> > class basic_regex; typedef basic_regex<char> regex; typedef basic_regex<wchar_t> wregex; } 要弄明白这个库是如何使用的,请假定我们在写一个信用卡处理程序,信用卡号通常是一个16位数字的字符串,4个一组,以/ /或/-/分开。在象数据库存储一个信用卡号之前(有些事并非必要,不过客户会很欣赏!),我们应该校验卡号是否是正确的格式。为了匹配任一数字,我们可以用正则表达式[0-9],但是字符范围是依赖于本地化的。替之以使用POSIX标准形式[[: Digit:]],或是regex++和perl形式的简写d(注意:许多老的库会使用硬编码的C形式的本地化,因此这对他们不是一个问题)。让我们使用如下的正则表达式校验信用卡号的格式: (d{4}[- ]){3}d{4} 在这里圆括号的作用是组成子表达式(未来可能用作标记匹配),{4}意思是“精确匹配四次”。这是一个用于perl、awk和eprep的扩展正则表达式句法。regex++也支持sed和grep使用的更老的“基本”句法,不过一般没什么用处,除非你有一些古老的表达式需要重用。 现在,让我们把那个表达式放进一段校验信用卡号的代码里: bool validate_card_format(const std::string s) { static const boost::regex e("(d{4}[- ]){3}d{4}"); return regex_match(s, e); } 请注意,必须在表达式里加入一些转义符(//):记住在正则表达式引擎处理转义字符之前,C++编译器会先处理一次,所以在嵌入CC++代码中时,转义字符必须被double一下。也请注意,所有的例子都是基于你的编译器支持Koenig lookup的基础上,如果不是的话(象VC6),你就得向函数调用中添加boost:: prefixes。 如果你熟悉信用卡处理机制,你会意识到当上面的格式符合人们读卡习惯的时候,未必等于那可以被在线支付系统接受;这需要把号码转换为一个16位数字(也可能是15位)的字符串,中间没有任何空格。我们需要的时可以方便的在两种格式间转换,这就是search和replace起作用的地方。熟悉sed和Perl的人们已经领先一步了。我们需要两种字符串————一种是正则表达式,另一种是格式字符串,它提供了对被替换文本的表述。在regex++中,这里的search和replace操作由算法regex_replace执行,对于我们的信用卡的例子,可以写成如下的两个算法来进行格式转换: // match any format with the regular expression: const boost::regex e("A(d{3,4})[- ]?(d{4})[- ]?(d{4})[- ]?(d{4})z"); const std::string machine_format("1234"); const std::string human_format("1-2-3-4"); std::string machine_readable_card_number(const std::string s) { return regex_replace(s, e, machine_format, boost::match_default | boost::format_sed); } std::string human_readable_card_number(const std::string s) { return regex_replace(s, e, human_format, boost::match_default | boost::format_sed); } 这里我们已经使用了被标记的子表达式来分离信用卡号的四个独立的部分,格式字符串使用类似sed的句法去替换以被格式化的文本。 上面的例子,我们不能直接操作匹配的结果,但通常匹配的结果出了整个匹配之外,还包含一系列子匹配。当需要报告一个正则表达式匹配的时候,它使用一个match_results的实例来完成,象以前那样,这个类的typedef们足以应付大多数情况: namespace boost{ typedef match_results<const char*> cmatch; typedef match_results<const wchar_t*> wcmatch; typedef match_results<std::string::const_iterator> smatch; typedef match_results<std::wstring::const_iterator> wsmatch; } regex_search和regex_match算法充分利用了match_results报告匹配结果;不同之处在于后者仅仅使用全部输入文本发现是否匹配,而前者会在文本中查找出一个匹配。 这些算法并不仅限于搜索正规的C字符串,任何双向迭代器类型都可以被搜索,无缝搜索几乎任何类型的数据都是允许的。 对于搜索和替换操作,除了我们已经看到的regex_replace,match_results类有一个格式化成员函数(regex_format)获取匹配结果和一个格式字符串,并且结合二者生成一个新字符串。 要迭代一个表达式匹配文本的所有结果, 我们有两个迭代器类型:regex_iterator枚举已匹配的match_results对象,而regex_token_iterator枚举一系列的字符串(类似于perl形式的split)。 对于那些讨厌模板的家伙,有一个高阶的封装类RegEx,它封装了低层的模板,提供了简单的界面给那些不需要这个库全部威力的人,但是仅仅支持窄字符集和扩展正则表达式句法。不过这个类已经被弃用,因为它不是正则表达式C++标准库提案的一部分。 POSIX API函数:regcomp, regexec, regfree and regerror,在窄字符集和unicode 版本下均可用,这些由需要这些API兼容的人提供。 最后,注意库现在已经支持运行时本地化和识别完全POSIX正则表达式句法————包括一些更先进的特征,如多字符比较元素和等价类————也提供对于其他正则库的兼容,比如GNU和BSD4正则包,和对perl5扩展形式的有限支持。 Configuration and setup Contents 编译器设置 本地化与traits选择 链接选项 算法选择 算法调优 Compiler setup. 不必对boost.regex做任何针对你编译器的特别设置——boost.config子系统已经考虑到这些了,如果你有什么问题(或是对自己的编译器和平台有不明白的地方),可以参看boost.config的设置脚本。 Locale and traits class selection. 下面的宏(参见user.hpp)控制着boost.regex与用户本地设置的交互: BOOST_REGEX_USE_C_LOCALE 强迫 boost.regex在它的traits类支持中使用全局C本地化: 这是默认非windows平台的行为,但是在windows平台上一般使用win32 API来完成本地支持。 BOOST_REGEX_USE_CPP_LOCALE 强迫boost.regex使用std::locale,正则表达式使用指定的本地化实例。 BOOST_REGEX_NO_W32 告诉boost.regex不使用任何 Win32 API 即使可用 (意即使用BOOST_REGEX_USE_C_LOCALE 除非设置了 BOOST_REGEX_USE_CPP_LOCALE). Linkage Options BOOST_REGEX_DYN_LINK 对于MSVC和Borland C++ builder,这个宏告知 boost.regex应该链接到boost.regex的动态链接库。boost.regex默认是静态链接即使使用了动态C运行时库。 BOOST_REGEX_NO_LIB 对于MSVC和Borland C++ builder, ,这个宏告知 boost.regex不要自动选择要连接的库。 Algorithm Selection BOOST_REGEX_V3 告诉boost.regex使用1.30版本的匹配算法,仅仅在你需要最大程度兼容旧的行为时定义它。 BOOST_REGEX_RECURSIVE 告诉boost.regex使用栈-递归算法。这通常是最快的选择(虽然快不了多少),但是在极端情况下有可能导致栈溢出,在win32平台应该尽量安全的使用它,其他平台不存在这个问题。 BOOST_REGEX_NON_RECURSIVE 告诉boost.regex不使用栈-递归算法。这可能速度上稍逊,但无论正则表达式有什么样的问题,代码总是安全的.这在非win32平台是默认的行为。 Algorithm Tuning 下面的选项仅在设置了BOOST_REGEX_RECURSIVE的时候使用。 BOOST_REGEX_HAS_MS_STACK_GUARD 告诉boost.regex支持MS形式的__try - __except块,可以安全的捕获栈溢出。 下面的选项仅在设置了BOOST_REGEX_NO_RECURSIVE的时候使用。 BOOST_REGEX_BLOCKSIZE 在非递归模式中,boost.regex使用较大的内存块作为状态机的栈,块越大,内存分配次数就越少。默认是4096B,对于大多数正则表达式,这足够大了,但是,你可能根据你的平台特性选择更小或更大的值。 BOOST_REGEX_MAX_BLOCKS 告诉boost.regex允许使用的大小为BOOST_REGEX_BLOCKSIZE的栈的数量。如果这个值被超出,boost.regex会停止查找匹配,并抛出std::runtime_error。默认值为1024,如果你要改变BOOST_REGEX_BLOCKSIZE的值很多,别忘记考虑这个值。 BOOST_REGEX_MAX_CACHE_BLOCKS 告诉boost.regex内部缓存中允许使用的大小为BOOST_REGEX_BLOCKSIZE的栈的数量 —— 内存块会尽可能从这个缓存中分配而不是用new。一般而言,当你要分配内存块的时候,这会比new操作快上几个数量级, 不过当boost.regex用完一大块内存时,速度可能会下降(默认情况下,每个BOOST_REGEX_BLOCKSIZE大小的内存最多16个块。)。 如果内存很紧张,试着把它定义为0(禁用缓存),可如果你觉得太慢,改成1或2,可能会有效果.另一方面,在多处理器、多线程系统中,你会发现最好设置一个更高的值。 Installation 当你从压缩包中解压这个库的时候,请务必保证它的内部目录结构(比如使用-d参数解压)。如果你不这么做,你最好马上把眼睛离开文档,删掉已解压的文件,重来! This library should not need configuring before use; most popular compilers/standard libraries/platforms are already supported "as is". If you do experience configuration problems, or just want to test the configuration with your compiler, then the process is the same as for all of boost; see the configuration library documentation. The library will encase all code inside namespace boost. Unlike some other template libraries, this library consists of a mixture of template code (in the headers) and static code and data (in cpp files). Consequently it is necessary to build the library/s support code into a library or archive file before you can use it, instructions for specific platforms are as follows: Borland C++ Builder Open up a console window and change to the libs egexuild directory. * Select the appropriate makefile (bcb4.mak for C++ Builder 4, bcb5.mak for C++ Builder 5, and bcb6.mak for C++ Builder 6). * Invoke the makefile (pass the full path to your version of make if you have more than one version installed, the makefile relies on the path to make to obtain your C++ Builder installation directory and tools) for example: make -fbcb5.mak The build process will build a variety of .lib and .dll files (the exact number depends upon the version of Borland/s tools you are using) the .lib and dll files will be in a sub-directory called bcb4 or bcb5 depending upon the makefile used. To install the libraries into your development system use: make -fbcb5.mak install library files will be copied to /lib and the dll/s to /bin, where corresponds to the install path of your Borland C++ tools. You may also remove temporary files created during the build process (excluding lib and dll files) by using: make -fbcb5.mak clean Finally when you use regex++ it is only necessary for you to add the root director to your list of include directories for that project. It is not necessary for you to manually add a .lib file to the project; the headers will automatically select the correct .lib file for your build mode and tell the linker to include it. There is one caveat however: the library can not tell the difference between VCL and non-VCL enabled builds when building a GUI application from the command line, if you build from the command line with the 5.5 command line tools then you must define the pre-processor symbol _NO_VCL in order to ensure that the correct link libraries are selected: the C++ Builder IDE normally sets this automatically. Hint, users of the 5.5 command line tools may want to add a -D_NO_VCL to bcc32.cfg in order to set this option permanently. If you would prefer to do a dynamic link to the regex libraries when using the dll runtime then define BOOST_REGEX_DYN_LINK (you must do this if you want to use boost.regex in multiple dll/s), otherwise Boost.regex will be staically linked by default. If you want to suppress automatic linking altogether (and supply your own custom build of the lib) then define BOOST_REGEX_NO_LIB. If you are building with C++ Builder 6, you will find that can not be used in a pre-compiled header (the actual problem is in which gets included by ), if this causes problems for you, then try defining BOOST_NO_STD_LOCALE when building, this will disable some features throughout boost, but may save you a lot in compile times! Microsoft Visual C++ You need version 6 of MSVC to build this library. If you are using VC5 then you may want to look at one of the previous releases of this library Open up a command prompt, which has the necessary MSVC environment variables defined (for example by using the batch file Vcvars32.bat installed by the Visual Studio installation), and change to the libs egexuild directory. Select the correct makefile - vc6.mak for "vanilla" Visual C++ 6 or vc6-stlport.mak if you are using STLPort. Invoke the makefile like this: nmake -fvc6.mak You will now have a collection of lib and dll files in a "vc6" subdirectory, to install these into your development system use: nmake -fvc6.mak installThe lib files will be copied to your lib directory and the dll files to in, where is the root of your Visual C++ 6 installation. You can delete all the temporary files created during the build (excluding lib and dll files) using: nmake -fvc6.mak clean Finally when you use regex++ it is only necessary for you to add the root directory to your list of include directories for that project. It is not necessary for you to manually add a .lib file to the project; the headers will automatically select the correct .lib file for your build mode and tell the linker to include it. Note that if you want to dynamically link to the regex library when using the dynamic C++ runtime, define BOOST_REGEX_DYN_LINK when building your project. If you want to add the source directly to your project then define BOOST_REGEX_NO_LIB to disable automatic library selection. There are several important caveats to remember when using boost.regex with Microsoft/s Compiler: * There have been some reports of compiler-optimization bugs affecting this library, (particularly with VC6 versions prior to service patch 5) the workaround is to build the library using /Oityb1 rather than /O2. That is to use all optimization settings except /Oa. This problem is reported to affect some standard library code as well (in fact I/m not sure if the problem is with the regex code or the underlying standard library), so it/s probably worthwhile applying this workaround in normal practice in any case. * If you have replaced the C++ standard library that comes with VC6, then when you build the library you must ensure that the environment variables "INCLUDE" and "LIB" have been updated to reflect the include and library paths for the new library - see vcvars32.bat (part of your Visual Studio installation) for more details. * If you are building with the full STLPort v4.x, then use the vc6-stlport.mak file provided and set the environment variable STLPORT_PATH to point to the location of your STLPort installation (Note that the full STLPort libraries appear not to support single-thread static builds). * If you are building your application with /Zc:wchar_t then you will need to modify the makefile to add /Zc:wchar_t before building the library. GNU G++(2.95 and 3.x) You can build with gcc using the normal boost Jamfile in /libs/regex/build, alternatively there is a conservative makefile for the g++ compiler. From the command prompt change to the /libs/regex/build directory and type: make -fgcc.mak At the end of the build process you should have a gcc sub-directory containing release and debug versions of the library (libboost_regex.a and libboost_regex_debug.a). When you build projects that use regex++, you will need to add the boost install directory to your list of include paths and add /libs/regex/build/gcc/libboost_regex.a to your list of library files. There is also a makefile to build the library as a shared library: make -fgcc-shared.makwhich will build libboost_regex.so and libboost_regex_debug.so. Both of the these makefiles support the following environment variables: CXXFLAGS: extra compiler options - note that this applies to both the debug and release builds. INCLUDES: additional include directories. LDFLAGS: additional linker options. LIBS: additional library files. For the more adventurous there is a configure script in /libs/config; see the config library documentation. Sun Forte Compiler 6.1 There is a makefile for the sun (6.1) compiler (C++ version 3.12). From the command prompt change to the /libs/regex/build directory and type: dmake -f sunpro.mak At the end of the build process you should have a sunpro sub-directory containing single and multithread versions of the library (libboost_regex.a, libboost_regex.so, libboost_regex_mt.a and libboost_regex_mt.so). When you build projects that use regex++, you will need to add the boost install directory to your list of include paths and add /libs/regex/build/sunpro/ to your library search path. Both of the these makefiles support the following environment variables: CXXFLAGS: extra compiler options - note that this applies to both the single and multithreaded builds. INCLUDES: additional include directories. LDFLAGS: additional linker options. LIBS: additional library files. LIBSUFFIX: a suffix to mangle the library name with (defaults to nothing). This makefile does not set any architecture specific options like -xarch=v9, you can set these by defining the appropriate macros, for example: dmake CXXFLAGS="-xarch=v9" LDFLAGS="-xarch=v9" LIBSUFFIX="_v9" -f sunpro.makwill build v9 variants of the regex library named libboost_regex_v9.a etc. Other compilers (building with bjam) There is a generic makefile (generic.mak ) provided in /libs/regex/build - see that makefile for details of environment variables that need to be set before use. Alternatively you can using the Jam based build system: cd into /libs/regex/build and run: bjam -sTOOLS=mytoolsetIf you need to configure the library for your platform, then refer to the config library documentation . Reference Types syntax_option_type 目录 纲要 描述 用于perl正则表达式的选项 用于POSIX扩展正则表达式的选项 用于POSIX基本正则表达式的选项 用于字符串本义的选项 纲要 syntax_option_type类型是具体掩码类型的执行体,用于控制解释正则表达式字符串的方式。为了方便注解,这里列出的所有常量都在basic_regex类里被重复定义一次。 namespace std{ namespace regex_constants{ typedef implementation-specific-bitmask-type syntax_option_type; // these flags are standardized: static const syntax_option_type normal; static const syntax_option_type icase; static const syntax_option_type nosubs; static const syntax_option_type optimize; static const syntax_option_type collate; static const syntax_option_type ECMAScript = normal; static const syntax_option_type JavaScript = normal; static const syntax_option_type JScript = normal; static const syntax_option_type basic; static const syntax_option_type extended; static const syntax_option_type awk; static const syntax_option_type grep; static const syntax_option_type egrep; static const syntax_option_type sed = basic; static const syntax_option_type perl; // these are boost.regex specific: static const syntax_option_type escape_in_lists; static const syntax_option_type char_classes; static const syntax_option_type intervals; static const syntax_option_type limited_ops; static const syntax_option_type newline_alt; static const syntax_option_type bk_plus_qm; static const syntax_option_type bk_braces; static const syntax_option_type bk_parens; static const syntax_option_type bk_refs; static const syntax_option_type bk_vbar; static const syntax_option_type use_except; static const syntax_option_type failbit; static const syntax_option_type literal; static const syntax_option_type nocollate; static const syntax_option_type perlex; static const syntax_option_type emacs; } // namespace regex_constants } // namespace std描述 设定这些元素会得到下表中所列的效果,一个有效的syntax_option_type类型的值总是确定的是这些值之一:normal, basic, extended, awk, grep, egrep, sed 或 perl set. 因为他们被重复定义了(见上),因此你用下列任何一种写法都是一样的: boost::regex_constants::constant_nameor boost::regex::constant_nameor boost::wregex::constant_name用于perl正则表达式的选项 下列选项只用于perl正则表达式: Element Standardized Effect if set ECMAScript Yes 指定用常规的语法识别正则表达式:这和ECMA-262是等效的——ECMAScript Language Specification, Chapter 15 part 10, RegExp(Regular Expression) Objects (FWD.1)。此模式下boost.regex会识别大多数perl兼容的扩展语法。 perl No 同上 normal No 同上 JScript No 同上 JavaScript No 同上 也可使用下列选项: Element Standardized Effect if set icase Yes 忽略大小写 nosubs Yes 不匹配子表达式 optimize Yes 更多地考虑匹配速度,而不是构造表达式的速度。不这样的话,你就无法在程序输出中检测效果。不过现在这个参数已经无效了。 collate Yes 指定字符范围是区域敏感的。 newline-alt No 指定‘ ’与间隔符‘|’等效。允许换行符间隔列表被用作间隔列表。 no_mod_m No 通常,从Boost.Regex的行为来看,perl m_modifier是打开的:断言^和$分别匹配行首和行尾,这个标志位和表达式前缀(?-m)是等效的。 no_mod_s No Boost.Regex是否匹配"."为一个换行符取决于match_dot_not_newline的设定。这个标志位与(?-s)等效,使无论match_not_dot_newline是否设定于match flag,"."都不匹配为换行符。 mod_s No 按义,与上相反 mod_x No 打开perl x-modifier: 使忽略表达式中的非转义空格。 用于POSIX扩展正则表达式的选项 下列选项只使用于POSIX扩展正则表达式: Element Standardized Effect if set extended Yes 指定使用POSIX扩展正则表达式in IEEE Std 1003.1-2001——Portable Operating System Interface (POSIX ), Base Definitions and Headers, Section 9, Regular Expressions (FWD.1). 另外,也支持一些perl形式的转义序列(POSIX 标准规定只有特定的字符可以被转义,所有其他的转义序列都可能导致未定义的行为。 egrep Yes 指定使用与POSIX 程序 egrep -E 相同的正则表达式 in IEEE Std 1003.1-2001, Portable Operating System Interface (POSIX ), Shells and Utilities, Section 4, Utilities, grep (FWD.1).更确切的说,与POSIX扩展句法是相同的,不过换行符会被作为间隔符。 也即,等同于POSIX扩展句法,不过除了‘|’,换行符会作为间隔符使用 awk Yes 指定使用与POSIX 程序 awk相同的正则表达式 in IEEE Std 1003.1-2001, Portable Operating System Interface (POSIX ), Shells and Utilities, Section 4, awk (FWD.1)。 这与 extended选项是一致的,但是会忽略转义符号。 也即,等同于POSIX扩展句法,但是允许字符类中含有转义序列。 此外,也支持一些perl形式的转义序列 (实际上awk句法只需要识别a v f and ,其他的perl形式的转义序列根据POSIX的规定会引发未定义的行为,但实际上Boost.Regex仍然可以识别)。 也可使用下列选项: Element Standardized Effect if set icase Yes 见上 nosubs Yes 见上 optimize Yes 见上 collate Yes 指定字符范围是区域敏感的。*这个选项在perl扩展正则表达式上是缺省的*,but can be unset to force ranges to be compared by code point only. newline_alt No 见上 no_escape_in_lists No 使列表内的转义字符成为普通字符,因之[]将被匹配为 //或是/b/。对于POSIX扩展,这个位缺省是打开的(原文此恐有误,似应为This bit is on by default),但是可以取消,使转义字符可以被重新识别。 no_bk_refs No 向后引用被禁止。对于POSIX扩展,这个位是缺省打开的,你可以取消从而启用向后引用。 用于POSIX基本正则表达式的选项 下列选项只使用于POSIX扩展正则表达式: Element Standardized Effect if set basic Yes 指定使用POSIX基本正则表达式in IEEE Std 1003.1-2001——Portable Operating System Interface (POSIX ), 基本定义和头文件见Section 9, Regular Expressions (FWD.1). sed No 同上 grep Yes 指定使用POSIX 程序 grep 相同的正则表达式 in IEEE Std 1003.1-2001, Portable Operating System Interface (POSIX ), Shells and Utilities, Section 4, Utilities, grep (FWD.1)。与POSIX基本句法是相同的,不过换行符会被作为间隔符 emacs No 指定识别语法为用于emacs的POSIX基本句法的超集。 也可使用下列选项: Element Standardized Effect if set icase Yes 见上 nosubs Yes 见上 optimize Yes 见上 collate Yes 见上 newline_alt No 见上 no_char_class No 不允许字符类别,如[[:alnum:]]。 no_escape_in_lists No 见上 no_interval No 不允许有限的重复,如a{2,3}。 bk_plus_qm No "+" 表示1到更多次,"?" 表示至少0次,反之它们会被"+"和"?"替代。 bk_vbar No "|"表示间隔符,"|" 成为其本义。 用于字符串本义的选项 下列选项用于将表达式解释为普通的字符串: Element Standardized Effect if set literal Yes 以本义处理字符串(无特殊字符) 下列选项可与literal结合使用 Element Standardized Effect if set icase Yes 见上 optimize Yes 见上 error_type 纲要 error_type表示各种在解析正则表达式的时候由库引发的错误类型。 namespace boost{ namespace regex_constants{ typedef implementation-specific-type error_type; static const error_type error_collate; static const error_type error_ctype; static const error_type error_escape; static const error_type error_backref; static const error_type error_brack; static const error_type error_paren; static const error_type error_brace; static const error_type error_badbrace; static const error_type error_range; static const error_type error_space; static const error_type error_badrepeat; static const error_type error_complexity; static const error_type error_stack; static const error_type error_bad_pattern; } // namespace regex_constants } // namespace boost描述 error_type类型是一个特定执行体的枚举,可以采用以下的值: 常量 含义 error_collate [[.name.]]块中指定了无效的比较元素. error_ctype [[:name:]]块中指定了无效的字符类名称。 error_escape An invalid or trailing escape was encountered. error_backref 对不存在的子表达式的向后引用。 error_brack 无效的字符集 [...]。 error_paren 不匹配/(/ 和 /)/. error_brace 不匹配 /{/ 和 /}/. error_badbrace {...}块中有无效内容。 error_range 字符范围无效,比如[d-a]。 error_space 内存溢出。 error_badrepeat 试图重复某些不能重复的元素——比如 a*+ error_complexity 表达式过于复杂,无法处理。 error_stack 程序栈空间溢出。 error_bad_pattern 其他未定义的错误。 match_flag_type 纲要 match_flag_type类型是具体掩码类型的执行体(17.3.2.1.2),用来控制正则表达式如何匹配一个字符序列。关于format flag行为更多细节在格式句法指南中描述。 namespace boost{ namespace regex_constants{ typedef implementation-specific-bitmask-type match_flag_type; static const match_flag_type match_default = 0; static const match_flag_type match_not_bob; static const match_flag_type match_not_eob; static const match_flag_type match_not_bol; static const match_flag_type match_not_eol; static const match_flag_type match_not_bow; static const match_flag_type match_not_eow; static const match_flag_type match_any; static const match_flag_type match_not_null; static const match_flag_type match_continuous; static const match_flag_type match_partial; static const match_flag_type match_single_line; static const match_flag_type match_prev_avail; static const match_flag_type match_not_dot_newline; static const match_flag_type match_not_dot_null; static const match_flag_type format_default = 0; static const match_flag_type format_sed; static const match_flag_type format_perl; static const match_flag_type format_no_copy; static const match_flag_type format_first_only; static const match_flag_type format_all; } // namespace regex_constants } // namespace boost 描述 match_flag_type类型是具体掩码类型的执行体(17.3.2.1.2). 在匹配一个范围为[first, last)的字符序列时,对于不同的flag,其效果如下图所示: 元素 效果 match_default 匹配表达式十部根据以下标准规则做任何修正:ECMA-262, ECMAScript Language Specification, Chapter 15 part 10, RegExp? (Regular Expression) Objects (FWD.1) match_not_bob "A"不匹配子序列[first,first) match_not_eob "z"和""不匹配子序列[last,last) match_not_bol "^"不匹配子序列[first,first) match_not_eob "$"不匹配子序列[last,last) match_not_bow ""不匹配子序列[first,first) match_not_wow ""不匹配子序列[last,last) match_any 如果有一个以上的可能匹配,则任何匹配都可以接受 match_not_null 不匹配空序列 match_continuous 表达式必须匹配起始于first的子序列 match_partial 如果没有找到匹配, match_extra match_single_line match_prev_avail match_not_dot_newline 表达式"."不匹配新行 match_not_dot_null 表达式"."不匹配// format_default format_sed format_perl format_all format_no_copy format_first_copy 指定搜索和替换操作的时候,只有正则表达式第一次出现的时候被替换 class regex_error *纲要* #include <boost/pattern_except.hpp> 类regex_error定义了当一个表示正则表达式的字符串被转换为有限状态机出现错误时,抛出异常的对象类型。 namespace boost{ class bad_pattern : public std::runtime_error { public: explicit bad_pattern(const std::string& s) : std::runtime_error(s){}; }; class bad_expression : public bad_pattern { public: bad_expression(const std::string& s) : bad_pattern(s) {} }; } // namespace boost *描述* regex_error(const std::string& s, regex_constants::error_type err, std::ptrdiff_t pos); regex_error(boost::regex_constants::error_type err); *效果*: 构造一个regex_error对象。 boost::regex_constants::error_type code()const; *效果*: 返回所产生的解析错误错误码。 std::ptrdiff_t position()const; *效果*: 返回解析终止的位置。 脚注: 选择std::runtime_error作为bad_pattern的基类是有争议的;依赖于库如何使用,异常可能是逻辑错误 (程序员写表达式时)或运行时错误(用户使用表达式时).单一的类regex_error取代了之前的bad_pattern 和 bad_expression,以保持和标准化提案的同步。 class regex_traits *纲要* namespace boost{ template <class charT, class implementationT = sensible_default_choice> struct regex_traits : public implementationT { regex_traits() : implementationT() {} }; template <class charT> struct c_regex_traits; template <class charT> struct cpp_regex_traits; template <class charT> struct w32_regex_traits; } // namespace boost描述 类regex_traits是以下之一的实际执行类的瘦包装。 · c_regex_traits: 这个类已经废弃,它封装了c本地化,在非Win32平台上缺省使用,这使得c++本地化将不可用。 · cpp_regex_traits: 非Win32平台上缺省使用,允许regex类包含一个 std::locale实例 · w32_regex_traits: Win32平台上缺省使用,允许regex类被包含一个LCID(地区代码)。 class template basic_regex 纲要 #include <boost/regex.hpp> 模板类basic_regex封装了正则表达式的解析和编译工作,它有三个模板参数: charT: 定义字符类型, 例如 char 或是 wchar_t。 traits: 定义字符类型的行为, for example which character class names are recognized. 默认的traits是: regex_traits。 Allocator: 内存分配器。 基于易用性考虑,这两个typedefs定义了两个标准的basic_regex实例,除非你需要自己的traits 和 allocators,否则你不需要再做任何事: namespace boost{ template <class charT, class traits = regex_traits<charT>, class Allocator = std::allocator<charT> > class basic_regex; typedef basic_regex<char> regex; typedef basic_regex<wchar_t> wregex; } basic_regex的定义如下: 它非常接近于basic_string,满足作为一个value_type为chatT的常量容器的必要条件。 namespace boost{ template <class charT, class traits = regex_traits<charT>, class Allocator = allocator<charT> > class basic_regex { public: // types: typedef charT value_type; typedef implementation defined const_iterator; typedef const_iterator iterator; typedef typename Allocator::reference reference; typedef typename Allocator::const_reference const_reference; typedef typename Allocator::difference_type difference_type; typedef typename Allocator::size_type size_type; typedef Allocator allocator_type; typedef regex_constants::syntax_option_type flag_type; typedef typename traits::locale_type locale_type; // constants: static const regex_constants::syntax_option_type normal = regex_constants::normal; static const regex_constants::syntax_option_type icase = regex_constants::icase; static const regex_constants::syntax_option_type nosubs = regex_constants::nosubs; static const regex_constants::syntax_option_type optimize = regex_constants::optimize; static const regex_constants::syntax_option_type collate = regex_constants::collate; static const regex_constants::syntax_option_type ECMAScript = normal; static const regex_constants::syntax_option_type JavaScript = normal; static const regex_constants::syntax_option_type JScript = normal; // these flags are optional, if the functionality is supported // then the flags shall take these names. static const regex_constants::syntax_option_type basic = regex_constants::basic; static const regex_constants::syntax_option_type extended = regex_constants::extended; static const regex_constants::syntax_option_type awk = regex_constants::awk; static const regex_constants::syntax_option_type grep = regex_constants::grep; static const regex_constants::syntax_option_type egrep = regex_constants::egrep; static const regex_constants::syntax_option_type sed = basic = regex_constants::sed; static const regex_constants::syntax_option_type perl = regex_constants::perl; // construct/copy/destroy: explicit basic_regex(const Allocator& a = Allocator()); explicit basic_regex(const charT* p, flag_type f = regex_constants::normal, const Allocator& a = Allocator()); basic_regex(const charT* p1, const charT* p2, flag_type f = regex_constants::normal, const Allocator& a = Allocator()); basic_regex(const charT* p, size_type len, flag_type f, const Allocator& a = Allocator()); basic_regex(const basic_regex&); template <class ST, class SA> explicit basic_regex(const basic_string<charT, ST, SA>& p, flag_type f = regex_constants::normal, const Allocator& a = Allocator()); template <class InputIterator> basic_regex(InputIterator first, inputIterator last, flag_type f = regex_constants::normal, const Allocator& a = Allocator()); ~basic_regex(); basic_regex& operator=(const basic_regex&); basic_regex& operator= (const charT* ptr); template <class ST, class SA> basic_regex& operator= (const basic_string<charT, ST, SA>& p); // iterators: const_iterator begin() const; const_iterator end() const; // capacity: size_type size() const; size_type max_size() const; bool empty() const; unsigned mark_count()const; // // modifiers: basic_regex& assign(const basic_regex& that); basic_regex& assign(const charT* ptr, flag_type f = regex_constants::normal); basic_regex& assign(const charT* ptr, unsigned int len, flag_type f); template <class string_traits, class A> basic_regex& assign(const basic_string<charT, string_traits, A>& s, flag_type f = regex_constants::normal); template <class InputIterator> basic_regex& assign(InputIterator first, InputIterator last, flag_type f = regex_constants::normal); // const operations: Allocator get_allocator() const; flag_type flags() const; basic_string<charT> str() const; int compare(basic_regex&) const; // locale: locale_type imbue(locale_type loc); locale_type getloc() const; // swap void swap(basic_regex&) throw(); }; template <class charT, class traits, class Allocator> bool operator == (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs); template <class charT, class traits, class Allocator> bool operator != (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs); template <class charT, class traits, class Allocator> bool operator < (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs); template <class charT, class traits, class Allocator> bool operator <= (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs); template <class charT, class traits, class Allocator> bool operator >= (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs); template <class charT, class traits, class Allocator> bool operator > (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs); template <class charT, class io_traits, class re_traits, class Allocator> basic_ostream<charT, io_traits>& operator << (basic_ostream<charT, io_traits>& os, const basic_regex<charT, re_traits, Allocator>& e); template <class charT, class traits, class Allocator> void swap(basic_regex<charT, traits, Allocator>& e1, basic_regex<charT, traits, Allocator>& e2); typedef basic_regex<char> regex; typedef basic_regex<wchar_t> wregex; } // namespace boost 描述: 类basic_regex有以下公有成员函数: basic_regex constants static const regex_constants::syntax_option_type normal = regex_constants::normal; static const regex_constants::syntax_option_type icase = regex_constants::icase; static const regex_constants::syntax_option_type nosubs = regex_constants::nosubs; static const regex_constants::syntax_option_type optimize = regex_constants::optimize; static const regex_constants::syntax_option_type collate = regex_constants::collate; static const regex_constants::syntax_option_type ECMAScript = normal; static const regex_constants::syntax_option_type JavaScript = normal; static const regex_constants::syntax_option_type JScript = normal; static const regex_constants::syntax_option_type basic = regex_constants::basic; static const regex_constants::syntax_option_type extended = regex_constants::extended; static const regex_constants::syntax_option_type awk = regex_constants::awk; static const regex_constants::syntax_option_type grep = regex_constants::grep; static const regex_constants::syntax_option_type egrep = regex_constants::egrep; static const regex_constants::syntax_option_type sed = basic = regex_constants::sed; static const regex_constants::syntax_option_type perl = regex_constants::perl; 这些常量成员作为那些被声明在namespace boost::regex_constants中的常量的替代; 它们在各自的范围内具有相同的名字、型别和值。 basic_regex constructors 在所有的basic_regex构造函数中, 构造函数和成员函数的任何内存的分配都在这个对象的生命期内使用一份拷贝。 basic_regex(const Allocator& a = Allocator());效果: 构造一个basic_regex类。它的另一些影响如下表: Element value empty() true size() 0 str() basic_string() basic_regex(const charT* p, flag_type f = regex_constants::normal, const Allocator& a = Allocator());前提: p 不是空指针。 异常: 如果p指向一个无效的表达式,则抛出bad_expression。 效果: 构造一个basic_regex对象; 这个对象内部的有限状态机被包含在c字符串所包含的正则表达式构造,并且根据f中的option标志进行解释。下表是另外一些影响: Element Value empty() false size() std::distance(p1,p2) str() basic_string(p1,p2) flag() f make_count() The number of marked sub-expressions within the expression. basic_regex(const charT* p, size_type len, flag_type f, const Allocator& a = Allocator());前提: p不是空指针, len < max_size(). 异常: 如果p指向一个无效的表达式,则抛出bad_expression。 效果: 构造一个basic_regex对象; 这个对象内部的有限状态机被包含在[p, p+len)的字符序列所包含的正则表达式构造,并且根据f中的option标志进行解释。下表是另外一些影响: Element Value empty() false size() len str() basic_string(p1,p2) flag() f make_count() The number of marked sub-expressions within the expression. basic_regex(const basic_regex& e);效果: 构造一个拷贝自对象basic_regex e的basic_regex对象。下表是另外一些影响: Element Value empty() e.empty() size() e.size() str() e.str() flag() e.flag() make_count() e.make_count() template <class ST, class SA> basic_regex(const basic_string<charT, ST, SA>& s, flag_type f = regex_constants::normal, const Allocator& a = Allocator());异常: 如果s是一个无效的表达式,则抛出bad_expression。 效果: 构造一个basic_regex对象; 这个对象内部的有限状态机被字符串s所包含的正则表达式构造,并且根据f中的option标志进行解释。下表是另外一些影响: Element Value empty() false size() s.size() str() s flag() f make_count() The number of marked sub-expressions within the expression. template <class ForwardIterator> basic_regex(ForwardIterator first, ForwardIterator last, flag_type f = regex_constants::normal, const Allocator& a = Allocator());异常: 如果序列[first, last)不是一个有效的正则表达式,则抛出bad_expression。 效果: 构造一个basic_regex对象; 这个对象内部的有限状态机被包含在[first,last)的字符序列所包含的正则表达式构造,并且根据f中的option标志进行解释。下表是另外一些影响: Element Value empty() false size() distance(first,last)) str() basic_string(first,last) flag() f make_count() The number of marked sub-expressions within the expression. basic_regex& operator=(const basic_regex& e);效果: 返回assign(e.str(), e.flags())的结果。 basic_regex& operator=(const charT* ptr);前提: p不是一个空指针. 效果: 返回assign(ptr)的结果 template <class ST, class SA> basic_regex& operator=(const basic_string<charT, ST, SA>& p);效果: 返回assign(p)的结果。 basic_regex iterators const_iterator begin() const;效果: 返回一个指向表示正则表达式的字符序列的初始迭代器。 const_iterator end() const;效果: 返回一个指向表示正则表达式的字符序列的终止迭代器。 basic_regex capacity size_type size() const;效果: 返回表示正则表达式的字符序列的长度。 size_type max_size() const;效果: 返回表示正则表达式的字符序列的最大长度。 bool empty() const;效果: 如果对象未包含一个有效的正则表达式则返回true,反之,返回false。 unsigned mark_count() const;效果: 返回标记的子表达式的数量。 basic_regex assign basic_regex& assign(const basic_regex& that);效果: 返回assign(that.str(), that.flags()). basic_regex& assign(const charT* ptr, flag_type f = regex_constants::normal);效果: 返回assign(string_type(ptr), f). basic_regex& assign(const charT* ptr, unsigned int len, flag_type f);效果: 返回assign(string_type(ptr, len), f). template <class string_traits, class A> basic_regex& assign(const basic_string<charT, string_traits, A>& s, flag_type f = regex_constants::normal);异常: 如果s不是一个有效的正则表达式,抛出bad_expression。 返回值: this. 效果: 指定字符串s中的正则表达式,根据f中的option标志进行解释。表中是另外一些效果: Element Value empty() false size() s.size() str() s flag() f make_count() The number of marked sub-expressions within the expression. template <class InputIterator> basic_regex& assign(InputIterator first, InputIterator last, flag_type f = regex_constants::normal);前提: InputIterator?的型别符合Input Iterator的要求。(24.1.1). 效果: 返回assign(string_type(first, last), f). basic_regex constant operations Allocator get_allocator() const;效果: 返回一个被传递给构造函数的内存分配器的拷贝。 flag_type flags() const;效果: 返回被传递给构造函数或是最后调用assign的正则表达式句法标志的拷贝。 basic_string<charT> str() const;效果: 返回被传递给构造函数或是最后调用assign的字符序列的拷贝。 int compare(basic_regex& e)const;效果: If flags() == e.flags() then returns str().compare(e.str()), otherwise returns flags() - e.flags(). basic_regex locale locale_type imbue(locale_type l);效果: 返回traits_inst.imbue(l)的结果,traits_inst是一个存储在对象中的模板参数traits的实例(被缺省初始化)。 调用imbue会使当前包含的任何正则表达式无效。 影响: empty() == true. locale_type getloc() const;效果: 返回traits_inst.getloc(),traits_inst是一个存储在对象中的模板参数traits的实例(被缺省初始化)。 basic_regex swap void swap(basic_regex& e) throw();效果: 交换两个正则表达式的内容。 影响: *this包含了e中的字符,e包含了*this中的正则表达式。 复杂度: 常数时间 *basic_regex non-member functions* *basic_regex non-member comparison operators * basic_regex对象间的比较是实验性的;请注意这些很可能从标准库提案中被移除,因此如果你希望代码具有可移植性,那么要小心这点。 template <class charT, class traits, class Allocator> bool operator == (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs);效果: 返回 lhs.compare(rhs) == 0. template <class charT, class traits, class Allocator> bool operator != (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs);效果: 返回 lhs.compare(rhs) = 0. template <class charT, class traits, class Allocator> bool operator < (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs);效果: 返回 lhs.compare(rhs) < 0. template <class charT, class traits, class Allocator> bool operator <= (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs);效果: 返回 lhs.compare(rhs) <= 0. template <class charT, class traits, class Allocator> bool operator >= (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs);效果: 返回 lhs.compare(rhs) >= 0. template <class charT, class traits, class Allocator> bool operator > (const basic_regex<charT, traits, Allocator>& lhs, const basic_regex<charT, traits, Allocator>& rhs);效果: 返回 lhs.compare(rhs) > 0. basic_regex inserter basic_regex流插入器是实验性的, 它向流输出表达式的文本: template <class charT, class io_traits, class re_traits, class Allocator> basic_ostream<charT, io_traits>& operator << (basic_ostream<charT, io_traits>& os const basic_regex<charT, re_traits, Allocator>& e);效果: 返回 (os << e.str()). basic_regex non-member swap template <class charT, class traits, class Allocator> void swap(basic_regex<charT, traits, Allocator>& lhs, basic_regex<charT, traits, Allocator>& rhs);效果: 调用 lhs.swap(rhs). class template sub_match 纲要 #include <boost/regex.hpp> 正则表达式不同于许多简单的模式匹配算法,因为它们同时匹配整体和子表达式:每一个子表达式使用圆括号分隔。有一些函数可以将子匹配告知用户: 你可以定义一个match_results对象来操作子匹配的集合索引,每一个子匹配都可以放在一个sub_match对象中。 sub_match类型的对象只能通过match_results对象的下标获取,并且可以与 std::basic_string,const charT* 或者 const charT 比较,或是添加到其中,生成一个新的std::basic_string 对象。 当由sub_match<>表示的标记子匹配参与了正则表达式匹配时,matched为true,first和second表示构成匹配的范围[first,second)。否则matched为false, first和second仍然是缺省的未定义值。 当由sub_match<>表示的标记子匹配被重复时,sub_match对象代表最后一次重复获得的匹配。完整的捕获集可以通过captures()成员函数访问(注:这里有隐含有严重的效能损失,所以你必须显式地启用这个特征)。 如果一个sub_match<>对象表示子匹配0——那意味着整个匹配——这样匹配总是为true,除非match_partial标志导致局部匹配被传递给正则表达式算法,这种情况下匹配可以为false,first和second表示构成局部匹配的字符范围。 namespace boost{ template <class BidirectionalIterator> class sub_match : public std::pair<BidirectionalIterator, BidirectionalIterator> { public: typedef typename iterator_traits<BidirectionalIterator>::value_type value_type; typedef typename iterator_traits<BidirectionalIterator>::difference_type difference_type; typedef BidirectionalIterator iterator; bool matched; difference_type length()const; operator basic_string<value_type>()const; basic_string<value_type> str()const; int compare(const sub_match& s)const; int compare(const basic_string<value_type>& s)const; int compare(const value_type* s)const; #ifdef BOOST_REGEX_MATCH_EXTRA typedef implementation-private capture_sequence_type; const capture_sequence_type& captures()const; #endif }; // // comparisons to another sub_match: // template <class BidirectionalIterator> bool operator == (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator != (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator < (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator > (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs); // // comparisons to a basic_string: // template <class BidirectionalIterator, class traits, class Allocator> bool operator == (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator != (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator < (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator > (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator >= (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator <= (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator == (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator != (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator < (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator > (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs); template <class BidirectionalIterator, class traits, class Allocator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs); // // comparisons to a pointer to a character array: // template <class BidirectionalIterator> bool operator == (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator != (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator < (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator > (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator >= (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator <= (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator == (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); template <class BidirectionalIterator> bool operator != (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); template <class BidirectionalIterator> bool operator < (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); template <class BidirectionalIterator> bool operator > (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); template <class BidirectionalIterator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); template <class BidirectionalIterator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); // // comparisons to a single character: // template <class BidirectionalIterator> bool operator == (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator != (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator < (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator > (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator >= (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator <= (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); template <class BidirectionalIterator> bool operator == (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); template <class BidirectionalIterator> bool operator != (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); template <class BidirectionalIterator> bool operator < (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); template <class BidirectionalIterator> bool operator > (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); template <class BidirectionalIterator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); template <class BidirectionalIterator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); // // addition operators: // template <class BidirectionalIterator, class traits, class Allocator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator> operator + (const std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& s, const sub_match<BidirectionalIterator>& m); template <class BidirectionalIterator, class traits, class Allocator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator> operator + (const sub_match<BidirectionalIterator>& m, const std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& s); template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (typename iterator_traits<BidirectionalIterator>::value_type const* s, const sub_match<BidirectionalIterator>& m); template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (const sub_match<BidirectionalIterator>& m, typename iterator_traits<BidirectionalIterator>::value_type const * s); template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (typename iterator_traits<BidirectionalIterator>::value_type const& s, const sub_match<BidirectionalIterator>& m); template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (const sub_match<BidirectionalIterator>& m, typename iterator_traits<BidirectionalIterator>::value_type const& s); template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (const sub_match<BidirectionalIterator>& m1, const sub_match<BidirectionalIterator>& m2); // // stream inserter: // template <class charT, class traits, class BidirectionalIterator> basic_ostream<charT, traits>& operator << (basic_ostream<charT, traits>& os, const sub_match<BidirectionalIterator>& m); } // namespace boost 描述 sub_match成员 typedef typename std::iterator_traits<iterator>::value_type value_type; 迭代器所指向的型别。 typedef typename std::iterator_traits<iterator>::difference_type difference_type; 表示两个迭代器距离的型别。 typedef iterator iterator_type; 迭代器的型别。 iterator first 指示匹配的起始位置的迭代器。 iterator second 指示匹配的结束位置的迭代器。 bool matched 指示在这个匹配中子表达式是否参予匹配的bool值。 static difference_type length();效果: 返回北匹配的字表达式的长度,0表示子表达式未被匹配。 operator basic_string<value_type>()const;效果: 转换*this为一个字符串: returns (matched ? basic_string(first, second) : basic_string()). basic_string<value_type> str()const;效果: 返回一个表示*this的字符串: (matched ? basic_string(first, second) : basic_string()). int compare(const sub_match& s)const;效果: 执行对字符串s的字典比较: returns str().compare(s.str()). int compare(const basic_string<value_type>& s)const;效果: 比较*this和字符串s: 返回 str().compare(s). int compare(const value_type* s)const;效果: 比较*this和c字符串s: returns str().compare(s). typedef implementation-private capture_sequence_type; 定义满足标准库序列需求的特定实现的类型(21.1.1 包含在 the optional Table 68 operations), value_type 是 sub_match。This type happens to be std::vector >, but you shouldn/t actually rely on that. const capture_sequence_type& captures()const; 效果: 返回一个包含这个子匹配所有捕获的序列。 充分条件: 库必须在BOOST_REGEX_MATCH_EXTRA被定义的情况下构建和使用,你也必须按照函数定义的顺序传递match_extra标志给匹配函数(regex_match, regex_search, regex_iterator 或 regex_token_iterator)并返回有用的信息。 原理: 允许这个特征有如下后果: * sub_match会占用更多的内存,这有可能导致在匹配复杂的表达式时栈空间更快地溢出。 * 匹配算法在处理某些特性时效能降低(象是独立的子表达式),甚至当match_extra没有使用时也会如此。 * 如果使用了match_extra,匹配算法会更低效(更慢)。多数情况下这会导致更多的内存分配 sub_match非成员operators Comparisons against self template <class BidirectionalIterator> bool operator == (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs.compare(rhs) == 0. template <class BidirectionalIterator> bool operator != (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs.compare(rhs) = 0. template <class BidirectionalIterator> bool operator < (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs.compare(rhs) < 0. template <class BidirectionalIterator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs.compare(rhs) <= 0. template <class BidirectionalIterator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs.compare(rhs) >= 0. template <class BidirectionalIterator> bool operator > (const sub_match<BidirectionalIterator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs.compare(rhs) > 0. Comparisons with std::basic_string template <class BidirectionalIterator, class traits, class Allocator> bool operator == (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs == rhs.str(). template <class BidirectionalIterator, class traits, class Allocator> bool operator != (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs = rhs.str(). template <class BidirectionalIterator, class traits, class Allocator> bool operator < (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs < rhs.str(). template <class BidirectionalIterator, class traits, class Allocator> bool operator > (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs > rhs.str(). template <class BidirectionalIterator, class traits, class Allocator> bool operator >= (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs >= rhs.str(). template <class BidirectionalIterator, class traits, class Allocator> bool operator <= (const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& lhs, const sub_match<BidirectionalIterator>& rhs);效果: returns lhs <= rhs.str(). template <class BidirectionalIterator, class traits, class Allocator> bool operator == (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs);效果: returns lhs.str() == rhs. template <class BidirectionalIterator, class traits, class Allocator> bool operator != (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs);效果: returns lhs.str() = rhs. template <class BidirectionalIterator, class traits, class Allocator> bool operator < (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs);效果: returns lhs.str() < rhs. template <class BidirectionalIterator, class traits, class Allocator> bool operator > (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs);效果: returns lhs.str() > rhs. template <class BidirectionalIterator, class traits, class Allocator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs);效果: returns lhs.str() >= rhs. template <class BidirectionalIterator, class traits, class Allocator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, const std::basic_string<iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& rhs);效果: returns lhs.str() <= rhs. Comparisons with null-terminated strings template <class BidirectionalIterator> bool operator == (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs == rhs.str(). template <class BidirectionalIterator> bool operator != (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs = rhs.str(). template <class BidirectionalIterator> bool operator < (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs < rhs.str(). template <class BidirectionalIterator> bool operator > (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs > rhs.str(). template <class BidirectionalIterator> bool operator >= (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs >= rhs.str(). template <class BidirectionalIterator> bool operator <= (typename iterator_traits<BidirectionalIterator>::value_type const* lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs <= rhs.str(). template <class BidirectionalIterator> bool operator == (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); 效果: returns lhs.str() == rhs. template <class BidirectionalIterator> bool operator != (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); 效果: returns lhs.str() = rhs. template <class BidirectionalIterator> bool operator < (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); 效果: returns lhs.str() < rhs. template <class BidirectionalIterator> bool operator > (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); 效果: returns lhs.str() > rhs. template <class BidirectionalIterator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); 效果: returns lhs.str() >= rhs. template <class BidirectionalIterator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const* rhs); 效果: returns lhs.str() <= rhs. <br /> Comparisons with a single character template <class BidirectionalIterator> bool operator == (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs == rhs.str(). template <class BidirectionalIterator> bool operator != (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs = rhs.str(). template <class BidirectionalIterator> bool operator < (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs < rhs.str(). template <class BidirectionalIterator> bool operator > (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs > rhs.str(). template <class BidirectionalIterator> bool operator >= (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs >= rhs.str(). template <class BidirectionalIterator> bool operator <= (typename iterator_traits<BidirectionalIterator>::value_type const& lhs, const sub_match<BidirectionalIterator>& rhs); 效果: returns lhs <= rhs.str(). template <class BidirectionalIterator> bool operator == (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); 效果: returns lhs.str() == rhs. template <class BidirectionalIterator> bool operator != (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); 效果: returns lhs.str() = rhs. template <class BidirectionalIterator> bool operator < (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); 效果: returns lhs.str() < rhs. template <class BidirectionalIterator> bool operator > (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); 效果: returns lhs.str() > rhs. template <class BidirectionalIterator> bool operator >= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); 效果: returns lhs.str() >= rhs. template <class BidirectionalIterator> bool operator <= (const sub_match<BidirectionalIterator>& lhs, typename iterator_traits<BidirectionalIterator>::value_type const& rhs); 效果: returns lhs.str() <= rhs. 附加的操作符 sub_match附加的操作符允许你添加一个sub_match对象到任何类型(兼容std::string)中去,就象添加一个std::string并且获得一个新的string一样。 template <class BidirectionalIterator, class traits, class Allocator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator> operator + (const std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& s, const sub_match<BidirectionalIterator>& m); 效果: returns s + m.str(). template <class BidirectionalIterator, class traits, class Allocator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator> operator + (const sub_match<BidirectionalIterator>& m, const std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type, traits, Allocator>& s); 效果: returns m.str() + s. template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (typename iterator_traits<BidirectionalIterator>::value_type const* s, const sub_match<BidirectionalIterator>& m); 效果: returns s + m.str(). template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (const sub_match<BidirectionalIterator>& m, typename iterator_traits<BidirectionalIterator>::value_type const * s);效果: returns m.str() + s. template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (typename iterator_traits<BidirectionalIterator>::value_type const& s, const sub_match<BidirectionalIterator>& m); 效果: returns s + m.str(). template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (const sub_match<BidirectionalIterator>& m, typename iterator_traits<BidirectionalIterator>::value_type const& s); 效果: returns m.str() + s. template <class BidirectionalIterator> std::basic_string<typename iterator_traits<BidirectionalIterator>::value_type> operator + (const sub_match<BidirectionalIterator>& m1, const sub_match<BidirectionalIterator>& m2);效果: returns m1.str() + m2.str(). Stream inserter template <class charT, class traits, class BidirectionalIterator> basic_ostream<charT, traits>& operator << (basic_ostream<charT, traits>& os const sub_match<BidirectionalIterator>& m);效果: returns (os << m.str()). class template match_results Contents Synopsis Description 纲要 #include <boost/regex.hpp> 正则表达式不同于许多简单的模式匹配算法,因为它们同时匹配整体和子表达式:每一个子表达式使用圆括号分隔。有一些函数可以将子匹配告知用户: 你可以定义一个match_results对象来操作子匹配的集合索引,每一个子匹配都可以放在一个sub_match对象中。 模板类match_results表示一个代表正则表达式匹配的字符序列的集合。match_results对象被传递给regex_match 和 regex_search,并且被regex_iterator返回。 存储集合的内存在必要的时候被match_results的成员函数分配或释放。 match_results对象符合作为序列的要求,这些要求在lib.sequence.reqmts中可以找到,只有操作符被限制为常量序列。 对于match_results模板,我们通常使用这几个已定义的typedefs——cmatch,wcmatch,smatch, 或wsmatch: template <class BidirectionalIterator, class Allocator = allocator<sub_match<BidirectionalIterator> > class match_results; typedef match_results<const char*> cmatch; typedef match_results<const wchar_t*> wcmatch; typedef match_results<string::const_iterator> smatch; typedef match_results<wstring::const_iterator> wsmatch; template <class BidirectionalIterator, class Allocator = allocator<sub_match<BidirectionalIterator> > class match_results { public: typedef sub_match<BidirectionalIterator> value_type; typedef const value_type& const_reference; typedef const_reference reference; typedef implementation defined const_iterator; typedef const_iterator iterator; typedef typename iterator_traits<BidirectionalIterator>::difference_type difference_type; typedef typename Allocator::size_type size_type; typedef Allocator allocator_type; typedef typename iterator_traits<BidirectionalIterator>::value_type char_type; typedef basic_string<char_type> string_type; // construct/copy/destroy: explicit match_results(const Allocator& a = Allocator()); match_results(const match_results& m); match_results& operator=(const match_results& m); ~match_results(); // size: size_type size() const; size_type max_size() const; bool empty() const; // element access: difference_type length(int sub = 0) const; difference_type position(unsigned int sub = 0) const; string_type str(int sub = 0) const; const_reference operator[](int n) const; const_reference prefix() const; const_reference suffix() const; const_iterator begin() const; const_iterator end() const; // format: template <class OutputIterator> OutputIterator format(OutputIterator out, const string_type& fmt, match_flag_type flags = format_default) const; string_type format(const string_type& fmt, match_flag_type flags = format_default) const; allocator_type get_allocator() const; void swap(match_results& that); #ifdef BOOST_REGEX_MATCH_EXTRA typedef typename value_type::capture_sequence_type capture_sequence_type; const capture_sequence_type& captures(std::size_t i)const; #endif }; template <class BidirectionalIterator, class Allocator> bool operator == (const match_results<BidirectionalIterator, Allocator>& m1, const match_results<BidirectionalIterator, Allocator>& m2); template <class BidirectionalIterator, class Allocator> bool operator != (const match_results<BidirectionalIterator, Allocator>& m1, const match_results<BidirectionalIterator, Allocator>& m2); template <class charT, class traits, class BidirectionalIterator, class Allocator> basic_ostream<charT, traits>& operator << (basic_ostream<charT, traits>& os, const match_results<BidirectionalIterator, Allocator>& m); template <class BidirectionalIterator, class Allocator> void swap(match_results<BidirectionalIterator, Allocator>& m1, match_results<BidirectionalIterator, Allocator>& m2);描述 match_results constructors In all match_results constructors, a copy of the Allocator argument is used for any memory allocation performed by the constructor or member functions during the lifetime of the object. match_results(const Allocator& a = Allocator());效果: Constructs an object of class match_results. The postconditions of this function are indicated in the table: Element Value empty() true size() 0 str() basic_string() match_results(const match_results& m);效果: Constructs an object of class match_results, as a copy of m. match_results& operator=(const match_results& m);效果: Assigns m to *this. The postconditions of this function are indicated in the table: Element Value empty() m.empty() size() m.size() str(n) m.str(n) for all integers n < m.size(). prefix() m.prefix(). suffix() m.suffix(). (*this)[n] m[n] for all integers n < m.size(). length(n) m.length(n) for all integers n < m.size(). position(n) m.position(n) for all integers n < m.size(). match_results size size_type size()const;效果: Returns the number of sub_match elements stored in *this; that is the number of marked sub-expressions in the regular expression that was matched plus one. size_type max_size()const;效果: Returns the maximum number of sub_match elements that can be stored in *this. bool empty()const;效果: Returns size() == 0. match_results element access difference_type length(int sub = 0)const;效果: Returns the length of sub-expression sub, that is to say: (*this)[sub].length(). difference_type position(unsigned int sub = 0)const;效果: Returns the starting location of sub-expression sub, or -1 if sub was not matched . string_type str(int sub = 0)const;效果: Returns sub-expression sub as a string: string_type((*this)[sub]). const_reference operator[](int n) const;效果: Returns a reference to the sub_match object representing the character sequence that matched marked sub-expression n. If n == 0 then returns a reference to a sub_match object representing the character sequence that matched the whole regular expression. If n is out of range, or if n is an unmatched sub-expression, then returns a sub_match object whose matched member is false. const_reference prefix()const;效果: Returns a reference to the sub_match object representing the character sequence from the start of the string being matched/searched, to the start of the match found. const_reference suffix()const;效果: Returns a reference to the sub_match object representing the character sequence from the end of the match found to the end of the string being matched/searched. const_iterator begin()const;效果: Returns a starting iterator that enumerates over all the marked sub-expression matches stored in *this. const_iterator end()const;效果: Returns a terminating iterator that enumerates over all the marked sub-expression matches stored in *this. match_results reformatting template <class OutputIterator> OutputIterator format(OutputIterator out, const string_type& fmt, match_flag_type flags = format_default);前提: The type OutputIterator? conforms to the Output Iterator requirements (24.1.2). 效果: Copies the character sequence [fmt.begin(), fmt.end()) to OutputIterator? out. For each format specifier or escape sequence in fmt, replace that sequence with either the character(s) it represents, or the sequence of characters within *this to which it refers. The bitmasks specified in flags determines what format specifiers or escape sequences are recognized, by default this is the format used by ECMA-262, ECMAScript Language Specification, Chapter 15 part 5.4.11 String.prototype.replace. 返回值: out. string_type format(const string_type& fmt, match_flag_type flags = format_default);效果: Returns a copy of the string fmt. For each format specifier or escape sequence in fmt, replace that sequence with either the character(s) it represents, or the sequence of characters within *this to which it refers. The bitmasks specified in flags determines what format specifiers or escape sequences are recognized, by default this is the format used by ECMA-262, ECMAScript Language Specification, Chapter 15 part 5.4.11 String.prototype.replace. Allocator access allocator_type get_allocator()const;效果: Returns a copy of the Allocator that was passed to the object/s constructor. Swap void swap(match_results& that);效果: Swaps the contents of the two sequences. 充分条件: *this contains the sequence of matched sub-expressions that were in that, that contains the sequence of matched sub-expressions that were in *this. 复杂度: 常数时间 Captures typedef typename value_type::capture_sequence_type capture_sequence_type; Defines an implementation-specific type that satisfies the requirements of a standard library Sequence (21.1.1 including the optional Table 68 operations), whose value_type is a sub_match. This type happens to be std::vector >, but you shouldn/t actually rely on that. const capture_sequence_type& captures(std::size_t i)const; 效果: returns a sequence containing all the captures obtained for sub-expression i. 返回值: (*this).captures(); Preconditions: the library must be built and used with BOOST_REGEX_MATCH_EXTRA defined, and you must pass the flag match_extra to the regex matching functions (regex_match, regex_search, regex_iterator or regex_token_iterator) in order for this member function to be defined and return useful information. Rationale: Enabling this feature has several consequences: * sub_match occupies more memory resulting in complex expressions running out of memory or stack space more quickly during matching. * The matching algorithms are less efficient at handling some features (independent sub-expressions for example), even when match_extra is not used. * The matching algorithms are much less efficient (i.e. slower), when match_extra is used. Mostly this is down to the extra memory allocations that have to take place. match_results non-members template <class BidirectionalIterator, class Allocator> bool operator == (const match_results<BidirectionalIterator, Allocator>& m1, const match_results<BidirectionalIterator, Allocator>& m2);效果: Compares the two sequences for equality. template <class BidirectionalIterator, class Allocator> bool operator != (const match_results<BidirectionalIterator, Allocator>& m1, const match_results<BidirectionalIterator, Allocator>& m2);效果: Compares the two sequences for inequality. template <class charT, class traits, class BidirectionalIterator, class Allocator> basic_ostream<charT, traits>& operator << (basic_ostream<charT, traits>& os, const match_results<BidirectionalIterator, Allocator>& m);效果: Writes the contents of m to the stream os as if by calling os << m.str(); Returns os.. template <class BidirectionalIterator, class Allocator> void swap(match_results<BidirectionalIterator, Allocator>& m1, match_results<BidirectionalIterator, Allocator>& m2);效果: Swaps the contents of the two sequences. Algorithms regex_match Contents Synopsis Description Examples Synopsis #include <boost/regex.hpp> regex_match算法决定了一个给定的正则表达式是否完全匹配一个给定的字符序列,这个序列由一对双向迭代器标识,算法定义如下,主要作用是数据输入校验。 *注意:仅当表达式完全匹配输入序列,函数值才为真。* 如果你想搜索序列中某个部分请使用regex_search。如果你要匹配字符串的前缀,那么请设置match_continuous标志,并使用regex_search。 template <class BidirectionalIterator, class Allocator, class charT, class traits, class Allocator2> bool regex_match(BidirectionalIterator first, BidirectionalIterator last, match_results<BidirectionalIterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template <class BidirectionalIterator, class charT, class traits, class Allocator2> bool regex_match(BidirectionalIterator first, BidirectionalIterator last, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template <class charT, class Allocator, class traits, class Allocator2> bool regex_match(const charT* str, match_results<const charT*, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template <class ST, class SA, class Allocator, class charT, class traits, class Allocator2> bool regex_match(const basic_string<charT, ST, SA>& s, match_results<typename basic_string<charT, ST, SA>::const_iterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template <class charT, class traits, class Allocator2> bool regex_match(const charT* str, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template <class ST, class SA, class charT, class traits, class Allocator2> bool regex_match(const basic_string<charT, ST, SA>& s, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);Description template <class BidirectionalIterator, class Allocator, class charT, class traits, class Allocator2> bool regex_match(BidirectionalIterator first, BidirectionalIterator last, match_results<BidirectionalIterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);前提: Type BidirectionalIterator? meets the requirements of a Bidirectional Iterator (24.1.4). 效果: Determines whether there is an exact match between the regular expression e, and all of the character sequence [first, last), parameter flags is used to control how the expression is matched against the character sequence. Returns true if such a match exists, false otherwise. 异常: std::runtime_error if the complexity of matching the expression against an N character string begins to exceed O(N2), or if the program runs out of stack space while matching the expression (if Boost.regex is configured in recursive mode), or if the matcher exhausts it/s permitted memory allocation (if Boost.regex is configured in non-recursive mode). 影响: If the function returns false, then the effect on parameter m is undefined, otherwise the effects on parameter m are given in the table: Element Value m.size() e.mark_count() m.empty() false m.prefix().first first m.prefix().last first m.prefix().matched false m.suffix().first last m.suffix().last last m.suffix().matched false m[0].first first m[0].second last m[0].matched true if a full match was found, and false if it was a partial match (found as a result of the match_partial flag being set). m[n].first For all integers n < m.size(), the start of the sequence that matched sub-expression n. Alternatively, if sub-expression n did not participate in the match, then last. m[n].second For all integers n < m.size(), the end of the sequence that matched sub-expression n. Alternatively, if sub-expression n did not participate in the match, then last. m[n].matched|For all integers n < m.size(), true if sub-expression n participated in the match, false otherwise.| template <class BidirectionalIterator, class charT, class traits, class Allocator2> bool regex_match(BidirectionalIterator first, BidirectionalIterator last, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);效果: Behaves "as if" by constructing an instance of match_results what, and then returning the result of regex_match(first, last, what, e, flags). template <class charT, class Allocator, class traits, class Allocator2> bool regex_match(const charT* str, match_results<const charT*, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);效果: Returns the result of regex_match(str, str + char_traits::length(str), m, e, flags). template <class ST, class SA, class Allocator, class charT, class traits, class Allocator2> bool regex_match(const basic_string<charT, ST, SA>& s, match_results<typename basic_string<charT, ST, SA>::const_iterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);效果: Returns the result of regex_match(s.begin(), s.end(), m, e, flags). template <class charT, class traits, class Allocator2> bool regex_match(const charT* str, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);效果: Returns the result of regex_match(str, str + char_traits::length(str), e, flags). template <class ST, class SA, class charT, class traits, class Allocator2> bool regex_match(const basic_string<charT, ST, SA>& s, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);效果: Returns the result of regex_match(s.begin(), s.end(), e, flags). 样例 下面的例子处理一个ftp响应: #include <stdlib.h> #include <boost/regex.hpp> #include <string> #include <iostream> using namespace boost; regex expression("([0-9]+)(-| |$)(.*)"); // process_ftp: // on success returns the ftp response code, and fills // msg with the ftp response message. int process_ftp(const char* response, std::string* msg) { cmatch what; if(regex_match(response, what, expression)) { // what[0] contains the whole string // what[1] contains the response code // what[2] contains the separator character // what[3] contains the text message. if(msg) msg->assign(what[3].first, what[3].second); return std::atoi(what[1].first); } // failure did not match if(msg) msg->erase(); return -1; }regex_search Contents Synopsis Description Examples Synopsis #include <boost/regex.hpp> The algorithm regex_search will search a range denoted by a pair of bidirectional-iterators for a given regular expression. The algorithm uses various heuristics to reduce the search time by only checking for a match if a match could conceivably start at that position. The algorithm is defined as follows: template <class BidirectionalIterator, class Allocator, class charT, class traits, class Allocator2> bool regex_search(BidirectionalIterator first, BidirectionalIterator last, match_results<BidirectionalIterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template <class ST, class SA, class Allocator, class charT, class traits, class Allocator2> bool regex_search(const basic_string<charT, ST, SA>& s, match_results< typename basic_string<charT, ST,SA>::const_iterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template<class charT, class Allocator, class traits, class Allocator2> bool regex_search(const charT* str, match_results<const charT*, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default); template <class BidirectionalIterator, class Allocator, class charT, class traits> bool regex_search(BidirectionalIterator first, BidirectionalIterator last, const basic_regex<charT, traits, Allocator>& e, match_flag_type flags = match_default); template <class charT, class Allocator, class traits> bool regex_search(const charT* str, const basic_regex<charT, traits, Allocator>& e, match_flag_type flags = match_default); template<class ST, class SA, class Allocator, class charT, class traits> bool regex_search(const basic_string<charT, ST, SA>& s, const basic_regex<charT, traits, Allocator>& e, match_flag_type flags = match_default);Description template <class BidirectionalIterator, class Allocator, class charT, class traits, class Allocator2> bool regex_search(BidirectionalIterator first, BidirectionalIterator last, match_results<BidirectionalIterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);Requires: Type BidirectionalIterator? meets the requirements of a Bidirectional Iterator (24.1.4). 效果: Determines whether there is some sub-sequence within [first,last) that matches the regular expression e, parameter flags is used to control how the expression is matched against the character sequence. Returns true if such a sequence exists, false otherwise. 异常: std::runtime_error if the complexity of matching the expression against an N character string begins to exceed O(N2), or if the program runs out of stack space while matching the expression (if Boost.regex is configured in recursive mode), or if the matcher exhausts it/s permitted memory allocation (if Boost.regex is configured in non-recursive mode). Postconditions: If the function returns false, then the effect on parameter m is undefined, otherwise the effects on parameter m are given in the table: Element Value m.size() e.mark_count() m.empty() false m.prefix().first first m.prefix().last m[0].first m.prefix().matched m.prefix().first = m.prefix().second m.suffix().first m[0].second m.suffix().last last m.suffix().matched m.suffix().first = m.suffix().second m[0].first The start of the sequence of characters that matched the regular expression m[0].second The end of the sequence of characters that matched the regular expression m[0].matched true if a full match was found, and false if it was a partial match (found as a result of the match_partial flag being set). m[n].first For all integers n < m.size(), the start of the sequence that matched sub-expression n. Alternatively, if sub-expression n did not participate in the match, then last. m[n].second For all integers n < m.size(), the end of the sequence that matched sub-expression n. Alternatively, if sub-expression n did not participate in the match, then last. m[n].matched For all integers n < m.size(), true if sub-expression n participated in the match, false otherwise. template <class charT, class Allocator, class traits, class Allocator2> bool regex_search(const charT* str, match_results<const charT*, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);效果: Returns the result of regex_search(str, str + char_traits::length(str), m, e, flags). template <class ST, class SA, class Allocator, class charT, class traits, class Allocator2> bool regex_search(const basic_string<charT, ST, SA>& s, match_results<typename basic_string<charT, ST, SA>::const_iterator, Allocator>& m, const basic_regex<charT, traits, Allocator2>& e, match_flag_type flags = match_default);效果: Returns the result of regex_search(s.begin(), s.end(), m, e, flags). template <class iterator, class Allocator, class charT, class traits> bool regex_search(iterator first, iterator last, const basic_regex<charT, traits, Allocator>& e, match_flag_type flags = match_default);效果: Behaves "as if" by constructing an instance of match_results what, and then returning the result of regex_search(first, last, what, e, flags). template <class charT, class Allocator, class traits> bool regex_search(const charT* str const basic_regex<charT, traits, Allocator>& e, match_flag_type flags = match_default);效果: Returns the result of regex_search(str, str + char_traits::length(str), e, flags). template <class ST, class SA, class Allocator, class charT, class traits> bool regex_search(const basic_string<charT, ST, SA>& s, const basic_regex<charT, traits, Allocator>& e, match_flag_type flags = match_default);效果: Returns the result of regex_search(s.begin(), s.end(), e, flags). Examples The following example, takes the contents of a file in the form of a string, and searches for all the C++ class declarations in the file. The code will work regardless of the way that std::string is implemented, for example it could easily be modified to work with the SGI rope class, which uses a non-contiguous storage strategy. #include <string> #include <map> #include <boost/regex.hpp> // purpose: // takes the contents of a file in the form of a string // and searches for all the C++ class definitions, storing // their locations in a map of strings/int/s typedef std::map<std::string, int, std::less<std::string> > map_type; boost::regex expression("^(template[[:space:]]*<[^;:{]+>[[:space:]]*)?(class|struct)[[:space:]]*(<w+>([[:blank:]]*([^)]*))?[[:space:]]*)*(<w*>)[[:space:]]*(<[^;:{]+>[[:space:]]*)?({|:[^;{()]*{)"); void IndexClasses(map_type& m, const std::string& file) { std::string::const_iterator start, end; start = file.begin(); end = file.end(); boost::match_results<std::string::const_iterator> what; boost::match_flag_type flags = boost::match_default; while(regex_search(start, end, what, expression, flags)) { // what[0] contains the whole string // what[5] contains the class name. // what[6] contains the template specialisation if any. // add class name and position to map: m[std::string(what[5].first, what[5].second) + std::string(what[6].first, what[6].second)] = what[5].first - file.begin(); // update search position: start = what[0].second; // update flags: flags |= boost::match_prev_avail; flags |= boost::match_not_bob; } }regex_replace Contents Synopsis Description Examples Synopsis #include <boost/regex.hpp> The algorithm regex_replace searches through a string finding all the matches to the regular expression: for each match it then calls match_results::format to format the string and sends the result to the output iterator. Sections of text that do not match are copied to the output unchanged only if the flags parameter does not have the flag format_no_copy set. If the flag format_first_only is set then only the first occurrence is replaced rather than all occurrences. template <class OutputIterator, class BidirectionalIterator, class traits, class Allocator, class charT> OutputIterator regex_replace(OutputIterator out, BidirectionalIterator first, BidirectionalIterator last, const basic_regex<charT, traits, Allocator>& e, const basic_string<charT>& fmt, match_flag_type flags = match_default); template <class traits, class Allocator, class charT0 |
C++ Boost regex 文档(翻译)
最新推荐文章于 2024-09-19 14:31:18 发布
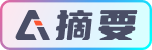