父组件 Tabs.vue
<template>
<div>
<h2>我是一个选项卡</h2>
<div class="tabs">
<el-button type="primary" :class="{ active: showtype === 'Home' }" @click="showtype = 'Home'" >Home</el-button>
<el-button type="primary" :class="{ active: showtype === 'Post' }" @click="showtype = 'Post'" >Post</el-button>
<el-button type="primary" :class="{ active: showtype === 'About' }" @click="showtype = 'About'" >About</el-button>
</div>
<!-- 展示某一个组件 -->
<div class="show">
<!-- 少量出现的用 v-if -->
<!-- <Home v-if="showtype === 'Home'" /> -->
<!-- <Post v-else-if="showtype === 'Post'" /> -->
<!-- <About v-else-if="showtype === 'About'" /> -->
<!-- 频繁切换的用 v-show -->
<!-- <Home v-show="showtype === 'Home'" /> -->
<!-- <Post v-show="showtype === 'Post'" /> -->
<!-- <About v-show="showtype === 'About'" /> -->
<!-- 语法糖 -->
<!-- 使用 vue 自带的 component 元素,加上特殊的 is 属性简化上面 v-if 的写法。-->
<!-- is 属性的属性值必须和组件名相同才能正确展示组件 -->
<!-- 动态组件 -->
<!-- <keep-alive> -->
<!-- <component :Title="title" @change-post="changeTitle" v-bind:is="showtype">
</component> -->
<!-- </keep-alive> -->
<!-- component 相当与 vue 的一个空组件,里面什么都没有,is 相当于里面要装什么,要变成什么。 -->
<!-- 变量可以是 1. 已注册组件的名字 2. 一个组件的选项对象 -->
<!-- 子组件想要修改或者同步父组件的 data ,一般情况是在父组件定义修改方法然后传递给子组件去执行,通过自定义事件方式。 -->
<!-- 上述做法官方提供了一个语法糖 .sync-->
<!-- $event 代表子组件传递的参数 -->
<!-- 子组件内调用传递的事件名必须写成 update:proName -->
<!-- <component :Title="title" @change-post="chan/geTitle" :is="showtype"> -->
<!-- <component :Title="title" v-on:update:title="title = $event" :is="showtype"> -->
<component :title.sync="title" :is="showtype"></component>
<!-- keep-alive -->
<!-- include - 字符串或正则表达式。只有名称匹配的组件会被缓存。 -->
<!-- exclude - 字符串或正则表达式。任何名称匹配的组件都不会被缓存。 -->
<!-- max - 数字。最多可以缓存多少组件实例。 -->
<!-- <keep-alive include="Post" exclude="About" :max="10"><component :is="showtype"></component></keep-alive> -->
</div>
</div>
</template>
子组件 Post.vue
<template>
<div class="post">
<div class="left">
<!-- <el-button @click="num = '1'" type="success" size="mini">第一篇</el-button> -->
<!-- <el-button @click="num = '2'" type="success" size="mini">第二篇</el-button> -->
<!-- <el-button @click="num = '3'" type="success" size="mini">第三篇</el-button> -->
<!-- 传参的修改方法 -->
<!-- 语法糖的方法 -->
<!-- this.$emit('update:title', newTitle) -->
<!-- <el-button @click="自己的方法()" type="success" size="mini">第一篇</el-button> -->
<!-- <el-button @click="$emit('update:title','1')" type="success" size="mini">第一篇</el-button> -->
<!-- <el-button @click="$emit('update:title','2')" type="success" size="mini">第二篇</el-button> -->
<!-- <el-button @click="$emit('update:title','3')" type="success" size="mini">第三篇</el-button> -->
<el-button @click="xxx(1)" type="success" size="mini">第一篇</el-button>
<el-button @click="xxx(2)" type="success" size="mini">第二篇</el-button>
<el-button @click="xxx(3)" type="success" size="mini">第三篇</el-button>
<!-- <el-button @click="$emit('change-post','1')" type="success" size="mini">第一篇</el-button> -->
<!-- <el-button @click="$emit('change-post','2')" type="success" size="mini">第二篇</el-button> -->
<!-- <el-button @click="$emit('change-post','3')" type="success" size="mini">第三篇</el-button> -->
</div>
<div class="right">
<!-- 切换次数多的话应该用 v-show 条件展示 -->
<!-- 问题:切换的时候怎么才能保留子组件里面的选项。 -->
<!-- 在父组件定义一个 title 写方法 。传给 Post 组件修改,当 Post 组件销毁的时候 title 是没有变化的,所以达到了目的。-->
<!-- 解决方案:在父组件中添加 <keep-alive> -->
<!-- <div v-if="num === '0'">请点击左侧的按钮</div> -->
<!-- <div v-if="num === '1'">Failed</div> -->
<!-- <div v-if="num === '2'">Failed to mount</div> -->
<!-- <div v-if="num === '3'">Failed to mount component:function</div> -->
<!-- 但是有一个不好的地方 -->
<!-- <div v-if="title === '0'">请点击左侧的按钮</div> -->
<!-- <div v-if="title === '1'">Failed</div> -->
<!-- <div v-if="title === '2'">Failed to mount</div> -->
<!-- <div v-if="title === '3'">Failed to mount component:function</div> -->
<div v-if="title === 0">请点击左侧的按钮</div>
<div v-if="title === 1">Failed</div>
<div v-if="title === 2">Failed to mount</div>
<div v-if="title === 3">Failed to mount component:function</div>
</div>
</div>
</template>
<script>
export default {
name: "Post",
props: ["title"],
data() {
return {
// num: 0,
// title: 0,
};
},
// created() {
// console.log("文章列表被创建");
// console.log(this.title);
// },
methods: {
xxx(a) {
this.$emit("update:title", a);
console.log(a);
// 其他的事
},
},
};
</script>
<style>
.post {
width: 800px;
height: 150px;
border: 1px solid #ccc;
overflow: auto;
}
.left {
width: 298px;
height: 130px;
margin-top: 5px;
border-right: 1px solid #ccc;
box-sizing: border-box;
float: left;
}
.left > button {
float: left;
}
.right {
width: 500px;
height: 150px;
float: left;
}
</style>
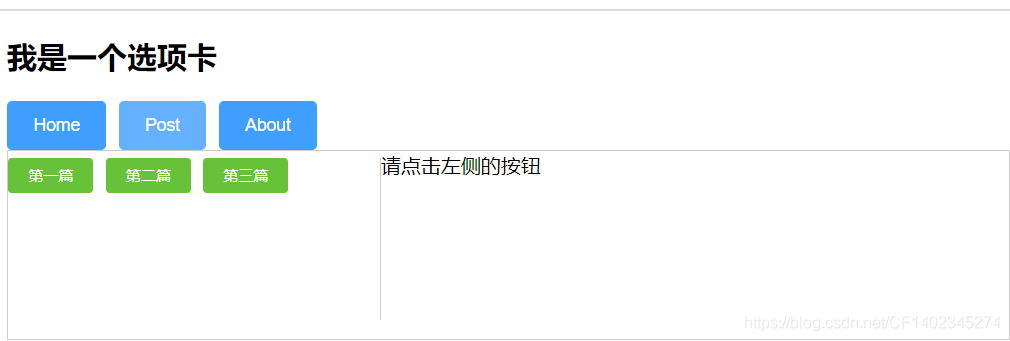
子组件向父组件传值的方法
<!-- -----------父组件----------------- -->
<component :title.sync="title" :is="showtype"></component>
title: 0,
<!-- ------------子组件----------------- -->
<el-button @click="xxx(1)" type="success" size="mini">第一篇</el-button>
<div v-if="title === 0">请点击左侧的按钮</div>
props: ["title"],
methods: {
xxx(a) {
this.$emit("update:title", a);
console.log(a);
// 其他的事
},
},
解释代码:
- 父组件定义变量,语法糖传参,子组件定义自己的方法
xxx(a)
,参数a
传给父组件的方法update:title
当做title
的值。然后子组件内props: ["title"]
接收父组件传过来值使用,接收父组件传的参不需要加this
。 - 数据的传递过程 子组件内
a
--> 父组件中title
—> 子组件引用title
使用。