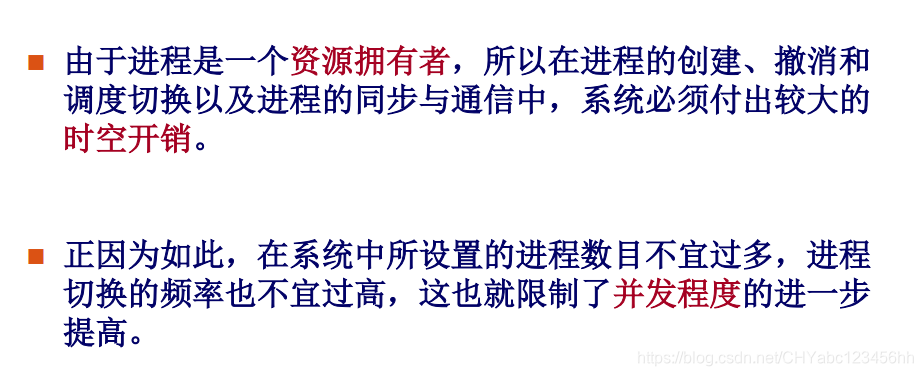
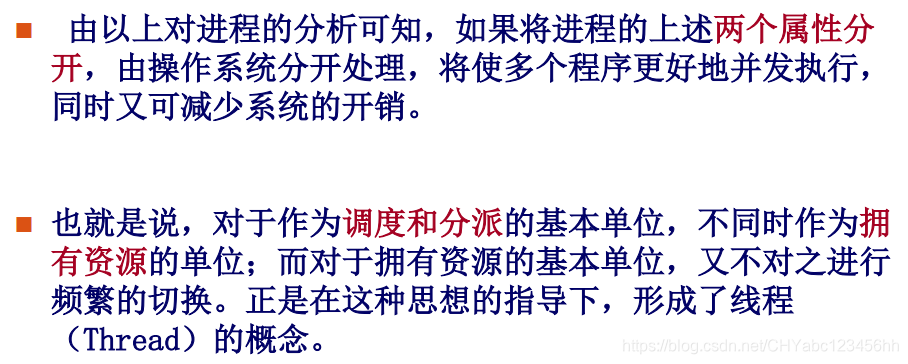
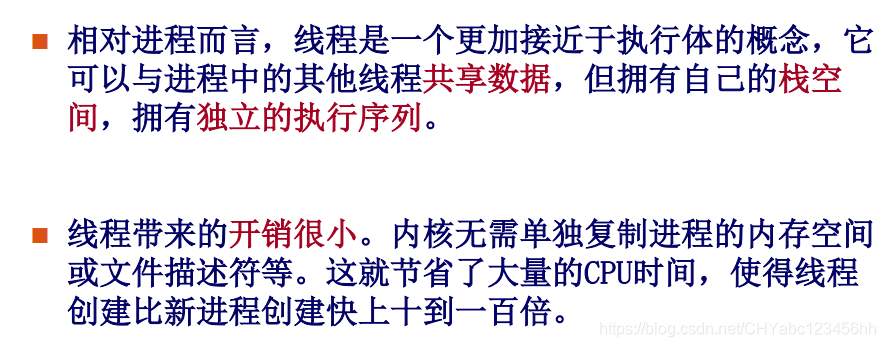
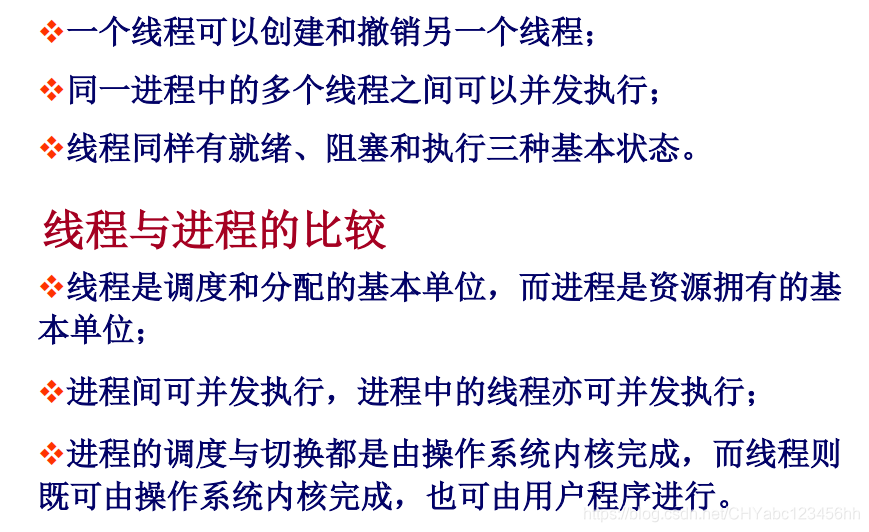
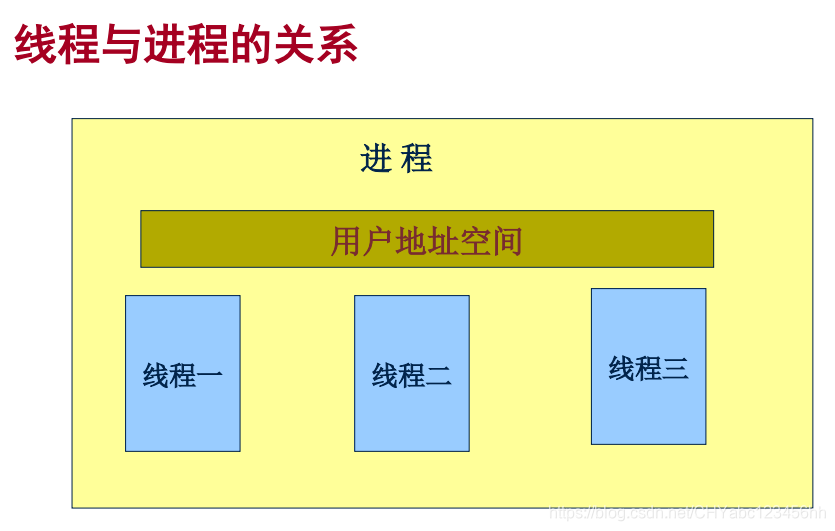
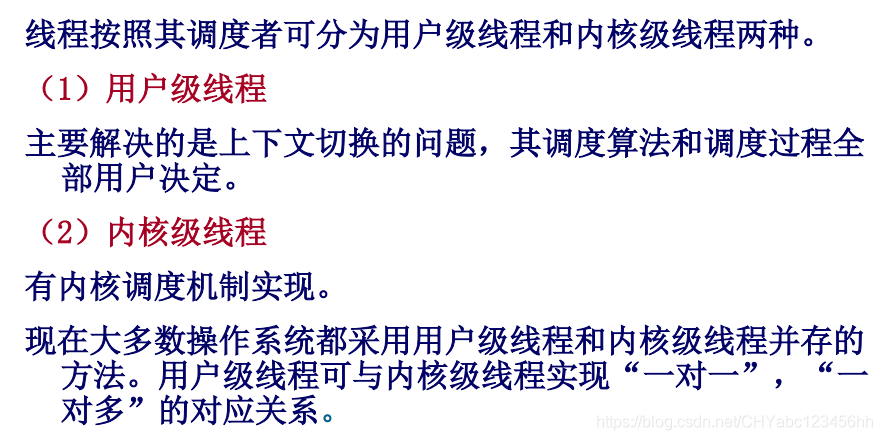

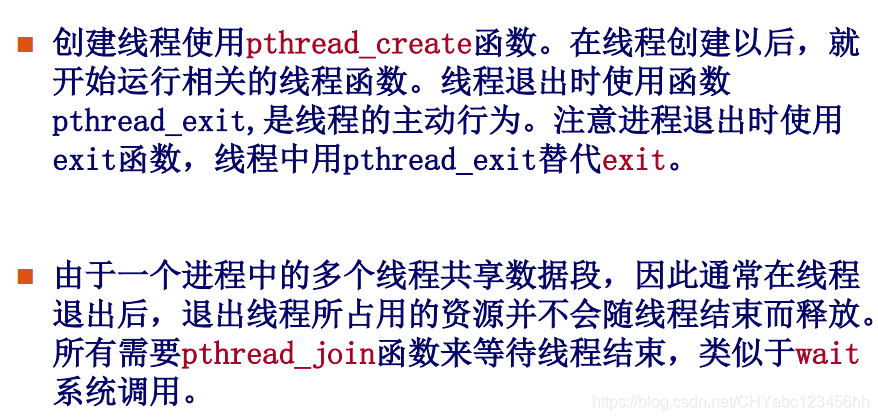
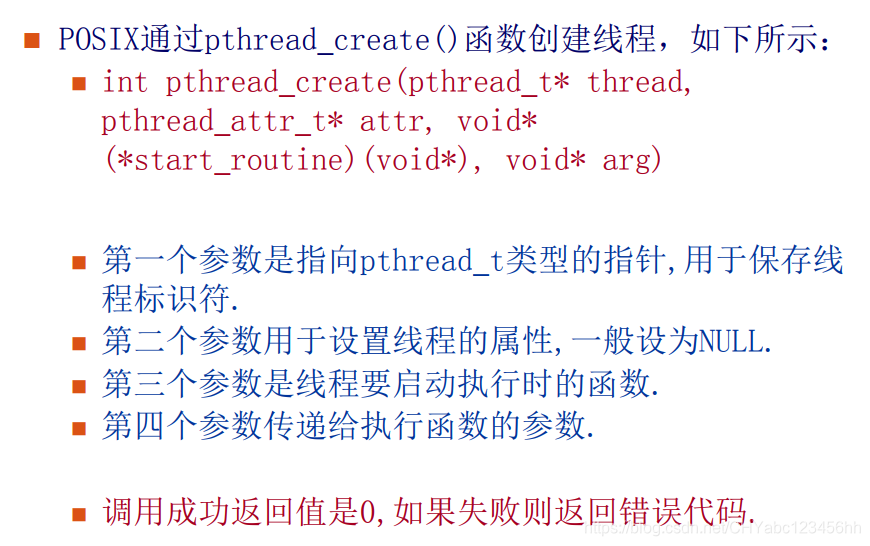
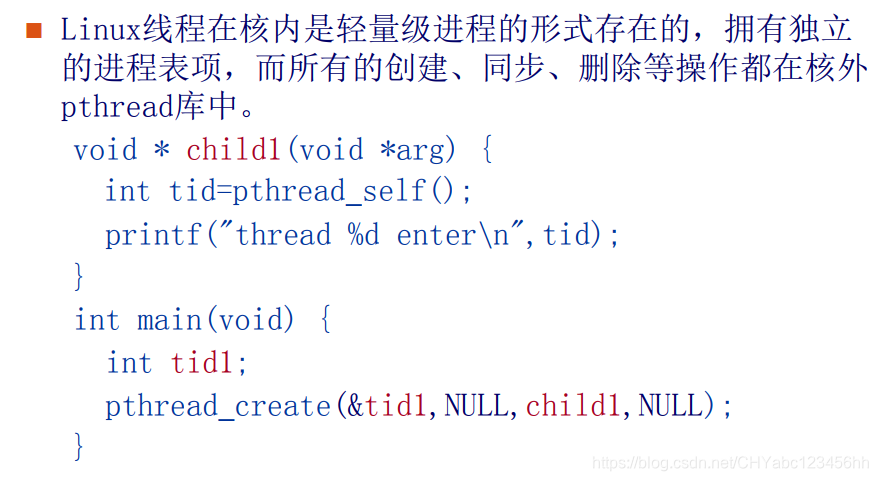
完整代码
#include <sys/shm.h>
#include <iostream>
#include <unistd.h>
#include <pthread.h>
void * child1(void *arg){
pthread_t tid = pthread_self();
printf("1 thread %lu \n",tid);
}
int main(int argc,char* argv[]) {
int result{};
pthread_t a_thread{};
pthread_t tid = pthread_self();
printf("mean thread %lu \n",tid);
result = pthread_create(&a_thread, nullptr,child1, nullptr);
if (result !=0){
printf("Thread create failed!");
}
return 0;
}
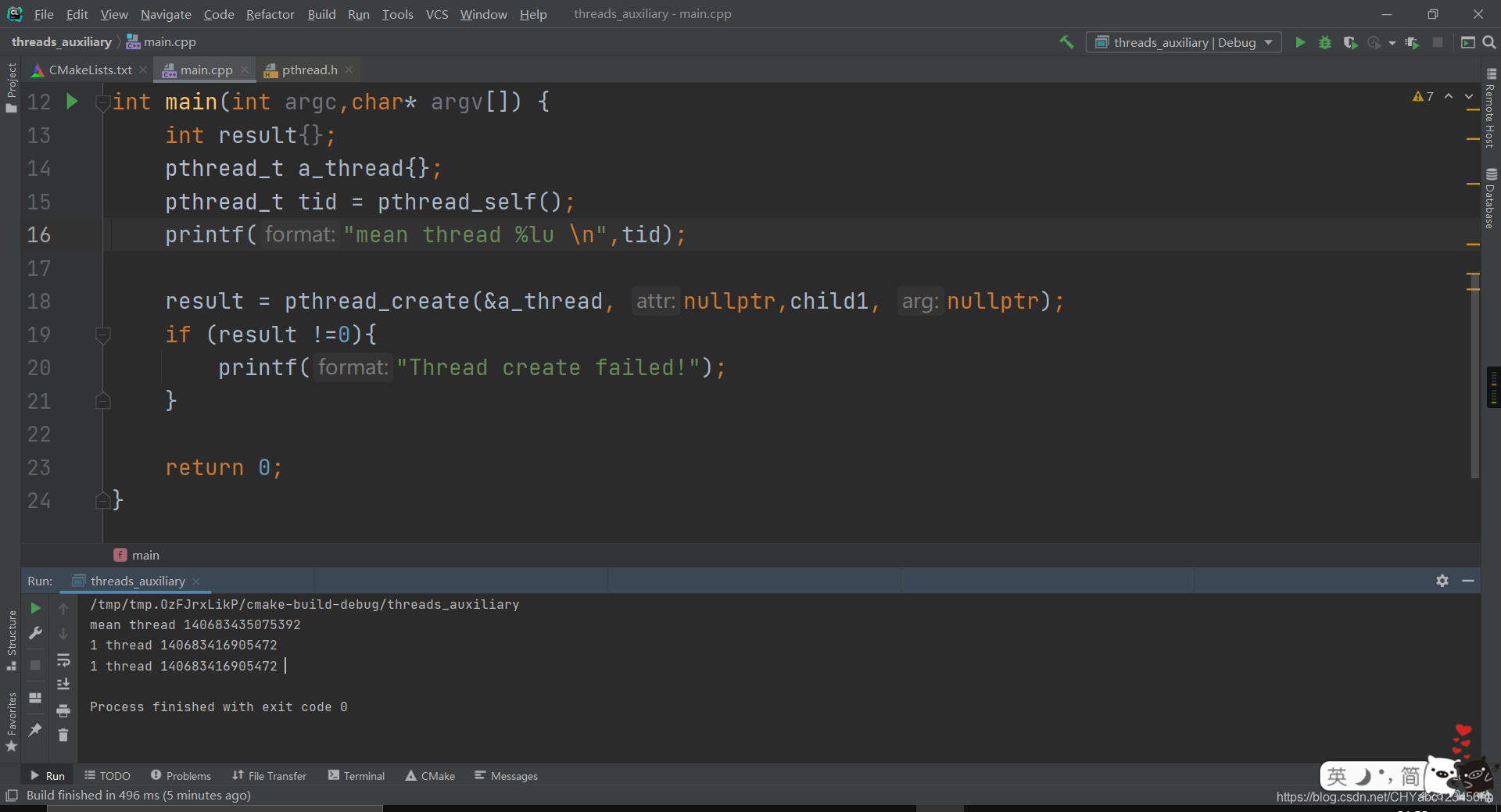
- 如果主线程 不延时,直接退出,子线程还未创建就结束了
线程终止
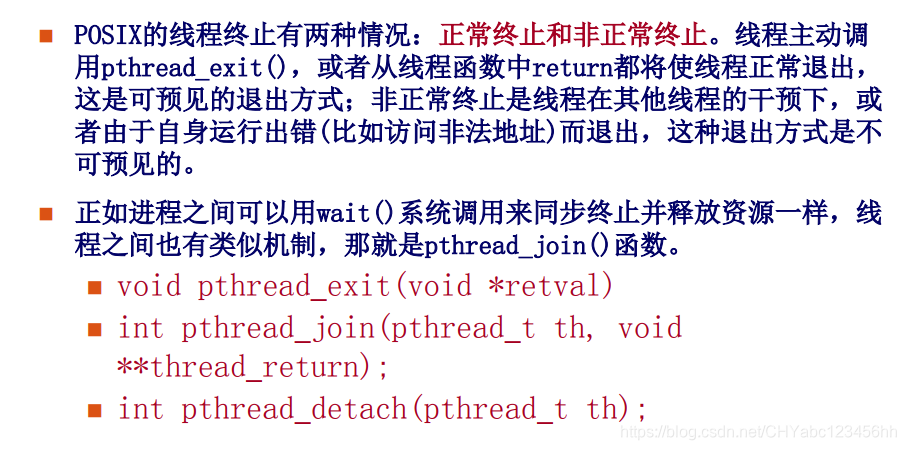
- pthread_join() 等待线程退出,第二个参数是线程退出返回的数值
#include <sys/shm.h>
#include <iostream>
#include <unistd.h>
#include <pthread.h>
#include <cstring>
void * child1(void *arg){
pthread_t tid = pthread_self();
printf("1 thread %lu \n",tid);
}
void * thread_function(void *arg);
int main(int argc,char* argv[]) {
int result{};
std::string message{"Hello World"};
pthread_t a_thread{};
pthread_t tid = pthread_self();
printf("mean thread %lu \n",tid);
result = pthread_create(&a_thread, nullptr,thread_function, (void *)(message.c_str()));
if (result !=0){
printf("Thread create failed!");
exit(EXIT_FAILURE);
}
printf("Waiting for thread to finish!\n");
void * thread_result;
result = pthread_join(a_thread,&thread_result);
if (result !=0){
printf("Thread join failed!");
exit(EXIT_FAILURE);
}
printf("Thread joined,it returned %s\n",(char *)thread_result);
printf("Message is now %s \n",message.c_str());
exit(EXIT_SUCCESS);
return 0;
}
void * thread_function(void *arg){
char * message = (char *)arg;
printf("thread_function is running.Argument was %s\n",message);
sleep(3);
strcpy(message,"Bye!");
pthread_exit((void *) "Thank you for the cpu time!");
}
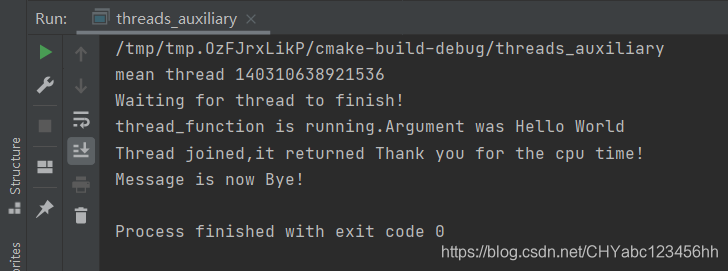
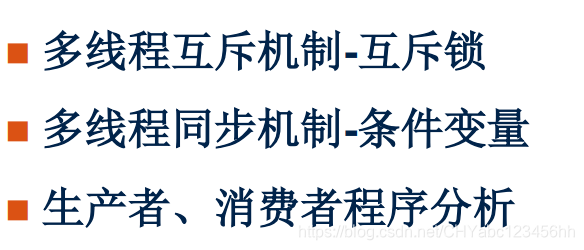
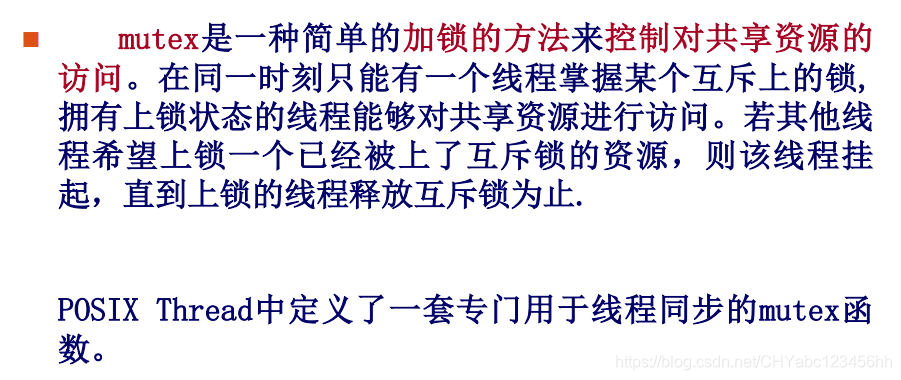
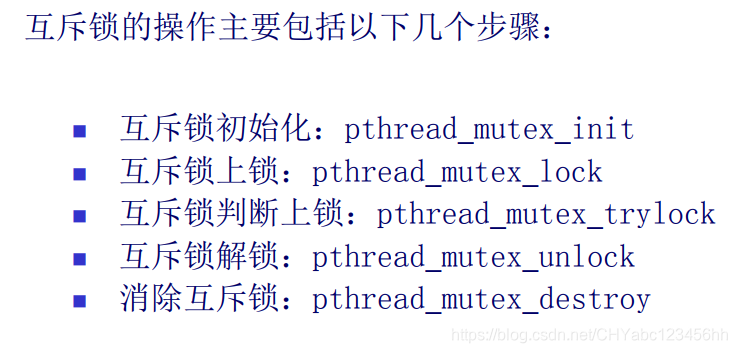
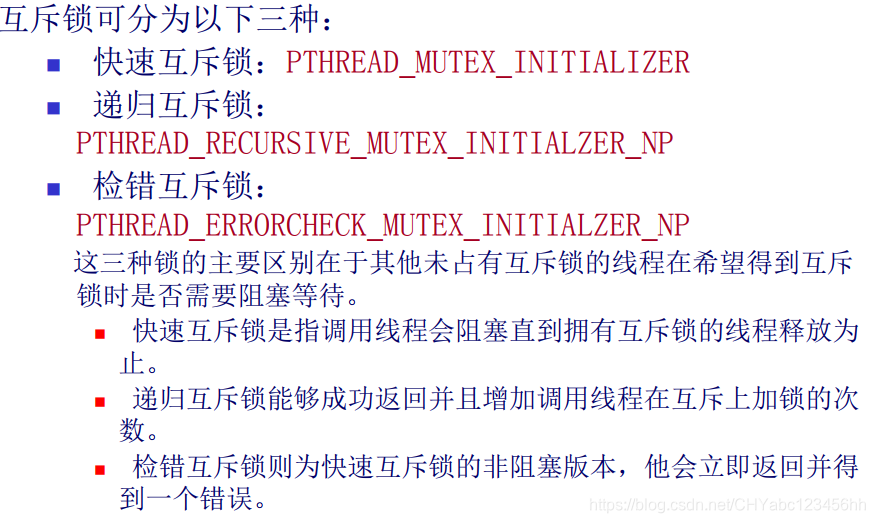
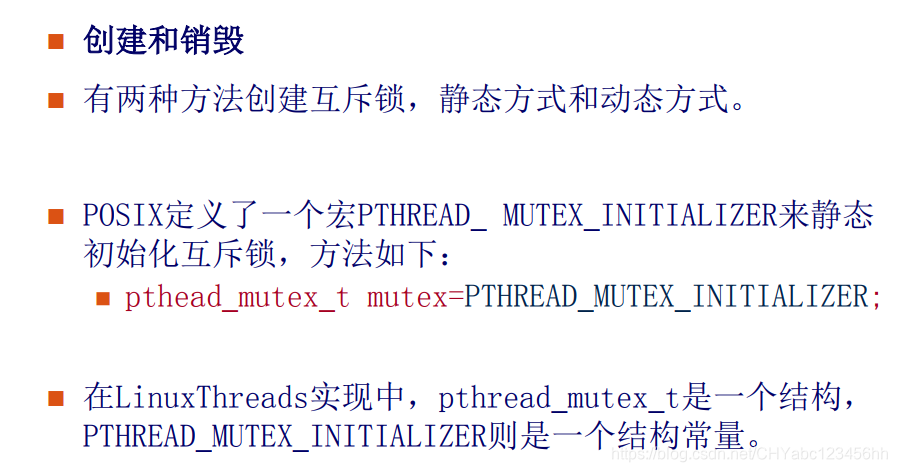
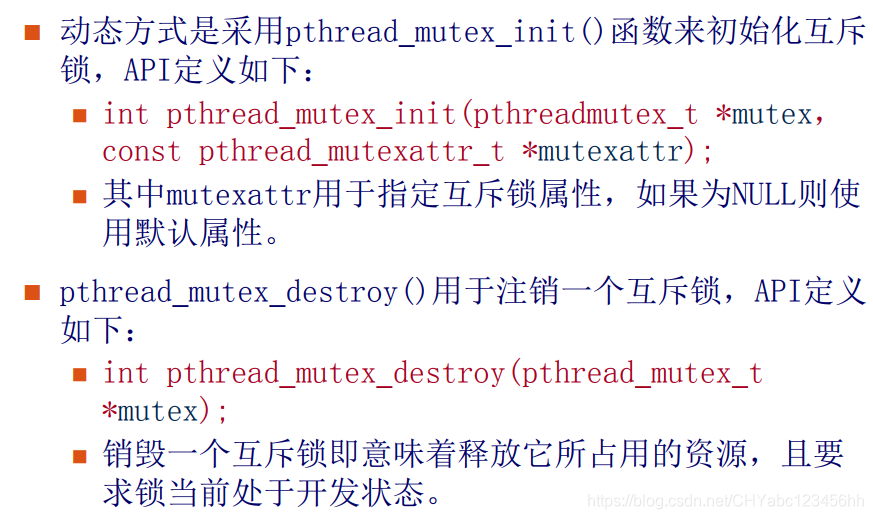
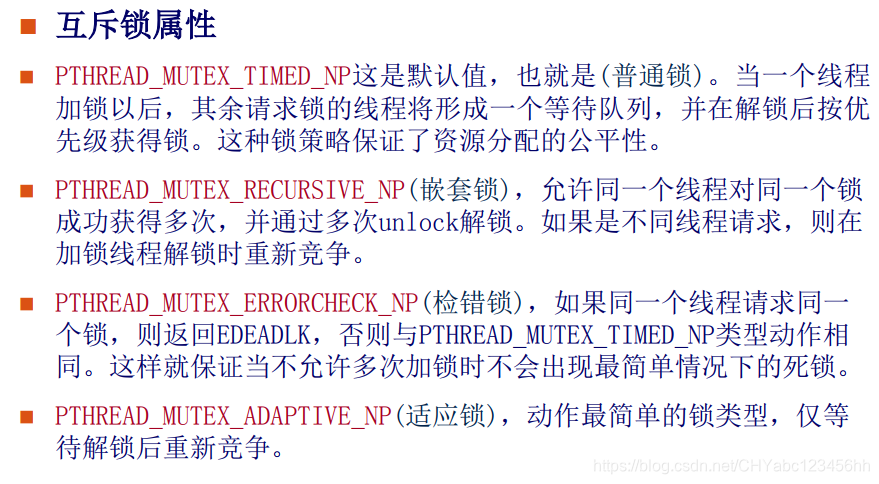
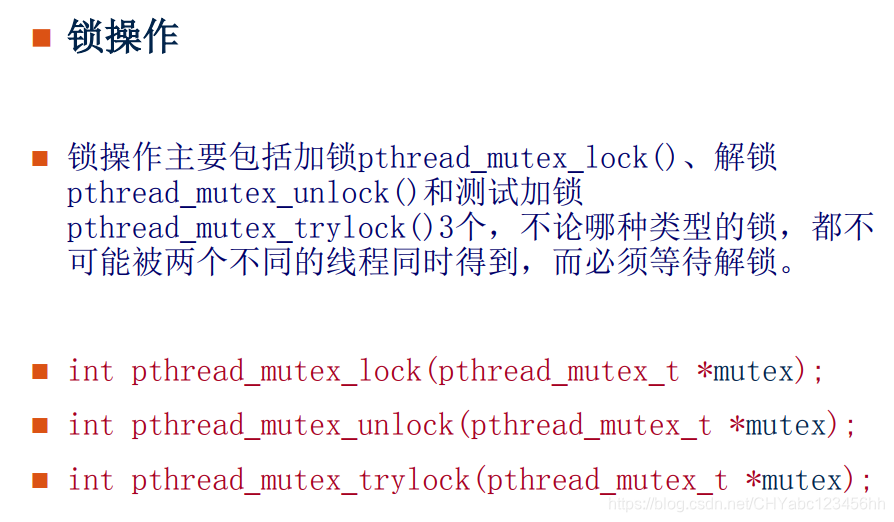
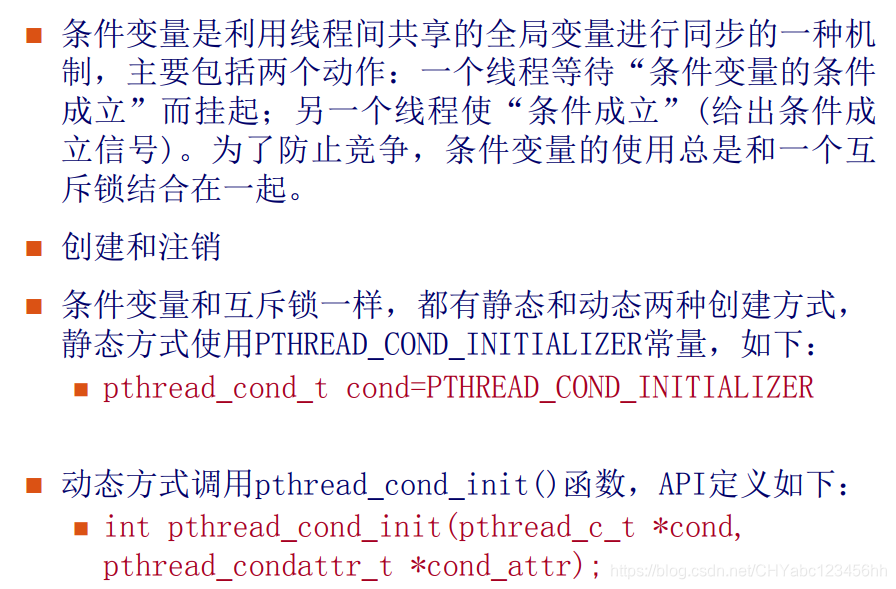
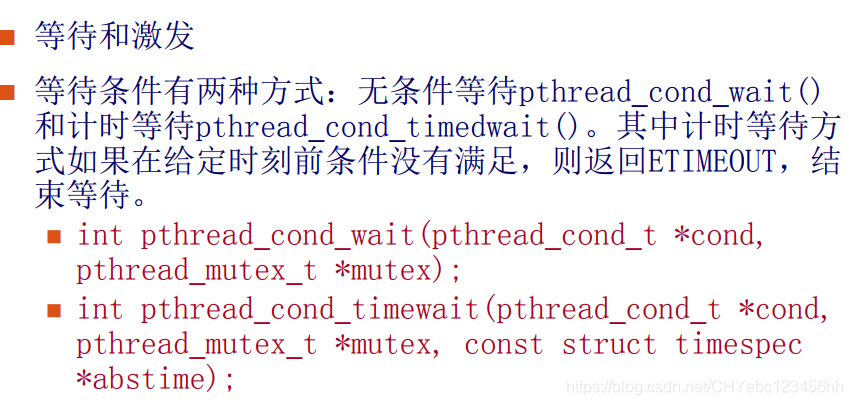
代码 生产者 消费者模型
#include <sys/shm.h>
#include <iostream>
#include <unistd.h>
#include <pthread.h>
#include <cstring>
#define BUFFER_SIZE 16
#define OVER -1
struct prodcons {
int buffer[BUFFER_SIZE];//设置缓冲区数组
pthread_mutex_t lock;//互斥锁
int read_pos,write_pos;//读写位置
pthread_cond_t not_empty;//缓冲区非空信号
pthread_cond_t not_full;//缓冲区非满信号
};
struct prodcons buffer;
void init(struct prodcons *b){
pthread_mutex_init(&b->lock, nullptr);
pthread_cond_init(&b->not_empty, nullptr);
pthread_cond_init(&b->not_full, nullptr);
b->read_pos = 0;
b->write_pos = 0;
}
void put(struct prodcons *b,int data){
pthread_mutex_lock(&b->lock);
//等待缓冲区非满
while ((b->write_pos + 1) % BUFFER_SIZE == b->read_pos ){
printf("wait for not full!\n");
pthread_cond_wait(&b->not_full,&b->lock);
}
//写数据并且指针前移
b->buffer[b->write_pos] = data;
b->write_pos++;
if (b->write_pos >= BUFFER_SIZE)
b->write_pos = 0;
//设置缓冲区非空的信号
pthread_cond_signal(&b->not_empty);
pthread_mutex_unlock(&b->lock);
}
int get(struct prodcons *b){
int data;
pthread_mutex_lock(&b->lock);
//等待缓冲区非空
while (b->write_pos == b->read_pos){
printf("wait for not empty!");
pthread_cond_wait(&b->not_empty,&b->lock);
}
//读数据并且指针前移
data = b->buffer[b->read_pos];
b->read_pos++;
if (b->read_pos >= BUFFER_SIZE)
b->read_pos = 0;
//设置缓冲区非满的信号
pthread_cond_signal(&b->not_full);
pthread_mutex_unlock(&b->lock);
return data;
}
void * producer(void *data){
for (int i = 0; i < 10; ++i) {
printf("put --> %d\n",i);
put(&buffer,i);
}
put(&buffer,OVER);
printf("producer stopped!\n");
return nullptr;
}
void * consumer(void *data){
int d{};
while (1){
d = get(&buffer);
if (d == OVER)
break;
printf("%d->get\n",d);
}
printf("consumer stopped!");
return nullptr;
}
int main(int argc,char* argv[]) {
pthread_t th_a{},th_b{};
void *ret_val;
struct prodcons x{};
init(&x);
pthread_create(&th_a, nullptr, reinterpret_cast<void *(*)(void *)>(producer), 0);
pthread_create(&th_b, nullptr,reinterpret_cast<void *(*)(void *)>(consumer), 0);
//等待生产者和消费者结束
pthread_join(th_a,&ret_val);
pthread_join(th_b,&ret_val);
return 0;
}
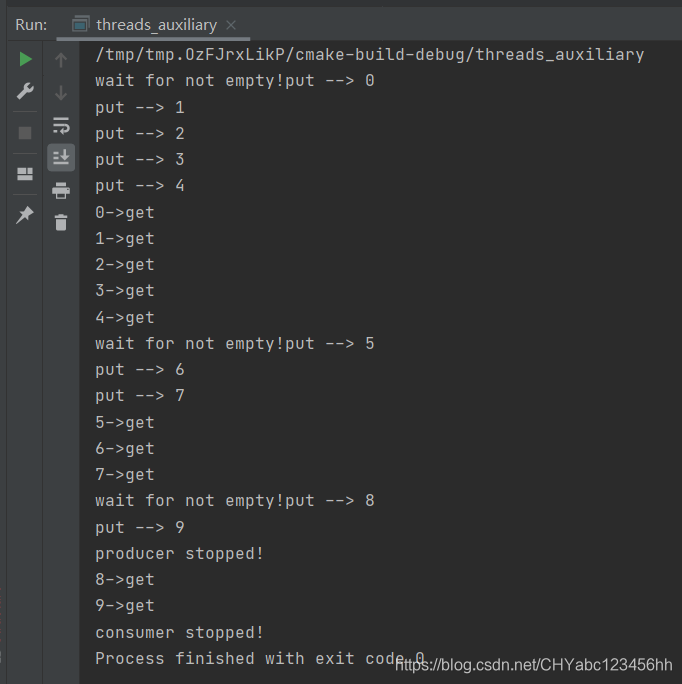
参考链接