Vue3
1.优点:
2.创建:
3.文件:
换运行插件:
4.运行:
setup函数:
setup函数中获取不到this(this 在定义的时候是Undefined)
reactive()和ref():
代码:
<script setup>
// reactive :接收一个对象类型的数据。返回一个响应式的对象
import { reactive } from 'vue'
import { ref } from 'vue'
// 只有用 reactive() 包裹的对象才能去响应式改变,否则不能改变
// 倘若是简单类型的对象,我们用ref(),但是这个简单和复杂都可以接受,
// 本质是自动增加了外层对象,使之成为复杂类型,再借助reactive实现的响应式
// 注意点: 1.script中访问数据,需要通过 .value
// 2.template 中不用,因为自动加了.value
// 推荐 数据定义同一用ref()
const state = reactive({
count: 100
})
const stateTwo = ref(10)
const setCount = () => {
state.count++
}
const setCountRef = () => {
stateTwo.value++
}
</script>
<template>
<div>
<div>{{ state.count }}</div>
<div>{{ stateTwo.value }}</div>
<button @click="setCount">+1</button>
<button @click="setCountRef">+1</button>
</div>
</template>
<style></style>
computed:
有读和写两种方法:get 和 set ,但是我们一般用只读,改的话另外写函数
<script setup>
import { ref, computed } from 'vue'
const list = ref([1, 2, 3, 4, 5, 6, 7, 8, 9])
const computedList = computed(() => {
// 在这里拿值别忘了 .value
return list.value.filter(item => item > 5)
})
</script>
<template>
<div>
<div>计算后属性:{{ computedList }}</div>
</div>
</template>
<style></style>
watch函数:
侦听不要加value,我们侦听对象,不是单个数字
immediate:
deep:
代码:
<script setup>
import { ref, watch } from 'vue'
const count = ref(0)
const nickname = ref('张三')
const changeName = () => {
nickname.value = '李四'
}
const changeCount = () => {
count.value++
}
// 1.单个对象 这个新值老值是函数固定好了的,不是我们想改就能改的
// watch( count,(newValue,oldValue) => {
// console.log( newValue,oldValue)
// })
// 2.多个对象
// watch([count,nickname], (newArr,oldArr) =>{
// console.log(newArr,oldArr)
// })
// 3.immediate: 一进页面就执行,从空数组变为有值的数组
// watch([count, nickname], (newArr,oldArr) => {
// console.log(newArr,oldArr)
// },{
// immediate: true
// })
// 4.deep 深度监视默认 watch进行的是浅层监视
// 1.const ref1 = ref(简单类型)可以直接监视
// 2.const ref2 = ref(复杂类型)监视不到复杂类型内部数据的变化
const userInfo = ref({
name: '张三',
age: 18
})
const setUserInfo = () => {
userInfo.value.age++
}
watch(userInfo, (newValue) => {
console.log(newValue)
}, {
immediate: true,
// 不加deep 监视不到对象
deep: true
})
</script>
<template>
<div>
<div>{{ count }}</div>
<button @click="changeCount">数字</button>
<div>{{ nickname }}</div>
<button @click="changeName">昵称</button>
<div>{{ userInfo }}</div>
<button @click="setUserInfo">年龄+1</button>
</div>
</template>
<style></style>
精准监听:
代码:
// 5.对于对象中的属性,进行精准监听,不确定加没加imediate函数
watch(() => userInfo.value.age,(newValue, oldValue) => {
console.log(oldValue, newValue)
})
5.周期函数:
代码:
<script setup>
import { onMounted } from 'vue';
const getList = () => {
setTimeout(() => {
console.log('发送请求获取数据')
},2000)
}
getList()
// 如果有写代码需要在mounted生命周期中执行
onMounted(() => {
console.log('mounted生命周期,可以提供多个')
})
</script>
<template>
<div></div>
</template>
<style></style>
6.父子通信
代码:
<script setup>
import { ref, onMounted } from 'vue'
import sonCom from '@/components/sonCom.vue'
const money = ref(101)
const getMoney = () => {
money.value = money.value + 10
}
const getList = () => {
setTimeout(() => {
console.log('发送请求获取数据')
},2000)
}
const changeFn = (use) => {
money.value = money.value - use
}
getList()
// 如果有写代码需要在mounted生命周期中执行
onMounted(() => {
console.log('mounted生命周期,可以提供多个')
})
</script>
<template>
<div>
<sonCom car = '宝马车'
:money="money"
@changeMoney = 'changeFn'>
</sonCom>
</div>
<button @click="getMoney">挣钱</button>
</template>
<style></style>
<script setup>
// 子组件
// 在这里接收父组件的方式需要借助 '编译器宏'函数,感觉还是以对象的形式封装
const props = defineProps({
car: String,
money: Number
})
console.log(props.car)
console.log(props.money)
const emit = defineEmits(['changeMoney'])
const delMoney = () => {
// 需要emit触发事件
emit('changeMoney',10)
}
</script>
<template>
<!-- 对于props传递来的数据,模板中可以直接使用,不用去接收.动态传值也一样 -->
<div>{{ car }}--{{ money }}</div>
<div class='son'>我是子组件</div>
<button @click='delMoney'>花钱</button>
</template>
<style>
.son {
height: 300px;
width: 300px;
border: 2px;
background-color: greenyellow;
}
</style>
7.模板引用:
通过ref标识获取真实的dom对象或者组件实例对象
代码:
<script setup>
// 模板引用
// 1.调用函数,生成ref对象
// 2.通过ref标识,进行绑定
// 3.通过ref对象.value即可访问到绑定的元素
import {onMounted, ref} from 'vue'
const inp = ref(null)
onMounted(() =>{
// console.log(inp.value.focus())
// inp.value.focus()
})
const clickFn = () => {
inp.value.focus()
}
</script>
<template>
<div>
<input ref="inp" type = 'text'>
<button @click="clickFn">点击输入框聚焦</button>
</div>
</template>
<style></style>
8.provide 和inject
10.defineOptions:
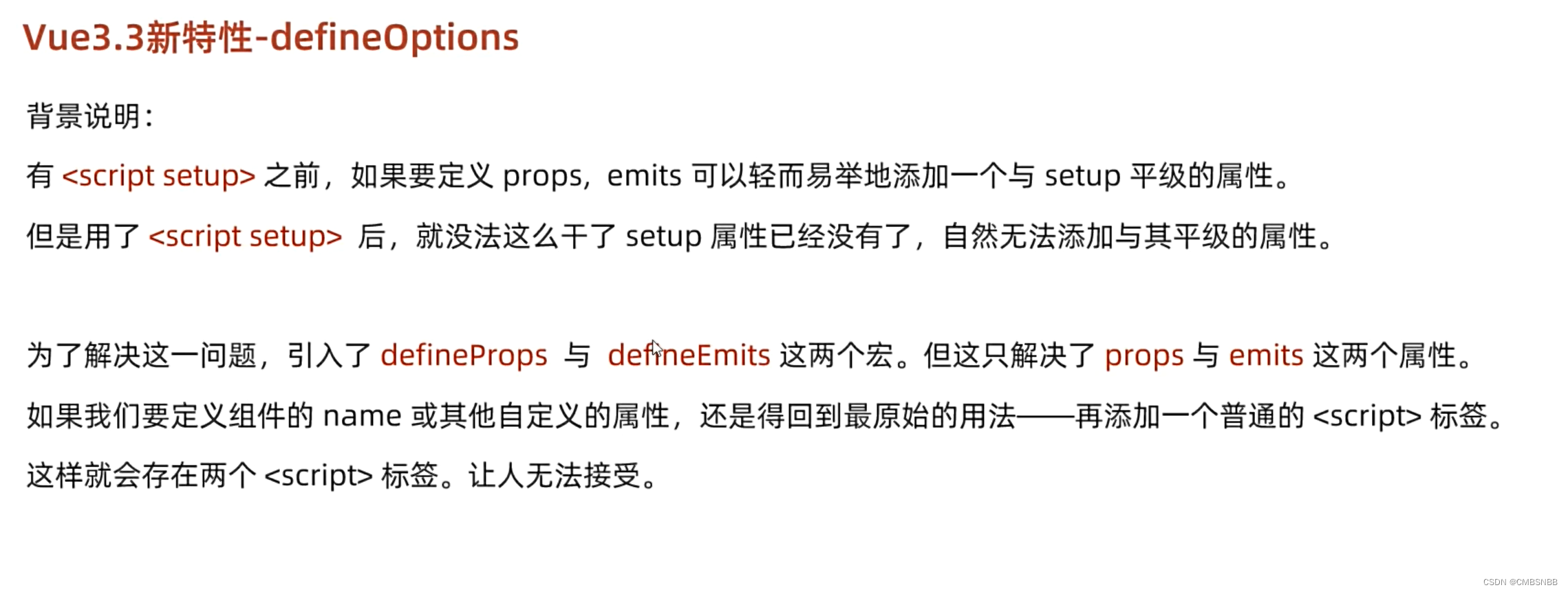
11. v-model 和 defineModel:
需要有如下配置: