文章中对输入输出流的用法介绍都在实例的注释中,请耐心通过实例来学习
一、文件字节流
1、字节输入流介绍
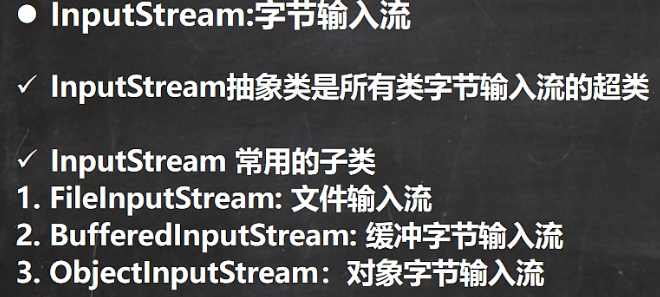
常见子类的继承关系
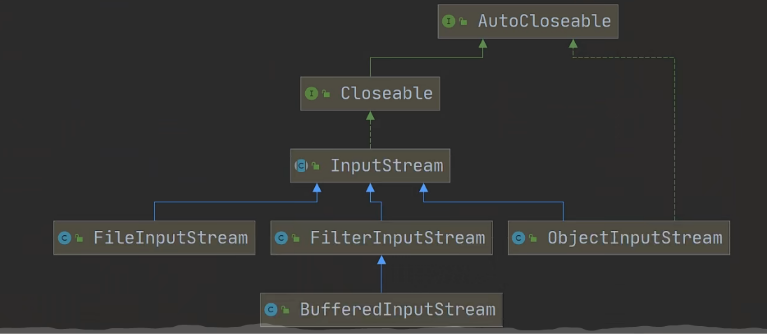
2、字节输出流介绍
继承关系图
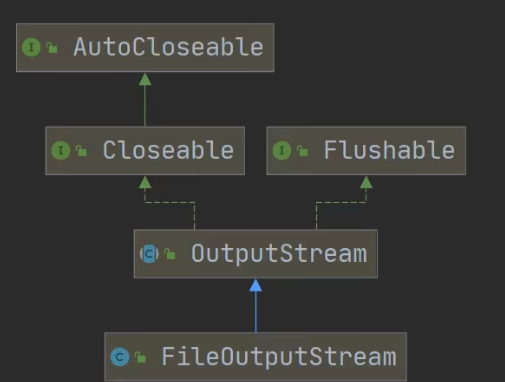
(一)fileInputStream文件字节输入流
1、方法一:单个字节的读取
//方法一:单个字节的读取read()
public void readFile01() {
String pathName = "E:\\hello.txt";
//定义一个readData接收读取到的字节
int readData = 0;
//后面的finally中想要调用这个对象,所以把这个对象名定义到try上面,可以让try和finally都能用
FileInputStream fileInputStream = null;
//创建IO 流对象
try {
//创建FileInputStream对象,用于读取文件中的内容
fileInputStream = new FileInputStream(pathName);
//read方法,是获取这个文件内的内容,返回值类型为int 如果返回的是 -1,那么证明读取结束
while ((readData = fileInputStream.read()) != -1){
//因为读取的数据类型是int,所以我需要把他强制转换成char类型
System.out.print((char)readData);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//流相当于一种资源,当我们用完之后一定记得关闭,关闭文件流,释放资源
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
2、方法二:多个字节的读取
//方法二:多个字节的读取,使用read(byte[] b)方法,定义一个字节数组,从该输入流中读取最多b.length字节的数组到字节数组
//如果文件到达尾部,也会返回一个-1
public void readFile02() {
String pathName = "E:\\hello.txt";
//定义一个字节类型的数组,一次读取8个字节
byte[] b = new byte[8];
//定义一个变量,接收read(byte[] b)返回的数组实际长度
int readLength = 0;
//后面的finally中想要调用这个对象,所以把这个对象名定义到try上面,可以让try和finally都能用
FileInputStream fileInputStream = null;
//创建IO 流对象
try {
//创建FileInputStream对象,用于读取文件中的内容
fileInputStream = new FileInputStream(pathName);
//read方法,是获取这个文件内的内容,返回值类型为int 如果返回的是 -1,那么证明读取结束
while ((readLength = fileInputStream.read(b)) != -1){
//把byte的字节数组转化成字符串,用new String方法,b表示字节数组名,0表示从0索引开始,最后一个表示字节数组的转换长度
System.out.print(new String(b,0,readLength));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//流相当于一种资源,当我们用完之后一定记得关闭,关闭文件流,释放资源
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
(二)、fileOutputStream文件字节输出流
实例是利用输入输出流完成文件的拷贝
package com.guohui.outputstream_;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class FileCopy {
public void copy() {
//首先先将图片输入到程序中
String filePathName = "E:\\weixin.png";
//创建接收的文件
String endPathName = "D:\\weixinnew.png";
//创建一个输入流对象
FileInputStream fileInputStream = null;
//创建一个输出流对象
FileOutputStream fileOutputStream = null;
//创建一个读取的字节数组,便于后续按照字节数组的长度快速读取文件中的数据
byte[] b = new byte[1024];
//创建个变量接收读取到的字节
int readLen = 0;
try {
fileInputStream = new FileInputStream(filePathName);
fileOutputStream = new FileOutputStream(endPathName);
while ((readLen = fileInputStream.read(b)) != -1) {
//读取到文件后,用fileOutputStream将文件内容写入到指定的文件中
//避免文件过大,读取慢,一般采用边读边写的方式
fileOutputStream.write(b, 0, readLen);
}
System.out.println("文件拷贝成功!");
} catch (IOException e) {
e.printStackTrace();
} finally {
//为减少资源的浪费,用完输入和输出流后要及时关闭,释放资源
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
fileOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
二、文件字符流
(一)FileReader(字符输入流)用法
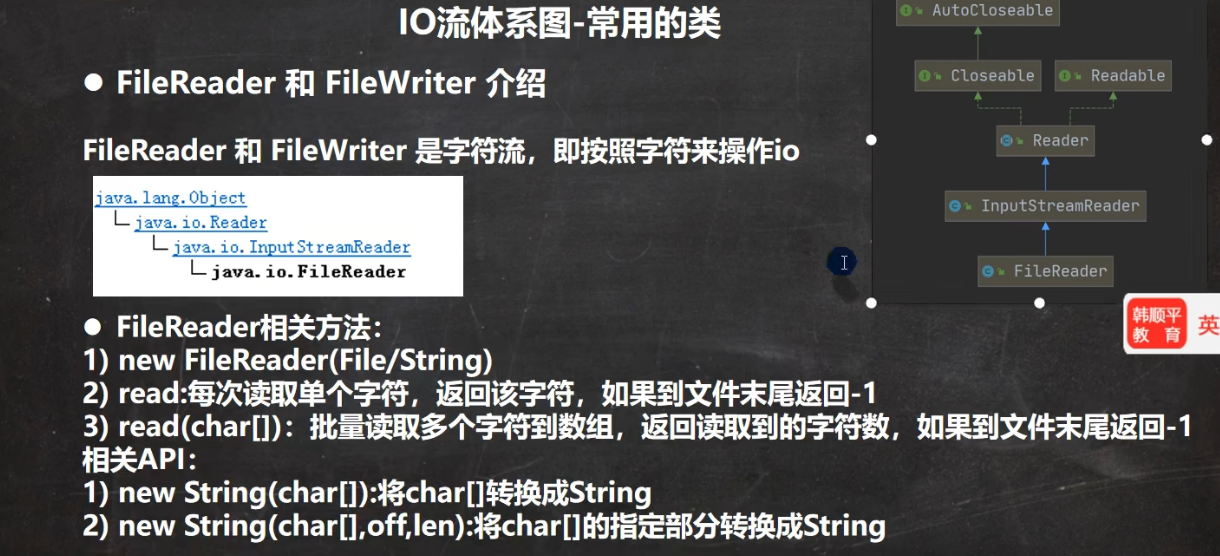
有两种读取输入的方法:
1、单个字符读取
//第一种方案,单个字符读取
public void fileReader1() {
//定义变量接收需要读取文件的路径
String pathName = "E:\\story.txt";
//定义一个变量接收读取到的值
int data = 0;
java.io.FileReader fileReader = null;
try {
fileReader = new java.io.FileReader(pathName);
while ((data = fileReader.read())!= -1){
System.out.print((char)data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
2、定义数组来读取,提高读取的效率
//第二种方案,字符数组来读取文件
public void readeFile2(){
//定义变量接收需要读取文件的路径
String pathName = "E:\\story.txt";
//定义一个字符数组
char[] buf = new char[20];
int readerLen = 0;
//抽取Filereader对象名
java.io.FileReader fileReader = null;
try {
java.io.FileReader reader = new java.io.FileReader(pathName);
while ((readerLen = reader.read(buf)) != -1){
System.out.print(new String(buf,0,readerLen));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
//这个地方在关闭字符读取流之前,加了一个非空的判断,就是为了避免在创建字符读取流的时候,报空指针异常
if (fileReader != null){
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
(二)、FileWriter(字符输出流)用法
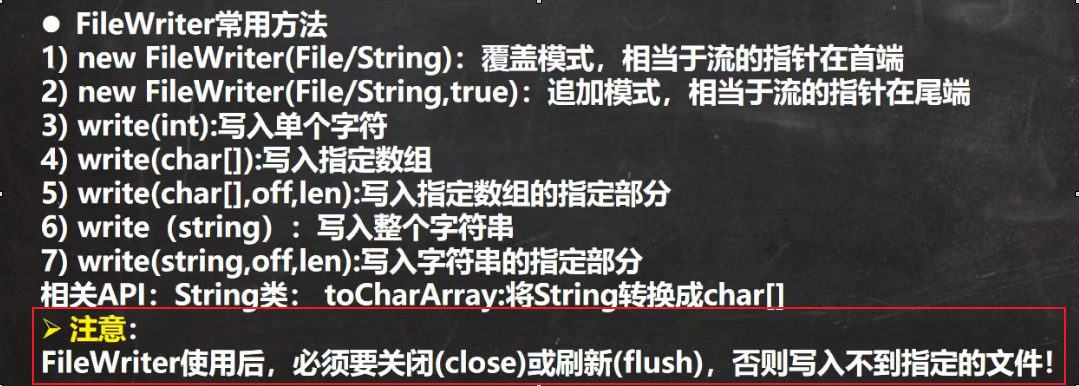
以一个样例来介绍字符输出流
需求:

有5中方法:
package com.guohui.writer_;
import java.io.FileWriter;
import java.io.IOException;
public class Writer {
public void writerFile(){
//定义想要输出的文件
String pathName = "e:\\note.txt";
FileWriter fileWriter = null;
//方法2中需要定义的数组
char[] chars = {'a','b','c'};
try {
fileWriter = new FileWriter(pathName,true);
//方法1: 写入单个字符,用write(int c)方法
fileWriter.write('H');
//方法2: 写入指定的数组,用write(char[])
fileWriter.write(chars);
//方法3: 写入指定数组的指定部分,用write(char[],off,len)方法
fileWriter.write("学习编程".toCharArray(),0,2);
//方法4: 写入整个字符串,用writr(String)方法
fileWriter.write("风雨之后,定见彩虹");
//方法5: 写入字符串的指定部分
fileWriter.write("你好北京 北京欢迎你",0,4);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {if (fileWriter != null){
fileWriter.flush();
}
} catch (IOException e) {
e.printStackTrace();
}
try {
fileWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}