Today Patrick waits for a visit from his friend Spongebob. To prepare for the visit, Patrick needs to buy some goodies in two stores located near his house. There is a d1 meter long road between his house and the first shop and a d2 meter long road between his house and the second shop. Also, there is a road of length d3 directly connecting these two shops to each other. Help Patrick calculate the minimum distance that he needs to walk in order to go to both shops and return to his house.
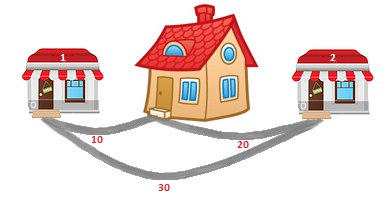
Patrick always starts at his house. He should visit both shops moving only along the three existing roads and return back to his house. He doesn't mind visiting the same shop or passing the same road multiple times. The only goal is to minimize the total distance traveled.
The first line of the input contains three integers d1, d2, d3 (1 ≤ d1, d2, d3 ≤ 108) — the lengths of the paths.
- d1 is the length of the path connecting Patrick's house and the first shop;
- d2 is the length of the path connecting Patrick's house and the second shop;
- d3 is the length of the path connecting both shops.
Print the minimum distance that Patrick will have to walk in order to visit both shops and return to his house.
10 20 30
60
1 1 5
4
The first sample is shown on the picture in the problem statement. One of the optimal routes is: house first shop
second shop
house.
In the second sample one of the optimal routes is: house first shop
house
second shop
house.
讨论:
d1+d2+d3
d1*2+d2*2
d1*2+d3*2
d2*2+d3*2
取最小值即可
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cmath>
using namespace std;
int d1,d2,d3;
int main()
{
#ifdef YZY
freopen("yzy.txt","r",stdin);
#endif
cin >> d1 >> d2 >> d3;
int ans = d1 + d2 + d3;
ans = min(ans,d1*2+d2*2);
ans = min(ans,d1*2+d3*2);
ans = min(ans,d2*2+d3*2);
cout << ans;
return 0;
}
While Patrick was gone shopping, Spongebob decided to play a little trick on his friend. The naughty Sponge browsed through Patrick's personal stuff and found a sequence a1, a2, ..., am of length m, consisting of integers from 1 to n, not necessarily distinct. Then he picked some sequence f1, f2, ..., fn of length n and for each number ai got number bi = fai. To finish the prank he erased the initial sequence ai.
It's hard to express how sad Patrick was when he returned home from shopping! We will just say that Spongebob immediately got really sorry about what he has done and he is now trying to restore the original sequence. Help him do this or determine that this is impossible.
The first line of the input contains two integers n and m (1 ≤ n, m ≤ 100 000) — the lengths of sequences fi and bi respectively.
The second line contains n integers, determining sequence f1, f2, ..., fn (1 ≤ fi ≤ n).
The last line contains m integers, determining sequence b1, b2, ..., bm (1 ≤ bi ≤ n).
Print "Possible" if there is exactly one sequence ai, such that bi = fai for all i from 1 to m. Then print m integers a1, a2, ..., am.
If there are multiple suitable sequences ai, print "Ambiguity".
If Spongebob has made a mistake in his calculations and no suitable sequence ai exists, print "Impossible".
3 3 3 2 1 1 2 3
Possible 3 2 1
3 3 1 1 1 1 1 1
Ambiguity
3 3 1 2 1 3 3 3
Impossible
In the first sample 3 is replaced by 1 and vice versa, while 2 never changes. The answer exists and is unique.
In the second sample all numbers are replaced by 1, so it is impossible to unambiguously restore the original sequence.
In the third sample fi ≠ 3 for all i, so no sequence ai transforms into such bi and we can say for sure that Spongebob has made a mistake.
分几类讨论就是:
若对于任意bi,f中有且仅有一个与之对应 有唯一解
若对于某bi,f中有多个对应 有多解
若对于某bi,f中无解 Impossible!
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cmath>
#include<vector>
using namespace std;
const int maxn = 1e5 + 10;
int a[maxn],n,m,i,j;
vector <int> pos[maxn];
int main()
{
#ifdef YZY
freopen("yzy.txt","r",stdin);
#endif
cin >> n >> m;
for (i = 1; i <= n; i++) {
int x; scanf("%d",&x);
pos[x].push_back(i);
}
bool flag = 0;
for (i = 1; i <= m; i++) {
int x; scanf("%d",&x);
if (!pos[x].size()) {
printf("Impossible");
return 0;
}
if (pos[x].size() > 1) {
flag = 1;
continue;
}
a[i] = pos[x][0];
}
if (flag) {
printf("Ambiguity");
return 0;
}
printf("Possible\n");
for (i = 1; i <= m; i++) printf("%d ",a[i]);
return 0;
}
One day Squidward, Spongebob and Patrick decided to go to the beach. Unfortunately, the weather was bad, so the friends were unable to ride waves. However, they decided to spent their time building sand castles.
At the end of the day there were n castles built by friends. Castles are numbered from 1 to n, and the height of the i-th castle is equal tohi. When friends were about to leave, Squidward noticed, that castles are not ordered by their height, and this looks ugly. Now friends are going to reorder the castles in a way to obtain that condition hi ≤ hi + 1 holds for all i from 1 to n - 1.
Squidward suggested the following process of sorting castles:
- Castles are split into blocks — groups of consecutive castles. Therefore the block from i to j will include castles i, i + 1, ..., j. A block may consist of a single castle.
- The partitioning is chosen in such a way that every castle is a part of exactly one block.
- Each block is sorted independently from other blocks, that is the sequence hi, hi + 1, ..., hj becomes sorted.
- The partitioning should satisfy the condition that after each block is sorted, the sequence hi becomes sorted too. This may always be achieved by saying that the whole sequence is a single block.
Even Patrick understands that increasing the number of blocks in partitioning will ease the sorting process. Now friends ask you to count the maximum possible number of blocks in a partitioning that satisfies all the above requirements.
The first line of the input contains a single integer n (1 ≤ n ≤ 100 000) — the number of castles Spongebob, Patrick and Squidward made from sand during the day.
The next line contains n integers hi (1 ≤ hi ≤ 109). The i-th of these integers corresponds to the height of the i-th castle.
Print the maximum possible number of blocks in a valid partitioning.
3 1 2 3
3
4 2 1 3 2
2
In the first sample the partitioning looks like that: [1][2][3].
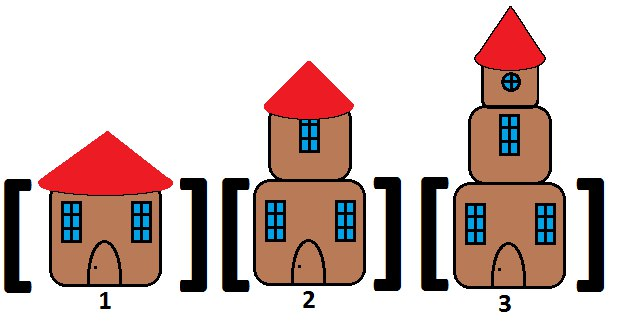
In the second sample the partitioning is: [2, 1][3, 2]
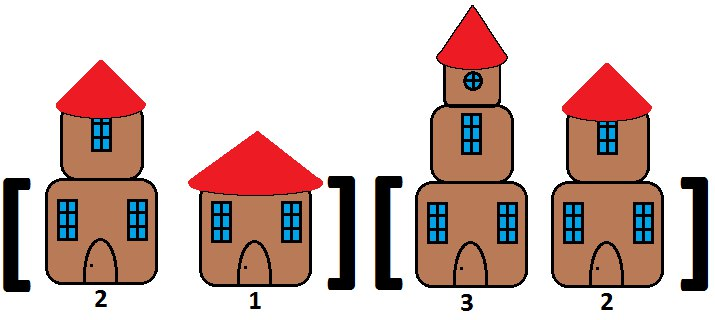
既然要分最多block 那么显然每块尽量小的好
预处理Min[i] = min{hj | i <= j <= n}
不难证明只要当前最高值<=Min[i]就可以分块
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cmath>
using namespace std;
const int maxn = 1e5 + 10;
int Min[maxn],h[maxn],n,i,j,ans = 1;
int main()
{
#ifdef YZY
freopen("yzy.txt","r",stdin);
#endif
cin >> n;
for (i = 1; i <= n; i++) scanf("%d",&h[i]);
Min[n] = h[n];
for (i = n-1; i > 0; i--) {
Min[i] = h[i]; Min[i] = min(Min[i+1],Min[i]);
}
int H = h[1];
for (i = 1; i <= n; i++) {
H = max(H,h[i]);
if (Min[i+1] >= H) {
++ans; continue;
}
}
cout << ans;
return 0;
}
Spongebob is already tired trying to reason his weird actions and calculations, so he simply asked you to find all pairs of n and m, such that there are exactly x distinct squares in the table consisting of n rows and m columns. For example, in a 3 × 5 table there are 15squares with side one, 8 squares with side two and 3 squares with side three. The total number of distinct squares in a 3 × 5 table is15 + 8 + 3 = 26.
The first line of the input contains a single integer x (1 ≤ x ≤ 1018) — the number of squares inside the tables Spongebob is interested in.
First print a single integer k — the number of tables with exactly x distinct squares inside.
Then print k pairs of integers describing the tables. Print the pairs in the order of increasing n, and in case of equality — in the order of increasing m.
26
6 1 26 2 9 3 5 5 3 9 2 26 1
2
2 1 2 2 1
8
4 1 8 2 3 3 2 8 1
In a 1 × 2 table there are 2 1 × 1 squares. So, 2 distinct squares in total.
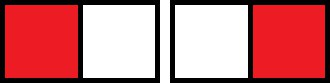
In a 2 × 3 table there are 6 1 × 1 squares and 2 2 × 2 squares. That is equal to 8 squares in total.
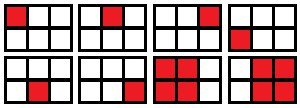
数学题。。。
设n<m
6x=n*(n+1)*(3m+1-n)
用平方和公式推导。。
于是枚举n求出m
注意考虑n=m时只能算一次呀。。
#include<iostream>
#include<cstdio>
#include<algorithm>
#include<cmath>
using namespace std;
typedef long long LL;
struct ANS{
LL m,n;
bool operator < (const ANS &b) const {
return m < b.m;
}
}A[5000000];
int ans = 0,j;
LL x,i;
int main()
{
#ifdef YZY
freopen("yzy.txt","r",stdin);
#endif
cin >> x; x *= 6;
for (i = 1;i <= 5000000; i++)
if (x % (i*(i+1)) == 0) {
LL y = x / (i*(i+1));
y += i-1;
if (y <= 0) break;
if (y / 3 < i) break;
if (y % 3 == 0) {
y /= 3;
//if (y <= i) break;
++ans;
A[ans].m = i;
A[ans].n = y;
if (i != y) {
++ans;
A[ans].m = y;
A[ans].n = i;
}
}
}
//for (j = 1; j <= ans; j++) A[j+ans].m = A[j].n,A[j+ans].n = A[j].m;
sort(A + 1,A + ans + 1);
printf("%d\n",ans);
for (i = 1; i <= ans; i++)
printf("%I64d %I64d\n",A[i].m,A[i].n);
return 0;
}