# pascal命名法
# 显示&隐式 object
class Person:
# 构造函数: 为对象分配存储空间
# class:cls-->当前类Person
def __new__(cls, *args, **kwargs):
print("__new__")
return super().__new__(cls)
# 初始化
def __init__(self):
print("__init__")
# 析构函数:对象在释放资源之前,所调用的最后一个函数
def __del__(self):
print("__del__")
if __name__ == '__main__':
person1 = Person()
person2 = Person()
class Apple:
new_name = "iphone 15"
def display_name(self):
# self: 该类(Apple)的对象,调用该类(Apple)的根目录资源
name = self.get_name()
print(f"Apple下根目录对应的变量{self.new_name}")
print(f"Apple下对应的函数{name}")
def get_name(self):
i = 1
return "kuke's iphone 14"
apple1 = Apple()
apple1.display_name()
class Apple:
name = "Tim"
I = [1, 2, 3]
a = Apple()
print(id(Apple.name) == id(a.name))
print(id(Apple.I) == id(a.I))
print(a.name, Apple.name)
a.name += "kuke"
a.I += [5, 4, 4]
print(id(Apple.name) == id(a.name))
print(id(Apple.I) == id(a.I))
print(a.name, Apple.name)
class HW:
# 类属性
name = "mate"
@classmethod
def get_name(cls):
print(cls.name)
print(HW.name)
HW.get_name()
class Student:
def __init__(self, name="hl", num=1914220):
self.name = name
self.num = num
s1 = Student()
print(s1.name, s1.num)
# 公有,私有(不存在真正私有,只是将内部变量名称转换‘"_你所要用的类名称"+"你所要调用的私有函数名称"’)
class Private:
__name = "hl"
def __func(self):
print("private func")
if __name__ == '__main__':
print(dir(Private))
Private()._Private__func()
print(Private()._Private__name)
class Static:
@staticmethod
def static_display(cls):
print("static")
@classmethod
def class_display(cls):
print("class")
Static().class_display()
Static().static_display(Static)
class Student:
def __init__(self, name):
self.name = name
def __call__(self, *args, **kwargs):
self.display()
def display(self):
print(f"你好{self.name}")
Student("Andy")()
import random
class Student:
def __init__(self, name, sex, age, tel, score):
self.name = name
self.age = age
self.tel = tel
self.score = score
self.sex = sex
def get_student(self):
print(f"name: {self.name}, sex: {self.sex}, age: {self.age}, tel: {self.tel}, score: {self.score}")
# List of random names and sexes
names = ["Alice", "Bob", "Charlie", "David", "Eva", "Frank", "Grace", "Henry", "Isabella", "Jack"]
sexes = ["男", "女"]
# List to store the generated students
students = []
# Generate 100 random students
for _ in range(100):
name = random.choice(names)
sex = random.choice(sexes)
age = random.randint(18, 25) # Random age between 18 and 25
tel = random.randint(10000000000, 19999999999) # Random 11-digit telephone number
score = random.randint(60, 100) # Random score between 60 and 100
student = Student(name, sex, age, tel, score)
students.append(student)
# Print the information of all students
for student in students:
student.get_student()
'''class Student:
def __init__(self, name, sex, age, tel, score):
self.name = name
self.age = age
self.tel = tel
self.score = score
self.sex = sex
def get_student(self):
print(f"name: {self.name}, sex: {self.sex}, age: {self.age}, tel: {self.tel}, score: {self.score}")
students=list()
for i in range (10):
students = [
Student("q", "男", 10, 666, 96),
Student("w", "女", 13, 999, 90),
Student("e", "男", 16, 966, 97),
Student("r", "男", 26, 996, 89),
Student("t", "男", 12, 696, 89),
Student("y", "女", 15, 886, 98),
Student("u", "男", 13, 688, 68),
Student("i", "女", 25, 866, 87),
Student("o", "女", 18, 686, 77),
Student("p", "男", 9, 868, 79)
]
for student in students:
student.get_student()
'''
class Book:
def __init__(self, name, isbn, price, author):
self.name = name
self.isbn = isbn
self.price = price
self.author = author
def get_book(self):
print(f"name:{self.name},isbn:{self.isbn},price:{self.price},author:{self.author}")
book_List = [Book("西由记", 123, 22, "wuser"),
Book("红喽梦", 223, 23, "caoser"),
Book("三国演绎", 334, 24, "luoser"),
Book("水壶传", 445, 25, "shige")
]
for book in book_List:
book.get_book()
book_List.append(Book("聊斋志异", "00000005", 198, "蒲松龄"))
index_to_remove = None
for i, book in enumerate(book_List):
if book.name == "西由记" and book.isbn == 123 and book.price == 22 and book.author == "wuser":
index_to_remove = i
break
if index_to_remove is not None:
del book_List[index_to_remove]
else:
print("要删除的书籍不存在于列表中。")
print("-------------")
for book in book_List:
book.get_book()
'''class A:
def __init__(self, name):
print(f"A.{name}")
class B(A):
def __init__(self, name):
super().__init__(name)
print(f"B.{name}")
b=B("hl")
'''
class A:
def test(self):
print("A.test()")
class B(A):
def test(self):
print("B.test()")
b = B()
b.test()
super(B,b).test()
print("__new__")
AI的系统学习之路 2.python类class的应用
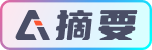