基本指令使用
将第一个 item 显示为红色,其余点击变红
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>HelloVue</title>
<script src="js/vue.js"></script>
<style>
.active {
color: red;
}
</style>
</head>
<body>
<div id="app">
<ul>
<li v-for="(item,index) in movies"
:class="{active:currentIndex === index}"
@click="itemClick(index)">{{item}}
</li>
</ul>
</div>
</body>
<script>
const vm = new Vue({
el: '#app',
data: {
movies: ['柯南', '海贼王', '火影忍者'],
currentIndex: 0
},
methods: {
itemClick(index){
this.currentIndex = index
}
}
})
</script>
</html>
购物车案例
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>HelloVue</title>
<style>
table {
border: 1px solid darkgreen;
border-collapse: collapse;
border-spacing: 0;
}
th,td{
padding: 8px 16px;
border: 2px solid slategray;
text-align: center;
}
th {
background-color: #d0e9c6;
color: #204d74;
font-weight: 600;
}
</style>
</head>
<body>
<div id="app">
<div v-if="movies.length">
<table>
<thead>
<th></th>
<th>名称</th>
<th>日期</th>
<th>票价</th>
<th>数量</th>
<th>操作</th>
</thead>
<tbody>
<tr v-for="(item,index) in movies">
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{{item.date}}</td>
<td>{{item.price | showPrice}}</td>
<td>
<button @click="decrement(index)" :disabled="item.count == 1">-</button>
{{item.count}}
<button @click="increment(index)">+</button>
</td>
<td>
<button @click="removeHandle()">移除</button>
</td>
</tr>
</tbody>
</table>
<h2>总价格:{{totalPrice | showPrice}}</h2>
</div>
<h2 v-else>购物车为空</h2>
</div>
<script src="../js/vue.js"></script>
<script src="../shoppingCart/main.js"></script>
</body>
<script>
const app = new Vue({
el: '#app',
data: {
movies: [
{
id: 1,
name: '柯南',
date: '2019-10-1',
price: 85.00,
count: 1
},
{
id: 2,
name: '海贼王',
date: '2019-10-3',
price: 64.00,
count: 1
},
{
id: 3,
name: '火影忍者',
date: '2019-9-15',
price: 82.00,
count: 1
},
{
id: 4,
name: '死神',
date: '2019-8-10',
price: 66.00,
count: 1
},
]
},
methods: {
increment(index) {
this.movies[index].count++
},
decrement(index) {
this.movies[index].count--
},
removeHandle(index) {
this.movies.splice(index, 1)
}
},
computed: {
totalPrice() {
let result = 0
for(let item of this.movies){
result += item.count * item.price
}
return result
}
},
filters: {
showPrice(price) {
//保留两位小数
return '¥' + price.toFixed(2)
}
}
})
</script>
</html>
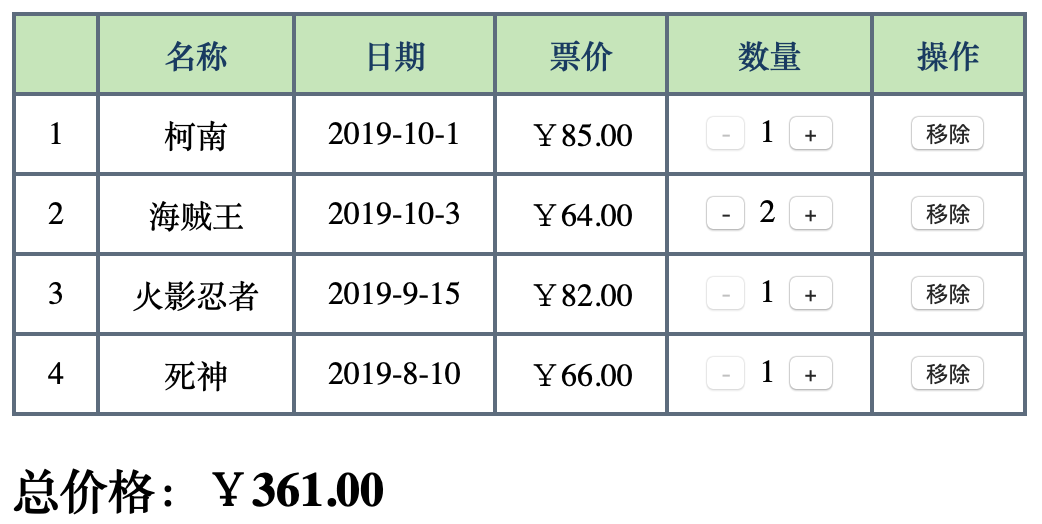
参考视频:小码哥 Vue 视频教程