Fortran 中的函数与子程序
简介
- Fortran 是不区分大小写的
- 函数(Function):
- 函数是一段具有输入和输出的代码块,它接受一些输入参数,经过一系列计算后返回一个结果。
- 在Fortran中,函数的定义以关键字"FUNCTION"开始,以"end function"结束
- Fortran 90中的函数必须在程序的`contains`块中定义,以便在主程序中调用
- 子程序(Subroutine):
- 子程序是一段独立的代码块,它可以被其他代码调用并执行。与函数不同的是,子程序可以不返回任何结果,或者通过参数列表传递结果。在Fortran中,子程序的定义以关键字"SUBROUTINE"开始,后面跟着子程序名和参数列表。子程序体内部可以包含一系列的计算语句,执行完后通过关键字"END SUBROUTINE"结束。
函数实例
exam1:不使用return语句
program function_example
implicit none
real :: length, width, area
write(*,*) "input length:"
read(*,*) length
write(*,*) "input width:"
read(*,*) width
area = calculate_area(length, width)
write(*,*) "the area of triangle", area
read(*,*)
contains
function calculate_area(length,width) result(area)
real, intent(in) :: length, width
real :: area
area = length * width
end function calculate_area
end program function_example
exam2:使用return语句
program return_example
implicit none
real :: num1, num2, average
write(*, *) "input two number:"
read(*, *) num1, num2
average = calculate_average(num1, num2)
write(*,*) "the average of the two numbers:", average
read(*,*)
contains
real function calculate_average(a, b)
real, intent(in) :: a, b
calculate_average = (a + b) / 2.0
return
end function calculate_average
end program return_example
子程序实例
exam1:Simple Subroutine
program main
implicit none
call print_message()
read(*,*)
end program main
subroutine print_message()
print *, "Hello, World!"
end subroutine print_message
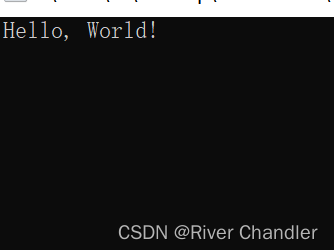
exam2:Subroutine with Arguments
program main
implicit none
real :: num1, num2, sum
num1 = 2.5
num2 = 3.7
call calculate_sum(num1, num2, sum)
print *, "The sum is:", sum
read(*,*)
end program main
subroutine calculate_sum(a, b, result)
real :: a, b, result
result = a + b
end subroutine calculate_sum
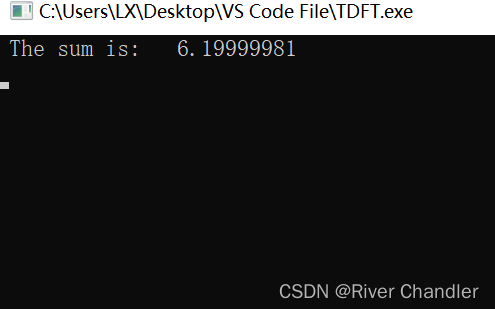
exam3:Subroutine with Intent
program main
implicit none
real :: numbers(5) = [1,2,3,4,5]
integer :: i
call square_array(numbers,5)
do i = 1, 5
print *, numbers(i)
end do
read(*,*)
end program main
subroutine square_array(arr, size)
integer, intent(in) :: size
real, intent(inout) :: arr(size)
integer :: i
do i = 1, size
arr(i) = arr(i) ** 2
end do
end subroutine square_array
program main
implicit none
real :: numbers(5) = [1,2,3,4,5]
integer :: i
integer :: j
j = size(numbers)
call square_array(numbers,j)
do i = 1, j
print *, numbers(i)
end do
read(*,*)
end program main
subroutine square_array(arr, size)
integer, intent(in) :: size
real, intent(inout) :: arr(size)
integer :: i
do i = 1, size
arr(i) = arr(i) ** 2
end do
end subroutine square_array
函数与子程序的发展史
- In Fortran, subroutines and functions are used to encapsulate a specific set of instructions that can be called and executed from different parts of a program.
- Subroutines are defined using the SUBROUTINE keyword followed by a name, and they do not return any values.
- They are typically used for performing a series of calculations or operations without returning a result. Subroutines can have input parameters, which are variables passed to the subroutine for use within its code.
- Functions, on the other hand, are defined using the FUNCTION keyword followed by a name. Functions are used to perform calculations and return a single value as the result.
- They can have input parameters, similar to subroutines, which are used in the calculation.
- Both subroutines and functions can be called from other parts of the program using their respective names.
- When calling a subroutine, the program flow jumps to the subroutine code, executes it, and then returns to the calling point.
- When calling a function, the result of the calculation is returned and can be assigned to a variable or used directly in an expression.
- 在C语言中,存在函数
- 在Java中,称为方法(method)
- 在Python中,也有函数(function)
- 通过区分function和subroutine,Fortran可以更好地满足不同的编程需求。这样的设计可以提高代码的可读性和可维护性,使得程序的逻辑更加清晰明确。
- Function适用于需要返回结果的计算任务
- Subroutine适用于需要执行一系列操作或修改参数值的任务。