京东抽奖项目开发笔记
前言:
这是一个独立项目,这个项目会由我们四个人来一起完成,所以首先就要分工合作,我是写前端的,所以我会先把基本的页面写出来
1.注册页
这是注册页的代码
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Document</title>
</head>
<link rel="stylesheet" href="css/login.css" />
<body>
<button class="message" data-icon="info"></button>
<div class="loginBox">
<h2>注册</h2>
<form action="" method="" class="codeForm">
<div class="item">
<input type="text" required id="phone" name="phone" />
<label for="">手机号</label>
</div>
<div class="item">
<input type="text" required id="code" name="code" />
<label for="">验证码</label>
<a href="" class="getCode">点击获取</a>
</div>
<button type="submit" class="btn">
下一步
<span></span>
<span></span>
<span></span>
<span></span>
</button>
</form>
</div>
</body>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/1.10.0/jquery.min.js"></script>
<script src="./js/modal_dialog.js"></script>
<script src="js/tccj.js"></script>
<script>
$(function () {
// 用户提交表单
$("form").on("submit", (e) => {
// 阻止默认行为
e.preventDefault();
// 判断手机号格式是否正确
var $phone = $("#phone").val();
if (!/^1[3456789]\d{9}$/.test($phone)) {
// 提示错误信息
$(".message").trigger("click");
return false;
}
});
});
</script>
</html>
CSS样式
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
input,
button {
background: transparent;
border: none;
outline: none;
}
body {
height: 100vh;
/* background: linear-gradient(#141e30, #243b55); */
background-image: linear-gradient(
60deg,
rgba(255, 165, 150, 0.5) 5%,
rgba(0, 228, 255, 0.35)
),
url(https://cdn.jsdelivr.net/gh/extheor/images/jdDraw%E5%9B%BE%E7%89%87/坚持.jpg);
display: flex;
justify-content: center;
align-items: center;
font-size: 16px;
color: #03e9f4;
}
.loginBox {
width: 470px;
height: 420px;
background: rgba(0, 0, 0, 0.5);
box-shadow: 0px 15px 25px 0 rgba(0, 0, 0, 0.6);
padding: 40px;
}
h2 {
text-align: center;
color: #fff;
margin-bottom: 30px;
font-weight: 800;
}
.loginWay {
width: 390px;
height: 30px;
line-height: 30px;
text-align: center;
font-size: 20px;
margin-bottom: 20px;
}
.loginWay > .codeLogin {
width: 36%;
height: 100%;
float: left;
cursor: pointer;
transition: 0.5s;
position: relative;
}
.loginWay > .codeLogin:hover {
font-size: 19px;
color: #cc9644;
}
.loginWay > .codeLogin > .line1 {
display: block;
width: 100%;
height: 2px;
background: #ecf0f1;
position: absolute;
left: 0;
bottom: -2px;
transition: all 1s cubic-bezier(0.175, 0.885, 0.32, 1.275) 0s;
}
.loginWay > .codeLogin:hover > .line1 {
background: rgb(157, 131, 82);
width: 30%;
}
.loginWay > .pwdLogin {
width: 31%;
height: 100%;
float: right;
cursor: pointer;
transition: 0.5s;
position: relative;
}
.loginWay > .pwdLogin:hover {
font-size: 19px;
color: #cc9644;
}
.loginWay > .pwdLogin > .line2 {
display: block;
width: 0;
height: 2px;
background: #ecf0f1;
position: absolute;
left: 0;
bottom: -2px;
transition: all 1s cubic-bezier(0.175, 0.885, 0.32, 1.275) 0s;
}
.loginWay > .pwdLogin:hover > .line2 {
background: rgb(157, 131, 82);
width: 30%;
}
.pwdForm {
display: none;
}
.item {
height: 45px;
border-bottom: 1px solid #fff;
margin-bottom: 40px;
position: relative;
}
.item input {
width: 100%;
height: 100%;
color: #fff;
padding-top: 20px;
}
.item > .getCode {
width: 70px;
height: 30px;
line-height: 30px;
text-align: center;
float: right;
position: relative;
left: 0;
top: -36px;
cursor: pointer;
text-decoration: none;
color: #ecf0f1;
}
.item > .getCode:hover {
color: aqua;
}
.item input:focus + label,
.item input:valid + label {
color: #fff;
font-size: 12px;
top: 0;
}
.item label {
position: absolute;
left: 0;
top: 12px;
transition: all 0.5s;
}
.btn {
padding: 10px 20px;
margin-top: 30px;
color: #03e9f4;
text-transform: uppercase;
letter-spacing: 2px;
position: relative;
overflow: hidden;
transition: all 0.2s;
}
.btn:hover {
background: #03e9f4;
border-radius: 5px;
color: #fff;
box-shadow: 0 0 5px 0 #03e9f4, 0 0 25px 0 #03e9f4, 0 0 50px 0 #03e9f4,
0 0 100px 0 #03e9f4;
}
.btn > span {
position: absolute;
}
.btn > span:nth-child(1) {
width: 100%;
height: 2px;
background: -webkit-linear-gradient(left, transparent, #03e9f4);
left: -100%;
top: 0;
animation: line1 1s infinite;
}
@keyframes rotate {
0% {
transform: rotateY(0deg);
width: 390px;
}
50% {
transform: rotateY(180deg);
width: 370px;
}
100% {
transform: rotateY(0deg);
width: 390px;
}
}
@keyframes line1 {
50%,
100% {
left: 100%;
}
}
.btn > span:nth-child(2) {
width: 2px;
height: 100%;
background: -webkit-linear-gradient(top, transparent, #03e9f4);
right: 0%;
top: -100%;
animation: line2 1s 0.25s infinite;
}
@keyframes line2 {
50%,
100% {
top: 100%;
}
}
.btn > span:nth-child(3) {
width: 100%;
height: 2px;
background: -webkit-linear-gradient(right, transparent, #03e9f4);
right: -100%;
bottom: 0;
animation: line3 1s 0.5s infinite;
}
@keyframes line3 {
50%,
100% {
right: 100%;
}
}
.btn > span:nth-child(4) {
width: 2px;
height: 100%;
background: -webkit-linear-gradient(bottom, transparent, #03e9f4);
left: 0%;
bottom: -100%;
animation: line4 1s 0.75s infinite;
}
@keyframes line4 {
50%,
100% {
bottom: 100%;
}
}
上面的代码是写的注册页的基本页面,CSS部分写的比较多,所以在写页面上花了很大一部分时间,js中还判断了手机号的格式是否正确,如果不正确会提示“手机号格式有误”(这个错误提示我用了插件,我会在下面给出相应的代码),如果输入的手机号正确,那么会进行Ajax请求(前端向后端发送用户所输入的数据,比如说:手机号和验证码),验证码的话是通过第三方API来获取的,这当然也必须是用Ajax发送请求,来获取验证码,这时就可以发送给后端了,后端会接收到数据,然后把该注册信息插入到数据库中进行存储,为以后的登录操作打下了良好的基础
-
jQuery弹窗库
// 封装模态框_提示框 // 提示框 // 图标 base64 码 全局变量 // 默认图标 var info_modal = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgCAYAAABzenr0AAADlUlEQVRYR82XS4hbdRjFz7l3ZsARamzryCQ31lft5A64qErFhYKK9bFwxmJRu/CxKbjzAXYmdyS0yYwu1J3oqt2otNQ+wEKxogiC+GgX0t44vlDnTqJW7LRoK53ce+SmTUw6j/wTRmq295zz/e6Xf/75PuIif9hOfTenHvWU76fC2wBeQahfYkjguMCyLH7Wu6L/wOHNnDXNNQJYs+2Xa7pYeV7UJoKXLR6uExDfka3X/JH0d61AFgUYmChfbYXhVgCbSFitwhqfCwghvC1YY0Uv+dNC3gUB3G3BnaTeBZlop/AcrTQjcYM/5nw4X868AO54sBkRXm/3rRcClRDBwtP+qPPmhZo5AG4huAvC+0tVvFYwhpC4vjiW+qARognAfal0FcLwq9YHrbMvRdBJ2PaN/pbkz7WEZoBCsJPARtN4AZ9SuA5En7FH2Ol7ziNzADKF6bUWdNg4CJjws84odsl2v51+mcBzpt6KrFsmveSXsb7eATc/tYfkcCcha/Klm7sYfWHqlbTX99IP1QGcV6cuWXYaMyR7jEOAV/zVqRewkaFbCMYJjJh7dQazTsLP8Wy1A5nx0pClaK9pQMPJ/h3E9wTWte/lkO+l9lcB3HxQIDFqGiLhDVC/1vXCOpL3mvpjnYCCn3W8KsBgPtgB4nGjAGnmmJe+vFHrbi0P0g6PGvnPiwTs8LPOk7UOHCJxt1GAcJrsvvZo9sp6B24YLw10Kyoa+f8FOORnnXvOdaAwdRDgetOA+EL527501Q9blp+MPR0BSAd9L33fuQ4Ugu0EnjAFiHWViAOTY6nJzgGw3fecpzo6hEsC0HgIM/nSsMVoj3EHhPdU6XnUz/X9We1ArrSyqzv8mKBrmhHRGi6OJvdVO5DMlXoTXeEJo4tonl9BtahEtxDEl9myVhCSzp7qRSJ4Nn2m8SreR/LBVmYAfx2bTSWQY6VJu0v24DfTp0D0tsqQtN/30kOxrg7Qzn0uyAdwvKmQsJLkYKvi8fMIvKmYTR1pAjh/Ie0GscEkpFONgN1+1nm45m+aBzL50ioiPEJyeacFFvNJ+kOw1zYOqXNGsky+dDsRffRfjGSAdYfvJT9phPz/DaU1uos6ltcg6osJ8RgBu51zUVtMItt+8euR/h8X8hqtZu7E1PUM+YzJahb/UVF8a0lWswupq8tpV/kBC+GtIvuqyykQUfytupyCn7PSfyAetUy7ZdQB07BOdP8AHHSWMKZhltwAAAAASUVORK5CYII='; // 成功图标 var success_modal = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgCAYAAABzenr0AAADd0lEQVRYR82X328UVRzFz5ntRgj4K+rOLtEAUV80MUENINhfs/iAxl+J8UFejCaW3emDiS/6D/gs4k4J8cXgiwkmICH60J2xpSj6IjEaY4yx+GumLdCAUdN2d46Zku3+mmV3yxact5m595zPnPu9c+8lbvDFG+yPzgG8l9dltHEQ0JOCtgM0KWwStQRwhtKfAr4SjE9nbl08hUcPL3XycR0BpLz8vkTIAyDu6EQUwO9CuD+wxk62a39VgDsnX8skl5IfgHiinVDce0Ef/ZtU7lL/2Hyr/i0B0m7uKYJHAN6+GvNKH0F+mXhxbtiZitOJBTCL+acN8pNrMa7tKyEMyYFZ673TjZpNAJmivVnUdwQ39gog0pEw87fw4F97ChdqdZsA0q59hsCOXpqvDId0Isg6z7QEWK528cO1MK/WRDgUWGMTlftqAgIzXv4cwHt6ASDhsoA3SLxJ4N6qps76lrOtCSDlju5OQLGV2i1QZF42NDg37JzNePkRiIdqNcoy7pvNHvw5eraSQMa13wbwVrdmje1rzc2J0a1GOZxoTDWkXp8Zdg7UAxTzp0HuuhaAWvO7JkbuT5QSUyRTTZrSMT/rPF8HkC7a0yQ2rx5A8yXCimJPj488QPZNtvp1S/g6yBaWZ9qVIRCY9uxFAn2rA9C8wP7AKnyfGs89lDCMqMpva6kl/eFnnbsbAPILBJNxnSQdFXmK0DsEG/4dVfOMm3tEMlwSt1z1Q5oAAKTd/C8Et8R1LFHbomij/4QhHKlCVM1NL7eToTFOYkO7FKNlO7AKOxuLcBJkf2wCkL+YwI6Lg85vpmu/Quh9AhcEDkWxm66dNYATANa3M78y4vg4sAovdDUNBU2X+kq7zg8c9k0v/2pZmjpvjf2YLub2gjzeavjigGKnYepz+7FEiC/ajN1PSiZ2BwMH56J2pms/S+Bot8UrlrcGw4em6xKIZkLGs38FsFydrS5JP/yzfuHxDQs3bVfIkySMTmJfaSN942edhyv3dRXd6WIk4RyoTd3EXgXAoJ8tTMYCRA/XcjkGdNy3nOdqE2veD3j7t0DGtwRv7iraNo073pBUissAjvUKQEApNDAwO1T4slHzumxKEWJfsMfx4j7o/7str6U13dGXDOnd634wqYuscjRTuFeMNq0xRzPyjMTPen4061Uxdl0Da2lc0f4PeKyXMJXpyrIAAAAASUVORK5CYII='; // 警告图标 var warning_modal = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgCAYAAABzenr0AAAC8ElEQVRYR8WXTUhUURTHf0fToEWQfbmoKKjIRSotCiwoiDCqTWCCRkGl84aEllmLQgqkWpbVvBlxIRUxfay0j00fEGSr1CIi0igMhCRpE0k5J+4bJsdxPu4bhzzb9///z/+de++55wo+Qq+xiGLqUdYDS4DlCDGUb8AYwgcmicpxxm1lJRdQoxTznQaEQ8BOoDgrR/mD8AQlIkHu5tLPaEDbKKKcJoQzwIpcQhm+v0IISoD+TPy0BtRlAcp9hNo8EyfTFDglDpfSac0woFcpZx6PgcoCJJ+SULop46jUM5msO82AupQAfcAmy+RfgBGgxhJ/TxzqshnoAFqsxIRjEqDLYDXEaYR2Kx6cF4ezCey/CqjLXqDHSkQZlyBlCaxeZiHz+WHFNSBhtwS8ZcYz4JVeGUJYaSkyJA5rp+00F7PZ7EIZkCDVyQZOAhft2B5qdgbimRskwO14BUJ8Qlj9Xw0oLyVIjWiECmK885G8MBXAW7Jloi5+y18oA8ZCkzFgjtKROaiASemKhnhojsWcGFB6jIF+hKo5MvDaLMFAHn1/9scw/seDpgK9CHt8VmBEnKmmpVFKGWfCp4bZhI9MBVwg4JtcRJU0M+j1kTD7vevbf3QZA01AxDdX+YrQiVKJePdIqW8NaBXtYDEl3kyXczzLI0F2Sow1iVb8AmGrrwTKBEIUGEZpRFjniw/vxaEibuA6Bynihg+BX8BmcXjj8ePX8UdgqQ+NVjOmJc8DZkNttBS4Iw71yVh1uQk0WvGVYYQN4vB7ykCYWnMsrATguTjsSDHwDNhuyd8nDr0GmzoTngNvDM8diiNBwt4SuDhAKDfJgLkiQU4ksDOnYj+lBDOUmlhlmbyPMrYlT8bpDJjxrBPhsJWoLUh5yyS7pIXRZErml1F8TrhQkP6gPEA4IA4/U/1mbT4aphr11naL7Y+m4D6jtOMQEUk/tFp1Pw1Rh9Bs/TiFp0A3o9ySNmLZzFsZSAikeZ6bJ7qJsXyf538B0n8BZanHYR0AAAAASUVORK5CYII='; // 错误图标 var error_modal = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgCAYAAABzenr0AAADd0lEQVRYR9WXS4gcVRSG/79qEnGjk3vLhc+4FBVd6CJIpqsmEXyShUpcZCGO+AqIqOALUdSFgqAJGEUxKIgujAoSHyQg0z2jC4UEYkCEREQiI2jd7owmIdpT9Uu16Xl0V1f1tNMEa9X0veec75zz31O3iNP88DTHx/8PoDE+emWS+jeSWivxPJIegF8F/YwEe4Np9+1yqtpXBQR4jSi4W9DjAC8uCiBphuTLBvF2VjFXBlMKkGWcyn8P4GVlzhavSzjkecmdZrLxdZFdIYCLzO2S9zaJM5cTfH6v1CTwoKm513vZ9wSIK/YWevxooMAdRoSeMFX3Yp6vXIA4spdA3D9w5nmRlG62tfquzqVcABcGB0BcsRLZL/iQM6vdWu7F8cV+uwBcxUzA83Z2BRd2kfgyBbYTOCM3SeF3j3pY4L0A1nf7SJ+xtfpzxQA9smeKLWYqfv9oxW5IiC9Arl4SQGqIuCaouh9cGDwL4ulOgOyIBjV3fk+A42PBuSd9zOSWXjriay4cnZr9yUXmeoC7AY609koNKg3NVOOgi8w6iHtAnpXnx0+aV41Oz+5vry1pgYvMXYD3Vq/eS/htlf5ef/bUH4calWBT6uljAMeYpmNZ8Hq4ZiyFv6dIvJKeDGruhR4A9nmATxWLTw6JKna6/r2rmM2+ksNZRvG43Qjxs176WJgNeNPW4kwjrWdpBUK7E+REufp1lEkamunGd9neerjmBsH7BOSqUlvhc1uLb/pPAAKOjSTNsN3LhXbQLwOQtDuouU0Dt0DCCXjaEEy6b9y4uVXgL63fkbkN4gcgS94v6Ru2Wr9vUBGe8JhcZ6qNr9onQeDJdjXqUbBF0rtFECUiNBcA3pHcIQP85SG5dj64mPX81CzQ7PxJKIHwiatHJ+N9uRXI/nSRPQjw8k4ISluzt1o/gygO7Q6SW3MSiW01PqdwEtZDe7/I17qN9SHBbBRvKxrFPvRIQtxBcGN3EnjU1OKXCgEUYaQu+yPIi8oUvZx1AYftn/Gl3IdmIcC/bWiN2k+B8mPVF4TU9NO5dYtHcE8NtBfiyGZlfKevACWbBD0QVN2reduGfiUT8VBQdTt6MQ73UspkIju2RQUqBciMT13L7xH0WJ/X8lcM4m0rci3vpG99mMi/mdCFrQ+TTKjEzFA/TFZCiANrYJjBM999aWCYEP8A7TSkMOyQWnEAAAAASUVORK5CYII='; // 关闭图标 var close_modal = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgCAYAAABzenr0AAACFElEQVRYR+2WTWsTQRjH/08mLxepKIoHQcRLBRX0IPhCay2ih/opQpYJCeSQjxLIy7Ahn8KTivZVhB4aqEJ70V5FUSwlkmSHRyZYWJZtd3YDWQ/d487M8//Nf5555iGk/FHK+jgD+H8dcF33wWg0+lStVo+myZNms3kun8/fdhznY1icUAeUUs8BvALQ9zxvOSmEEc9ms+8A3AXwUkr5OghxEsB5Zn5PRPcAbA+Hw2e1Wu0wjhONRmOuUCi8BXCfmXeI6KmU8rcVgJmklPJD9LXWy5VK5ZcNRKvVuiCEmOz8NHET69QkNBAANgDcAfBZa70QBaGUugRgFcCtKPFIADOh2+1e1FqbgBMIAEtSyh9hThhxZt4konkAuwAWwmz3r7W6hn4IZt4XQjxxHOebP5Drule01mvH4kKIpVKp9DPqyKwAgk4w8xchxKNjiHa7fZWI1onohtm5rbjVEfh38M+JLQA3DQQzL5rxTCbzAcC1uOKxAcyCXq93eTwerxsIAF8BiKTiiQBCIMyvWLbHTsJgIgVcMMN7uVxusVgsfo9KOutCdFIgvzgzH0xsJLpuIIQQj20yP7EDqSZhoBbM9hqmWohSLcVB8Zk+RgHx2T7HgWd4moZkLaoniGrJtj3Pe5G0JfN1RaYxWSmXy2+sC1Gn03k4GAz69Xr9T9zq5p+fqCmdRjDuWut+IG5g2/lnAKk78Bd192wwo+7w4AAAAABJRU5ErkJggg=='; // 问号按钮 var question_modal = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgCAYAAABzenr0AAADlUlEQVRYR72XXWhUVxDHf7MJpYlCjdWkUloLVWmgvhSCUigUffADioqyIuapJd5NUwQxxra2Nf1AaQWp4EfWbygWTH0RQhUUNWqhhbRNihhEUjWCDZiEpCGka7I75dw9m+xudu+9m9Se1/Of//zPnDlzZoSAS/dTQimrgI3AQoTnUSqABEIf0AvcRjjHP/woW4kFoRY/kJ5kLqPsQdmMUOKHt/vDKN8xxifygSsu78orQBsp5gW2I3wKzAjoOBs2BHzOXxyQRsZyceQUoIcpo4gW4M0pOs40U34lzjqp42E23yQBGuVVlMsIr/wnzidIBhHWyBZa03kzBGiUOSjtCC/6Olc6EAZcnLq5sThAjvRTzGJ5j0cp/nEBbpbP4CbwRl7nygjCV4RokRr+SMdpM88wQDXKfuA5jwP8BiwVh1GDmRAQ5WugwcN5H0UsSzl2k3QelShxhLspQj3OAuL8Asz24PpYIuwdF6BHKCfEA+BZD+XLxOGqG/EjhAkRBWZZ/CBQLw7H3f0mViJc8BDwN8LL4jDoRkCjNAGOh0GbRKiy2NeAW0DRJLyyViKct7iffF7RPnFoEPfu+hnwSaDPxOFLe7ofEDbkEdsqDm9bAd8AOzwi2k8Z5aJHWYFy0SfrT6J8j9AB7lWV5sQrPRJhnhWwG2j05BVWi0Y5CNT5PrsgAOWKRFhuI2UEb/I0U06INnEVSYZtmmuIEEukhk49xEyK6fEt4co1E4FOwCTWdNZjEqyQWn63pz+LEPYlVO4aAaaaeRUOP55uwDzRLut8H0K9n5G7r4yYKxhCmBnIYDKonzhV8j5/2sQzVXBbYC4lZiJwB1gU2CgdmKBaajlT8MknOLqmk4TDlDFbwjzRo7yLcmIKh2g1ETgM1BZsnPnkriO8VTAHnDIR8K7b+ViVMxKh2oa/G+GlggUIG4KW4lzc7ZCs+ygNAXqBbI4nDDMr9RmZn21LwSeYjoHSLBE2JgUco4IE932+42x39wBTA0wEKhHKA+tRYsRZaHrE9IbENAgfBiaBXeKwx+aAf91PJ1a+kAjms0rriIK0ZJnqpiogd0tmK9kcoA2Y7xsJ5QrCDXsF6xFe97WB/E1pytjt6ca49FTa8hDvSI0VbR3+X4PJz8QJBxpMxiOR7Hp3Ah/5/uv5Y58czcr4VsLEc8Ge3nAKpxll95SH02y14+O5aTSURe54DiZpzepF3Sk4OZ7HuBB0PP8X+s83WFbJv84AAAAASUVORK5CYII='; function $modal(data) { // data 必传 且为对象 // 赋值默认参数 if (data === undefined) { data = {} } // 弹框类型不传默认为 alert if (data.type === undefined) { data.type = 'alert' } tipIconImg = ''; //提示显示小图标 // 提示类型 默认不传为 info if (data.icon === 'success') { tipIconImg = success_modal } else if (data.icon === 'warning') { tipIconImg = warning_modal } else if (data.icon === 'error') { tipIconImg = error_modal } else if (data.icon === 'question') { tipIconImg = question_modal } else { tipIconImg = info_modal } // 弹框显示时长 confirm 下无效 if (data.timeout === undefined && data.type != 'confirm') { data.timeout = 2000; } else { // 最短显示时间为500 data.timeout < 500 ? data.timeout = 500 : data.timeout = data.timeout; } // 过渡动画 if (data.transition === undefined) { data.transition = 200 } // 遮罩层参数 if (data.mask === undefined) { data.mask } // 最小宽度 if (data.width === undefined) { data.width = 300 } // 禁止页面滚动 if (data.pageScroll === undefined) { data.pageScroll = true } // 提示框标题 if (data.title === undefined) { data.title = '提示' } // 遮罩层关闭 if (data.maskClose === undefined) { data.maskClose = false } // 取消按钮文字 if (data.cancelText === undefined) { data.cancelText = '取消' } // 确认按钮文字 if (data.confirmText === undefined) { data.confirmText = "确认" } // 距离顶部距离 if (data.top === undefined) { data.top = 100 } // 是否绝对剧中 if (data.center === undefined) { data.center = false } // 公共计算当前元剧中top 值 function calculate(e, sun) { if (data.center) { var tipHeight = e.height() + sun; console.log(tipHeight) var windowHeight = $(window).height(); console.log((windowHeight / 2) - (tipHeight / 2)) data.top = (windowHeight / 2) - (tipHeight / 2) } } // 生成相对唯一id 保证弹框绑定id唯一 保证事件绑定不重复 (此处用于保证弹框内层 继续 弹框 不出现事件绑定重复) var idText = "modailItem"; var date = (new Date().getTime() * Math.random() + '').substr(0, 10) idText += date; var titleSize = 16; //标题文字大小 var fontSize = 14; //字体大小 // 创建最外层元素 if ($('#modail-dialog-box').index() < 0) { var modelBox = '<div id="modail-dialog-box"></div>' $('body').append(modelBox) // 设置样式 $('#modail-dialog-box').css({ fontFamily: '微软雅黑', fontSize: fontSize + 'px', color: '#666666', }) } // 生成 message 提示 if (data.type === 'message') { $('#modail_message_box').html(''); $('#modail-dialog-box').append('<div id="modail_message_box"></div>') // 设置message提示框长度 var width_s = 40 + (fontSize * data.content.length) + fontSize + 4; // 不需要遮罩层 $('#modail_message_box').append('<div id="' + idText + '_box"></div>'); // 添加左侧图标 $('#' + idText + '_box').append('<div class="' + idText + '_item item1"><img src="' + tipIconImg + '" /></div>'); // 提示内容 $('#' + idText + '_box').append('<div class="' + idText + '_item item2">' + data.content + '</div>'); if (data.closable) { // 右侧关闭图标 width_s += fontSize + 4; $('#' + idText + '_box').append('<div class="' + idText + '_item item3"><img src="' + close_modal + '" /></div>'); } // 设置外层框样式 $('#' + idText + '_box').css({ width: width_s + 'px', padding: '10px 15px', background: '#fff', boxShadow: '0 1px 6px rgba(0,0,0,.2)', borderRadius: '5px', position: 'fixed', left: '0', margin: 'auto', right: '0', opacity: '0', }) // 设置文字内容框样式 $('.' + idText + '_item.item2').css({ margin: '0 8px' }) // 设置文字关闭按钮靠边样式 $('.' + idText + '_item.item3').css({ position: 'absolute', right: '15px', cursor: 'pointer' }) // 设置内层框公共样式 $('.' + idText + '_item').css({ display: 'inline-block' }) $('.' + idText + '_item img').css({ width: fontSize + 4 + 'px', verticalAlign: 'top', position: 'relative', top: '1px' }) // 计算 距离顶部距离 calculate($('#' + idText + '_box'), 20) $('#' + idText + '_box').css({ top: data.top - 60, }) // 显示动画 $('#' + idText + '_box').animate({ 'top': data.top, 'opacity': '1', }, data.transition) // 关闭动画 function close() { $('#' + idText + '_box').animate({ 'top': data.top - 60, 'opacity': '0', }, data.transition) // 删除DOM setTimeout(function () { $('#' + idText + '_box').remove(); }, data.transition) } // 定时关闭 var timers; if (!data.manual) { timers = setTimeout(close, data.timeout); } // 按钮关闭 $('.' + idText + '_item.item3').click(function () { // 清除定时器 clearInterval(timers) close(); }) } else if (data.type === 'alert') { // 是否禁止页面滚动 if (data.pageScroll) { $('body').css('overflow', 'hidden') } // alert 提示框展示 // 添加 alert 提示框 $('#modail-dialog-box').append('<div id="' + idText + '_box"></div>') // 是否添加遮罩层 if (data.mask) { $('#' + idText + '_box').append('<div class="' + idText + '_mask"></div>') // 设置mask外层弹框样式 $('.' + idText + '_mask').css({ position: 'fixed', top: 0, right: 0, left: 0, bottom: 0, background: 'rgba(0,0,0,0.4)', }); } // 生成中间层 $('#' + idText + '_box').append('<div class="' + idText + 'centerBox"></div>'); $('.' + idText + 'centerBox').css({ width: data.width + 'px', padding: '20px', background: '#ffffff', position: 'fixed', right: 0, left: 0, margin: 'auto', borderRadius: '5px', boxShadow: '0 1px 6px rgba(0,0,0,.2)', opacity: '0' }) // 生成头部以及提示内容部分 $('.' + idText + 'centerBox').append('<div class="' + idText + 'title"></div>') // 添加左侧图标 $('.' + idText + 'title').append('<div class="' + idText + '_item item1"><img src="' + tipIconImg + '" /></div>'); // 提示标题 $('.' + idText + 'title').append('<div class="' + idText + '_item item2">' + data.title + '</div>'); if (data.closable) { // 右侧关闭图标 $('.' + idText + 'title').append('<div class="' + idText + '_item item3"><img src="' + close_modal + '" /></div>'); } // 设置标题框文字样式 $('.' + idText + '_item.item2').css({ margin: '0 8px', fontSize: titleSize + 'px', color: '#333333' }) // 设置文字关闭按钮靠边样式 $('.' + idText + '_item.item3').css({ position: 'absolute', right: '15px', cursor: 'pointer' }) // 设置内层框公共样式 $('.' + idText + '_item').css({ display: 'inline-block' }) $('.' + idText + '_item img').css({ width: titleSize + 4 + 'px', verticalAlign: 'top', position: 'relative', top: '-1px' }) // 设置提示框 box 样式 $('.' + idText + 'title').css({ marginBottom: '10px' }) // $('.' + idText + 'centerBox').append('<div class="' + idText + 'body">' + data.content + '</div>') // 设置样式 $('.' + idText + 'body').css({ padding: '0 ' + (titleSize + 10) + 'px' }) // 计算距离顶部距离 calculate($('.' + idText + 'centerBox'), 40); $('.' + idText + 'centerBox').css({ top: data.top + 40, }) // 显示动画 $('.' + idText + 'centerBox').animate({ 'top': data.top, 'opacity': '1', }, data.transition); // 关闭动画 function close() { $('.' + idText + 'centerBox').animate({ 'top': data.top + 40, 'opacity': '0', }, data.transition) // 删除DOM setTimeout(function () { $('#' + idText + '_box').remove(); if (data.pageScroll) { $('body').css('overflow', 'auto') } }, data.transition) } // 定时关闭 var timers; if (!data.manual) { timers = setTimeout(close, data.timeout); } // 按钮关闭 $('.' + idText + '_item.item3').click(function () { // 清除定时器 clearInterval(timers) close(); }) // 遮罩层关闭 if (data.maskClose) { $('.' + idText + '_mask').click(function () { // 清除定时器 clearInterval(timers) close(); }) } } else if (data.type === 'confirm') { // 是否禁止页面滚动 if (data.pageScroll) { $('body').css('overflow', 'hidden') } // alert 提示框展示 // 添加 alert 提示框 $('#modail-dialog-box').append('<div id="' + idText + '_box"></div>') // 是否添加遮罩层 if (data.mask) { $('#' + idText + '_box').append('<div class="' + idText + '_mask"></div>') // 设置mask外层弹框样式 $('.' + idText + '_mask').css({ position: 'fixed', top: 0, right: 0, left: 0, bottom: 0, background: 'rgba(0,0,0,0.4)', }); } // 生成中间层 $('#' + idText + '_box').append('<div class="' + idText + 'centerBox"></div>'); $('.' + idText + 'centerBox').css({ width: data.width + 'px', padding: '20px', background: '#ffffff', position: 'fixed', right: 0, left: 0, margin: 'auto', borderRadius: '5px', boxShadow: '0 1px 6px rgba(0,0,0,.2)', opacity: '0' }) // 生成头部以及提示内容部分 $('.' + idText + 'centerBox').append('<div class="' + idText + 'title"></div>') // 添加左侧图标 $('.' + idText + 'title').append('<div class="' + idText + '_item item1"><img src="' + tipIconImg + '" /></div>'); // 提示标题 $('.' + idText + 'title').append('<div class="' + idText + '_item item2">' + data.title + '</div>'); if (data.closable) { // 右侧关闭图标 $('.' + idText + 'title').append('<div class="' + idText + '_item item3"><img src="' + close_modal + '" /></div>'); } // 设置标题框文字样式 $('.' + idText + '_item.item2').css({ margin: '0 8px', fontSize: titleSize + 'px', color: '#333333' }) // 设置文字关闭按钮靠边样式 $('.' + idText + '_item.item3').css({ position: 'absolute', right: '15px', cursor: 'pointer' }) // 设置内层框公共样式 $('.' + idText + '_item').css({ display: 'inline-block' }) $('.' + idText + '_item img').css({ width: titleSize + 4 + 'px', verticalAlign: 'top', position: 'relative', top: '-1px' }) // 设置提示框 box 样式 $('.' + idText + 'title').css({ marginBottom: '10px' }) // $('.' + idText + 'centerBox').append('<div class="' + idText + 'body">' + data.content + '</div>') // 设置样式 $('.' + idText + 'body').css({ padding: '0 ' + (titleSize + 10) + 'px' }) // 设置按钮 $('.' + idText + 'centerBox').append('<div class="' + idText + 'footer"><button class="button_s_model ' + idText + '_confirm">' + data.confirmText + '</button><button class="button_s_model ' + idText + '_cancel">' + data.cancelText + '</button><div class="clearBoth"></div></div>') $('.' + idText + 'footer').css({ paddingTop: '20px' }) // 设置样式 $('.button_s_model').css({ float: 'right', marginLeft: '20px', outline: 'none', border: 'none', padding: '8px 30px', cursor: 'pointer', borderRadius: '5px', }) // 计算距离顶部距离 calculate($('.' + idText + 'centerBox'), 40) $('.' + idText + 'centerBox').css({ top: data.top + 40, }) // 显示动画 $('.' + idText + 'centerBox').animate({ 'top': data.top, 'opacity': '1', }, data.transition); // 鼠标移入移出 $('.button_s_model').hover(function () { $(this).css({ 'opacity': '.8' }) }, function () { $(this).css({ 'opacity': '1' }) }) // 鼠标按下 $('.button_s_model').mousedown(function () { $(this).css({ 'opacity': '1' }) }).mouseup(function () { $(this).css({ 'opacity': '.8' }) }) // 取消按钮样式 $('.button_s_model.' + idText + '_cancel').css({ background: '#fff', borderBox: 'box-sizing', border: '1px solid #eee', }).click(function () { data.cancel(close) }) // 确认按钮样式 $('.button_s_model.' + idText + '_confirm').css({ background: '#2d8cf0', color: '#fff', }).click(function () { data.confirm(close) }) // 清除浮动 $('.clearBoth').css({ clear: 'both' }) // 关闭动画 function close() { $('.' + idText + 'centerBox').animate({ 'top': data.top + 40, 'opacity': '0', }, data.transition) // 删除DOM setTimeout(function () { $('#' + idText + '_box').remove(); if (data.pageScroll) { $('body').css('overflow', 'auto') } }, data.transition) } // 按钮关闭 $('.' + idText + '_item.item3').click(function () { close(); }) // 遮罩层关闭 if (data.maskClose) { $('.' + idText + '_mask').click(function () { close(); }) } } } // 错误提示 function $error(e){ $modal({ type: 'message', //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: 'error', // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: e, // 提示文字 center: true,// 是否绝对居中 默认为false 设置true后 top无效 top:100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: true, // 是否显示可关闭按钮 默认为 false }) } // 成功提示 function $success(e){ $modal({ type: 'message', //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: 'success', // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: e, // 提示文字 center: true,// 是否绝对居中 默认为false 设置true后 top无效 top:100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: true, // 是否显示可关闭按钮 默认为 false }) }
-
使用弹窗库
function message(e) { $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: e, // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: "手机号格式有误", // 提示文字 center: false, // 是否绝对居中 默认为false 设置true后 top无效 top: 100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: true, // 是否显示可关闭按钮 默认为 false }); } $(".message").click(function () { message($(this).attr("data-icon")); }); // $(".message1").click(function () { $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "info", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: "人间值得", // 提示文字 center: true, // 是否绝对居中 默认为false 设置true后 top无效 top: 100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: false, // 是否显示可关闭按钮 默认为 false }); }); // $(".message2").click(function () { $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "info", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: "人间值得", // 提示文字 top: 100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: false, // 是否显示可关闭按钮 默认为 false }); }); // alert function alert(e) { $modal({ type: "alert", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: e, // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) title: "问你个问题", // 提示文字 content: "人间值得?", // 提示文字 top: 300, //距离顶部距离 单位px center: true, // 是否绝对居中 默认为false 设置true后 top无效 transition: 300, //过渡动画 默认 200 单位ms closable: true, // 是否显示可关闭按钮 默认为 false mask: true, // 是否显示遮罩层 默认为 false pageScroll: true, // 是否禁止页面滚动 width: 300, // 单位 px 默认显示宽度 最下默认为300 maskClose: true, // 是否点击遮罩层可以关闭提示框 默认为false }); } $(".alert").click(function () { alert($(this).attr("data-icon")); }); // $(".alert2").click(function () { $modal({ type: "alert", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "info", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 999999999, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) 时间越长相当于需要手动关闭 title: "问你个问题", // 提示文字 content: "人间值得?", // 提示文字 transition: 300, //过渡动画 默认 200 单位ms top: 100, //距离顶部距离 单位px closable: true, // 是否显示可关闭按钮 默认为 false mask: false, // 是否显示遮罩层 默认为 false pageScroll: false, // 是否禁止页面滚动 width: 300, // 单位 px 默认显示宽度 最下默认为300 maskClose: true, // 是否点击遮罩层可以关闭提示框 默认为false }); }); // confirm $(".confirm").click(function () { $modal({ type: "confirm", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "info", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' title: "问你个问题", // 提示文字 content: "人间值得?", // 提示文字 transition: 300, //过渡动画 默认 200 单位ms closable: true, // 是否显示可关闭按钮 默认为 false mask: true, // 是否显示遮罩层 默认为 false top: 400, //距离顶部距离 单位px center: true, // 是否绝对居中 默认为false 设置true后 top无效 pageScroll: false, // 是否禁止页面滚动 width: 500, // 单位 px 默认显示宽度 最下默认为300 maskClose: true, // 是否点击遮罩层可以关闭提示框 默认为false cancelText: "取消", // 取消按钮 默认为 取消 confirmText: "确认", // 确认按钮 默认未 确认 cancel: function (close) { // 回调函数 // 函数返回关闭事件 调用-关闭弹窗 $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "success", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' content: "用户点击了取消", // 提示文字 }); close(); // 调用返回的 关闭弹框函数 才能关闭 }, confirm: function (close) { // 回调函数 // 函数返回关闭事件 调用-关闭弹窗 $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "success", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' content: "用户点击了确认", // 提示文字 }); close(); // 调用返回的 关闭弹框函数 才能关闭 }, }); }); // 快捷错误提示 // $error('这是一条错误提示!') // 快捷成功提示 // $success('这是一条成功提示!')
-
如果用户输入时输入了手机号格式有误,会是以下效果
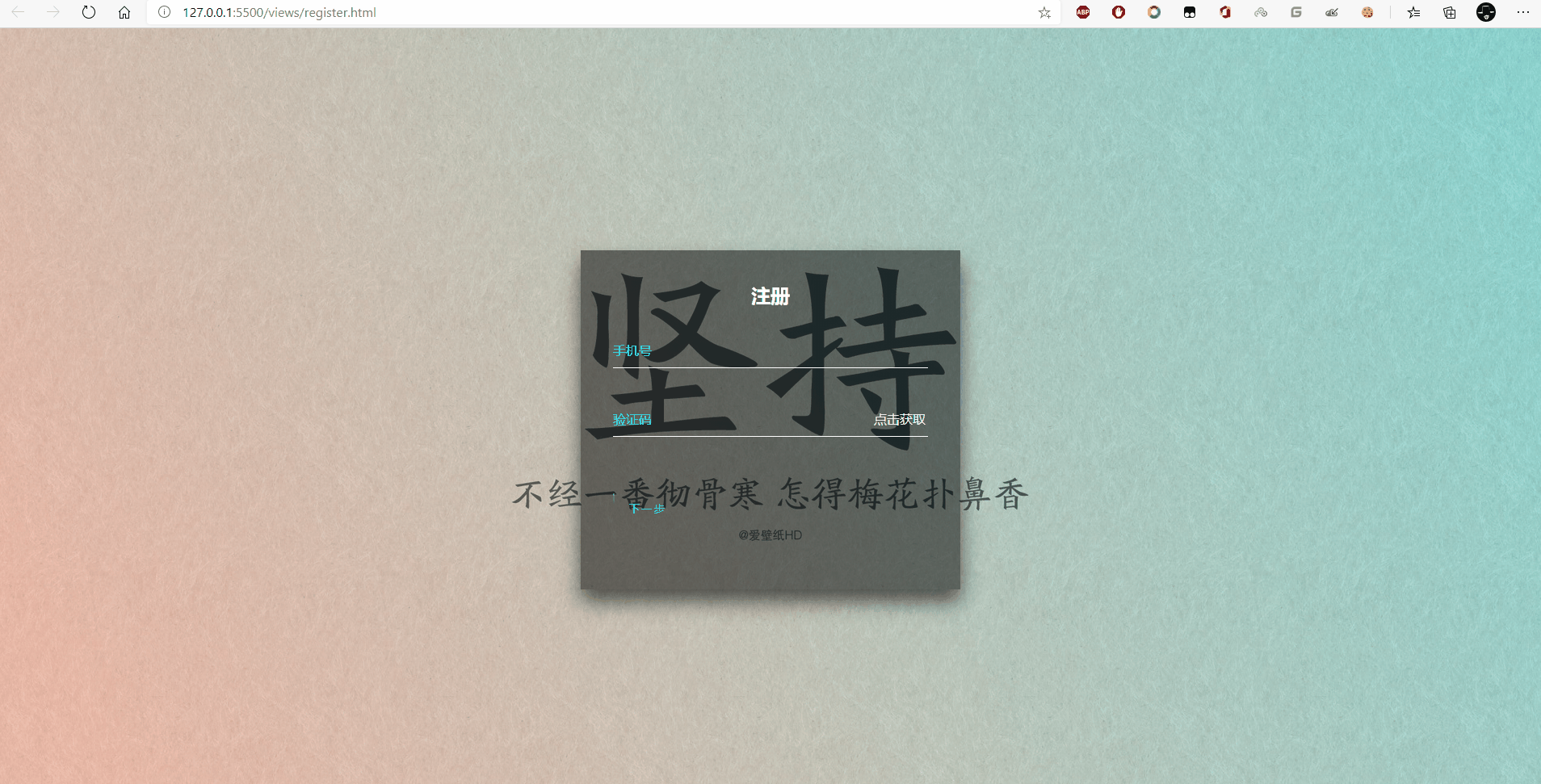
2.登录页
然后是写登录页的页面
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Document</title>
</head>
<link rel="stylesheet" href="css/login.css" />
<body>
<button class="message" data-icon="info"></button>
<div class="loginBox">
<h2>登录</h2>
<!-- 登录方式 -->
<div class="loginWay">
<!-- 短信验证码登录 -->
<div class="codeLogin">
短信验证码登录
<div class="line1"></div>
</div>
<span style="font-size: 29px; color: aquamarine">|</span>
<!-- 服务密码登录 -->
<div class="pwdLogin">
服务密码登录
<div class="line2"></div>
</div>
</div>
<form action="" method="" class="codeForm">
<div class="item">
<input type="text" required />
<label for="">手机号</label>
</div>
<div class="item">
<input type="text" required />
<label for="">验证码</label>
<a href="" class="getCode">点击获取</a>
</div>
<button type="submit" class="btn">
登录
<span></span>
<span></span>
<span></span>
<span></span>
</button>
</form>
<form action="" method="" class="pwdForm">
<div class="item">
<input type="text" required id="phone" name="phone" />
<label for="">手机号</label>
</div>
<div class="item">
<input type="password" required id="password" name="password" />
<label for="">密码</label>
</div>
<button type="submit" class="btn">
登录
<span></span>
<span></span>
<span></span>
<span></span>
</button>
</form>
</div>
</body>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/1.10.0/jquery.min.js"></script>
<script src="./js/modal_dialog.js"></script>
<script src="js/tccj.js"></script>
<script>
$(function () {
// 点击短信验证码登录触发
$(".codeLogin").on("click", (e) => {
// 登录操作切换动画
$(".line2").css("width", "0");
$(".line1").css("width", "100%");
// form表单旋转动画
$(".codeForm").css("display", "block");
$(".pwdForm").css("display", "none");
$(".codeForm").css("animation", "rotate 0.8s ease-in-out");
});
$(".codeForm").css("transform", "rotateY(0deg)");
// 点击服务密码登录触发
$(".pwdLogin").on("click", (e) => {
// 登录操作切换动画
$(".line2").css("width", "100%");
$(".line1").css("width", "0");
// form表单旋转动画
$(".codeForm").css("display", "none");
$(".pwdForm").css("display", "block");
$(".pwdForm").css("animation", "rotate 0.8s ease-in-out");
});
});
</script>
<script>
$(function () {
// 用户点击发送短信
$(".getCode").on("click", (e) => {
// 发送短信
$.ajax("", {
type: "get",
});
});
// 用户提交表单
$("form").on("submit", (e) => {
// 阻止默认行为
e.preventDefault();
// 判断手机号格式是否正确
var $phone = $("#phone").val();
if (!/^1[3456789]\d{9}$/.test($phone)) {
// 提示错误信息
$(".message").trigger("click");
return false;
}
});
});
</script>
</html>
因为CSS样式是用的上面的那个CSS,所以这里就不写了
直接看效果
3.添加头部tab
HTML部分
<header>
<a href="/" class="iconfont icon-shouye">首页</a>
<a href="/login" class="iconfont icon-denglu">登录</a>
<a href="/register" class="iconfont icon-zhuce">注册</a>
</header>
CSS部分
header {
width: 250px;
height: 50px;
position: fixed;
top: 0;
right: 0;
top: 10px;
}
header > a {
text-decoration: none;
color: #fff;
font-weight: 800;
margin-left: 20px;
position: relative;
}
header > a::after {
content: "";
display: block;
position: absolute;
left: 0;
bottom: -8px;
width: 0;
height: 3px;
background: #3498db;
transition: all 1s cubic-bezier(0.175, 0.885, 0.32, 1.275) 0s;
}
header > a:hover::after {
width: 100%;
}
实现效果如下
4.完善注册页的ajax请求
$(function () {
// 用户点击发送短信
// $(".getCode").on("click", (e) => {
// // 发送短信
// $.ajax("", {
// type: "get",
// });
// });
// 用户提交表单
$("form").on("submit", (e) => {
// 阻止默认行为
e.preventDefault();
// 判断手机号格式是否正确
var $phone = $("#phone").val();
if (!/^1[3456789]\d{9}$/.test($phone)) {
// 提示错误信息
$(".message").trigger("click");
return false;
} else {
// 手机号格式正确
// 表单序列化
var data = $("form").serializeArray();
// 提交注册信息
$.ajax("/register", {
type: "post",
data,
success: function (response) {
if (response.code === 200) {
console.log(response.message);
// 用户注册完毕直接跳到抽奖页面
window.location = "/";
} else if (response.code === 400) {
$(".message4").trigger("click");
console.log(response.message);
}
},
});
}
});
});
5.完善登录页的ajax请求
$(function () {
// 用户点击发送短信
// $(".getCode").on("click", (e) => {
// // 发送短信
// $.ajax("", {
// type: "get",
// });
// });
// 用户提交表单--验证码登录
$(".codeForm").on("submit", (e) => {
// 阻止默认行为
e.preventDefault();
// 判断手机号格式是否正确
var $phone = $("#phone").val();
if (!/^1[3456789]\d{9}$/.test($phone)) {
// 提示错误信息
$(".message").trigger("click");
return false;
} else {
// 手机号格式正确
// 表单序列化
var data = $(".codeForm").serializeArray();
// 手机验证码登录
$.ajax("/loginY", {
type: "post",
data,
success: function (response) {
if (response.code === 200) {
// console.log(response.message);
// 提示用户登录成功弹窗
// $(".message2").trigger("click");
// 用户登录完毕直接跳到抽奖页面
window.location = "/";
} else if (response.code === 400) {
$(".message1").trigger("click");
console.log(response.message);
}
},
});
}
});
$(".pwdForm").on("submit", (e) => {
e.preventDefault();
// 判断手机号格式是否正确
var $phone = $("#phone2").val();
if (!/^1[3456789]\d{9}$/.test($phone)) {
// 提示错误信息
$(".message").trigger("click");
return false;
} else {
// 手机号格式正确
// 表单序列化
var data = $(".pwdForm").serializeArray();
// 手机验证码登录
$.ajax("/loginM", {
type: "post",
data,
success: function (response) {
if (response.code === 200) {
$(".message2").trigger("click");
// console.log(response.message);
// 用户登录完毕直接跳到抽奖页面
window.location = "/";
} else if (response.code === 400) {
$(".message3").trigger("click");
// console.log(response.message);
}
},
});
}
});
});
其中用到的弹窗插件为,首先在需要用到弹窗插件的页面中添加以下button按钮,其class值为message、message1、message2、message3…
<button class="message" data-icon="info"></button>
<button class="message1" data-icon="warning"></button>
<button class="message2" data-icon="success"></button>
<button class="message3" data-icon="warning"></button>
-
tccj__info.js
.message
–手机号格式有误
.message1
–此用户未注册
.message2
–登录成功
.message3
–用户名或密码错误
function message(e) { $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: e, // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: "手机号格式有误", // 提示文字 center: false, // 是否绝对居中 默认为false 设置true后 top无效 top: 100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: true, // 是否显示可关闭按钮 默认为 false }); } $(".message").click(function () { message($(this).attr("data-icon")); }); $(".message1").click(function () { $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "warning", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: "此用户未注册", // 提示文字 center: false, // 是否绝对居中 默认为false 设置true后 top无效 top: 100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: false, // 是否显示可关闭按钮 默认为 false }); }); // $(".message2").click(function () { $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "success", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: "登录成功", // 提示文字 top: 100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: false, // 是否显示可关闭按钮 默认为 false }); }); $(".message3").click(function () { $modal({ type: "message", //弹框类型 'alert' or 'confirm' or 'message' message提示(开启之前如果之前含有弹框则清除) icon: "warning", // 提示图标显示 'info' or 'success' or 'warning' or 'error' or 'question' timeout: 2000, // 单位 ms 显示多少毫秒后关闭弹框 ( confirm 下无效 | 不传默认为 2000ms | 最短显示时间为500ms) content: "用户名或密码错误", // 提示文字 top: 100, //距离顶部距离 单位px transition: 300, //过渡动画 默认 200 单位ms closable: false, // 是否显示可关闭按钮 默认为 false }); });
-
然后去modal_dialog.js文件中去设置以下message提示框长度
// 设置message提示框长度 var width_s = 40 + fontSize * data.content.length + fontSize + 10;
提示:可以在文件中直接用 CTRL + F 搜索即可
现在你的弹窗应该已经可以正常运作了,只需要在需要弹窗的地方自动触发button按钮即可
$(".message").trigger("click"); // 自动点击class值为message的元素
- 弹窗效果如下
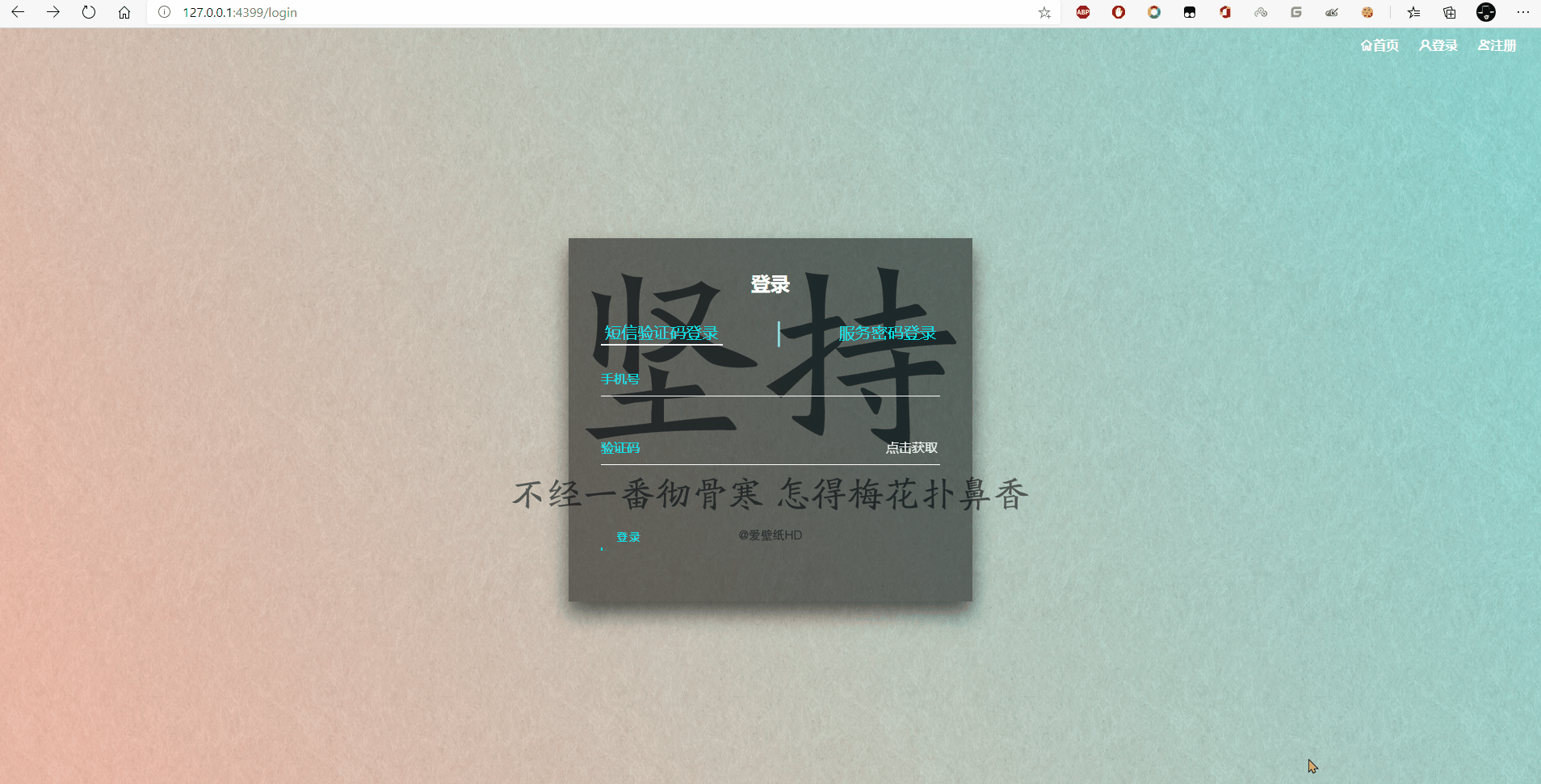
6.抽奖页 – 首页
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>首页</title>
<link rel="stylesheet" href="/css/index.css" />
<link
rel="stylesheet"
href="http://at.alicdn.com/t/font_2067208_jokj9bfucw.css"
/>
<link rel="stylesheet" href="/css/login.css" />
<script src="/js/jQuery-1.12.4.js"></script>
<script src="/js/jqueryRotate.js"></script>
<script>
$(function () {
$(".pointer").click(function () {
var duArry = [
{ num: 4350, name: "免单4999" },
{ num: 1550, name: "免单50元" },
{ num: 2250, name: "免单50元" },
{ num: 2311, name: "免单10元" },
{ num: 2281, name: "免单10元" },
{ num: 2300, name: "免单10元" },
{ num: 2000, name: "免单5元" },
{ num: 1980, name: "免单5元" },
{ num: 2720, name: "免单5元" },
{ num: 2700, namm: "免单5元" },
{ num: 1650, name: "免分期服务费" },
{ num: 1660, name: "免分期服务费" },
{ num: 1670, name: "免分期服务费" },
{ num: 1680, name: "免分期服务费" },
{ num: 1690, name: "免分期服务费" },
{ num: 1703, name: "提高白条额度" },
{ num: 1710, name: "提高白条额度" },
{ num: 1719, name: "提高白条额度" },
{ num: 1728, name: "提高白条额度" },
{ num: 1738, name: "提高白条额度" },
{ num: 1746, name: "提高白条额度" },
{ num: 1755, name: "未中奖" },
{ num: 1763, name: "未中奖" },
{ num: 1770, name: "未中奖" },
{ num: 1776, name: "未中奖" },
{ num: 1783, name: "未中奖" },
{ num: 1790, name: "未中奖" },
{ num: 1758, name: "未中奖" },
{ num: 1779, name: "未中奖" },
{ num: 1762, name: "未中奖" },
{ num: 1789, name: "未中奖" },
{ num: 1774, name: "未中奖" },
];
// 定义一个随机度数
var du = Math.floor(Math.random() * duArry.length);
var userPhone = $("#userPhone").text();
// 判断度数是否为undefined
while (duArry[du].name === undefined) {
du = Math.floor(Math.random() * duArry.length);
if (duArry[du].name !== undefined) {
break;
}
}
// 旋转轮盘
$(".rotate>img").rotate({
angle: 0,
animateTo: duArry[du].num,
duration: 5000,
callback: function () {
$.ajax("/reduce", {
type: "get",
data: {
phone: userPhone,
prize: duArry[du].name,
},
success: function (number) {
// console.log(number.data);
// 修改文本内容
$(".degree").text("您还剩余抽奖次数为" + number.data + "次");
if (number.data == 0) {
$(".pointer").unbind();
$.ajax("/show", {
type: "get",
data: {
phone: userPhone,
},
success: function (data) {
$.each(data, function (index, value) {
// console.log(value);
if (Array.isArray(value)) {
// console.log(value);
$.each(value, (index, prize) => {
console.log(prize);
$(".mask>ul").append($(`<li>${prize}</li>`));
});
// $(".mask>ul").append($(`<li>${value}</li>`));
}
});
},
}).then(function () {
// $(".mask").css("transform", "scale(1)");
// $(".mask").css("transition", "all 2s");
$(".mask").css(
"animation",
"scale 0.5s ease-in-out forwards"
);
});
}
},
});
},
});
});
});
</script>
</head>
<body>
<header>
<a href="/" class="iconfont icon-shouye">首页</a>
<a href="/login" class="iconfont icon-denglu">登录</a>
<a href="/quit" class="iconfont icon-tuichu_huaban1">退出</a>
</header>
<div class="container">
<div class="pointer"><img src="/img/pointer.png" alt="" /></div>
<div class="rotate"><img src="/img/jp.png" alt="" /></div>
</div>
<p class="degree">您的抽奖次数为<a> 3 </a>次</p>
<div class="mask">
<ul>
<!-- <li>未中奖</li>
<li>未中奖</li>
<li>未中奖</li> -->
</ul>
</div>
<script src="/js/index.js"></script>
</body>
</html>
使用JS动画来完成抽奖动画,用户抽三次奖,最后会出一个结果,不能继续抽奖
效果如下:
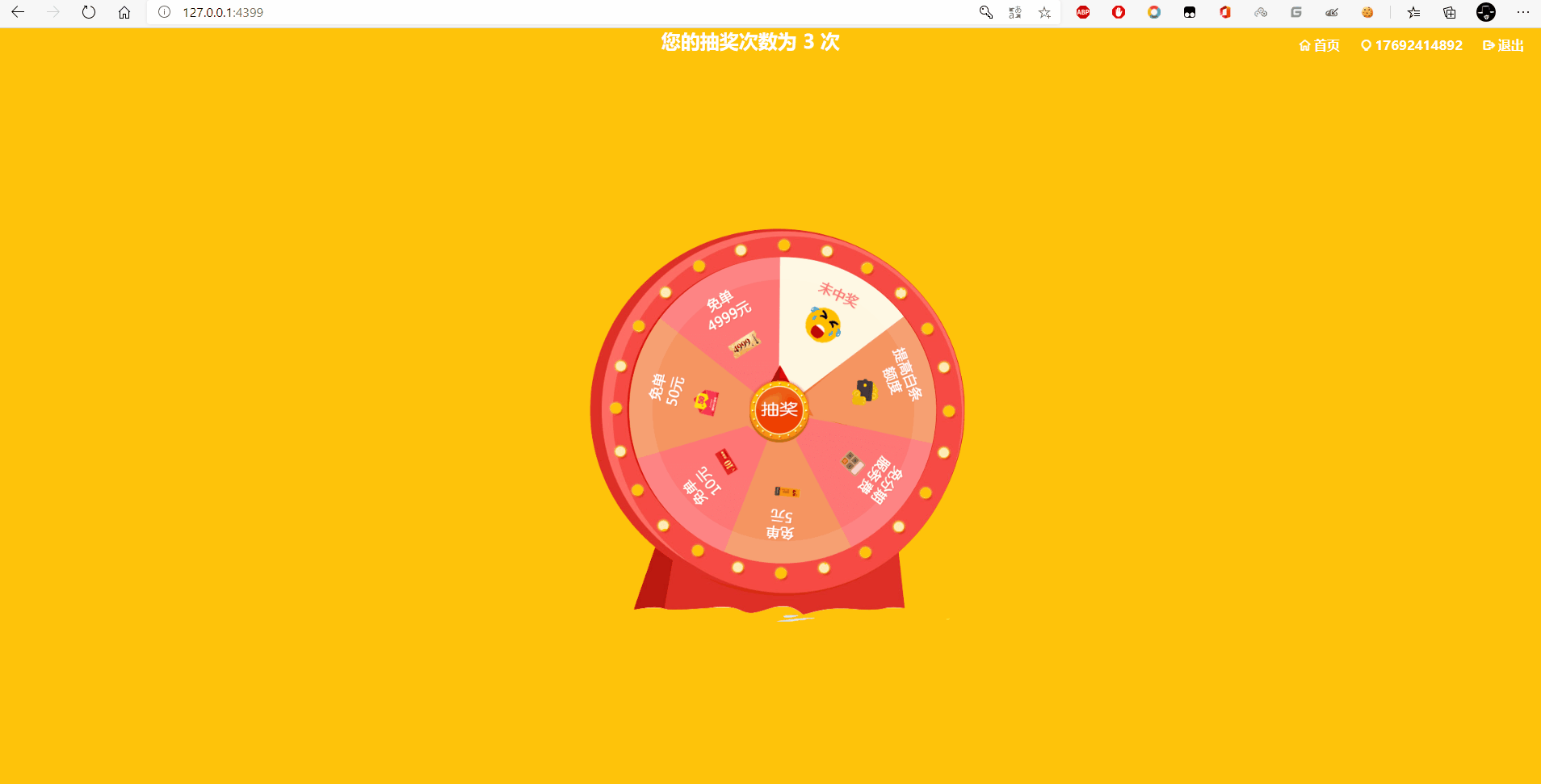
7.完成注册验证码接口的接入
第一次搞这个,遇到了很多的问题,没有及时解决,问题包括:什么阿里云天级流控、接口被占用啊等等一系列后端问题,于是后端说这是阿里云默认的限制,你短信不能发送的太快,于是呢我就换了个手机号发送。
如下错误信息
Error [isv.BUSINESS_LIMIT_CONTROLError]: 触发天级流控Permits:10, URL: https://dysmsapi.aliyuncs.com/
at processTicksAndRejections (internal/process/task_queues.js:97:5) {
data: {
RequestId: '8FD669CC-506F-46B2-BA4F-5702EFD0A1CB',
Code: 'isv.BUSINESS_LIMIT_CONTROL'
},
code: 'isv.BUSINESS_LIMIT_CONTROL',
url: 'https://dysmsapi.aliyuncs.com/',
entry: {
url: 'https://dysmsapi.aliyuncs.com/',
request: { headers: [Object: null prototype] },
response: { statusCode: 200, headers: [Object] }
}
}
-
1.首先我注册、登录时表单出现默认提交的情况,于是去百度上看了一下,只需要在需要点击提交的按钮上添加
onclick="return false"
,即可禁止默认提交行为<button type="submit" class="btn codeBtn" onclick="return false">
-
2.注册页的逻辑,这都是我自己想到的需求,可能不太完美吧,希望大佬多多建议
- 手机号必须是符合手机号格式的才能注册
- 密码必须是包含数字、字母、特殊符号中的其中两种的才能注册
- 验证码必须是和后端发送过来的验证码一致才能注册
- 点击下一步会自动进行登录操作,完成登录
$(function () { // 用户输入的手机号 var phone; // 用户输入的密码 var password; // 获取到的后端发送过来的验证码 var codeRes; // 设置一个点击状态,如果点击了获取验证码,则设置为true, // 如果点击了btn(下一步)而没有点击获取验证码,则设置为false var codeState = false; // 用户点击发送短信 -- 点击获取验证码 $(".getCode").on("click", (e) => { e.preventDefault(); // 当点击验证码后就获取到用户刚才输入的手机号和密码 phone = $("#phone").val(); password = $("#password").val(); // console.log(phone, password); // 判断手机号格式是否正确 if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else if ( !/^(?![a-z]+$)(?![A-Z]+$)(?!([^(0-9a-zA-Z)])+$)^.{12,16}$/.test( password ) ) { // 验证数字,大写字母,小写字母,特殊字符四选三组成的密码强度,且长度在12到16个数之间 $(".message9").trigger("click"); } else { var data = { phone, password, }; // 发送短信 $.ajax("/codeRequire", { type: "post", data, success: function (response) { // console.log(response); // console.log(response.code); // 表示用户已经点击了获取验证码 if (response.code === 304) { // 此用户已注册 $(".message8").trigger("click"); } else { // 验证码发送成功弹窗 $(".message7").trigger("click"); // 验证码获取倒计时 -- 60秒 var codeTime = 60; var timer = setInterval(() => { codeTime--; // 把每次的倒计时都重新渲染到HTML页面 $(".getCode").attr("value", codeTime); // 通过元素覆盖实现禁止点击 $(".coverGetCode").css("display", "block"); if (codeTime <= 0) { // 清除计时器 clearInterval(timer); $(".getCode").attr("value", "重新获取"); $(".coverGetCode").css("display", "none"); } }, 1000); // console.log(codeTime); // 60 // 获取从后端接收到的验证码 codeRes = response.data; console.log("验证码:", codeRes); codeState = true; } }, }); } }); // 用户提交表单 $(".btn").on("click", (e) => { if (codeState === true) { // 阻止默认行为 e.preventDefault(); var data = { phone, password, }; // 提交注册信息 $.ajax("/register", { type: "post", data, success: function (response) { // 获取用户输入的验证码 var codeInput = $("#code").val(); console.log("用户输入的验证码:", codeInput); // 如果用户没有注册 if (response.code === 200) { if (codeInput == codeRes) { // 验证码正确 console.log(response.message); // // 用户注册完毕直接跳到抽奖页面 // window.location = "/"; // 注册完成后完成登录功能 -- 使用密码登录 // 表单序列化 // 手机验证码登录 $.ajax("/loginM", { type: "post", data, success: function (response) { if (response.code === 200) { $(".message2").trigger("click"); // console.log(response.message); // 用户登录完毕直接跳到抽奖页面 window.location = "/"; // $("body").html() } else if (response.code === 400) { $(".message3").trigger("click"); // console.log(response.message); } }, }); } else { // 验证码错误 $(".message5").trigger("click"); } } else if (response.code === 400) { $(".message4").trigger("click"); console.log(response.message); } }, }); } else { $(".message6").trigger("click"); } }); });
-
3.效果演示
8.完成登录验证码接口的接入
-
1.有的人可能会说,为什么一个验证码接口要分为两个:一个注册验证码接口,和一个登录验证码接口,原因就是
-
登录验证码接口是我改的
加了用户手机号查询,用户点击获取验证码后,前端首先会给后端返回数据(手机号 – phone),然后 后端会去数据库里去查询有没有这个用户,如果没有就把数据返回给前端,然后前端会把该数据以任何形式渲染到页面中,提示用户未注册,这样可以提升用户体验感,不可能是不管你有没有注册,先给你发送验证码,先验证验证码对不对然后再点击登录后,,发现你没有注册,这样你可能就是反手就是一个退出了。
-
注册验证码接口是我加的
和上面一样
只不过是注册验证码接口是查询用户是不是注册了,如果用户已经注册了,提示用户已注册。
-
-
2.登录页的逻辑,和注册页的逻辑基本一样,这里不再赘述
$(function () { // 用户输入的手机号 var phone; // 获取到的后端发送过来的验证码 var codeRes; // 设置一个点击状态,如果点击了获取验证码,则设置为true, // 如果点击了btn(下一步)而没有点击获取验证码,则设置为false var codeState = false; // 用户点击发送短信 -- 点击获取验证码 $(".getCode").on("click", (e) => { e.preventDefault(); // 当点击验证码后就获取到用户刚才输入的手机号和密码 phone = $("#phone").val(); // console.log(phone, password); // 判断手机号格式是否正确 if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else { var data = { phone, }; // 发送短信 $.ajax("/code", { type: "post", data, success: function (response) { // console.log(response); // console.log(response.code); // 表示用户已经点击了获取验证码 if (response.code === 400) { // 此用户未注册 $(".message1").trigger("click"); } else { // 验证码发送成功弹窗 $(".message7").trigger("click"); // 验证码获取倒计时 -- 60秒 var codeTime = 60; var timer = setInterval(() => { codeTime--; // 把每次的倒计时都重新渲染到HTML页面 $(".getCode").attr("value", codeTime); // 通过元素覆盖实现禁止点击 $(".coverGetCode").css("display", "block"); if (codeTime <= 0) { // 清除计时器 clearInterval(timer); $(".getCode").attr("value", "重新获取"); $(".coverGetCode").css("display", "none"); } }, 1000); // console.log(codeTime); // 60 // 获取从后端接收到的验证码 codeRes = response.data; console.log("验证码:", codeRes); codeState = true; } }, }); } }); // 用户提交表单--验证码登录 $(".codeBtn").on("click", (e) => { if (codeState === true) { // 阻止默认行为 e.preventDefault(); // 判断手机号格式是否正确 phone = $("#phone").val(); if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else { // 手机号格式正确 // 表单序列化 var data = $(".codeForm").serializeArray(); // 手机验证码登录 $.ajax("/loginY", { type: "post", data, success: function (response) { if (response.code === 200) { // console.log(response.message); // 提示用户登录成功弹窗 $(".message2").trigger("click"); // 用户登录完毕直接跳到抽奖页面 window.location = "/"; } else if (response.code === 400) { $(".message1").trigger("click"); console.log(response.message); } }, }); } } else { $(".message6").trigger("click"); } }); // 密码登录 $(".pwdForm").on("submit", (e) => { e.preventDefault(); // 判断手机号格式是否正确 phone = $("#phone2").val(); if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else { // 手机号格式正确 // 表单序列化 var data = $(".pwdForm").serializeArray(); // 手机验证码登录 $.ajax("/loginM", { type: "post", data, success: function (response) { if (response.code === 200) { $(".message2").trigger("click"); // console.log(response.message); // 用户登录完毕直接跳到抽奖页面 window.location = "/"; } else if (response.code === 400) { $(".message3").trigger("click"); // console.log(response.message); } }, }); } }); });
-
效果如下
被限制了,晚点吧
9.页面跳转路由不刷新
…渐渐崩溃
今天又学到了新东西,刷新了我对注释的认知🥁
你猜下面这段代码放到我代码里会怎样???(就这样被注释着执行)
// $("body").html(`{% include "index.html" %}`);
结果是它居然能正常执行,搞的我一脸懵逼…,最后是实在受不了了,把它删了,就没事了,好像是不能包含 script
标签
原理,在ajax中有一股神秘的力量,我不知从何而来,我想触及到它,但我无法触及它,不管你有没有注释都会正常的执行
上面的代码意思是 我要跳转到 index.html
页面,而且我的 index.html
页面中在没有登录的情况下会自动跳转到登录页面,所以…就这样完成了递归原理来刷新页面,效果如下:
后来经过我们的一番讨论,想到了怎么完成这样的一个功能 – 页面变化而路由不变
那就是通过jQuery在想要跳转页面的地方都来重新绘制此页面,把此页面绘制成为想要跳转到的那个页面的样子,这当然在其中页遇到了问题,那就是 script
标签不能被直接拿来绘制,需要先创建 script
标签,在为其添加文件路径,最后把 script
标签之间append到body中即可
-
注册成功后显示用户抽奖界面(注:页面不刷新)
- 在全局定义那个loginM路由的形参,就可以在全局封装一个用来绘制抽奖页的函数了
$(function () { // 用户输入的手机号 var phone; // 用户输入的密码 var password; // 获取到的后端发送过来的验证码 var codeRes; // 全局从后端接收到的data数据 var timesLoginM; // 绘制首页的函数 function index() { setTimeout(() => { $("body").html(` <link rel="stylesheet" href="/css/index.css" /> <header> <a href="/" class="iconfont icon-shouye">首页</a> <a href="/login" class="iconfont icon-denglu">登录</a> <a href="/quit" class="iconfont icon-tuichu_huaban1">退出</a> </header> <div class="container"> <div class="pointer"><img src="/img/pointer.png" alt="" /></div> <div class="rotate"><img src="/img/jp.png" alt="" /></div> </div> <p class="degree">您的抽奖次数为<a> ${timesLoginM} </a>次</p> <div class="mask"> <ul> <!-- <li>未中奖</li> <li>未中奖</li> <li>未中奖</li> --> </ul> </div> `); //引入第一个script元素 var script1 = document.createElement("script"); script1.type = "text/javascript"; script1.src = "/js/index.js"; //填自己的js路径 $("body").append(script1); //引入第二个script元素 var script2 = document.createElement("script"); script2.type = "text/javascript"; script2.src = "/js/jQuery-1.12.4.js"; //填自己的js路径 $("body").append(script2); //引入第三个script元素 var script3 = document.createElement("script"); script3.type = "text/javascript"; script3.src = "/js/jqueryRotate.js"; //填自己的js路径 $("body").append(script3); }, 2000); } // 设置一个点击状态,如果点击了获取验证码,则设置为true, // 如果点击了btn(下一步)而没有点击获取验证码,则设置为false var codeState = false; // 用户点击发送短信 -- 点击获取验证码 $(".getCode").on("click", (e) => { e.preventDefault(); // 当点击验证码后就获取到用户刚才输入的手机号和密码 phone = $("#phone").val(); password = $("#password").val(); // console.log(phone, password); // 判断手机号格式是否正确 if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else if ( !/^(?![a-z]+$)(?![A-Z]+$)(?!([^(0-9a-zA-Z)])+$)^.{12,16}$/.test(password) ) { // 验证数字,大写字母,小写字母,特殊字符四选三组成的密码强度,且长度在12到16个数之间 $(".message9").trigger("click"); } else { var data = { phone, password, }; // 发送短信 $.ajax("/codeRequire", { type: "post", data, success: function (response) { // console.log(response); // console.log(response.code); // 表示用户已经点击了获取验证码 if (response.code === 304) { // 此用户已注册 $(".message8").trigger("click"); } else { // 验证码发送成功弹窗 $(".message7").trigger("click"); // 验证码获取倒计时 -- 60秒 var codeTime = 60; var timer = setInterval(() => { codeTime--; // 把每次的倒计时都重新渲染到HTML页面 $(".getCode").attr("value", codeTime); // 通过元素覆盖实现禁止点击 $(".coverGetCode").css("display", "block"); if (codeTime <= 0) { // 清除计时器 clearInterval(timer); $(".getCode").attr("value", "重新获取"); $(".coverGetCode").css("display", "none"); } }, 1000); // console.log(codeTime); // 60 // 获取从后端接收到的验证码 codeRes = response.data; console.log("验证码:", codeRes); codeState = true; } }, }); } }); // 用户提交表单 $(".btn").on("click", (e) => { if (codeState === true) { // 阻止默认行为 e.preventDefault(); var data = { phone, password, }; // 提交注册信息 $.ajax("/register", { type: "post", data, success: function (response) { // 获取用户输入的验证码 var codeInput = $("#code").val(); console.log("用户输入的验证码:", codeInput); // 如果用户没有注册 if (response.code === 200) { if (codeInput == codeRes) { // 验证码正确 console.log(response.message); // // 用户注册完毕直接绘制个抽奖页面 // 注册完成后完成登录功能 -- 使用密码登录 // 表单序列化 // 手机验证码登录 $.ajax("/loginM", { type: "post", data, success: function (response) { if (response.code === 200) { $(".message2").trigger("click"); // console.log(response.message); // 用户登录完毕直接绘制个抽奖页面 // window.location = "/"; index(); } else if (response.code === 400) { $(".message3").trigger("click"); // console.log(response.message); } }, }); } else { // 验证码错误 $(".message5").trigger("click"); } } else if (response.code === 400) { $(".message4").trigger("click"); console.log(response.message); } }, }); } else { $(".message6").trigger("click"); } }); });
我们会发现已经实现了注册成功后 2秒钟后 显示用户抽奖界面,并且页面不刷新
-
判断用户如果没有注册的话,直接跳转到用户注册界面(注:页面不刷新)
- 同样是绘制页面,这次绘制的是注册页
// 绘制注册页的函数 function register() { setTimeout(() => { $("body").html(` <button class="message" data-icon="info"></button> <button class="message4" data-icon="info"></button> <button class="message5" data-icon="error"></button> <button class="message6" data-icon="error"></button> <button class="message7" data-icon="success"></button> <button class="message8" data-icon="warning"></button> <button class="message9" data-icon="warning"></button> <header style="width: 176px"> <a href="/login" class="iconfont icon-denglu">登录</a> <a href="/register" class="iconfont icon-zhuce">注册</a> </header> <div class="loginBox"> <h2>注册</h2> <form action="" method="" class="registerForm"> <div class="item"> <input type="text" required id="phone" name="phone" /> <label for="">手机号</label> </div> <div class="item"> <input type="password" required id="password" name="password" /> <label for="">密码</label> </div> <div class="item"> <input type="text" id="code" name="code" required /> <label for="">验证码</label> <input type="button" class="getCode" value="点击获取" /> <input type="button" class="coverGetCode" value="" /> </div> <button type="submit" class="btn" οnclick="return false"> 下一步 <span></span> <span></span> <span></span> <span></span> </button> </form> </div> `); //引入第一个script元素 var script1 = document.createElement("script"); script1.type = "text/javascript"; script1.src = "https://cdn.bootcdn.net/ajax/libs/jquery/1.10.0/jquery.min.js"; //填自己的js路径 $("body").append(script1); //引入第二个script元素 var script2 = document.createElement("script"); script2.type = "text/javascript"; script2.src = "/js/modal_dialog.js"; //填自己的js路径 $("body").append(script2); //引入第三个script元素 var script3 = document.createElement("script"); script3.type = "text/javascript"; script3.src = "/js/tccj_info.js"; //填自己的js路径 $("body").append(script3); //引入第四个script元素 var script4 = document.createElement("script"); script4.type = "text/javascript"; script4.src = "/js/register.js"; //填自己的js路径 $("body").append(script4); }, 2000); } // 用户点击发送短信 -- 点击获取验证码 $(".getCode").on("click", (e) => { e.preventDefault(); // 当点击验证码后就获取到用户刚才输入的手机号和密码 phone = $("#phone").val(); // console.log(phone, password); // 判断手机号格式是否正确 if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else { var data = { phone, }; // 发送短信 $.ajax("/code", { type: "post", data, success: function (response) { // console.log(response); console.log(response.code); // 表示用户已经点击了获取验证码 if (response.code === 400) { console.log(response.datas); // 此用户未注册 $(".message1").trigger("click"); // 用户登录完毕直接绘制个抽奖页面 register(); } else { // 验证码发送成功弹窗 $(".message7").trigger("click"); // 验证码获取倒计时 -- 60秒 var codeTime = 60; var timer = setInterval(() => { codeTime--; // 把每次的倒计时都重新渲染到HTML页面 $(".getCode").attr("value", codeTime); // 通过元素覆盖实现禁止点击 $(".coverGetCode").css("display", "block"); if (codeTime <= 0) { // 清除计时器 clearInterval(timer); $(".getCode").attr("value", "重新获取"); $(".coverGetCode").css("display", "none"); } }, 1000); // console.log(codeTime); // 60 // 获取从后端接收到的验证码 codeRes = response.data; console.log("验证码:", codeRes); codeState = true; } }, }); } });
我们会发现用户如果没有注册的话,直接跳转到用户注册界面,并且页面不刷新
效果如下:
-
登录成功后显示用户抽奖界面(注:页面不刷新)
- 同样也是绘制页面,只不过这次是绘制抽奖界面
// 绘制首页的函数 function index() { setTimeout(() => { $("body").html(` <link rel="stylesheet" href="/css/index.css" /> <header> <a href="/" class="iconfont icon-shouye">首页</a> <a href="/login" class="iconfont icon-denglu">登录</a> <a href="/quit" class="iconfont icon-tuichu_huaban1">退出</a> </header> <div class="container"> <div class="pointer"><img src="/img/pointer.png" alt="" /></div> <div class="rotate"><img src="/img/jp.png" alt="" /></div> </div> <p class="degree">您的抽奖次数为<a> ${timesLoginM} </a>次</p> <div class="mask"> <ul> <!-- <li>未中奖</li> <li>未中奖</li> <li>未中奖</li> --> </ul> </div> `); //引入第一个script元素 var script1 = document.createElement("script"); script1.type = "text/javascript"; script1.src = "/js/index.js"; //填自己的js路径 $("body").append(script1); //引入第二个script元素 var script2 = document.createElement("script"); script2.type = "text/javascript"; script2.src = "/js/jQuery-1.12.4.js"; //填自己的js路径 $("body").append(script2); //引入第三个script元素 var script3 = document.createElement("script"); script3.type = "text/javascript"; script3.src = "/js/jqueryRotate.js"; //填自己的js路径 $("body").append(script3); }, 2000); } // 用户提交表单--验证码登录 $(".codeBtn").on("click", (e) => { if (codeState === true) { // 阻止默认行为 e.preventDefault(); // 判断手机号格式是否正确 phone = $("#phone").val(); if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else { // 手机号格式正确 // 表单序列化 // var data = $(".codeForm").serializeArray(); var data = { phone, }; // 手机验证码登录 $.ajax("/loginY", { type: "post", data, success: function (response) { console.log(response.code); if (response.code === 200) { // console.log(response.message); // 提示用户登录成功弹窗 $(".message2").trigger("click"); // 直接绘制个首页 index(); } else if (response.code === 400) { $(".message1").trigger("click"); console.log(response.message); } }, }); } } else { $(".message6").trigger("click"); } }); // 密码登录 $(".pwdBtn").on("click", (e) => { e.preventDefault(); // 判断手机号格式是否正确 phone = $("#phone2").val(); if (!/^1[3456789]\d{9}$/.test(phone)) { // 提示错误信息 $(".message").trigger("click"); return false; } else { // 手机号格式正确 // 表单序列化 var data = $(".pwdForm").serializeArray(); // 密码登录 $.ajax("/loginM", { type: "post", data, success: function (response) { if (response.code === 200) { $(".message2").trigger("click"); // console.log(response.message); // 用户登录完毕直接绘制个抽奖页面 // window.location = "/"; timesLoginM = response.data.times; index(); } else if (response.code === 400) { $(".message3").trigger("click"); // console.log(response.message); } }, }); } });
我们会发现登录成功后显示用户抽奖界面,并且页面是不会刷新的
效果如下:
至此,项目已基本完成。