通过继承Thread类创建
/**
* @description: 通过继承Thread类创建线程
* @author: Cyril
* @create: 2019/10/22
*/
public class ThreadDemo extends Thread {
/**
* 重写run方法
*/
@Override
public void run() {
System.out.println("通过继承Thread类创建线程");
}
public static void main(String[] args) {
System.out.println("创建线程");
ThreadDemo threadDemo = new ThreadDemo();
System.out.println("启动线程");
threadDemo.start();
}
}
通过实现Runnable接口创建
/**
* @description: Runnable接口创建线程
* @author: Cyril
* @create: 2019/10/22
*/
public class RunnableDemo implements Runnable {
/**
* 重写run方法
*/
@Override
public void run() {
System.out.println("通过实现Runnable接口创建线程");
}
public static void main(String[] args) {
System.out.println("实现Runnable接口");
RunnableDemo runnableDemo = new RunnableDemo();
System.out.println("创建线程");
Thread thread = new Thread(runnableDemo);
System.out.println("启动线程");
thread.start();
}
}
通过Callable创建
- 实现Callable接口,相对于实现Runnable接口的方式,Callable接口可以有返回值,并且可以抛出异常。
- 需要FutureTask对象的支持,接收运算结果。
/**
* @description: Callable接口创建线程
* @author: Cyril
* @create: 2019/10/23
*/
public class CallableDemo {
public static void main(String[] args) {
TestCallableDemo testCallableDemo = new TestCallableDemo();
//执行FutureTask
FutureTask task = new FutureTask<Integer>(testCallableDemo);
Thread thread = new Thread(task);
//启动线程
thread.start();
try {
//获取返回结果
Integer sum = (Integer) task.get();
System.out.println("求和值为:" + sum);
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
/**
* 实现Callable接口
*/
static class TestCallableDemo implements Callable<Integer> {
@Override
public Integer call() throws Exception {
int count = 0;
for (int i = 0; i <= 1000; i++) {
count += i;
}
return count;
}
}
}
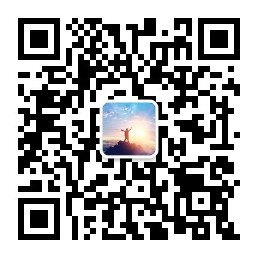