跟调用WebServices一样,这里写下两种方式。
- 添加服务引用
通过服务契约接口和服务地址调用
一、添加服务应用
第一步:右键引用,选择添加服务引用
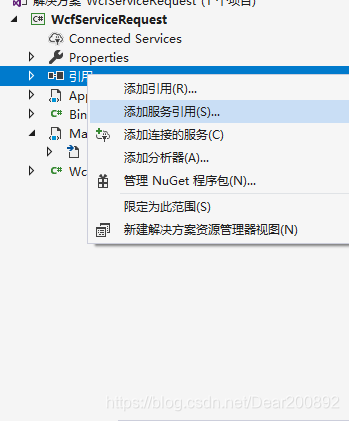
第二步:输入服务引用地址
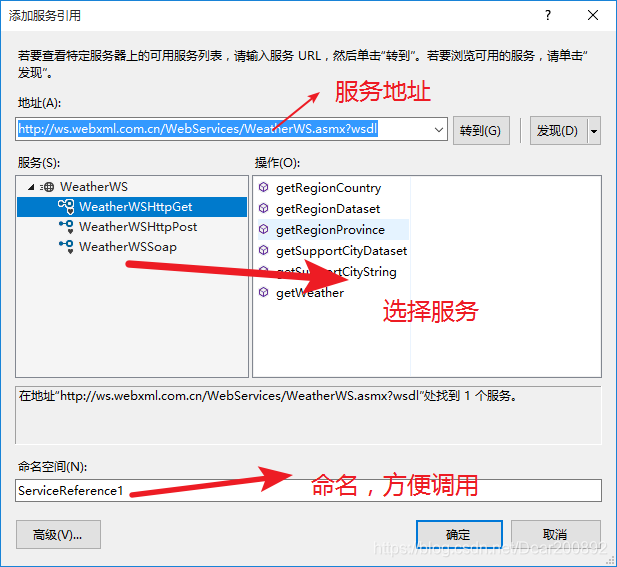
点击确定后,解决方案下面就会自动生成两个文件
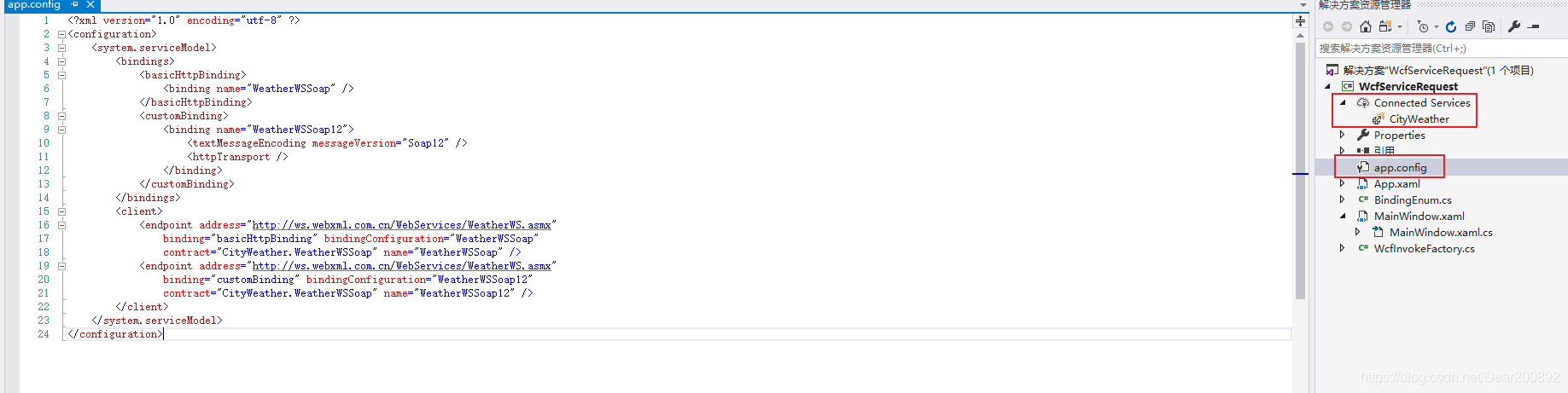
第三步:调用服务
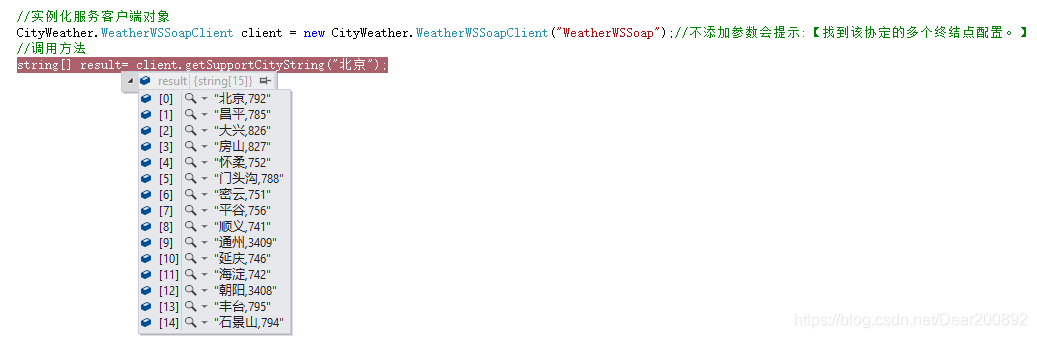
二、服务契约
这种方式比较简单,因为不需要任何配置文件就可解决,只需知道服务地址就可以调用。
第一步:创建参数请求界面(可省略)
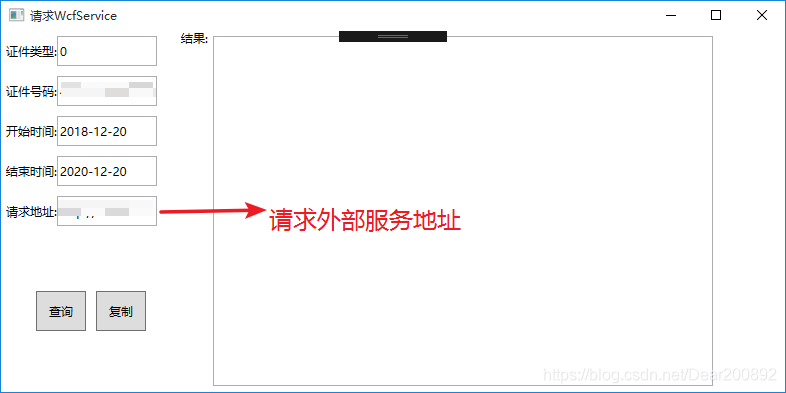
其余四个文本框为请求参数,我这里是要编写一个测试程序给别人调用服务。
第二步:编写WCF服务工厂
public class WcfInvokeFactory
{
#region WCF服务工厂
/// <summary>
/// 通过URL创建WCF服务
/// </summary>
/// <typeparam name="T">服务</typeparam>
/// <param name="bing">通信类型</param>
/// <param name="url">WCF服务路径</param>
/// <returns></returns>
public static T CreateServiceByUrl<T>(BindingEnum bing, string url)
{
try
{
if (string.IsNullOrEmpty(url)) throw new NotSupportedException("请求地址不能为空!");
EndpointAddress address = new EndpointAddress(url);
Binding binding = CreateBinding(bing);
ChannelFactory<T> factory = new ChannelFactory<T>(binding, address);
return factory.CreateChannel();
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
}
/// <summary>
/// 通过URL创建WCF服务
/// </summary>
/// <typeparam name="T">服务</typeparam>
/// <param name="bing">通信类型</param>
/// <param name="url">WCF服务路径</param>
/// <param name="methodName">请求方法</param>
/// <param name="arg">请求方法参数</param>
/// <returns></returns>
public static object CreateServiceByUrl<T>(BindingEnum bing, string url,string methodName, params object[] arg)
{
try
{
if (string.IsNullOrEmpty(url)) throw new NotSupportedException("请求地址不能为空!");
EndpointAddress address = new EndpointAddress(url);
Binding binding = CreateBinding(bing);
using (ChannelFactory<T> factory = new ChannelFactory<T>(binding, address))
{
T instance = factory.CreateChannel();
using (instance as IDisposable)
{
Type type = typeof(T);
System.Reflection.MethodInfo method = type.GetMethod(methodName);
return method.Invoke(instance, arg);
}
}
}
catch (Exception ex)
{
throw new Exception(ex.Message);
}
}
#endregion
#region 创建传输协议
/// <summary>
/// 创建传输协议
/// </summary>
/// <param name="binding">传输协议名称</param>
/// <returns></returns>
private static Binding CreateBinding(BindingEnum bing)
{
Binding bindinginstance = null;
switch (bing)
{
case BindingEnum.BasicHttpBinding://出现异常:远程服务器返回错误: (415) Cannot process the message because the content type 'text/xml; charset=utf-8' was not the expected type 'application/soap+xml; charset=utf-8'.。
BasicHttpBinding bhb = new BasicHttpBinding();
bhb.Security.Mode = BasicHttpSecurityMode.None;
bhb.MaxBufferSize = 2147483647;
bhb.MaxBufferPoolSize = 2147483647;
bhb.MaxReceivedMessageSize = 2147483647;
bhb.ReaderQuotas.MaxStringContentLength = 2147483647;
bhb.CloseTimeout = new TimeSpan(0, 30, 0);
bhb.OpenTimeout = new TimeSpan(0, 30, 0);
bhb.ReceiveTimeout = new TimeSpan(0, 30, 0);
bhb.SendTimeout = new TimeSpan(0, 30, 0);
bindinginstance = bhb;
break;
case BindingEnum.NetTcpBinding:
NetTcpBinding ntb = new NetTcpBinding();
ntb.MaxReceivedMessageSize = 65535000;
ntb.Security.Mode = SecurityMode.None;
bindinginstance = ntb;
break;
case BindingEnum.WSHttpBinding:
WSHttpBinding wshb = new WSHttpBinding(SecurityMode.None);
wshb.TransactionFlow = true;
wshb.MaxReceivedMessageSize = 2147483646;
wshb.MaxBufferPoolSize = 2147483646;
wshb.ReaderQuotas.MaxDepth = 32;
wshb.ReaderQuotas.MaxStringContentLength = 52428800;
wshb.ReaderQuotas.MaxArrayLength = 52428800;
wshb.ReaderQuotas.MaxBytesPerRead = 52428800;
wshb.ReaderQuotas.MaxNameTableCharCount = 16384;
wshb.Security.Message.ClientCredentialType = System.ServiceModel.MessageCredentialType.Windows;
wshb.Security.Transport.ClientCredentialType = System.ServiceModel.HttpClientCredentialType.Windows;
bindinginstance = wshb;
break;
default:
break;
}
return bindinginstance;
}
#endregion
}
public enum BindingEnum
{
BasicHttpBinding = 0,
NetTcpBinding = 1,
WSHttpBinding = 2
}
第三步:调用服务方法

图片中的的msg为请求方法的参数。