演示
实例一
- 引入依赖
import cn.hutool.core.date.LocalDateTimeUtil;
- 若比较时间中,结束时间早于开始时间,则返回负数
LocalDateTime start= act.getGmtCreate(); //开始时间
LocalDateTime end= LocalDateTime.now(); //结束时间
Duration betweenCreate = LocalDateTimeUtil.between(start, end);
Long betweenL = betweenCreate.toHours();//转化成小时差, 也可转化成毫秒,分钟差
int count = betweenL.intValue(); //相差的时间数
LocalDate now = LocalDate.now();
System.out.println(now);
LocalDateTime stDate = LocalDateTime.of(now, LocalTime.MIN);
LocalDateTime endDate = LocalDateTime.of(now, LocalTime.MAX);
System.out.println(stDate);
System.out.println(endDate);
- 输出结果
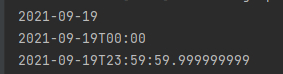
实例二
- 引入依赖
import cn.hutool.core.date.DateTime;
- 需求:
- 传参: 字符串
02:00:00
- 返回当天该时间前一小时段的2个字符时间数据:
2023-02-28 01:00:01
, 2023-02-28 02:00:00
DateTime dateTime = DateUtil.parseTimeToday("02:00:00");
DateTime time = DateUtil.offsetMinute(dateTime, -59);
DateTime dateTime1 = DateUtil.offsetSecond(time, -59);
System.out.println(dateTime.toString());
System.out.println(time.toString());
System.out.println(dateTime1.toString());
- 输出
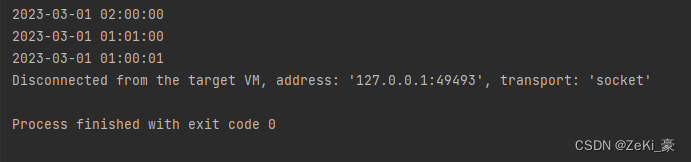
实例三
long now = System.currentTimeMillis();
String currentTime = com.universe.titan.test.util.DateUtil.formatDateyyyyMMddHHmmss(now);
String beginTime = currentTime.substring(0,8)+"000000";
String endTime = currentTime.substring(0,8)+"235959";
System.out.println(beginTime);
System.out.println(endTime);
public class DateUtil {
private static final Logger LOGGER = LoggerFactory.getLogger(DateUtil.class);
private static final int[] leapDays = { 31, 29, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
private static final int[] commDays = { 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31 };
public static final String PTN_YYYYMMDDHHMMSSSS = "yyyy-MM-dd HH:mm:ss";
//此格式 大小写不可改动
public static final String PTN_YYMMDDHHMM = "yyMMddHHmm";
public static final String PTN_MMDDHHMMSS = "MMddHHmmss";
public static final String PTN_YYYY_MM_DD = "yyyy-MM-dd";
public static final String GMT8 = "GMT+8";
public static final SimpleDateFormat usFormat = new SimpleDateFormat("EEE MMM dd HH:mm:ss z yyyy",Locale.US);
/**
* 数字格式的日期时间字符串, yyyyMMddHHmmss.
*/
public static final String NUMBER_DATE_TIME_FORMAT = "yyyyMMddHHmmss";
/**
* 默认的日期时间格式,yyyy-MM-dd' 'HH:mm:ss.
*/
public static final String ISO_DATE_TIME_FORMAT = "yyyy-MM-dd HH:mm:ss";
public static String formatDateyyyyMMddHHmmss(long time) {
return formatDate(time, NUMBER_DATE_TIME_FORMAT);
}
private static String formatDate(long time, String pattern) {
return DateFormatUtils.format(time, pattern);
}
public static String formatDate(Date srcDate, String pattern) {
if (srcDate == null) {
return null;
}
return org.apache.commons.lang3.time.DateFormatUtils.format(srcDate, pattern);
}
public static String getDateTime() {
return formatDate(new Date(), ISO_DATE_TIME_FORMAT);
}
/**
* 格林威治时间字符串 转化为Date
* @param date
* @return
* @throws Exception
*/
public static Date getUsDate(String date) {
try {
return usFormat.parse(date);
} catch (ParseException e) {
return new Date(date);
}
}
/**
* 根据月份获取天数
*/
public static int getDaysOfMonth(String month) {
String[] a = month.split("-");
int y = Integer.parseInt(a[0]);
int m = Integer.parseInt(a[1]);
if (isLeapYear(y)) {
return leapDays[m - 1];
}else {
return commDays[m - 1];
}
}
/**
* 是否为闰年
*/
public static boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
/**
* 取得当前时间
*
* @return 返回格式:yyyy-MM-dd HH:mm:ss
*/
public static String getCurrentDateTime() {
Date d = new Date();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");// 设置日期格式
return df.format(d);
}
/**
* 两个时间之间的天数
*
* @param date1
* @param date2
* @return
*/
public static long getDays(String date1, String date2) {
if (date1 == null || date1.equals("")) {
return 0;
}
if (date2 == null || date2.equals("")){
return 0;
}
// 转换为标准时间
SimpleDateFormat myFormatter = new SimpleDateFormat("yyyy-MM-dd");
Date date = null;
Date mydate = null;
try {
date = myFormatter.parse(date1);
mydate = myFormatter.parse(date2);
} catch (Exception e) {
}
long day = (date.getTime() - mydate.getTime()) / (24 * 60 * 60 * 1000);
return day;
}
/**
*
* @Description:取得两个时间相差秒数
* @param date1
* @param date2
* @return
* @return:long
*/
public static long getDiffer(Date date1, Date date2) {
return (date1.getTime() - date2.getTime()) / 1000;
}
/**
* 获取今天还剩多少时间 ,毫秒值
*
* @return
* @throws ParseException
*/
public static long getTodayEnd() throws ParseException {
String endData = getCurrentDate() + " 23:59:59";
SimpleDateFormat sf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
long time = sf.parse(endData).getTime();
return time - System.currentTimeMillis();
}
/**
*
* @Description:取得当前日期
* @return:String 格式:yyyy-MM-dd
*/
public static String getCurrentDate() {
Date d = new Date();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");// 设置日期格式
return df.format(d);
}
public static Date strToDate(String dateStr) throws ParseException {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");// 设置日期格式
return df.parse(dateStr);
}
public static Date strToDateErrUseCurrentDate(String dateStr) {
Date d;
try {
if (dateStr == null){
d = new Date();
}else {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");// 设置日期格式
d = df.parse(dateStr);
}
}catch (Exception e){
d = new Date();
}
return d;
}
public static Date strToDate(String dateStr, String format) throws ParseException {
SimpleDateFormat df = new SimpleDateFormat(format);// 设置日期格式
return df.parse(dateStr);
}
/**
* 获取昨天日期 格式:yyyy-MM-dd
*
* @return
*/
public static String getYesterday() {
Date d = new Date(System.currentTimeMillis() - 86400000);
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");// 设置日期格式
return df.format(d);
}
/**
* 时间格式化
*
* @param date
* @return yyyy-MM-dd
*/
public static String format(Date date) {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");// 设置日期格式
return df.format(date);
}
/**
* 时间格式化
*
* @param date
* @return yyyy-MM-dd HH:mm:ss
*/
public static String format1(Date date) {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");// 设置日期格式
return df.format(date);
}
/**
* 获取这个时间的周一
*
* @param date
* @return
*/
public static Date getThisWeekMonday(Date date) {
Calendar cal = Calendar.getInstance();
cal.setTime(date);
// 获得当前日期是一个星期的第几天
int dayWeek = cal.get(Calendar.DAY_OF_WEEK);
if (1 == dayWeek) {
cal.add(Calendar.DAY_OF_MONTH, -1);
}
// 设置一个星期的第一天,按中国的习惯一个星期的第一天是星期一
cal.setFirstDayOfWeek(Calendar.MONDAY);
int day = cal.get(Calendar.DAY_OF_WEEK);
// 根据日历的规则,给当前日期减去星期几与一个星期第一天的差值
cal.add(Calendar.DATE, cal.getFirstDayOfWeek() - day);
return cal.getTime();
}
/**
* 获取本周周一时间
*
* @return
*/
public static String getThisWeekMonday() {
return format(getThisWeekMonday(new Date()));
}
/**
*
* @Description:获得当前时间,毫秒
* @return
* @return:long
*/
public static long currentTimeMillis() {
return System.currentTimeMillis();
}
/**
*
* @Description:去掉日期后面毫秒.0
* @param str
* @return
* @return:String
*/
public static String formatDate(String str) {
String result = "";
try {
if ("".equals(str) || "null".equals(str) || null == str) {
return result;
} else {
result = str.lastIndexOf(".0") != -1 ? str.substring(0, str.lastIndexOf(".0")) : str;
}
} catch (Exception e) {
// TODO: handle exception
}
return result;
}
/**
*
* @Description:获取时间的字符串形式
* @param date
* @param format
* @return:String
*/
public static String getDateStr(Date date, String format) {
if (date == null) {
return "";
}
DateFormat fmt = new SimpleDateFormat(format);
return fmt.format(date);
};
/**
*
* @Description:是否是正确的时间格式
* @param str yyyy-MM-dd
* @return:boolean
*/
public static boolean isDateStr(String str) {
DateFormat format = new SimpleDateFormat("yyyy-MM-dd");
try {
Date date = format.parse(str);
return str.equals(format.format(date));
} catch (ParseException e) {
return false;
}
}
/**
* 是否是正确的时间格式
* @param str yyyy-MM-dd HH:mm:ss
* @return 正确返回true,错误返回false
*/
public static boolean isDateTimeStr(String str) {
DateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
Date date = format.parse(str);
return str.equals(format.format(date));
} catch (ParseException e) {
return false;
}
}
/**
* 添加秒数
* @param date 时间
* @param s 添加多少秒,单位:秒
* @return
*/
public static Date addSecond(Date date,int s) {
long ms = s*1000;
long time = date.getTime() + ms;
return new Date(time);
}
/**
* 字符串转时间类型
*/
public static Date getDate(String date, String format) {
DateFormat fmt = new SimpleDateFormat(format);
try {
return fmt.parse(date);
} catch (ParseException e) {
new RuntimeException(e);
}
return null;
};
/**
* 获得前后几天的时间
*/
public static Date addDays(Date date, int days) {
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.add(Calendar.DAY_OF_MONTH, days);
Date afterDate = calendar.getTime();
return afterDate;
};
/**
* 获取前后几天的时间
* @param number
* @return yyyy-MM-dd
*/
public static String byNumberFindDate(Integer number) {
Date addDays = addDays(new Date(), number);
return format(addDays);
}
public static List<String> findDates(String dStart, String dEnd) throws ParseException {
List<String> data = new ArrayList<String>();
DateFormat format = new SimpleDateFormat("yyyy-MM-dd");
Calendar cStart = Calendar.getInstance();
cStart.setTime(format.parse(dStart));
// 别忘了,把起始日期加上
data.add(dStart);
// 此日期是否在指定日期之后
while (format.parse(dEnd).after(cStart.getTime())) {
// 根据日历的规则,为给定的日历字段添加或减去指定的时间量
cStart.add(Calendar.DAY_OF_MONTH, 1);
data.add(format(cStart.getTime()));
}
return data;
}
/**
* 获得当前日期时间
*/
public static Date getCurrDay() {
return getDate(getDateStr(new Date(), "yyyyMMdd"), "yyyyMMdd");
};
/**
* 取得当前月份
*
* @return 返回格式:yyyy-MM
*/
public static String getCurrMonthTime() {
Date d = new Date();
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM");// 设置日期格式
return df.format(d);
}
/**
* 给定一个日期型字符串,返回加减n天后的日期型字符串
*
* @param dateStr 给定的字符型日期
* @param n n天后日期
* @return
*/
public static String nDaysAfterOneDateString(String dateStr, int n) {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
Date tmpDate = null;
try {
tmpDate = df.parse(dateStr);
} catch (Exception e) {
//LogUtil.error(LOGGER, "日期转换错误:" + e.getMessage(), e);
}
long nDay = (tmpDate.getTime() / (24 * 60 * 60 * 1000) + 1 + n) * (24 * 60 * 60 * 1000);
tmpDate.setTime(nDay);
return df.format(tmpDate);
}
/**
*
* @Description:取得当前月下一个月
* @return
* @return:String
*/
public static String getAfterMonth() {
Calendar cal = Calendar.getInstance();
cal.add(Calendar.MONTH, 1);
SimpleDateFormat dft = new SimpleDateFormat("yyyyMM");
String lastMonth = dft.format(cal.getTime());
return lastMonth;
}
/**
* @Description: 根据提供的年月日获取该月份的第一天
*/
public static String getBeginDayofMonth(Date date, String format) {
date.getTime();
Calendar startDate = Calendar.getInstance();
startDate.setTime(date);
startDate.set(Calendar.DAY_OF_MONTH, 1);
startDate.set(Calendar.HOUR_OF_DAY, 0);
startDate.set(Calendar.MINUTE, 0);
startDate.set(Calendar.SECOND, 0);
startDate.set(Calendar.MILLISECOND, 0);
Date firstDate = startDate.getTime();
SimpleDateFormat myFmt7 = new SimpleDateFormat(format);
return myFmt7.format(firstDate);
}
/**
* @Description: 根据提供的年月获取该月份的最后一天
*/
public static String getEndDayofMonth(Date date, String format) {
Calendar startDate = Calendar.getInstance();
startDate.setTime(date);
startDate.set(Calendar.DAY_OF_MONTH, startDate.getActualMaximum(Calendar.DAY_OF_MONTH));
startDate.set(Calendar.HOUR_OF_DAY, 23);
startDate.set(Calendar.MINUTE, 59);
startDate.set(Calendar.SECOND, 59);
startDate.set(Calendar.MILLISECOND, 999);
Date firstDate = startDate.getTime();
SimpleDateFormat myFmt7 = new SimpleDateFormat(format);
return myFmt7.format(firstDate);
}
/**
* @Description: 返回小时
* @param date 日期
* @return 返回小时
*/
public static int getHour(Date date) {
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
return calendar.get(Calendar.HOUR_OF_DAY);
}
/**
* @Description: 返回当前时间的小时数
* @return 返回小时
*/
public static int getCurrentDateHour() {
return getHour(new Date());
}
/**
* @Description: 返回当前时间的是哪一天
* @return 返回小时
*/
public static int getCurrentDateDay() {
Calendar calendar = Calendar.getInstance();
return calendar.get(Calendar.DAY_OF_MONTH);
}
/**
* 获取指定时间对应的毫秒数
*
* @param time "HH:mm:ss"
* @return
*/
public static long getTimeMillis(String time) {
try {
DateFormat dateFormat = new SimpleDateFormat("yy-MM-dd HH:mm:ss");
DateFormat dayFormat = new SimpleDateFormat("yy-MM-dd");
Date curDate = dateFormat.parse(dayFormat.format(new Date()) + " " + time);
return curDate.getTime();
} catch (ParseException e) {
//LogUtil.error(LOGGER, "获取指定时间对应的毫秒数错误:" + e.getMessage(), e);
}
return 0;
}
/**
* 常用的简单日期格式 yyyy-MM-dd HH:mm:ss 可兼容 yyyy-MM-dd, 但是天必须有,天之后的不足将自动补充0
*
* @param dateStr
* @return
*/
public static Date parseSimpleDate(String dateStr) {
String[] arr = dateStr.split(" ");
if (arr.length == 1) {
dateStr = dateStr + " 00:00:00";
} else {
String[] arr2 = arr[1].split(":");
if (arr2.length == 1) {
dateStr = dateStr + ":00:00";
} else if (arr2.length == 2) {
dateStr = dateStr + ":00";
}
}
return parseDate(dateStr, PTN_YYYYMMDDHHMMSSSS);
}
/**
* 格式化日期字符串,返回日期对象
*
* @param dateString 日期字符串
* @return 格式化后的日期对象
*/
public static Date parseDate(String dateString, String pattern) {
SimpleDateFormat sdf = DateFormatHolder.formatFor(pattern);
try {
return sdf.parse(dateString);
} catch (ParseException e) {
throw new RuntimeException("incorrect date format string.");
}
}
/**
* 格式化日期字符串,返回日期字符串
*
* @param dateString 日期字符串
* @return 格式化后的日期字符串
*/
public static String parseDateStr(String dateString, String pattern) {
SimpleDateFormat sdf = DateFormatHolder.formatFor(pattern);
return sdf.format(dateString);
}
/**
* 判断一个字符串是不是日期格式
* @param str
* @return 是:就返回日期字符串 不是:就返回空
*/
public static String isDateTime(String str){
DateTimeFormatter ldt = DateTimeFormatter.ofPattern(PTN_YYYYMMDDHHMMSSSS.replace("y", "u"))
.withResolverStyle(ResolverStyle.STRICT);
try {
LocalDate.parse(str, ldt);
} catch (DateTimeParseException | NullPointerException e) {
return null;
}
return str;
}
/**
* A factory for {@link SimpleDateFormat}s. The instances are stored in a
* threadlocal way because SimpleDateFormat is not threadsafe as noted in
* {@link SimpleDateFormat its javadoc}.
*
*/
final static class DateFormatHolder {
private static final ThreadLocal<SoftReference> THREADLOCAL_FORMATS = new ThreadLocal<SoftReference>() {
@Override
protected SoftReference<Map> initialValue() {
return new SoftReference<Map>(new HashMap<String, SimpleDateFormat>());
}
};
/**
* creates a {@link SimpleDateFormat} for the requested format string.
*
* @param pattern a non-<code>null format String according to
* {@link SimpleDateFormat}. The format is not checked against
* <code>null since all paths go through {@link DateUtil}.
* @return the requested format. This simple dateformat should not be used to
* {@link SimpleDateFormat#applyPattern(String) apply} to a different
* pattern.
*/
public static SimpleDateFormat formatFor(String pattern) {
SoftReference<Map> ref = THREADLOCAL_FORMATS.get();
Map<String, SimpleDateFormat> formats = ref.get();
if (formats == null) {
formats = new HashMap<String, SimpleDateFormat>();
THREADLOCAL_FORMATS.set(new SoftReference<Map>(formats));
}
SimpleDateFormat format = formats.get(pattern);
if (format == null) {
format = new SimpleDateFormat(pattern);
formats.put(pattern, format);
}
return format;
}
}
/**
*
* @Description:时间字符串转DATE("EEE MMM dd HH:mm:ss z yyyy")
* @param timeStr 时间字符串
* @param format 时间格式
* @param locale 时区
* @return Date
*/
public static Date str2Date(String timeStr, String format, Locale locale) {
SimpleDateFormat dateFormat = new SimpleDateFormat(format, locale);
try {
return dateFormat.parse(timeStr);
} catch (ParseException e) {
e.printStackTrace();
}
return null;
}
/**
*
* yyyy-MM-dd HH:mm:ss 格式时间字符串 转为 YYMMDDHHMM 字符串
* @param dateTimeStr 时间字符串
* @return Date
*/
public static String dateTimeStr2yyMMdhhmmStr(String dateTimeStr) {
try {
if (StringUtils.isEmpty(dateTimeStr)){
return null;
}
Date date = DateUtil.parseDate(dateTimeStr, DateUtil.PTN_YYYYMMDDHHMMSSSS);
DateTimeFormatter df4 = DateTimeFormatter.ofPattern(DateUtil.PTN_YYMMDDHHMM);
Instant instant = date.toInstant();
ZoneId zone = ZoneId.systemDefault();
LocalDateTime localDateTime = LocalDateTime.ofInstant(instant, zone);date.toInstant();
String s = localDateTime.format(df4);
return s;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
/**
*
* mmddhhmmss 格式时间字符串 转为 毫秒时间戳
* ps:月日时分秒字符串转 毫秒时间戳
* @param mmddhhmmssStr 时间字符串
* @return long
*/
public static Long mmddhhmmss2TimeMillisStr(String mmddhhmmssStr) {
try {
if (StringUtils.isEmpty(mmddhhmmssStr)){
return System.currentTimeMillis();
}
Calendar calendar = Calendar.getInstance();
calendar.set(Calendar.MONTH,Integer.valueOf(mmddhhmmssStr.substring(0,2))-1);
calendar.set(Calendar.DAY_OF_MONTH,Integer.valueOf(mmddhhmmssStr.substring(2,4)));
calendar.set(Calendar.HOUR_OF_DAY,Integer.valueOf(mmddhhmmssStr.substring(4,6)));
calendar.set(Calendar.MINUTE,Integer.valueOf(mmddhhmmssStr.substring(6,8)));
calendar.set(Calendar.SECOND,Integer.valueOf(mmddhhmmssStr.substring(8,10)));
System.out.println(DateUtil.format1(calendar.getTime()));
return calendar.getTimeInMillis();
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
/**
*
* mmddhhmmss 格式时间字符串 转为 毫秒时间戳 20210207182214
* ps:月日时分秒字符串转 毫秒时间戳
* @param yyyymmddhhmmssStr 时间字符串
* @return long
*/
public static Long yyyyMmDdHhmmss2TimeMillisStr(String yyyymmddhhmmssStr) {
try {
if (StringUtils.isEmpty(yyyymmddhhmmssStr)){
return System.currentTimeMillis();
}
//20210207182214
Calendar calendar = Calendar.getInstance();
calendar.set(Calendar.YEAR,Integer.valueOf(yyyymmddhhmmssStr.substring(0,4)));
calendar.set(Calendar.MONTH,Integer.valueOf(yyyymmddhhmmssStr.substring(4,6))-1);
calendar.set(Calendar.DAY_OF_MONTH,Integer.valueOf(yyyymmddhhmmssStr.substring(6,8)));
calendar.set(Calendar.HOUR_OF_DAY,Integer.valueOf(yyyymmddhhmmssStr.substring(8,10)));
calendar.set(Calendar.MINUTE,Integer.valueOf(yyyymmddhhmmssStr.substring(10,12)));
calendar.set(Calendar.SECOND,Integer.valueOf(yyyymmddhhmmssStr.substring(12,14)));
return calendar.getTimeInMillis();
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
//计算相差天数
public static Integer differentDays(Long currentTime, Long beforeTime) {
//try {
// long between = cn.hutool.core.date.DateUtil.between(new Date(beforeTime), new Date(currentTime), DateUnit.DAY);
// long between1 = cn.hutool.core.date.DateUtil.between(
// cn.hutool.core.date.DateUtil.parse(cn.hutool.core.date.DateUtil.format(new Date(currentTime), "yyyy-MM-dd")).toJdkDate(),
// cn.hutool.core.date.DateUtil.parse(cn.hutool.core.date.DateUtil.format(new Date(beforeTime), "yyyy-MM-dd")).toJdkDate(), DateUnit.DAY);
Long between = cn.hutool.core.date.DateUtil.betweenDay(new Date(beforeTime), new Date(currentTime), true);
return between.intValue();
}
/**
* UTC时间转化为北京时间
* @param str UTC时间,格式为 YYMMDDhhmmsstnnp,具体见文档,北京时间为东八区,即nnp为32+
* YY 年份的最后2位(00-99)
* MM 月份(01-12)
* DD 日(01-31)
* hh 小时(00-23)
* mm 分(00-59)
* ss 秒(00-59)
* t 十分之一秒(0-9)
* nn 本地时间(如前13个二进制八位数所示)与UTC(世界时常数)时间(00-48)之间的时间差(以一刻钟为单位)。
* p
* + 时间超前于UTC time.
* - 时间超前于UTC time.
* R 本地时间相对于当前的SMSC时间。
* 对于千年问题,采用时间窗口的方法来解决,具体方法为YY>90解释为19YY;否则解释为20YY。
* @return 北京时间,格式为yyyy-MM-dd HH:mm:ss.S
*/
public static String utcTime2BJS(String str) {
try {
String YY = str.substring(0,2);
String nn = str.substring(13,15);
String p = str.substring(15);
// 年份前缀
String pre = Integer.parseInt(YY) > 90 ? "19" : "20";
// 时间字符串转date
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHHmmssSSS");
Calendar cal = Calendar.getInstance();
Date date = sdf.parse(pre + str.substring(0, 13));
cal.setTime(date);
if (!"R".equals(p)) {
// Integer.parseInt(p.equals("-") ? p + nn : nn) 为当地时区时间
// 32 - Integer.parseInt(p.equals("-") ? p + nn : nn)转为当地时间间隔北京时间的时区数
cal.add(Calendar.MINUTE, (32 - Integer.parseInt(p.equals("-") ? p + nn : nn)) * 15);
}
return new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.S").format(cal.getTime());
} catch (ParseException e) {
e.printStackTrace();
return "";
}
}
public static void main(String[] args) {
//System.out.println(mmddhhmmss2TimeMillisStr("0426152333"));
System.out.println(utcTime2BJS("210430123417402+"));
}
}
- 输出
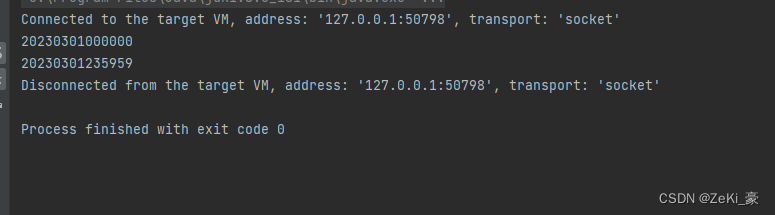