文章目录
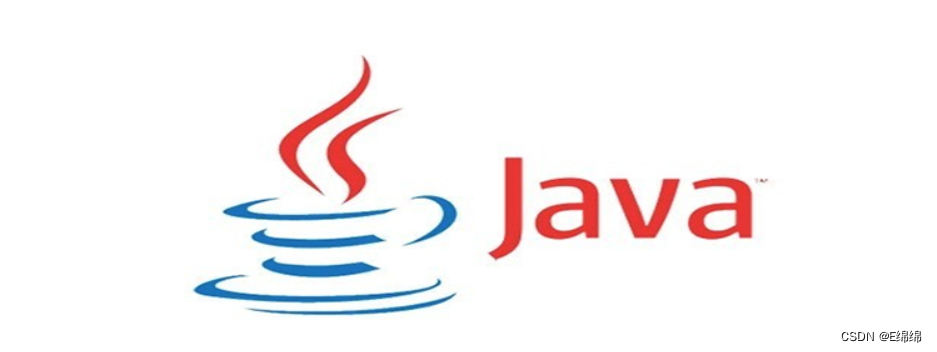
引言
文件上传和下载是Web应用程序中常见的需求。在现代应用中,用户需要上传各种类型的文件,如图片、文档、视频等,或者下载生成的报告和数据文件。SpringBoot通过其强大的生态系统和简化的配置,能够高效地实现文件上传和下载功能。本文将详细介绍如何使用SpringBoot实现这一功能,并讨论相关的安全性和最佳实践。
项目初始化
首先,我们需要创建一个SpringBoot项目。可以通过Spring Initializr快速生成一个项目,添加所需的依赖项。
添加依赖
在pom.xml
中添加以下依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
</dependency>
配置文件存储位置
为了方便管理上传的文件,我们需要在项目中配置文件存储的位置。可以在application.properties
文件中进行配置:
file.upload-dir=/tmp/uploads
这将文件上传目录设置为/tmp/uploads
,你也可以根据需要更改为其他路径。
实现文件上传功能
创建文件上传控制器
创建一个控制器类,用于处理文件上传请求。
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import java.io.File;
import java.io.IOException;
@RestController
public class FileUploadController {
@Value("${file.upload-dir}")
private String uploadDir;
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file,
RedirectAttributes redirectAttributes) {
if (file.isEmpty()) {
return "Please select a file to upload.";
}
try {
File dest = new File(uploadDir + File.separator + file.getOriginalFilename());
file.transferTo(dest);
return "You successfully uploaded " + file.getOriginalFilename() + "!";
} catch (IOException e) {
e.printStackTrace();
return "Failed to upload " + file.getOriginalFilename() + "!";
}
}
}
创建上传页面
使用Thymeleaf创建一个简单的文件上传页面。在src/main/resources/templates
目录下创建一个upload.html
文件:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>File Upload</title>
</head>
<body>
<h1>Upload a File</h1>
<form method="POST" enctype="multipart/form-data" action="/upload">
<input type="file" name="file"/>
<input type="submit" value="Upload"/>
</form>
</body>
</html>
实现文件下载功能
创建文件下载控制器
创建一个控制器类,用于处理文件下载请求。
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
import java.nio.file.Path;
import java.nio.file.Paths;
@RestController
public class FileDownloadController {
@Value("${file.upload-dir}")
private String uploadDir;
@GetMapping("/download/{filename}")
public ResponseEntity<Resource> downloadFile(@PathVariable String filename) {
try {
Path filePath = Paths.get(uploadDir).resolve(filename).normalize();
Resource resource = new UrlResource(filePath.toUri());
if (resource.exists()) {
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
} else {
return ResponseEntity.notFound().build();
}
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.notFound().build();
}
}
}
安全性和最佳实践
文件大小限制
为了防止用户上传过大的文件,可以在application.properties
中设置文件大小限制:
spring.servlet.multipart.max-file-size=2MB
spring.servlet.multipart.max-request-size=2MB
文件类型验证
为了防止上传恶意文件,可以在上传控制器中添加文件类型验证:
import org.springframework.web.bind.annotation.RequestMapping;
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file,
RedirectAttributes redirectAttributes) {
if (file.isEmpty()) {
return "Please select a file to upload.";
}
String contentType = file.getContentType();
if (!isValidContentType(contentType)) {
return "Invalid file type. Only PNG, JPEG, and PDF are allowed.";
}
try {
File dest = new File(uploadDir + File.separator + file.getOriginalFilename());
file.transferTo(dest);
return "You successfully uploaded " + file.getOriginalFilename() + "!";
} catch (IOException e) {
e.printStackTrace();
return "Failed to upload " + file.getOriginalFilename() + "!";
}
}
private boolean isValidContentType(String contentType) {
return contentType.equals("image/png") ||
contentType.equals("image/jpeg") ||
contentType.equals("application/pdf");
}
文件名和路径验证
为了防止路径遍历攻击,需要验证上传文件的文件名和路径:
import org.springframework.web.util.UriUtils;
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file,
RedirectAttributes redirectAttributes) {
if (file.isEmpty()) {
return "Please select a file to upload.";
}
String fileName = UriUtils.encodePath(file.getOriginalFilename(), "UTF-8");
Path destinationPath = Paths.get(uploadDir).resolve(fileName).normalize();
if (!destinationPath.startsWith(Paths.get(uploadDir))) {
return "Invalid file path.";
}
try {
file.transferTo(destinationPath.toFile());
return "You successfully uploaded " + file.getOriginalFilename() + "!";
} catch (IOException e) {
e.printStackTrace();
return "Failed to upload " + file.getOriginalFilename() + "!";
}
}
文件下载时的安全性
在处理文件下载请求时,也需要注意路径遍历攻击,并对文件路径进行验证:
@GetMapping("/download/{filename}")
public ResponseEntity<Resource> downloadFile(@PathVariable String filename) {
try {
String encodedFileName = UriUtils.encodePath(filename, "UTF-8");
Path filePath = Paths.get(uploadDir).resolve(encodedFileName).normalize();
if (!filePath.startsWith(Paths.get(uploadDir))) {
return ResponseEntity.badRequest().body(null);
}
Resource resource = new UrlResource(filePath.toUri());
if (resource.exists()) {
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
} else {
return ResponseEntity.notFound().build();
}
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.notFound().build();
}
}
测试与部署
在完成文件上传和下载功能的开发后,应该进行充分的测试,确保所有功能都能正常工作。可以使用JUnit和MockMVC进行单元测试和集成测试。
示例:编写单元测试
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.multipart;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@SpringBootTest
@AutoConfigureMockMvc
public class FileUploadTests {
@Autowired
private MockMvc mockMvc;
@Test
public void testFileUpload() throws Exception {
mockMvc.perform(multipart("/upload")
.file("file", "test content".getBytes()))
.andExpect(status().isOk());
}
}
通过这种方式,可以确保应用的各个部分在开发过程中得到充分的测试,减少上线后的问题。
部署
SpringBoot应用可以打包成可执行的JAR文件,方便部署。通过mvn package
命令,可以生成一个包含所有依赖的JAR文件。
mvn package
java -jar target/demo-0.0.1-SNAPSHOT.jar
这种打包方式使得SpringBoot应用的部署变得非常简单,不再需要复杂的服务器配置。
结论
通过本文的介绍,我们了解了如何使用SpringBoot实现文件上传和下载