1.数据库建表
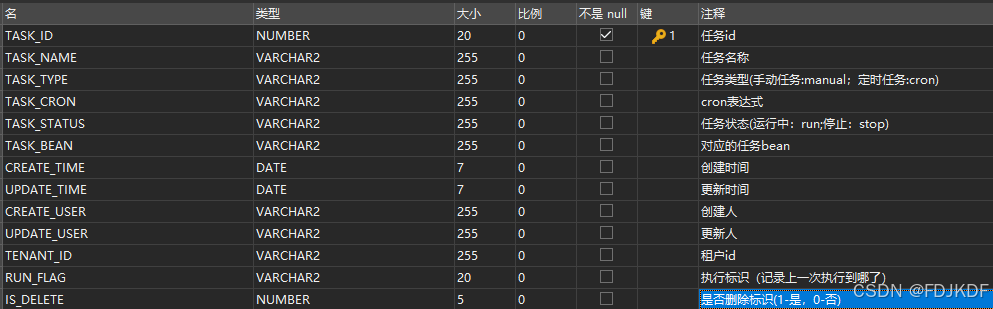
2.引入依赖
<dependency>
<groupId>org.quartz-scheduler</groupId>
<artifactId>quartz</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
3.创建实体类
@Data
@EqualsAndHashCode(callSuper = false)
@TableName("TASK_INFO")
public class TaskInfo {
private static final long serialVersionUID = 1L;
@ApiModelProperty(value = "主键")
@TableId(value = "task_id", type = IdType.AUTO)
private Long taskId;
@ApiModelProperty(value = "租户ID")
private String tenantId;
@ApiModelProperty(value = "任务名称")
private String taskName;
@ApiModelProperty(value = "任务类型")
private String taskType;
@ApiModelProperty(value = "任务的cron表达式")
private String taskCron;
@ApiModelProperty(value = "任务状态")
private String taskStatus;
@ApiModelProperty(value = "任务对应bean")
private String taskBean;
@ApiModelProperty(value = "创建时间")
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone="GMT+8")
private Date createTime;
@ApiModelProperty(value = "修改时间")
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone="GMT+8")
private Date updateTime;
@ApiModelProperty(value = "创建人")
private String createUser;
@ApiModelProperty(value = "修改人")
private String updateUser;
@ApiModelProperty(value = "修改时间")
private String runFlag;
@ApiModelProperty(value = "是否删除标识(1-是,0-否)")
private Integer isDelete;
@ApiModelProperty(value = "医院名称")
@TableField(exist = false)
private String hospitalName;
@ApiModelProperty(value = "任务下次的执行时间")
@TableField(exist = false)
private List<String> runtimeList;
}
4.开启关闭任务接口
@RestController
@Slf4j
@Api(value = "任务接口", tags = "任务接口")
@RequestMapping("/task")
public class TaskController {
@Resource
private ITaskInfoService iTaskInfoService;
@ApiOperation(value = "新增任务接口")
@PostMapping("/saveJob")
public Result<String> saveJob(@RequestBody TaskInfo taskInfo) {
try {
iTaskInfoService.saveJob(taskInfo);
return Result.data("新增成功");
}catch (Exception e) {
return Result.fail(e.getMessage());
}
}
@ApiOperation(value = "开启任务接口")
@GetMapping("/startJob")
public Result<String> startJob(@RequestParam @ApiParam("任务id") Long taskId) {
try {
iTaskInfoService.startJob(taskId);
return Result.data("开启成功");
}catch (GlobalException e) {
return Result.fail(e.getMessage());
}
}
@ApiOperation(value = "停止任务接口")
@GetMapping("/stopJob")
public Result<String> stopJob(@RequestParam @ApiParam("任务id") Long taskId) {
try {
iTaskInfoService.stopJob(taskId);
return Result.data("停止成功");
}catch (Exception e) {
return Result.fail(e.getMessage());
}
}
@ApiOperation(value = "更新任务接口")
@PostMapping("/updateJob")
public Result<String> updateJob(@RequestBody TaskInfo taskInfo) {
try {
iTaskInfoService.updateJob(taskInfo);
return Result.data("更新成功");
}catch (Exception e) {
return Result.fail(e.getMessage());
}
}
@ApiOperation(value = "删除任务接口")
@DeleteMapping("/deleteJob")
public Result<String> deleteJob(@RequestParam("taskId") @ApiParam("任务id") Long taskId) {
try {
iTaskInfoService.deleteJob(taskId);
return Result.data("删除成功");
}catch (Exception e) {
return Result.fail(e.getMessage());
}
}
@ApiOperation(value = "获取全部任务接口")
@PostMapping("/getAllJob")
public Result<Page<TaskInfo>> getAllJob(@RequestBody BaseSearchDto searchDto) {
try {
return Result.data(iTaskInfoService.getAllJob(searchDto));
}catch (GlobalException e) {
return Result.fail(e.getMessage());
}
}
@ApiOperation(value = "获取任务实现类标识")
@PostMapping("/getTaskImpl")
public Result< List<Map<String, String>>> getTaskImpl() {
try {
Map<String, String> jobMap = PackageUtil.getClassNameJar("com.tqmed.saas.report.job");
// 构建集合
List<Map<String, String>> list = new ArrayList<>();
for (Map.Entry<String, String> entry : jobMap.entrySet()) {
String id = entry.getKey();
String name = entry.getValue();
Map<String, String> item = new HashMap<>();
item.put("id", id);
item.put("name", name);
list.add(item);
}
return Result.data(list);
}catch (Exception e) {
return Result.fail(e.getMessage());
}
}
}
package com.tqmed.saas.report.service.impl;
@Service
public class TaskInfoServiceImpl extends ServiceImpl<TaskInfoMapper, TaskInfo> implements ITaskInfoService {
@Resource
private JobManage jobManage;
@Resource
private Scheduler scheduler;
@Override
public void saveJob(TaskInfo taskInfo) throws GlobalException {
if( taskInfo.getTaskCron()!=null && !taskInfo.getTaskCron().equals("") && !CronExpression.isValidExpression(taskInfo.getTaskCron()))
throw new GlobalException("corn表达式格式不正确");
TaskInfo info = lambdaQuery().eq(TaskInfo::getTaskBean, taskInfo.getTaskBean()).eq(TaskInfo::getTenantId, taskInfo.getTenantId()).eq(TaskInfo::getIsDelete,JobConstant.NO_DELETE).one();
if(info!=null){
throw new GlobalException("该租户下任务已存在请勿重复添加");
}
QueryWrapper<TaskInfo> queryWrapper = new QueryWrapper<>();
queryWrapper.select("max(TASK_ID) as TASK_ID");
TaskInfo one = getOne(queryWrapper);
if (one == null) {
taskInfo.setTaskId(1L);
}else {
taskInfo.setTaskId(one.getTaskId()+1);
}
taskInfo.setUpdateUser(SecurityUtils.getUsername());
taskInfo.setCreateUser(SecurityUtils.getUsername());
taskInfo.setTaskType("cron");
taskInfo.setTaskStatus("stop");
taskInfo.setCreateTime(new Date());
taskInfo.setUpdateTime(new Date());
taskInfo.setIsDelete(0);
save(taskInfo);
}
@Override
public void startJob(Long taskId) throws GlobalException {
TaskInfo taskInfo = getById(taskId);
if (taskInfo.getTaskStatus().equals(JobConstant.RUN)) {
throw new GlobalException("该任务已经在运行中了");
}
if( taskInfo.getTaskCron()!=null && !taskInfo.getTaskCron().equals("") && !CronExpression.isValidExpression(taskInfo.getTaskCron()))
throw new GlobalException("corn表达式格式不正确");
jobManage.startJob(taskInfo);
try {
if (!scheduler.isShutdown()) {
scheduler.start();
}
} catch (SchedulerException e) {
throw new RuntimeException(e);
}
taskInfo.setTaskStatus(JobConstant.RUN);
updateById(taskInfo);
}
@Override
public void stopJob(Long taskId) throws GlobalException {
TaskInfo taskInfo = getById(taskId);
if (taskInfo.getTaskStatus().equals(JobConstant.STOP)) {
throw new GlobalException("该任务已经停止运行了");
}
jobManage.stopJob(taskInfo);
taskInfo.setTaskStatus(JobConstant.STOP);
updateById(taskInfo);
}
@Override
public void updateJob(TaskInfo taskInfo) throws GlobalException {
if( taskInfo.getTaskCron()!=null && !taskInfo.getTaskCron().equals("") && !CronExpression.isValidExpression(taskInfo.getTaskCron()))
throw new GlobalException("corn表达式格式不正确");
if(taskInfo.getRunFlag()!=null && !taskInfo.getRunFlag().equals("")){
try {
SimpleDateFormat flagpattern = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
flagpattern.setLenient(false);
flagpattern.parse(taskInfo.getRunFlag());
}catch (ParseException e){
throw new GlobalException("执行标识格式不正确!请输入正确的执行标识");
}
}
//更新需要先停止任务
if (jobManage.checkJobStatus(taskInfo)){
throw new GlobalException("更新操作需要先停止任务");
}
taskInfo.setUpdateUser(SecurityUtils.getUsername());
taskInfo.setUpdateTime(new Date());
updateById(taskInfo);
}
@Override
public void deleteJob(Long taskId) throws GlobalException {
TaskInfo taskInfo = getById(taskId);
//删除需要先停止任务
if (jobManage.checkJobStatus(taskInfo)){
throw new GlobalException("删除操作需要先停止任务");
}
taskInfo.setIsDelete(JobConstant.TRUE_DELETE);
taskInfo.setUpdateUser(SecurityUtils.getUsername());
taskInfo.setUpdateTime(new Date());
updateById(taskInfo);
}
@Override
public Page<TaskInfo> getAllJob(BaseSearchDto searchDto) throws GlobalException {
Page<TaskInfo> page = new Page<>(searchDto.getPage(),searchDto.getPageSize());
LambdaQueryWrapper<TaskInfo> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.eq(TaskInfo::getIsDelete, JobConstant.NO_DELETE);
if(searchDto.getTaskName()!=null && !searchDto.getTaskName().equals(""))
queryWrapper.like(TaskInfo::getTaskName,searchDto.getTaskName());
if(searchDto.getTenantId()!=null && !searchDto.getTenantId().equals(""))
queryWrapper.eq(TaskInfo::getTenantId,searchDto.getTenantId());
if(searchDto.getTaskStatus()!=null && !searchDto.getTaskStatus().equals(""))
queryWrapper.eq(TaskInfo::getTaskStatus,searchDto.getTaskStatus());
//设置医院名称
Page<TaskInfo> pageParm = page(page, queryWrapper);
List<TaskInfo> records = pageParm.getRecords();
for (TaskInfo record : records) {
// record.setHospitalName(sysClient.getHospitalNameByTenantId(record.getTenantId()).getData());
try {
List<String> runtimeList = new ArrayList<>();
CronExpression cron = new CronExpression(record.getTaskCron());
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date currentDate = new Date();
for (int i = 0; i < 3; i++) {// 获取未来3次执行的时间
Date nextExecutionTime = cron.getNextValidTimeAfter(currentDate);
runtimeList.add(format.format(nextExecutionTime));
currentDate = nextExecutionTime;
}
record.setRuntimeList(runtimeList);
} catch (ParseException e) {
throw new GlobalException("cron表达式异常");
}
}
return pageParm.setRecords(records);
}
@Override
public TaskInfo getTaskRunFlag(Integer taskId) {
TaskInfo taskInfo = baseMapper.selectById(taskId);
return taskInfo;
}
//
@Override
public Boolean updateTaskRunFlag(Integer taskId, String nextRunFlag) {
// SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
// String nextFlagTask = dateFormat.format(nextRunFlag);
TaskInfo taskInfo = new TaskInfo();
taskInfo.setTaskId(Long.valueOf(taskId));
taskInfo.setRunFlag(nextRunFlag);
return baseMapper.updateById(taskInfo)>0;
}
}
@Slf4j
@Component
public class JobManage {
@Resource
private Scheduler scheduler;
public void startJob(TaskInfo taskInfo) throws GlobalException {
try {
if (scheduler.checkExists(JobKey.jobKey(taskInfo.getTaskId().toString()))) {
throw new GlobalException("这个任务已经在运行了了");
}
JobDetail jobDetail = JobBuilder.newJob((Class<? extends Job>) Class.forName(taskInfo.getTaskBean()))
.withIdentity(JobKey.jobKey(taskInfo.getTaskId().toString(), JobConstant.TASK_GROUP) )
.build();
CronTrigger trigger = TriggerBuilder.newTrigger()
.withIdentity(TriggerKey.triggerKey(taskInfo.getTaskId().toString(),JobConstant.TASK_GROUP))
.withSchedule(CronScheduleBuilder.cronSchedule(taskInfo.getTaskCron()))
.build();
scheduler.scheduleJob(jobDetail, trigger);
} catch (Exception e) {
e.printStackTrace();
log.error("开始任务出错e={}",e.getMessage());
}
}
public void stopJob(TaskInfo taskInfo) throws GlobalException {
try {
if (!scheduler.checkExists(JobKey.jobKey(taskInfo.getTaskId().toString(),JobConstant.TASK_GROUP))) {
throw new GlobalException("这个任务已经停止了");
}
scheduler.deleteJob(JobKey.jobKey(taskInfo.getTaskId().toString(),JobConstant.TASK_GROUP));
} catch (Exception e) {
e.printStackTrace();
throw new GlobalException(e.getMessage());
}
}
public Boolean checkJobStatus(TaskInfo taskInfo) {
try {
if (scheduler.checkExists(JobKey.jobKey(taskInfo.getTaskId().toString(),JobConstant.TASK_GROUP))) {
return Boolean.TRUE;
}
} catch (SchedulerException e) {
e.printStackTrace();
}
return Boolean.FALSE;
}
}
5.创建任务
@Slf4j
@Component("PatientInfoJob")
@DisallowConcurrentExecution
@ClassAlias("上传患者基本信息")
public class PatientInfoJob implements Job {
@Resource
private ITaskInfoService taskInfoService;
@Resource
private SpPatientMapper spPatientMapper;
@Resource
private RequestToken requestToken;
@Override
public void execute(JobExecutionContext jobExecutionContext) throws JobExecutionException {
log.info("任务2:----上传患者基本信息----开始");
String taskId = jobExecutionContext.getJobDetail().getKey().getName();
log.info("执行的任务taskId == {}",taskId);
TaskInfo taskInfo = taskInfoService.getTaskRunFlag(Integer.valueOf(taskId));
String taskRunFlag=taskInfo.getRunFlag();
if(taskRunFlag==null ){
log.info("上传患者基本信息RunFlag为【null】 --- 第一次上传患者基本信息");
}
List<PatientInfoDto> patientInfoList=spPatientMapper.patientInfo(taskRunFlag,taskInfo.getTenantId());
for (PatientInfoDto patientInfoDto : patientInfoList) {
patientInfoDto.setOpEmHpExMark("1");//门诊
patientInfoDto.setCardtype("0");//身份证
if(patientInfoDto.getSex().equals("0000")||patientInfoDto.getSex().equals("女")) patientInfoDto.setSex("2");
else if(patientInfoDto.getSex().equals("0001")||patientInfoDto.getSex().equals("男")) patientInfoDto.setSex("1");
else patientInfoDto.setSex("未知");
if(StringUtils.isEmpty(patientInfoDto.getGbname())) patientInfoDto.setGbname("不祥");
patientInfoDto.setCardtypeOri("0");//身份证
patientInfoDto.setCardtypeNameOri("身份证");
}
// 检查列表是否为空
if (!patientInfoList.isEmpty()) {
// 获取时间最大值,即列表中最后一个元素的 entryTime
Optional<String> max = patientInfoList.stream().map(PatientInfoDto::getFilingDate).filter(entryTime -> entryTime != null).max(String::compareTo);
if(max.isPresent()){
taskRunFlag=max.get();
}
} if(!patientInfoList.isEmpty()&& StringUtils.isEmpty(taskRunFlag)){
Date date = new Date();//获得系统时间.
SimpleDateFormat sdf = new SimpleDateFormat( "yyyy-MM-dd HH:mm:ss" );
taskRunFlag=sdf.format(date);
}
if(patientInfoList.isEmpty()){
log.info("{}上传患者基本信息---->【空】", LocalDateTime.now());
return;
}
try {
// String url = "http://127.0.0.1:8080/addresses/token1";
String token = requestToken.token();
if(StringUtils.isEmpty(token)){
throw new GlobalException("RequestToken获取token为空");
}
int batchSize = 100; // 每批次的大小
List<List<PatientInfoDto>> partition = ListUtils.partition(patientInfoList, batchSize);
for (List<PatientInfoDto> patientInfos : partition) {
List<Object> list = new ArrayList<>(patientInfos);
Request request = new Request(list,token);
Client client = new Client(UrlConstant.IP+UrlConstant.PATIENT_INFO_URL);
Response response = client.execute(request);
JSONObject resObject = JSON.parseObject(response.getBody());
if (resObject.getInteger("code")==200) {
KmeResult result = JSON.parseObject(response.getBody(), KmeResult.class);
if(result.getCode()==200){
log.info("上传===上传患者基本信息 arr = {}",list);
}else{
String errorMsg="上传患者基本信息错误信息:"+result.getMsg();
log.info(errorMsg);
Optional<String> min = patientInfos.stream().map(PatientInfoDto::getFilingDate).filter(entryTime -> entryTime != null).max(String::compareTo);
if(min.isPresent()) taskRunFlag=min.get();
throw new GlobalException(errorMsg);
}
}else {
String errorMsg="上传患者基本信息错误信息:"+resObject.toString();
log.info(errorMsg);
Optional<String> min = patientInfos.stream().map(PatientInfoDto::getFilingDate).filter(entryTime -> entryTime != null).max(String::compareTo);
if(min.isPresent()) taskRunFlag=min.get();
throw new GlobalException(errorMsg);
}
}
} catch (Exception e) {
throw new RuntimeException(e.getMessage());
}
//设置当前执行标识
taskInfoService.updateTaskRunFlag(Integer.valueOf(taskId),taskRunFlag);
log.info("任务2:========================= 上传患者基本信息任务完毕 ==============================");
}
}
6.服务启动初始化任务
@Slf4j
@Service
public class InitJob implements CommandLineRunner {
@Resource
private ITaskInfoService iTaskInfoService;
@Resource
private JobManage jobManage;
@Resource
private Scheduler scheduler;
public static final Map<String, Class<? extends Job>> map = new HashMap<>();
static {
}
@Override
public void run(String... args) throws Exception {
log.info("---------------开始初始化任务---------------");
LambdaQueryWrapper<TaskInfo> queryWrapper = new LambdaQueryWrapper<>();
queryWrapper.eq(TaskInfo::getIsDelete, JobConstant.NO_DELETE);
List<TaskInfo> taskInfos = iTaskInfoService.getBaseMapper().selectList(queryWrapper);
for (TaskInfo taskInfo : taskInfos) {
if (taskInfo.getTaskStatus().equals(JobConstant.RUN)) {
jobManage.startJob(taskInfo);
}
}
try {
scheduler.start();
} catch (SchedulerException e) {
e.printStackTrace();
}
log.info("---------------初始化任务结束--------------");
}
}
6.工具类、常量、自定义注解
public class PackageUtil {
/**
* 获取某包下(包括该包的所有子包)所有类
*
* @param packageName
* 包名
* @return 类的完整名称
*/
public static List<String> getClassName(String packageName) {
return getClassName(packageName, true);
}
/**
* 获取某包下所有类
*
* @param packageName
* 包名
* @param childPackage
* 是否遍历子包
* @return 类的完整名称
*/
public static List<String> getClassName(String packageName, boolean childPackage) {
List<String> fileNames = null;
ClassLoader loader = Thread.currentThread().getContextClassLoader();
String packagePath = packageName.replace(".", "/");
URL url = loader.getResource(packagePath);
if(url!=null&&url.getProtocol().equals("jar")){
String packageNameJar="BOOT-INF.classes."+packageName;
String packagePathJar = packageNameJar.replace(".", "/");
url=loader.getResource(packagePathJar);
}
if (url != null) {
String type = url.getProtocol();
if (type.equals("file")) {
fileNames = getClassNameByFile(url.getPath(), null, childPackage);
} else if (type.equals("jar")) {
fileNames = getClassNameByJar(url.getPath(), childPackage);
}
} else {
fileNames = getClassNameByJars(((URLClassLoader) loader).getURLs(), packagePath, childPackage);
}
return fileNames;
}
/**
* 从项目文件获取某包下所有类
*
* @param filePath
* 文件路径
* @param className
* 类名集合
* @param childPackage
* 是否遍历子包
* @return 类的完整名称
*/
private static List<String> getClassNameByFile(String filePath, List<String> className, boolean childPackage) {
List<String> myClassName = new ArrayList<>();
File file = new File(filePath);
File[] childFiles = file.listFiles();
for (File childFile : childFiles) {
if (childFile.isDirectory()) {
if (childPackage) {
myClassName.addAll(getClassNameByFile(childFile.getPath(), myClassName, childPackage));
}
} else {
String childFilePath = childFile.getPath();
if (childFilePath.endsWith(".class")) {
childFilePath = childFilePath.substring(childFilePath.indexOf("\\classes") + 9,
childFilePath.lastIndexOf("."));
childFilePath = childFilePath.replace("\\", ".");
myClassName.add(childFilePath);
}
}
}
return myClassName;
}
/**
* 从jar获取某包下所有类
*
* @param jarPath
* jar文件路径
* @param childPackage
* 是否遍历子包
* @return 类的完整名称
*/
private static List<String> getClassNameByJar(String jarPath, boolean childPackage) {
List<String> myClassName = new ArrayList<>();
String[] jarInfo = jarPath.split("!");
String jarFilePath = jarInfo[0].substring(jarInfo[0].indexOf("/"));
String packagePath = jarInfo[1].substring(1);
try {
JarFile jarFile = new JarFile(jarFilePath);
Enumeration<JarEntry> entrys = jarFile.entries();
while (entrys.hasMoreElements()) {
JarEntry jarEntry = entrys.nextElement();
String entryName = jarEntry.getName();
if (entryName.endsWith(".class")) {
if (childPackage) {
if (entryName.startsWith(packagePath)) {
entryName = entryName.replace("/", ".").substring(0, entryName.lastIndexOf("."));
myClassName.add(entryName);
}
} else {
int index = entryName.lastIndexOf("/");
String myPackagePath;
if (index != -1) {
myPackagePath = entryName.substring(0, index);
} else {
myPackagePath = entryName;
}
if (myPackagePath.equals(packagePath)) {
entryName = entryName.replace("/", ".").substring(0, entryName.lastIndexOf("."));
myClassName.add(entryName);
}
}
}
}
} catch (Exception e) {
e.printStackTrace();
}
String prefixToRemove = "BOOT-INF.classes.";
List<String> strings = new ArrayList<>();
for (String s : myClassName) {
if(s.startsWith(prefixToRemove)){
String classNames1 = s.substring(prefixToRemove.length());
strings.add(classNames1);
}
}
return strings;
}
/**
* 从所有jar中搜索该包,并获取该包下所有类
*
* @param urls
* URL集合
* @param packagePath
* 包路径
* @param childPackage
* 是否遍历子包
* @return 类的完整名称
*/
private static List<String> getClassNameByJars(URL[] urls, String packagePath, boolean childPackage) {
List<String> myClassName = new ArrayList<>();
if (urls != null) {
for (int i = 0; i < urls.length; i++) {
URL url = urls[i];
String urlPath = url.getPath();
// 不必搜索classes文件夹
if (urlPath.endsWith("classes/")) {
continue;
}
String jarPath = urlPath + "!/" + packagePath;
myClassName.addAll(getClassNameByJar(jarPath, childPackage));
}
}
return myClassName;
}
public static Map<String, String> getClassNameJar(String packageName) {
Map<String, String> classNames = new HashMap<>();
List<String> className = getClassName(packageName, true);
// String prefixToRemove = "BOOT-INF.classes.";
// for (String s : className) {
// if(s.startsWith(prefixToRemove)){
// String classNames1 = s.substring(prefixToRemove.length());
// String classAlias = getClassAlias(classNames1);
// classNames.put(classNames1,classAlias);
// }
// }
for (String s : className) {
String classAlias = getClassAlias(s);
classNames.put(s,classAlias);
}
return classNames;
}
private static String getClassAlias(String className) {
// 这里假设 ClassAlias 是一个自定义的注解,用于标记类的中文别名
try {
Class<?> clazz = Class.forName(className);
ClassAlias aliasAnnotation = clazz.getAnnotation(ClassAlias.class);
if (aliasAnnotation != null) {
return aliasAnnotation.value(); // 返回类的中文别名
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return null;
}
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface ClassAlias {
String value(); // 定义一个value属性来表示别名
}
public class JobConstant {
public static final Integer NO_DELETE = 0;
public static final Integer TRUE_DELETE = 1;
public static final String RUN = "run";
public static final String STOP = "stop";
public static final String MANUAL_TASK = "manual";
public static final String TASK_GROUP = "kme_report";
public static final String HIS_AUTH_TOKEN="HIS_AUTH_TOKEN";//redis存的token的key
public static final Integer TIME=23;//token过期时间
public static final String APP_KEY="";
public static final String APP_SECRET="";
}
package com.tqmed.saas.report.openapi.util;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.tqmed.saas.common.utils.RedisUtils;
import com.tqmed.saas.report.constant.JobConstant;
import com.tqmed.saas.report.constant.UrlConstant;
import lombok.extern.slf4j.Slf4j;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.TimeUnit;
/**
* @author xqc
* @create 2024-06-17 9:04
*/
@Slf4j
@Component
public class RequestToken {
@Autowired
private RedisUtils redisUtils;
public String token() throws IOException {
String resultToken = (String)redisUtils.get(JobConstant.HIS_AUTH_TOKEN);
if(resultToken!=null){
return resultToken;
}
CloseableHttpClient client = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost(UrlConstant.TOKEN_URL);
Map map = new HashMap<>();
map.put("appKey", JobConstant.APP_KEY);
map.put("appSecret",JobConstant.APP_SECRET);
String jsonString = JSONObject.toJSONString(map);
//将json转为httpentity对象
StringEntity stringEntity=null;
try {
stringEntity = new StringEntity(jsonString);
} catch (UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
httpPost.setEntity(stringEntity);
httpPost.setHeader("Content-Type","application/json");
//定义返回对象
CloseableHttpResponse resp=null;
try {
resp = client.execute(httpPost);
int code = resp.getStatusLine().getStatusCode();
int i=1;
String string = EntityUtils.toString(resp.getEntity());
JSONObject jsonObject = JSON.parseObject(string);
while (i<=5) {
if(code == 200) {
if (jsonObject.getIntValue("code") == 200) {
JSONObject data = jsonObject.getJSONObject("data");
resultToken = data.getString("token");
redisUtils.set(JobConstant.HIS_AUTH_TOKEN, resultToken, JobConstant.TIME, TimeUnit.HOURS);
break;
} else if (i < 5 && i >= 2) {
log.info("上传===获取token失败3秒后第{}次尝试", i);
Thread.sleep(3000);
}else if(i==5){
String errorMsg="RequestToken获取token失败:"+string;
log.info(errorMsg);
}
}else {
String errorMsg="RequestToken获取token失败:"+string;
log.info(errorMsg);
}
i++;
}
} catch (Exception e) {
e.printStackTrace();
}finally {
client.close();
resp.close();
}
return resultToken;
}
}
package com.tqmed.saas.report.openapi;
import com.alibaba.fastjson.JSON;
import com.tqmed.saas.report.constant.SystemHeader;
import com.tqmed.saas.report.openapi.util.*;
/**
* Client
*/
public class Client {
private String apiUrl;
private String appKey;
private String appSecret;
private String encodingAesKey;
public Client(String apiUrl) {
this.apiUrl=apiUrl;
// this.appKey = appKey;
// this.appSecret = appSecret;
// this.encodingAesKey = encodingAesKey;
}
/**
* 发送请求
*
* @param request request对象
* @return Response
* @throws Exception
*/
public Response execute(Request request) throws Exception {
request.setApiUrl(this.apiUrl);
// request.setAppKey(this.appKey);
// request.setAppSecret(this.appSecret);
// request.setEncodingAesKey(this.encodingAesKey);
// request.addHeader(SystemHeader.X_CA_KEY,request.getAppKey());
// request.addHeader(SystemHeader.X_CA_NONCE, UUID.randomUUID().toString());
// request.addHeader("Content-Type", "application/json");
request.addHeader(SystemHeader.HIS_AUTH, request.getToken());
String jsonStr= JSON.toJSONString(request.getBodys());
// String encryptStr;
// if(StringUtils.isNotEmpty(request.getToken())){
// encryptStr = AESUtils.encrypt(jsonStr, request.getEncodingAesKey());
// }else {
// encryptStr =jsonStr;
// }
// String contentMd5 = MessageDigestUtil.base64AndMD5(encryptStr);
// request.addHeader(SystemHeader.X_CONTENT_MD5, contentMd5);
// String signature = SignUtil.sign(request.getAppSecret(), request.getHeaders());
// request.addHeader(SystemHeader.X_CA_SIGNATURE,signature);
request.setStringBody(jsonStr);
return OpenApiUtils.post(request);
}
// public static void main(String[] args) throws Exception {
// String body="[1]";
// String encryptStr = AESUtils.encrypt(body, "1234567890123456");
// String contentMd5 = MessageDigestUtil.base64AndMD5(encryptStr.getBytes());
// System.out.println(contentMd5);
//
// }
}