工具类篇大全
前言
日期Date是编程中最常使用的util类之一,毫无疑问日期就是代表时间,数据的创建时间、更新时间、交易时间、记录时间等;是一个尤为重要的字段和属性。通常在前端展示、数据库存储、数据传输和程序中转换,格式也有多种:Date、String、Long;操作也分为时间格式转换、大小(先后)比较。
一、格式转换大全
public static final String TIME_TEMPLATE = "yyyy-MM-dd HH:mm:ss";
public static final String TIME_TEMPLATE2 = "yyyyMMddHHmmss";
public static final String PERIOD_TEMPLATE = "yyyy-MM";
public static final String DATE_TEMPLATE_Y_M_D = "yyyy-MM-dd";
public static final String DATE_TEMPLATE_YMD = "yyyyMMdd";
/**
* 代替SimpleDateFormat DATETIME_PATTERN 线程不安全方案
*/
protected static final FastDateFormat TIME_FORMAT = FastDateFormat.getInstance(TIME_TEMPLATE);
protected static final FastDateFormat TIME_FORMAT2 = FastDateFormat.getInstance(TIME_TEMPLATE2);
/**
* 代替SimpleDateFormat DATE_PATTERN 线程不安全方案
*/
protected static final FastDateFormat DATE_FORMAT = FastDateFormat.getInstance(DATE_TEMPLATE_Y_M_D);
/**
* 代替SimpleDateFormat DATE_PATTERN 线程不安全方案
*/
protected static final FastDateFormat DATE_FORMAT2 = FastDateFormat.getInstance(DATE_TEMPLATE_YMD);
/**
* 代替SimpleDateFormat PERIOD_PATTERN 线程不安全方案
*/
protected static final FastDateFormat PERIOD_FORMAT = FastDateFormat.getInstance(PERIOD_TEMPLATE);
- Date转成String格式的时间
/**
* TITIL 日期转换成字符串 yyyy/MM/dd HH:mm:ss
* @DateTime 2017年6月23日 下午2:50:27
*
* @param date
* @return
*/
public static String convertDateToStringFormatTime(Date date) {
if (date == null) {
return null;
}
return TIME_FORMAT.format(date);
}
/**
* TITIL 日期转换成字符串 yyyymmddhhmiss
* @DateTime 2017年6月23日 下午2:50:27
*
* @param date
* @return
*/
public static String convertDateToStringFormatTime2(Date date) {
if (date == null) {
return null;
}
return TIME_FORMAT2.format(date);
}
/**
* TITIL 日期转换成字符串 yyyy-MM-dd
* @DateTime 2017年6月23日 下午2:50:27
*
* @param date
* @return
*/
public static String convertDateToString(Date date) {
if (date == null) {
return null;
}
return DATE_FORMAT.format(date);
}
/**
* TITIL 日期转换成字符串 yyyyMMdd
* @DateTime 2017年6月23日 下午2:50:27
*
* @param date
* @return
*/
public static String convertDateToString2(Date date) {
if (date == null) {
return null;
}
return DATE_FORMAT2.format(date);
}
/**
* @author
* @DateTime 2018年7月16日 下午8:47:34
*
* @param date
* @return
*/
public static String convertDateToPeriod(Date date) {
if (date == null) {
return null;
}
return PERIOD_FORMAT.format(date);
}
- String转成Date格式的时间
/**
* titil : 字符串(YYYY/MM/DD)转日期
* @DateTime 2018年1月18日 下午3:23:00
*
* @param strDate
* @return
* @throws ParseException
*/
public static Date convertStringToDate(String strDate) {
try {
return TIME_FORMAT.parse(strDate);
} catch (ParseException pe) {
throw new JobRuntimeException(pe.getMessage(), pe);
}
}
public static Date convertStringToDate2(String strDate) {
try {
return TIME_FORMAT2.parse(strDate);
} catch (ParseException pe) {
throw new JobRuntimeException(pe.getMessage(), pe);
}
}
/**
* titil : 字符串(YYYY/MM/DD)转日期
* @author
* @DateTime 2018年1月18日 下午3:23:00
*
* @param strDate
* @return
* @throws ParseException
*/
public static Date convertStringDateToDate(String strDate) {
try {
return DATE_FORMAT2.parse(strDate);
} catch (ParseException pe) {
throw new JobRuntimeException(pe.getMessage(), pe);
}
}
public static Date convertStringToDateFormatYmd(String strDate) {
try {
return DATE_FORMAT.parse(strDate);
} catch (ParseException pe) {
throw new JobRuntimeException(pe.getMessage(), pe);
}
}
- Long转成Date格式的时间
public static Date convertSecondLongToDate(Long secondLong) {
Date date = null;
if (secondLong == null || secondLong.longValue() == 0) {
return date;
}
date = new Date(secondLong * 1000);
return date;
}
- Date转成Long格式时间
public static Long convertSecondLongToDate(Date date) {
Long long = null;
if (date == null) {
return null;
}
long = date.getTime();
return long;
}
二、SimpleDateFormat 包
/**
* 日期型转换为YYYY-MM-DD类型
* @DateTime 2017年8月11日 下午2:07:41
*
* @param glDate
* @return
* @throws ParseException
* formatDateToDay
*/
public static Date convertByDate(Date glDate) throws ParseException {
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");
String s = simpleDateFormat.format(glDate);
Date date = simpleDateFormat.parse(s);
return date;
}
/**
* 日期型转换为YYYY-MM-DD类型
* @DateTime 2017年8月11日 下午2:07:41
*
* @param glDate
* @return
* @throws ParseException
* formatDateToDay
*/
public static Date convertByDateTime(Date glDate) throws ParseException {
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String s = simpleDateFormat.format(glDate);
Date date = simpleDateFormat.parse(s);
return date;
}
/**
* 查询期间的最后一天
* @DateTime 2017年8月16日 下午2:15:53
*
* @param periodName
* @return
* @throws ParseException
* getLastDayOfPeriod
*/
public static Date getPeriodLastDay(String periodName) throws ParseException {
Calendar calendar = Calendar.getInstance();
DateFormat format1 = new SimpleDateFormat("yyyy-MM");
calendar.setTime(format1.parse(periodName));
calendar.set(Calendar.DAY_OF_MONTH, calendar.getActualMaximum(Calendar.DAY_OF_MONTH));
calendar.set(Calendar.HOUR_OF_DAY, calendar.getActualMaximum(Calendar.HOUR_OF_DAY));
calendar.set(Calendar.MINUTE, calendar.getActualMaximum(Calendar.MINUTE));
calendar.set(Calendar.SECOND, calendar.getActualMaximum(Calendar.SECOND));
calendar.set(Calendar.MILLISECOND, calendar.getActualMaximum(Calendar.MILLISECOND));
return calendar.getTime();
}
三、Calendar 包
/**
* 查询期间的最后一天
* @DateTime 2017年8月16日 下午2:15:53
*
* @param periodName
* @return
* @throws ParseException
* getLastDayOfPeriod
*/
public static Date getPeriodLastDay(String periodName) throws ParseException {
Calendar calendar = Calendar.getInstance();
DateFormat format1 = new SimpleDateFormat("yyyy-MM");
calendar.setTime(format1.parse(periodName));
calendar.set(Calendar.DAY_OF_MONTH, calendar.getActualMaximum(Calendar.DAY_OF_MONTH));
calendar.set(Calendar.HOUR_OF_DAY, calendar.getActualMaximum(Calendar.HOUR_OF_DAY));
calendar.set(Calendar.MINUTE, calendar.getActualMaximum(Calendar.MINUTE));
calendar.set(Calendar.SECOND, calendar.getActualMaximum(Calendar.SECOND));
calendar.set(Calendar.MILLISECOND, calendar.getActualMaximum(Calendar.MILLISECOND));
return calendar.getTime();
}
/**
* 查询期间的第一天
* @DateTime 2017年8月16日 下午2:15:53
*
* @param periodName
* @return
* @throws ParseException
*/
public static Date getPeriodFirstDay(String periodName) throws ParseException {
Calendar calendar = Calendar.getInstance();
DateFormat format1 = new SimpleDateFormat("yyyy-MM");
calendar.setTime(format1.parse(periodName));
return calendar.getTime();
}
/**获取具体时间
* @DateTime 2017年8月11日 下午4:47:16
*
* @param glDate
* @param dayNum
* @return
*/
public static Date addDayByDate(Date glDate, int dayNum) {
Calendar cl = Calendar.getInstance();
cl.setTime(glDate);
cl.add(Calendar.DATE, dayNum);
Date date = cl.getTime();
return date;
}
/**获取具体时间
* @DateTime 2017年9月19日 下午5:12:57
*
* @param date
* @return
*/
public static Date getUpperLastDay(Date date) {
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.set(Calendar.DAY_OF_MONTH, 1);
calendar.add(Calendar.DAY_OF_MONTH, -1);
return calendar.getTime();
}
四、时间大小比较
/**
* @author
* @DateTime 2018年2月8日 下午8:57:16
*
* @param d1
* @param d2
* @return
*/
public static int compareDate(Date d1, Date d2) {
try {
if (d1 == null && d2 == null) {
return 0;
} else if (d1.getTime() > d2.getTime()) {
return 1;
} else if (d1.getTime() < d2.getTime()) {
return -1;
} else {
return 0;
}
} catch (Exception exception) {
Log.error(exception.getMessage());
throw new RuntimeException(exception.getMessage(), exception);
}
}
既然都看完了整篇文章,相信对你一定有所帮助。原创不易,勿做伸手党。
点击下方【打赏】小编,或者关注公众号给予支持,你们的每一份鼓励都将是小编伟大的动力。
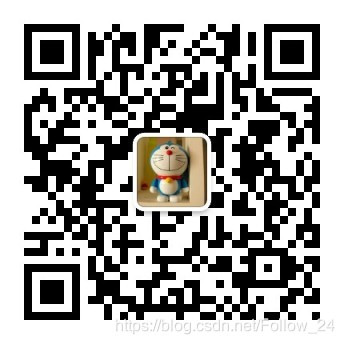