- Memcached 是一个高性能的分布式内存对象缓存系统,用于动态Web应用以减轻数据库负载。它通过在内存中缓存数据和对象来减少读取数据库的次数,从而提高动态、数据库驱动网站的速度。
- 下载memcahed链接驱动
没有的可以在这里下载:https://pan.baidu.com/s/1hsqzHoK
提取码:6eju - 新建一个java project,将jar文件添加到项目中
- 简单的测试
package com.el;
import java.net.InetSocketAddress;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import net.spy.memcached.CASResponse;
import net.spy.memcached.CASValue;
import net.spy.memcached.MemcachedClient;
public class myTestDemo {
private static MemcachedClient mcc;
public static void main(String[] args) {
Init();
MecachedSet();
MecachedAdd();
MemcachedReplace();
MemcachedAppend();
MemcachedPrepend();
MemcachedCAS();
MemcachedGet();
MemcachedDelete();
MemcachedIncrOrDecr();
ShutDown();
}
public static void Init() {
try {
mcc = new MemcachedClient(new InetSocketAddress("127.0.0.1", 11211));
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("connect server success!");
}
public static void ShutDown() {
mcc.shutdown();
}
public static void MecachedSet() {
Future fo = mcc.set("emample0", 900, "emample0 value");
try {
System.out.println("set status:" + fo.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
System.out.println("test value in cache - " + mcc.get("emample0"));
}
public static void MecachedAdd() {
Future f1 = mcc.set("emample1", 900, "emample1 value");
try {
System.out.println("set status:" + f1.get());
System.out.println("emample1 value in cache - " + mcc.get("emample1"));
Future f2 = mcc.add("emample12", 900, "memcached");
System.out.println("add status:" + f2.get());
f2 = mcc.add("codingground", 900, "All Free Compilers");
System.out.println("add status:" + f2.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
System.out.println("emample12 value in cache - " + mcc.get("emample12"));
System.out.println("codingground value in cache - " + mcc.get("codingground"));
}
public static void MemcachedReplace() {
Future fo = mcc.set("example2", 900, "example2 value");
try {
System.out.println("add status:" + fo.get());
System.out.println("runoob value in cache - " + mcc.get("example2"));
fo = mcc.replace("example2", 900, "example2 Library");
System.out.println("replace status:" + fo.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
System.out.println("example value in cache - " + mcc.get("example2"));
}
public static void MemcachedAppend() {
Future f1 = mcc.set("example3", 900, "example3 value");
try {
System.out.println("set status:" + f1.get());
System.out.println("runoob value in cache - " + mcc.get("example3"));
Future f2 = mcc.append("example3", "for all");
System.out.println("append status:" + f2.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
System.out.println("runoob value in cache - " + mcc.get("example3"));
}
public static void MemcachedPrepend() {
Future f1 = mcc.set("expample4", 900, "expample4 value");
try {
System.out.println("set status:" + f1.get());
System.out.println("runoob value in cache - " + mcc.get("expample4"));
Future f2 = mcc.prepend("expample4", "Free ");
System.out.println("prepend status:" + f2.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
System.out.println("runoob value in cache - " + mcc.get("expample4"));
}
public static void MemcachedCAS() {
Future fo = mcc.set("expample5", 900, "expample4 value");
try {
System.out.println("set status:" + fo.get());
System.out.println("runoob value in cache - " + mcc.get("expample5"));
CASValue casValue = mcc.gets("expample5");
System.out.println("CAS token - " + casValue);
CASResponse casresp = mcc.cas("expample5", casValue.getCas(), 900, "expample5-Library");
System.out.println("CAS Response - " + casresp);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
System.out.println("runoob value in cache - " + mcc.get("expample5"));
}
public static void MemcachedGet() {
Future fo = mcc.set("expample6", 900, "expample6 value");
try {
System.out.println("set status:" + fo.get());
System.out.println("expample6 value in cache - " + mcc.get("expample6"));
CASValue casValue = mcc.gets("expample6");
System.out.println("CAS value in cache - " + casValue);
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
public static void MemcachedDelete() {
Future f1 = mcc.set("expample7", 900, "expample7 value");
try {
System.out.println("set status:" + f1.get());
System.out.println("expample7 value in cache - " + mcc.get("expample7"));
Future f2 = mcc.delete("expample7");
System.out.println("delete status:" + f2.get());
System.out.println("expample7 value in cache - " + mcc.get("expample7"));
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
public static void MemcachedIncrOrDecr() {
Future fo = mcc.set("number", 900, "1000");
try {
System.out.println("set status:" + fo.get());
System.out.println("value in cache - " + mcc.get("number"));
System.out.println("value in cache after increment - " + mcc.incr("number", 111));
System.out.println("value in cache after decrement - " + mcc.decr("number", 112));
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
}
}
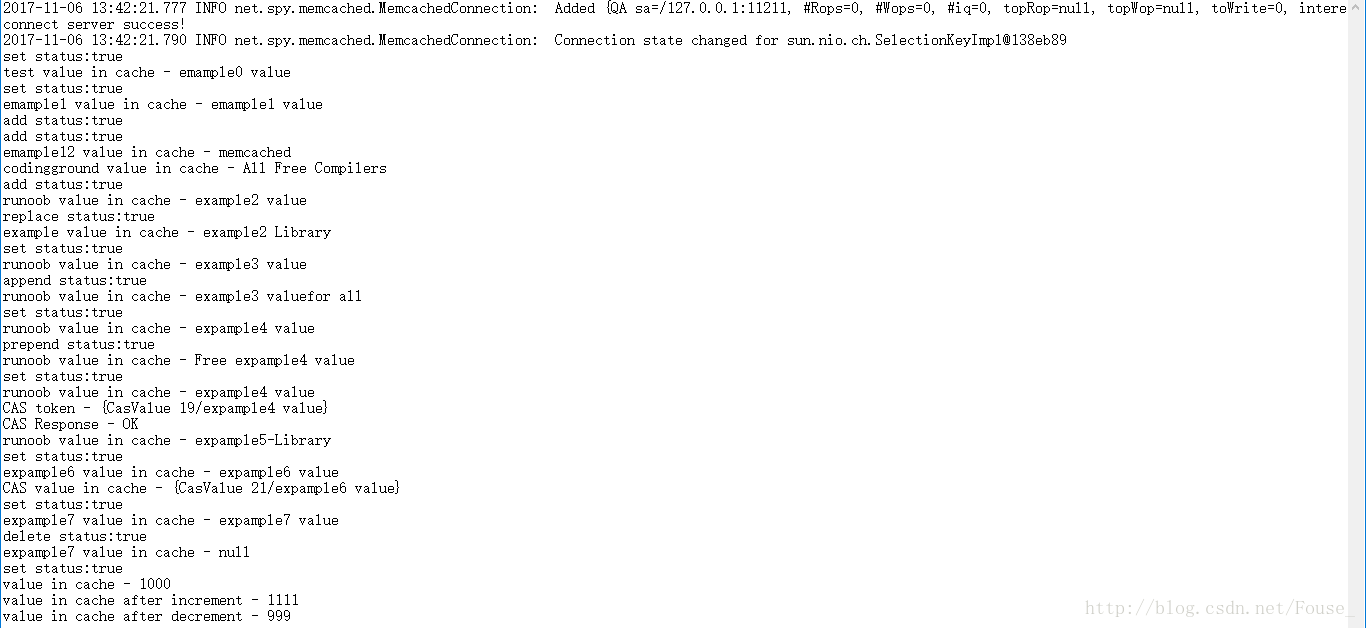