Composite pattern
组合模式
From Wikipedia, the free encyclopedia
In computer science , the composite pattern is a partitioning design pattern . Composite allows a group of objects to be treated in the same way as a single instance of an object. The intent of composite is to "compose objects into tree structures to represent part-whole hierarchies. Composite lets clients treat individual objects and compositions uniformly."
在计算机科学中,组合模式 是一种分离的设计模式。组合允许一组对象当作一个对象的单独的实例,采用统一的处理法方式来进行处理。组合的意图是把对象组装成树形的结构来代表 部分-整体的层次结构。组合让客户可以采用统一的方式来处理不同的对象和组件。
内容
|
<script type="text/javascript"></script>
动机
When dealing with tree-structured data, programmers often have to discriminate between a leaf-node and a branch. This makes code more complex, and therefore, error prone. The solution is an interface that allows treating complex and primitive objects uniformly. In object-oriented programming , a composite is an object (e.g., a shape) designed as a composition of one-or-more similar objects (other kinds of shapes/geometries), all exhibiting similar functionality. This is known as a "has-a " relationship between objects. The key concept is that you can manipulate a single instance of the object just as you would a group of them. The operations you can perform on all the composite objects often have a least common denominator relationship. For example, if defining a system to portray grouped shapes on a screen, it would be useful to define resizing a group of shapes to have the same effect (in some sense) as resizing a single shape.
当处理树形结构的数据的时候,程序员经常需要花时间在节点到底是叶节点还是枝节点上进行区分。这样导致的问题就是代码更加复杂,也因为这样,导致了更多的错误。这个解决方案的就是一个接口,允许统一的处理那些复杂的或简单的对象。在面向对象中,一个组件是一个对象(比喻说是一个形状),它是一个或多个相似类型对象的集合(其他的形状),这些对象之间享有类似的功能。这就是总所周知的对象之间的“has-a”关系。这个模式的最大的好处就在于你可以处理一个对象,就跟处理多个对象一样。你需要在所有的组件对象上做的操作拥有一个最小的公分母,比喻说,如果定义一个系统来自屏幕上绘制成组的形状,那么下面的方式更好一些,定义一组形状有统一的改变大小的方法(某种意义上)的,就如同改变一个形状的大小。
何时实用
Composite can be used when clients should ignore the difference between compositions of objects and individual objects.[1] If programmers find that they are using multiple objects in the same way, and often have nearly identical code to handle each of them, then composite is a good choice; it is less complex in this situation to treat primitives and composites as homogeneous.
组合模式适用于下面的情况,当用户需要忽略多个不同的对象和一个单独对象之间的不同。当一个程序员发现,他在不停的使用一个方法访问不同的对象,而且经常的使用近乎同样的方法来处理他们,这个时候,组合就是一个很好的选择;使用组合可以更简单的处理简单的同类对象的组合。
结构
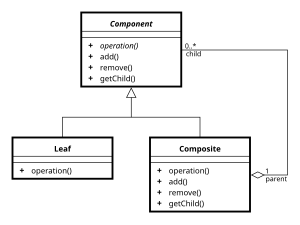

组件
- 所有组件的一个抽象,包括组合的那些
- 定义了组合中所有的对象的接口
- 适当的实现对应所有的类的针对接口的默认行为
- 定义一个简单来访问和管理他们的孩子组件
- (可选的) 定义一个接口来递归的访问它的父亲节点,如果方便的话
叶子
- 定义组合中所有的叶子对象
- 使用所有的组件方法
组合
- 代表一个组合组件(组件有孩子节点)
- 实现方法去操作孩子节点
- 实现所有的组件方法,通常情况下代理给他们的孩子节点
例子
Java
下面的例子,使用Java编写,实现了一个图形类,它可能是一个椭圆或者是一个多个图形的集合,每一个图形都是可以打印的。
import java.util.List;
import java.util.ArrayList;
/** "Component" */
interface
Graphic {
//Prints the graphic.
public void print();
}
/** "Composite" */
class CompositeGraphic implements Graphic {
//Collection of child graphics.
private List<Graphic> mChildGraphics = new ArrayList<Graphic>();
//Prints the graphic.
public void print() {
for (Graphic graphic : mChildGraphics) {
graphic.print();
}
}
//Adds the graphic to the composition.
public void add(Graphic graphic) {
mChildGraphics.add(graphic);
}
//Removes the graphic from the composition.
public void remove(Graphic graphic) {
mChildGraphics.remove(graphic);
}
}
/** "Leaf" */
class Ellipse implements Graphic {
//Prints the graphic.
public void print() {
System.out.println("Ellipse");
}
}
/** Client */
public class Program {
public static void main(String[] args) {
//Initialize four ellipses
Ellipse ellipse1 = new Ellipse();
Ellipse ellipse2 = new Ellipse();
Ellipse ellipse3 = new Ellipse();
Ellipse ellipse4 = new Ellipse();
//Initialize three composite graphics
CompositeGraphic graphic = new CompositeGraphic();
CompositeGraphic graphic1 = new CompositeGraphic();
CompositeGraphic graphic2 = new CompositeGraphic();
//Composes the graphics
graphic1.add(ellipse1);
graphic1.add(ellipse2);
graphic1.add(ellipse3);
graphic2.add(ellipse4);
graphic.add(graphic1);
graphic.add(graphic2);
//Prints the complete graphic (four times the string "Ellipse").
graphic.print(); }
}