1.单独的再shell中创建一个表格(三步走)
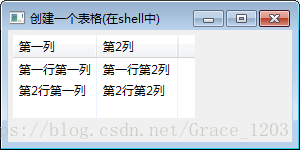
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.*;
import org.eclipse.swt.layout.*;
public class B{
public static void main(String[] args){
Display display=new Display();
Shell shell=new Shell(display);
shell.setText("创建一个表格(在shell中)");
shell.setLayout(new GridLayout());
//(1)创建一个表格Table
Table table=new Table(shell,SWT.FULL_SELECTION);
//(2)创建列TableColumn
// TableColumn tableColumn1=new TableColumn(table,SWT.NONE);
// tableColumn1.setText("第一列");
// TableColumn tableColumn2=new TableColumn(table,SWT.NONE);
// tableColumn2.setText("第2列");
//法二:
String tableHeader[]={"第一列","第2列"};
for(int i=0;i<tableHeader.length;i++){
TableColumn tableColumn=new TableColumn(table,SWT.None);
tableColumn.setText(tableHeader[i]);
}
//设置显示表头
table.setHeaderVisible(true);
//(3)添加行数据TableItem
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"第一行第一列","第一行第2列"});
item=new TableItem(table,SWT.None);
item.setText(new String[]{"第2行第一列","第2行第2列"});
//(4)布局调整
for(int i=0;i<table.getColumnCount();i++){
table.getColumn(i).pack();
}
//法二:
// for(int i=0;i<tableHeader.length;i++){
// table.getColumn(i).pack();
// }
//打开窗口,进行窗口的显示
shell.setSize(300,150);
//shell.pack();
shell.open();
while(!shell.isDisposed()){
//当窗口没有被释放的时候
if(!display.readAndDispatch()){
display.sleep();
}
}
display.dispose();
}
}
2.在viewForm中创建工具栏和表格(整体写)——无事件
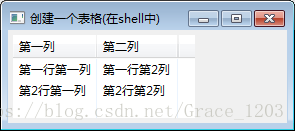
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.*;
import org.eclipse.swt.layout.*;
public class E{
public static void main(String[] args){
Display display=new Display();
Shell shell=new Shell(display);
shell.setText("创建一个表格(在shell中)");
shell.setLayout(new GridLayout());
//(1)创建一个表格Table
Table table=new Table(shell,SWT.FULL_SELECTION);
//(2)创建表头tableHeader,列TableColumn
String[] tableHeader={"第一列","第二列"};
for(int i=0;i<tableHeader.length;i++){
TableColumn tableColumn=new TableColumn(table,SWT.None);
tableColumn.setText(tableHeader[i]);
}
//设置显示表头
table.setHeaderVisible(true);
//(3)添加行数据TableItem
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"第一行第一列","第一行第2列"});
item=new TableItem(table,SWT.None);
item.setText(new String[]{"第2行第一列","第2行第2列"});
//(4)布局调整
for(int i=0;i<tableHeader.length;i++){
table.getColumn(i).pack();
}
//法二:
// for(int i=0;i<table.getColumnCount();i++){
// table.getColumn(i).pack();
// }
//打开窗口,进行窗口的显示
//shell.setSize(300,150);
shell.pack();
shell.open();
while(!shell.isDisposed()){
//当窗口没有被释放的时候
if(!display.readAndDispatch()){
display.sleep();
}
}
display.dispose();
}
}
3.在viewForm中创建工具栏和表格(分开写)——无事件
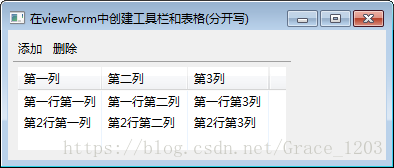
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.ViewForm;
import org.eclipse.swt.layout.*;
import org.eclipse.swt.widgets.*;
public class J {
//全局变量
private Shell shell=null;
private ViewForm viewForm=null;
private ToolBar toolBar=null;
private Table table=null;
private Composite composite=null;
//创建一个viewForm,用于放置工具栏和表格
private void createViewForm(){
viewForm=new ViewForm(shell,SWT.None);
viewForm.setLayout(new GridLayout());
//创建一个工具栏
createToolBar();
//将工具栏绑定在viewForm中
viewForm.setTopLeft(toolBar);
//创建一个面板(表格)
createComposite();
//创建一个面板,将表格显示在面板composite中
viewForm.setContent(composite);
}
//创建一个工具栏
private void createToolBar(){
toolBar=new ToolBar(viewForm,SWT.FLAT);
//在工具栏中创建工具项
final ToolItem addToolItem=new ToolItem(toolBar,SWT.PUSH);
addToolItem.setText("添加");
final ToolItem delToolItem=new ToolItem(toolBar,SWT.PUSH);
delToolItem.setText("删除");
}
//创建一个面板
private void createComposite(){
composite=new Composite(viewForm,SWT.None);
composite.setLayout(new GridLayout());
//在面板中创建表格
createTable();
}
//创建表格,三步走
private void createTable(){
//(1)创建表格对象
//Table table=new Table(shell,SWT.HIDE_SELECTION);//仅选择第一项
table=new Table(composite,SWT.FULL_SELECTION);//选中一行
//Table table=new Table(shell,SWT.CHECK);//多选框
//(2)创建3列表头,创建表头的字符串数组
TableColumn column1=new TableColumn(table,SWT.None);
column1.setText("第一列");
TableColumn column2=new TableColumn(table,SWT.None);
column2.setText("第二列");
TableColumn column3=new TableColumn(table,SWT.None);
column3.setText("第3列");
//设置显示表头
table.setHeaderVisible(true);
//(3)创建一行数据
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"第一行第一列","第一行第二列","第一行第3列"});
item=new TableItem(table,SWT.None);
item.setText(new String[]{"第2行第一列","第2行第二列","第2行第3列"});
//(4)重新布局表格列,否则需要设置大小
for(int i=0;i<table.getColumnCount();i++){
table.getColumn(i).pack();
}
}
//创建一个主窗口,因为shell是一个私有的变量
private void createShell(){
shell=new Shell();
shell.setText("在viewForm中创建工具栏和表格(分开写)");
shell.setLayout(new GridLayout());
createViewForm();//添加工具栏和表格
shell.pack();
}
//主函数
public static void main(String args[]){
Display display=new Display();
J thisClass=new J();
thisClass.createShell();//创建这个窗口
thisClass.shell.open();//打开这个窗口
//显示整个窗口
while(!thisClass.shell.isDisposed()){
if(!display.readAndDispatch()){
display.sleep();
}
}
display.dispose();
}
}
4.添加事件,在viewForm中创建工具栏和表格(整体写)
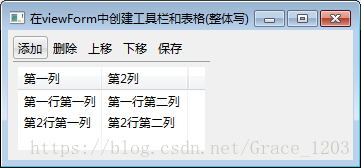
import org.eclipse.swt.SWT;
import org.eclipse.swt.widgets.*;
import org.eclipse.swt.custom.ViewForm;
import org.eclipse.swt.layout.*;
public class C{
public static void main(String[] args){
Display display=new Display();
Shell shell=new Shell(display);
shell.setText("在viewForm中创建工具栏和表格(整体写)");
shell.setLayout(new GridLayout());
//一.创建一个viewForm,在sShell中创建,用于盛放工具栏和表格
ViewForm viewForm=new ViewForm(shell,SWT.None);
//(1.1)创建一个工具栏,在viewForm中创建
ToolBar toolBar=new ToolBar(viewForm,SWT.FLAT);
//添加工具项,在工具栏中添加
final ToolItem addToolItem=new ToolItem(toolBar,SWT.PUSH);
addToolItem.setText("添加");
final ToolItem delToolItem=new ToolItem(toolBar,SWT.PUSH);
delToolItem.setText("删除");
final ToolItem backToolItem=new ToolItem(toolBar,SWT.PUSH);
backToolItem.setText("上移");
final ToolItem forwardToolItem=new ToolItem(toolBar,SWT.PUSH);
forwardToolItem.setText("下移");
final ToolItem saveToolItem=new ToolItem(toolBar,SWT.PUSH);
saveToolItem.setText("保存");
//(1.2)在viewForm中显示工具栏
viewForm.setTopLeft(toolBar);
//(2.1)创建一个面板,在viewForm中创建
Composite composite=new Composite(viewForm,SWT.None);
//设置Composite的布局
composite.setLayout(new GridLayout());
//(2.1.1)创建一个表格,在composite中创建
final Table table=new Table(composite,SWT.FULL_SELECTION);
//创建列(即表头)
TableColumn column1=new TableColumn(table,SWT.None);
column1.setText("第一列");
TableColumn column2=new TableColumn(table,SWT.None);
column2.setText("第2列");
//显示表头
table.setHeaderVisible(true);
//创建一行数据
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"第一行第一列","第一行第二列"});
item=new TableItem(table,SWT.None);
item.setText(new String[]{"第2行第一列","第2行第二列"});
//(2.1.2)重新布局表格
for(int i=0;i<table.getColumnCount();i++){
table.getColumn(i).pack();
}
//(2.2)在viewForm中显示composite面板
viewForm.setContent(composite);
//工具栏按钮的事件处理
Listener listener=new Listener(){
public void handleEvent(Event event){
if(event.widget==addToolItem){
//如果是添加按钮,则添加一条记录
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"增加一行","增加一行","增加一行"});
}
else if(event.widget==delToolItem){
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>-1){
table.remove(selectedRow);
}
}
else if(event.widget==backToolItem){//上移
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>0){
table.setSelection(selectedRow-1);
}
}
else if(event.widget==forwardToolItem){//下移
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>-1&&selectedRow<table.getItemCount()-1){
table.setSelection(selectedRow+1);
}
}
else if(event.widget==saveToolItem){
TableItem[] items=table.getItems();
//保存到文件或者数据库中,数据持久化
}
}
};
addToolItem.addListener(SWT.Selection, listener);
delToolItem.addListener(SWT.Selection, listener);
backToolItem.addListener(SWT.Selection, listener);
forwardToolItem.addListener(SWT.Selection, listener);
saveToolItem.addListener(SWT.Selection, listener);
//打开窗口,进行窗口的显示
//shell.setSize(400,400);
shell.pack();
shell.open();
while(!shell.isDisposed()){
//当窗口没有被释放的时候
if(!display.readAndDispatch()){
display.sleep();
}
}
display.dispose();
}
}
5.添加事件,在viewForm中创建工具栏和表格(分开写)
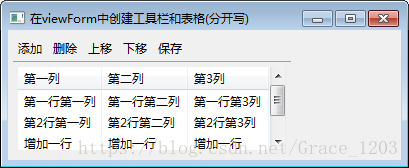
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.ViewForm;
import org.eclipse.swt.layout.*;
import org.eclipse.swt.widgets.*;
public class D{
//全局变量
private Shell shell=null;
private ViewForm viewForm=null;
private ToolBar toolBar=null;
private Table table=null;
private Composite composite=null;
//创建一个viewForm,用于放置工具栏和表格
private void createViewForm(){
viewForm=new ViewForm(shell,SWT.None);
viewForm.setLayout(new GridLayout());
//创建一个工具栏
createToolBar();
//将工具栏绑定在viewForm中
viewForm.setTopLeft(toolBar);
//创建一个面板(表格)
createComposite();
//创建一个面板,将表格显示在面板composite中
viewForm.setContent(composite);
}
//创建一个工具栏
private void createToolBar(){
toolBar=new ToolBar(viewForm,SWT.FLAT);
//在工具栏中创建工具项
final ToolItem addToolItem=new ToolItem(toolBar,SWT.PUSH);
addToolItem.setText("添加");
final ToolItem delToolItem=new ToolItem(toolBar,SWT.PUSH);
delToolItem.setText("删除");
final ToolItem backToolItem=new ToolItem(toolBar,SWT.PUSH);
backToolItem.setText("上移");
final ToolItem forwardToolItem=new ToolItem(toolBar,SWT.PUSH);
forwardToolItem.setText("下移");
final ToolItem saveToolItem=new ToolItem(toolBar,SWT.PUSH);
saveToolItem.setText("保存");
//工具栏按钮的事件处理
Listener listener=new Listener(){
public void handleEvent(Event event){
if(event.widget==addToolItem){
//如果是添加按钮,则添加一条记录
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"增加一行","增加一行","增加一行"});
}
else if(event.widget==delToolItem){
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>-1){
table.remove(selectedRow);
}
}
else if(event.widget==backToolItem){//上移
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>0){
table.setSelection(selectedRow-1);
}
}
else if(event.widget==forwardToolItem){//下移
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>-1&&selectedRow<table.getItemCount()-1){
table.setSelection(selectedRow+1);
}
}
else if(event.widget==saveToolItem){
TableItem[] items=table.getItems();
//保存到文件或者数据库中,数据持久化
}
}
};
addToolItem.addListener(SWT.Selection, listener);
delToolItem.addListener(SWT.Selection, listener);
backToolItem.addListener(SWT.Selection, listener);
forwardToolItem.addListener(SWT.Selection, listener);
saveToolItem.addListener(SWT.Selection, listener);
}
//创建一个面板
private void createComposite(){
composite=new Composite(viewForm,SWT.None);
composite.setLayout(new GridLayout());
//在面板中创建表格
createTable();
}
//创建表格,三步走
private void createTable(){
//(1)创建表格对象
//Table table=new Table(shell,SWT.HIDE_SELECTION);//仅选择第一项
table=new Table(composite,SWT.FULL_SELECTION);//选中一行
//Table table=new Table(shell,SWT.CHECK);//多选框
//(2)创建3列表头,创建表头的字符串数组
TableColumn column1=new TableColumn(table,SWT.None);
column1.setText("第一列");
TableColumn column2=new TableColumn(table,SWT.None);
column2.setText("第二列");
TableColumn column3=new TableColumn(table,SWT.None);
column3.setText("第3列");
//显示表头
table.setHeaderVisible(true);
//(3)创建一行数据
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"第一行第一列","第一行第二列","第一行第3列"});
item=new TableItem(table,SWT.None);
item.setText(new String[]{"第2行第一列","第2行第二列","第2行第3列"});
//(4)重新布局表格列,否则需要设置大小
for(int i=0;i<table.getColumnCount();i++){
table.getColumn(i).pack();
}
}
//创建一个主窗口,因为shell是一个私有的变量
private void createShell(){
shell=new Shell();
shell.setText("在viewForm中创建工具栏和表格(分开写)");
shell.setLayout(new GridLayout());
createViewForm();//添加工具栏和表格
shell.pack();
}
//主函数
public static void main(String args[]){
Display display=new Display();
D thisClass=new D();
thisClass.createShell();//创建这个窗口
thisClass.shell.open();//打开这个窗口
//显示整个窗口
while(!thisClass.shell.isDisposed()){
if(!display.readAndDispatch()){
display.sleep();
}
}
display.dispose();
}
}
6.添加菜单(单击表格,会出现删除的菜单选项)
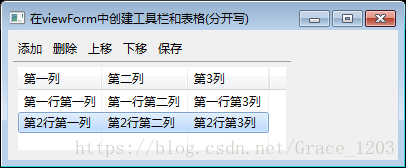
import org.eclipse.swt.SWT;
import org.eclipse.swt.custom.ViewForm;
import org.eclipse.swt.layout.*;
import org.eclipse.swt.widgets.*;
public class D{
//全局变量
private Shell shell=null;
private ViewForm viewForm=null;
private ToolBar toolBar=null;
private Table table=null;
private Composite composite=null;
private Menu menu=null;
//创建一个viewForm,用于放置工具栏和表格
private void createViewForm(){
viewForm=new ViewForm(shell,SWT.None);
viewForm.setLayout(new GridLayout());
//创建一个工具栏
createToolBar();
//将工具栏绑定在viewForm中
viewForm.setTopLeft(toolBar);
//创建一个面板(表格)
createComposite();
//创建一个面板,将表格显示在面板composite中
viewForm.setContent(composite);
}
//创建一个工具栏
private void createToolBar(){
toolBar=new ToolBar(viewForm,SWT.FLAT);
//在工具栏中创建工具项
final ToolItem addToolItem=new ToolItem(toolBar,SWT.PUSH);
addToolItem.setText("添加");
final ToolItem delToolItem=new ToolItem(toolBar,SWT.PUSH);
delToolItem.setText("删除");
final ToolItem backToolItem=new ToolItem(toolBar,SWT.PUSH);
backToolItem.setText("上移");
final ToolItem forwardToolItem=new ToolItem(toolBar,SWT.PUSH);
forwardToolItem.setText("下移");
final ToolItem saveToolItem=new ToolItem(toolBar,SWT.PUSH);
saveToolItem.setText("保存");
//工具栏按钮的事件处理
Listener listener=new Listener(){
public void handleEvent(Event event){
if(event.widget==addToolItem){
//如果是添加按钮,则添加一条记录
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"增加一行","增加一行","增加一行"});
}
else if(event.widget==delToolItem){
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>-1){
table.remove(selectedRow);
}
}
else if(event.widget==backToolItem){//上移
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>0){
table.setSelection(selectedRow-1);
}
}
else if(event.widget==forwardToolItem){//下移
//获得当前选中行的索引号
int selectedRow=table.getSelectionIndex();
//如果选中了某一行,则删除这一行
if(selectedRow>-1&&selectedRow<table.getItemCount()-1){
table.setSelection(selectedRow+1);
}
}
else if(event.widget==saveToolItem){
//TableItem[] items=table.getItems();
//保存到文件或者数据库中,数据持久化
}
}
};
addToolItem.addListener(SWT.Selection, listener);
delToolItem.addListener(SWT.Selection, listener);
backToolItem.addListener(SWT.Selection, listener);
forwardToolItem.addListener(SWT.Selection, listener);
saveToolItem.addListener(SWT.Selection, listener);
}
//创建一个面板
private void createComposite(){
composite=new Composite(viewForm,SWT.None);
composite.setLayout(new GridLayout());
//在面板中创建表格
createTable();
}
//创建表格,三步走
private void createTable(){
//(1)创建表格对象
//Table table=new Table(shell,SWT.HIDE_SELECTION);//仅选择第一项
table=new Table(composite,SWT.FULL_SELECTION);//选中一行
//Table table=new Table(shell,SWT.CHECK);//多选框
//(2)创建3列表头,创建表头的字符串数组
TableColumn column1=new TableColumn(table,SWT.None);
column1.setText("第一列");
TableColumn column2=new TableColumn(table,SWT.None);
column2.setText("第二列");
TableColumn column3=new TableColumn(table,SWT.None);
column3.setText("第3列");
//显示表头
table.setHeaderVisible(true);
//(3)创建一行数据
TableItem item=new TableItem(table,SWT.None);
item.setText(new String[]{"第一行第一列","第一行第二列","第一行第3列"});
item=new TableItem(table,SWT.None);
item.setText(new String[]{"第2行第一列","第2行第二列","第2行第3列"});
//(4)重新布局表格列,否则需要设置大小
for(int i=0;i<table.getColumnCount();i++){
table.getColumn(i).pack();
}
}
//创建一个主窗口,因为shell是一个私有的变量
private void createShell(){
shell=new Shell();
shell.setText("在viewForm中创建工具栏和表格(分开写)");
shell.setLayout(new GridLayout());
createViewForm();//添加工具栏和表格
createMenu();//添加一个菜单
shell.pack();
}
//创建一个菜单
private void createMenu(){
//创建一个弹出式菜单
menu=new Menu(shell,SWT.POP_UP);
//创建一个菜单项
MenuItem delMenuItem=new MenuItem(menu,SWT.None);
delMenuItem.setText("删除");
//将菜单添加到表格上,即创建一个表格菜单
table.setMenu(menu);
//为删除注册事件
delMenuItem.addListener(SWT.Selection,new Listener(){
public void handleEvent(Event event){
//获得被选择的文本
TableItem[] items=table.getSelection();
for(int i=0;i<items.length;i++){
System.out.println(items[i].getText());
}
//获得被选择的表格项的索引
int SelectionIndex=table.getSelectionIndex();
if(SelectionIndex>-1){
table.remove(SelectionIndex);
}
}
});
}
//主函数
public static void main(String args[]){
Display display=new Display();
D thisClass=new D();
thisClass.createShell();//创建这个窗口
thisClass.shell.open();//打开这个窗口
//显示整个窗口
while(!thisClass.shell.isDisposed()){
if(!display.readAndDispatch()){
display.sleep();
}
}
display.dispose();
}
}