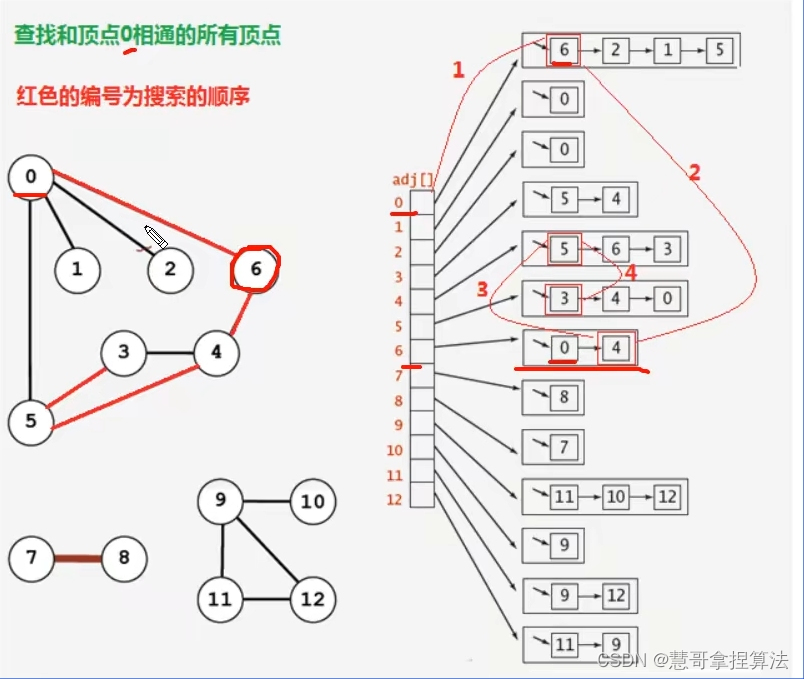
import java.util.Iterator;
public class Queue<T> implements Iterable<T> {
private Node<T> head;
private Node<T> last;
private int N;
private class Node<T> {
private T item;
private Node<T> next;
public Node(T item,Node<T> next){
this.item = item;
this.next = next;
}
}
public Queue(){
this.head = new Node<T>(null,null) ;
this.last = null;
this.N = 0;
}
public boolean isEmpty(){
return N==0;
}
public int size(){
return N;
}
public void enqueue(T t){
if(last==null){
last = new Node<T> (t,null);
head.next = last;
}else{
Node<T> oldLast = last;
last = new Node<T>(t,null);
oldLast.next = last;
}
N++;
}
public T dequeue(){
if(isEmpty()) return null;
Node<T> oldFirst = head.next;
head.next = oldFirst.next;
N--;
if(isEmpty()) last = null;
return oldFirst.item;
}
@Override
public Iterator iterator() {
return new QIterator();
}
private class QIterator implements Iterator{
private Node<T> n;
public QIterator(){
this.n = head;
}
@Override
public boolean hasNext() {
return n.next != null;
}
@Override
public T next() {
n = n.next;
return n.item;
}
}
}
public class Graph {
private int v;
private int E;
private Queue<Integer>[] adj;
public Graph(int v) {
this.v = v;
this.E = 0;
this.adj = new Queue[v];
for (int i = 0; i < adj.length; i++) {
adj[i] = new Queue<Integer>();
}
}
public int v(){
return v;
}
public int E(){
return E;
}
public void addEdge(int v,int w){
adj[v].enqueue(w);
adj[w].enqueue(v);
E++;
}
public Queue<Integer> adj(int v){
return adj[v];
}
}
import com.example.demo.code10.queue.Queue;
public class DepthFirstSearch {
private boolean[] marked;
private int count;
public DepthFirstSearch(Graph g,int s) {
this.marked = new boolean[g.v()];
this.count = 0;
System.out.print(s+" ");
dfs(g,s);
}
private void dfs(Graph g, int s) {
marked[s] = true;
for (Integer w : g.adj(s)) {
if(!marked[w]){
dfs(g,w);
System.out.print(w+" ");
}
}
count ++;
}
public boolean marked(int w){
return marked[w];
}
public int count(){
return count;
}
}
public class DepthFirstSearchTest {
public static void main(String[] args) {
Graph graph = new Graph(13);
graph.addEdge(0,5);
graph.addEdge(0,1);
graph.addEdge(0,2);
graph.addEdge(0,6);
graph.addEdge(5,3);
graph.addEdge(5,4);
graph.addEdge(3,4);
graph.addEdge(4,6);
graph.addEdge(7,8);
graph.addEdge(9,11);
graph.addEdge(9,10);
graph.addEdge(9,12);
graph.addEdge(11,12);
DepthFirstSearch search = new DepthFirstSearch(graph, 0);
System.out.println();
System.out.println(search.count());
System.out.println(search.marked(7));
System.out.println(search.marked(5));
}
}
BFS
package com.example.demo.code60;
import com.example.demo.code10.queue.Queue;
import java.util.LinkedList;
public class BreadFirstSearch {
private boolean [] marked;
private int count;
private Queue<Integer> waitSearch;
public BreadFirstSearch(Graph g,int s ) {
this.marked = new boolean[g.v()];
this.count = 0;
this.waitSearch = new Queue<Integer>();
bfs(g,s);
}
private void bfs(Graph g, int s) {
marked[s] = true;
waitSearch.enqueue(s);
while (!waitSearch.isEmpty()) {
Integer wait = waitSearch.dequeue();
Queue<Integer> adj = g.adj(wait);
for (Integer w : adj) {
if(!marked[w]) {
waitSearch.enqueue(w);
marked[w] = true;
count++;
System.out.print(w+" ");
}
}
}
}
public static void main(String[] args) {
Graph graph = new Graph(13);
graph.addEdge(0,5);
graph.addEdge(0,1);
graph.addEdge(0,2);
graph.addEdge(0,6);
graph.addEdge(5,3);
graph.addEdge(5,4);
graph.addEdge(3,4);
graph.addEdge(4,6);
graph.addEdge(7,8);
graph.addEdge(9,11);
graph.addEdge(9,10);
graph.addEdge(9,12);
graph.addEdge(11,12);
BreadFirstSearch search = new BreadFirstSearch(graph,0);
}
}