服务器相关概念
1.服务器
2.IP地址
3.域名
4.http协议的作用
5.http协议报文
请求行 | 存放当前请求地址 , get或post请求 |
---|
请求体 | 存放 提交给后台的参数 , post 帐号密码 |
请求头 | token 登陆凭证 , 当前浏览器相关信息 |
响应行 | http状态码是什么 , 200 成功.404找不到.500服务器出错 |
---|
响应体 | 响应客户端请求数据 |
响应头 | 告诉浏览器当前的返回的内容的类型是什么 |
核心模块 path
- 处理路径
- path.join 拼接
- __dirname 返回当前文件的绝对路径
1.拼接路径
const path = require("path");
const url = path.join("data", "image", "2.json");
console.log(url);
2.path 获取文件绝对路径
const path = require('path');
const url = path.join('data', 'images', '2.jpg');
console.log(__dirname);
const myfile = path.join(__dirname, './01 - path获取文件绝对路径.js');
console.log(myfile);
3.开启一个服务器
const http = require('http');
const app = http.createServer((req, res) => {
res.end('我是你爸爸真伟大,养你这么大');
});
app.listen(8001, () => {
console.log('第二个服务器');
});
4-响应对象response常用属性
const http = require('http');
const app = http.createServer((request, response) => {
response.setHeader('Content-Type', 'text/ plain;charset=utf-8');
response.statusCode = 200;
response.end('我是你爸爸真伟大');
});
app.listen(8001, () => {
console.log('8001 开启成功');
});
5.返回随机数
const http = require('http');
const app = http.createServer((request, response) => {
console.log(response);
if (request.url === 'random') {
response.end(Math.random() + '');
} else {
response.statusCode = 404;
}
});
app.listen(8001, () => {
console.log('8001,开启');
6.服务器提供静态资源
const http = require('http');
const fs = require('fs');
const app = http.createServer((req, res) => {
if (req.url === '/index.html') {
res.setHeader('Content-Type', ' text/html');
let index = fs.readFileSync('./public/index.html', 'utf-8');
res.end(index);
} else if (req.url === '/chart.html') {
res.setHeader('Content-Type', ' text/html');
let chart = fs.readFileSync('./public/chart.html', 'utf8');
res.end(chart);
} else if (req.url === '/css/index.css') {
res.setHeader('Content-Type', 'text/css');
let css = fs.readFileSync('./css/index.css', 'utf8');
res.end(css);
} else if (req.url === '/js/index.js') {
res.setHeader('Content-Type', 'application/x-javascript');
let js = fs.readFileSync('./js/index.js', 'utf8');
res.end(js);
} else if (req.url === '/images/5f8de1ffa140a12d88f1131a64114897.png') {
res.setHeader('Content-Type', ' application/x-img');
let img = fs.readFileSync('./images/5f8de1ffa140a12d88f1131a64114897.png');
res.end(img);
} else {
res.statusCode = 404;
res.end('');
}
});
app.listen(8001, () => {
console.log('8001,开启');
});
优化代码
const http = require('http');
const fs = require('fs');
const app = http.createServer((req, res) => {
const url = req.url;
console.log(url);
try {
const file = fs.readFileSync('public' + url);
res.end(file);
} catch (error) {
res.statusCode = 404;
res.end('');
}
});
app.listen(8001, () => {
console.log('8001');
});
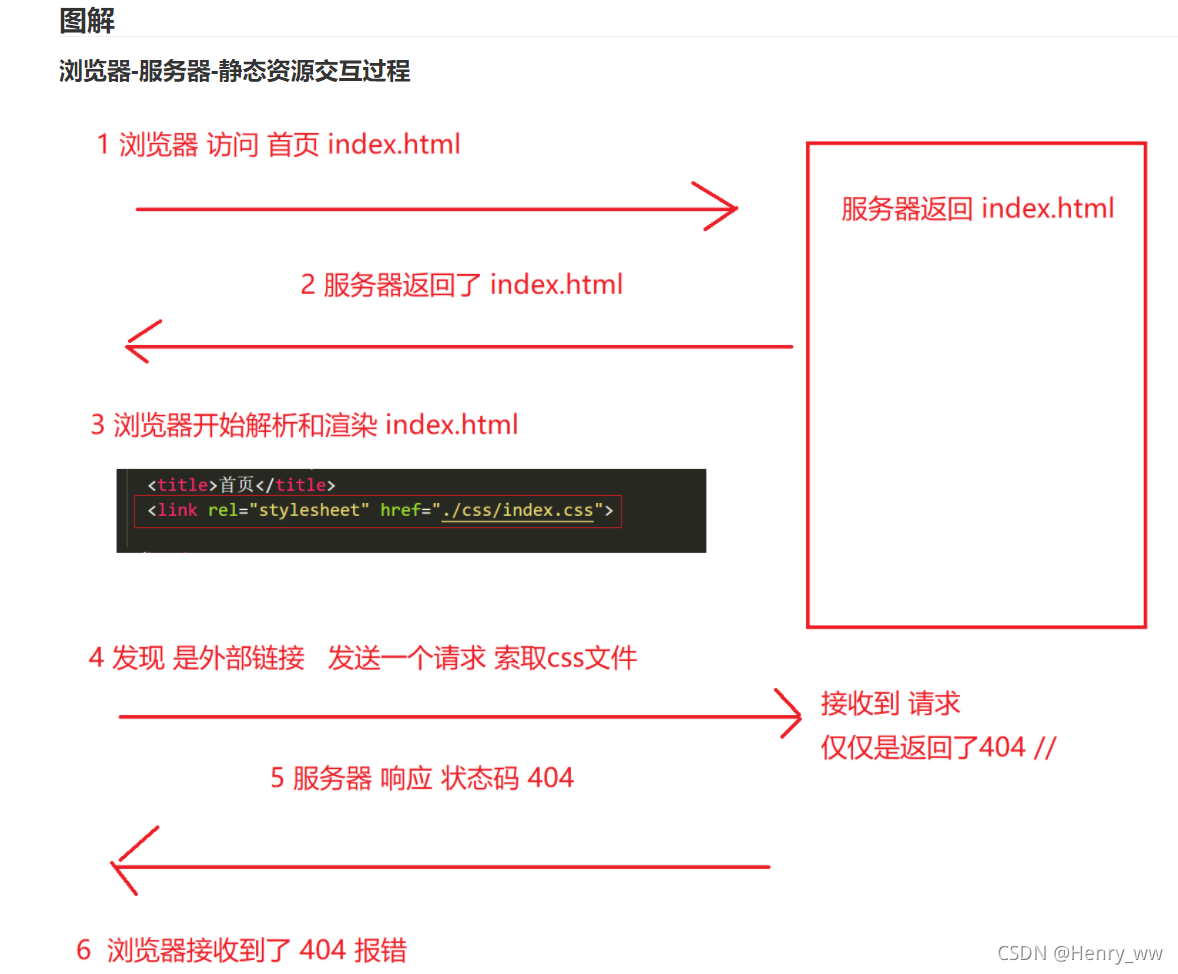