public class HorseChessboard {
private static int X;// 棋盘的列
private static int Y;// 棋盘的行
// 标记棋盘各个位置是否被访问
private static boolean visited[];
// 标记棋盘全部位置是否都被访问
private static boolean finished;
public static void main(String[] args) {
X = 8;
Y = 8;
int row = 1;// 马儿初始位置的行
int column = 1;// 马儿初始位置的列
// 创建棋盘
int[][] chessboard = new int[X][Y];
visited = new boolean[X * Y];// 初始值都是flase
// 测试
System.out.println("骑士周游开始运算~~");
long start = System.currentTimeMillis();
traversalChessboard(chessboard, row - 1, column - 1, 1);
long end = System.currentTimeMillis();
System.out.println("总耗时:" + (end - start) + " 毫秒");
// 输出棋盘
for (int[] rows : chessboard) {
for (int step : rows) {
System.out.print(step + "\t");
}
System.out.println();
}
}
/**
* 完成骑士周游问题的算法
*
* @param chessboard 棋盘
* @param row 马儿当前位置的行 0开始
* @param column 马儿当前位置的列 0开始
* @param step 是第几步 初始1
*/
public static void traversalChessboard(int[][] chessboard, int row, int column, int step) {
chessboard[row][column] = step;
// row = 4*8 column = 4 马儿当前位置:4*8+4+1-1 = 36
visited[row * X + column] = true;// 标记当前位置已经访问
// 获取当前位置可以走的下一个位置的集合
ArrayList<Point> ps = next(new Point(column, row));
// 对ps进行排序,对ps所有对象Point对象的下一个的位置的数目进行非递归排序
sort(ps);
// 遍历ps
while (!ps.isEmpty()) {
Point p = ps.remove(0);// 取出下一个可以走的位置
// 判断该点是否已经访问过
if (!visited[p.y * X + p.x]) {// 说明还未访问过
traversalChessboard(chessboard, p.y, p.x, step + 1);
}
}
// 判断马儿是否完成了任务, 使用step和应该走的步数比较
// 如果还没达到数量,这表示没有完成任务,将整个棋盘置0
// 说明: step < X*Y 情况有两种
// 1. 棋盘到目前位置,仍然还没走完
// 2. 棋盘处于回溯状态
if (step < X * Y && !finished) {
chessboard[row][column] = 0;//清空该位置的步数
visited[row * X + column] = false; //让该位置为未被访问
} else {
finished = true;
}
}
/**
* 根据当前位置(Point对象),计算马儿还能走哪些位置(Piont),并放入到一个集合中(ArraysList),最多有8个位置
*
* @param curPoint
* @return
*/
public static ArrayList<Point> next(Point curPoint) {
// 创建一个ArraysList
ArrayList<Point> ps = new ArrayList<Point>();
// 创建一个p1
Point p1 = new Point();
// 表示马儿可以走5这个位置
if ((p1.x = curPoint.x - 2) >= 0 && (p1.y = curPoint.y - 1) >= 0) {
ps.add(new Point(p1));
}
// 表示马儿可以走6这个位置
if ((p1.x = curPoint.x - 1) >= 0 && (p1.y = curPoint.y - 2) >= 0) {
ps.add(new Point(p1));
}
// 表示马儿可以走7这个位置
if ((p1.x = curPoint.x + 1) < X && (p1.y = curPoint.y - 2) >= 0) {
ps.add(new Point(p1));
}
// 表示马儿可以走0这个位置
if ((p1.x = curPoint.x + 2) < X && (p1.y = curPoint.y - 1) >= 0) {
ps.add(new Point(p1));
}
// 表示马儿可以走1这个位置
if ((p1.x = curPoint.x + 2) < X && (p1.y = curPoint.y + 1) < Y) {
ps.add(new Point(p1));
}
// 表示马儿可以走2这个位置
if ((p1.x = curPoint.x + 1) < X && (p1.y = curPoint.y + 2) < Y) {
ps.add(new Point(p1));
}
// 表示马儿可以走3这个位置
if ((p1.x = curPoint.x - 1) >= 0 && (p1.y = curPoint.y + 2) < Y) {
ps.add(new Point(p1));
}
// 表示马儿可以走4这个位置
if ((p1.x = curPoint.x - 2) >= 0 && (p1.y = curPoint.y + 1) < Y) {
ps.add(new Point(p1));
}
return ps;
}
//根据当前这个一步的所有下一步的选择位置,进行非递减排序,减少回溯
public static void sort(ArrayList<Point> ps) {
ps.sort(new Comparator<Point>() {
@Override
public int compare(Point o1, Point o2) {
// TODO Auto-generated method stub
int count1 = next(o1).size();
int count2 = next(o2).size();
if(count1 < count2) {
return -1;
}else if(count1 == count2) {
return 0;
}else {
return -1;
}
}
});
}
}
关于马踏棋盘算法(为什么在70行当finished等于true时,就会退出运行)
于 2024-02-29 22:47:57 首次发布
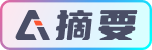