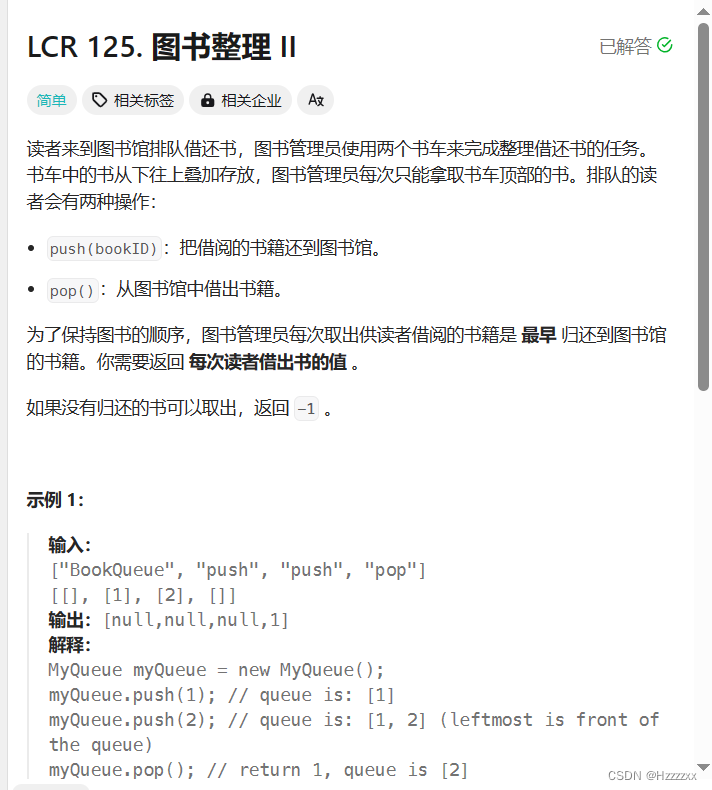
class CQueue {
public:
stack<int> st1;
stack<int> st2;
CQueue() {
}
void appendTail(int value) {
st1.push(value);
}
int deleteHead() {
if(st1.empty()) return -1;
while(!st1.empty())
{
int tmp = st1.top();
st1.pop();
st2.push(tmp);
}
int res = st2.top();
st2.pop();
while(!st2.empty())
{
int tmp = st2.top();
st2.pop();
st1.push(tmp);
}
return res;
}
};
/**
* Your CQueue object will be instantiated and called as such:
* CQueue* obj = new CQueue();
* obj->appendTail(value);
* int param_2 = obj->deleteHead();
*/
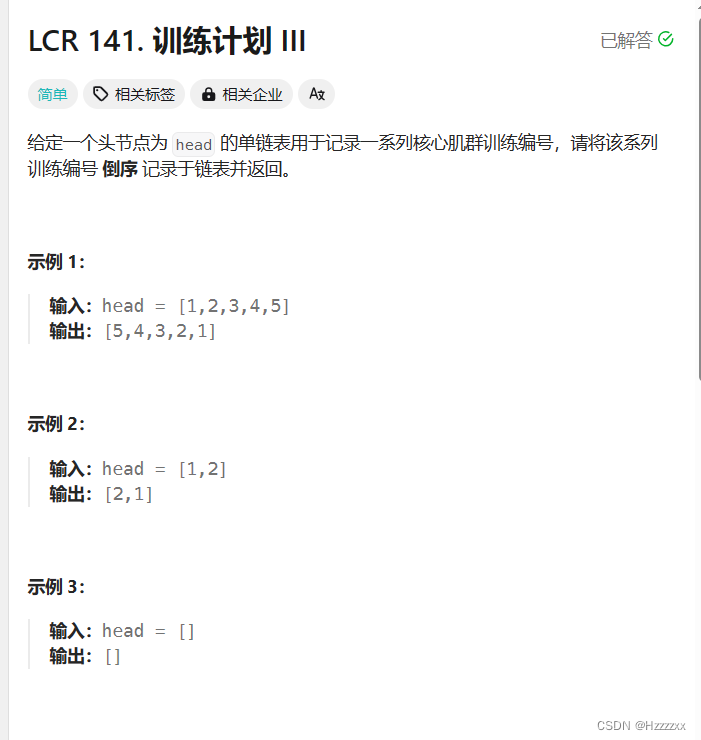
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* trainningPlan(ListNode* head) {
ListNode *cur = head;
ListNode *pre = nullptr;
while(cur)
{
ListNode* tmp = cur->next;
cur->next = pre;
pre = cur;
cur = tmp;
}
return pre;
}
};
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* trainningPlan(ListNode* head) {
return recur(head,nullptr);
}
ListNode* recur(ListNode* cur,ListNode* pre)
{
if(!cur) return pre;
ListNode* res = recur(cur->next,cur);
cur->next = pre;
return res;
}
};