/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode* deleteNode(ListNode* head, int val) {
ListNode* cur = head;
ListNode* pre = nullptr;
if(head->val == val) return head->next;
while(cur)
{
if(cur->val == val)
{
pre->next = cur->next;
break;
}
pre = cur;
cur = cur->next;
}
return head;
}
};
leetcode每日一题第六十二天
最新推荐文章于 2024-10-31 16:16:13 发布
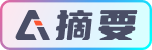