前端实现计算器。。应该不难。
第一步:先建个项目:
项目结构如下:
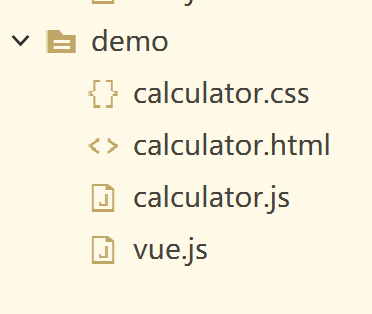
注意:这个vue.js懂的都懂,官网下载或者一些编译器都自带的,这个不放代码了。
不多说,其他直接上代码。
第二步:html代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="calculator.css">
</head>
<body>
<div id="app">
<table id="cal">
<tr>
<td id="output" colspan="4">{{ output }}</td>
</tr>
<tr v-for="row in keys">
<td v-if="key.value===0" :colspan="2" v-for="key in row" @click="keyClick(key)" :class="{'bg-yellow': key.type === 'op', 'bg-gray': key.type === 'num'}">
{{ key.label }}</td>
<td v-if="key.value!=0" v-for="key in row" @click="keyClick(key)" :class="{'bg-yellow': key.type === 'op', 'bg-gray': key.type === 'num'}">
{{ key.label }}</td>
</tr>
</table>
</div>
<script src="vue.js"></script>
<script src="calculator.js"></script>
</body>
</html>
第三步:CSS代码:
.bg-yellow {
background-color: #FF9C34 !important;
}
.bg-gray {
background-color: #62645D !important;
}
#cal {
margin: unset;
}
#cal #output {
background-color: #222 !important;
text-align: right;
font-size: 3em;
font-weight: bold;
padding: 0px .2em;
height: 2em;
}
#cal td {
font-size: 1.5rem;
font-family: Arial;
width: 3em;
height: 2.8em;
background-color: #333;
color: white;
text-align: center;
vertical-align: middle;
border-radius: 20px;
}
第四步:JS代码:
new Vue({
el: '#app',
data: {
output: 0,
str: '',
b: false,
d: false,
count: 0,
expr: '',
keys: [
[{
label: 'AC',
value: 'reset',
type: 'reset'
},
{
label: '+/-',
value: 'sign',
type: 'negate'
},
{
label: '%',
value: '%',
type: 'op'
},
{
label: '÷',
value: '/',
type: 'op'
}
],
[{
label: '7',
value: 7,
type: 'num'
},
{
label: '8',
value: 8,
type: 'num'
},
{
label: '9',
value: 9,
type: 'num'
},
{
label: '×',
value: '*',
type: 'op'
}
],
[{
label: '4',
value: 4,
type: 'num'
},
{
label: '5',
value: 5,
type: 'num'
},
{
label: '6',
value: 6,
type: 'num'
},
{
label: '+',
value: '+',
type: 'op'
}
],
[{
label: '1',
value: 1,
type: 'num'
},
{
label: '2',
value: 2,
type: 'num'
},
{
label: '3',
value: 3,
type: 'num'
},
{
label: '-',
value: '-',
type: 'op'
}
],
[{
label: '0',
value: 0,
type: 'num'
},
{
label: '',
value: "",
type: 'num'
},
{
label: '.',
value: '.',
type: 'num'
},
{
label: '=',
value: '=',
type: 'exec'
}
],
]
},
methods: {
keyClick: function(key) {
switch (key.type) {
case 'num':
case 'op':
this.expr += key.value;
s = this.changeNumber(key.value)
this.expr = s
this.output = this.expr;
break;
case 'exec':
this.d = true
this.output = eval(this.expr);
this.str = this.output + ''
break;
case 'reset':
this.expr = '';
this.str = '';
this.output = 0;
break;
case 'negate':
this.str = "-1*(" + this.str + ")";
this.output = eval(this.str);
this.str = this.output + "";
break;
}
},
changeNumber: function(num) {
if (this.d == true && typeof num == "number") {
this.str = num + '';
this.d = false;
return this.str;
} else {
this.d = false;
if (this.str.length == 0) {
if (num == 0) {
this.str = "0";
return this.str;
} else {
this.str += num;
return this.str;
}
} else {
if (num == 0) {
if (this.str == "0") {
this.str = "";
this.str += num;
return this.str;
} else {
a = this.str.charAt(this.str.length - 2) //判断前一位是否是符号
c = this.isSign(a)
if (this.str.charAt(this.str.length - 1) == "0" && c) {
if (a == '.') {
this.str += num;
return this.str;
} else {
this.str = this.str
return this.str;
}
} else {
if (this.str.charAt(this.str.length - 1) == ".") {
this.str += num;
return this.str;
} else {
this.str += num;
return this.str;
}
}
}
} else if (num != 0 && typeof num == "number") {
if (this.str == "0") {
this.str = "";
this.str += num;
return this.str;
} else {
if (this.str.charAt(this.str.length - 1) == "0" && !this.havePoint(this.str)) {
this.str = this.str.substring(0, this.str.length - 1) + num;
return this.str;
} else {
this.str += num;
return this.str;
}
}
} else {
this.str = this.str + ''
s = this.str.charAt(this.str.length - 1);
this.b = this.isSign(s)
if (this.b && num != '.') {
if (this.isS(num)) {
temp = this.str.charAt(this.str.length - 1)
if (temp == num) {
this.str = this.str.substring(0, this.str.length - 1) + num;
return this.str;
} else {
if (this.havePoint(this.str)) {
this.str += num;
return this.str;
} else {
this.str = this.str.substring(0, this.str.length - 1) + num;
return this.str;
}
}
} else {
this.str = this.str.substring(0, this.str.length - 1) + num;
return this.str;
}
} else {
if (this.str.charAt(this.str.length - 1) == '.' && num == '.') {
this.str = this.str;
return this.str;
} else {
is = this.havePoint(this.str)
if (is && num == '.') {
this.str = this.str + '';
return this.str;
} else {
this.str += num;
return this.str;
}
}
}
}
}
}
},
havePoint: function(string) {
n = string.length - 1;
while (n > 0) {
if (string.charAt(n) == '.') {
return true;
} else {
s = string.charAt(n)
if (this.isSign(s)) {
return false
} else {
n--;
}
}
}
},
isSign: function(s) {
if (s == '+' || s == '-' || s == '*' || s == '/' || s == '%' || s == '.') {
return true;
} else {
return false;
}
},
isAB: function(strs, num) {
if (strs.charAt(strs.length - 1) == num) {
return true
} else {
return false
}
},
isS: function(s) {
if (s == '+' || s == '-' || s == '*' || s == '/' || s == '%') {
return true;
} else {
return false;
}
},
}
})
最终的结果:
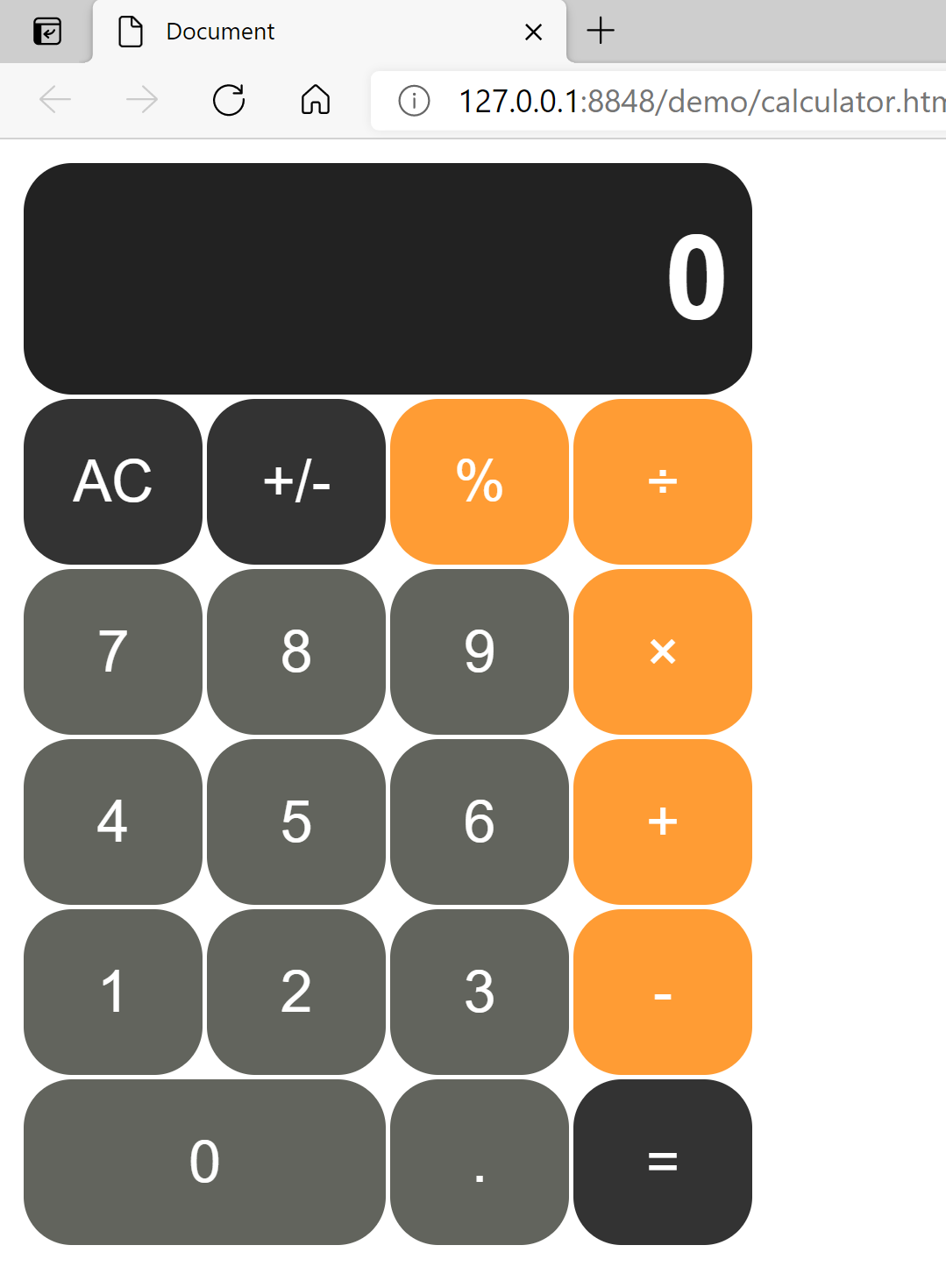
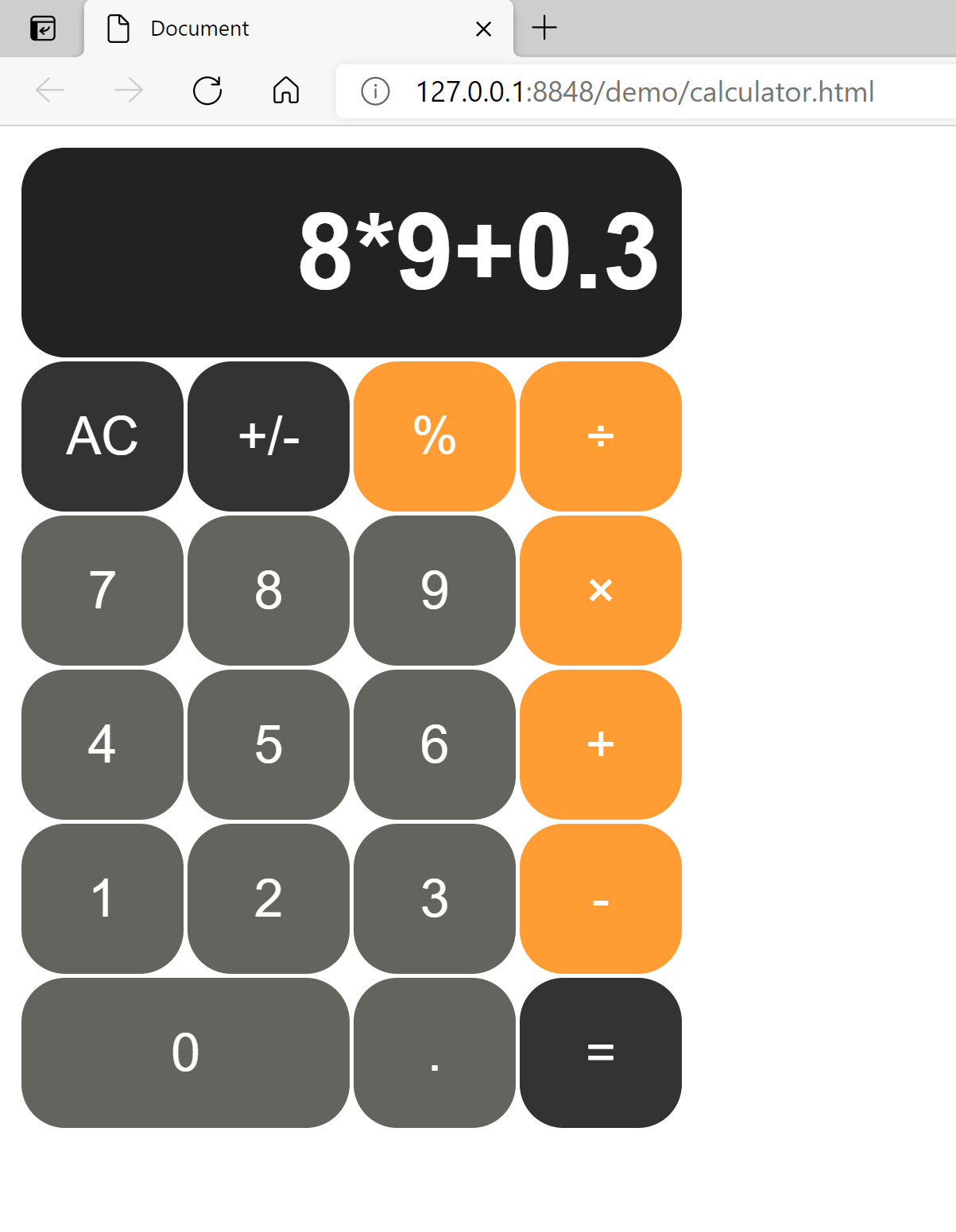
搞定苹果计算器。
谢谢阅读,无误点赞,有误还望评论区指正。