原理图
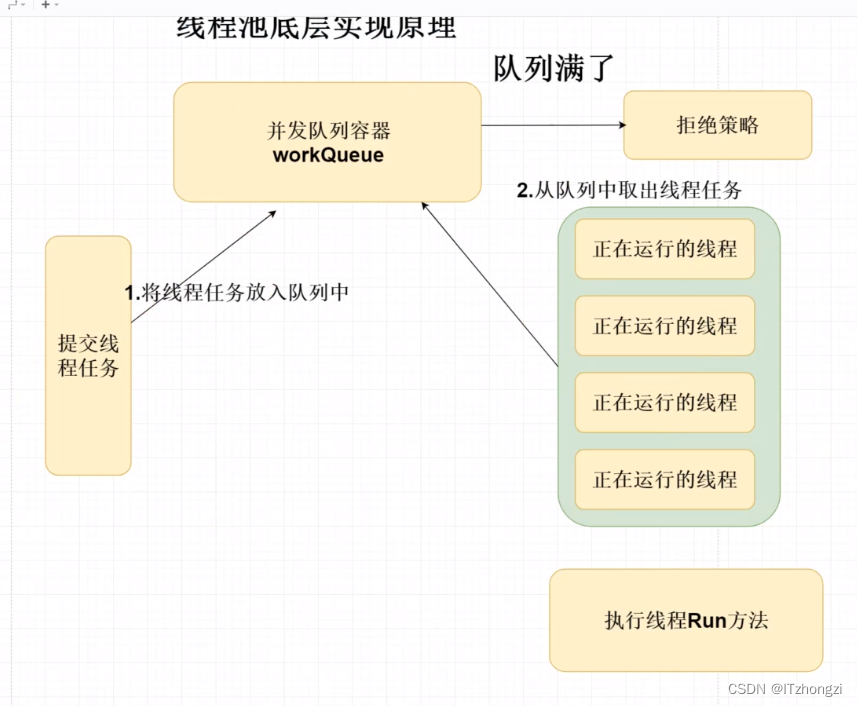
原理解析
- 提交任务到队列容器
- 开启多个一直运行的线程
- 线程从队列容器中取出任务执行
核心代码
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.BlockingDeque;
import java.util.concurrent.LinkedBlockingDeque;
public class MyExecutors {
private BlockingDeque<Runnable> runnableDueue;
private List<WorkThread> threadList;
public boolean isRun = true;
public MyExecutors(int maxThreadCount, int queueSize) {
runnableDueue = new LinkedBlockingDeque<Runnable>(queueSize);
threadList = new ArrayList<WorkThread>(maxThreadCount);
for (int i = 0; i < maxThreadCount; i++) {
new WorkThread().start();
}
}
class WorkThread extends Thread {
@Override
public void run() {
System.out.println("创建一个线程:" + Thread.currentThread().getName());
while (isRun || runnableDueue.size() > 0) {
Runnable task = runnableDueue.poll();
if (task != null) {
task.run();
}
}
}
}
public boolean execute(Runnable command) {
return runnableDueue.offer(command);
}
public static void main(String[] args) {
MyExecutors myExecutors = new MyExecutors(2, 10);
for (int i = 0; i < 10; i++) {
int finalI = i;
myExecutors.execute(() -> System.out.println(Thread.currentThread().getName() + "," + finalI));
}
myExecutors.isRun = false;
}
}