c#ATM之功能篇(存钱,取钱,转账,交易明细)完结篇带详细注释。一共七个功能,不难,代码还有优化空间,之后再发一篇ATM之优化篇。
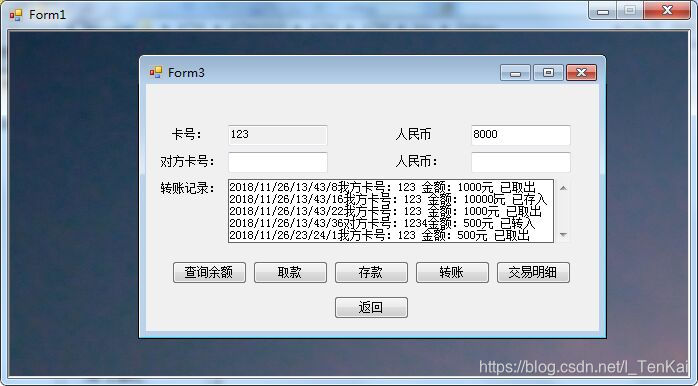
首先先添加一条命名空间
1
| using System.Data.SqlClient;
|
1 2 3 4 5 6 7 8 9 10 11 12
| public Form3() { InitializeComponent();
} public Form3(string str)//构造一个函数用来接收来自Form1的卡号 { InitializeComponent(); textBox1.Text = str; //MessageBox.Show(str);打印从form1传来的参数 验证是不是登录时的卡号 }
|
1, button1查询余额的功能
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| private void button1_Click(object sender, EventArgs e) { //连接数据库字符串 string count = "SERVER=.;DATABASE=OX;USER=SA;PWD=1;Integrated Security=True;"; //执行sql语句 string sql = "select * from DATA where ID='" + textBox1.Text.Trim() + "'"; SqlConnection con = new SqlConnection(count); con.Open(); //创建操作对象cmd SqlCommand cmd = new SqlCommand(sql, con); SqlDataReader dr = cmd.ExecuteReader(); if (dr.HasRows) { dr.Read(); //读取余额填充到textBox2.Text中 textBox2.Text = dr["MORY"].ToString().Trim(); //有打开就要有关闭哦 dr.Close(); con.Close(); }
|
2, button2取钱功能
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64
| //取钱的功能:→要判断自己有多少钱,能取的钱不能大于自己的本金,钱不能取负数 private void button2_Click(object sender, EventArgs e) { //先判断textBox2.Text等不等于空,不等于空就执行else里面的语块句 if (textBox2.Text=="") { MessageBox.Show("请输入金额"); } else { string count = "SERVER=.;DATABASE=OX;USER=SA;PWD=1;Integrated Security=True;"; string sql01 = "select * from DATA where ID='" + textBox1.Text.Trim() + "'"; SqlConnection con01 = new SqlConnection(count); con01.Open(); SqlCommand cmd01 = new SqlCommand(sql01, con01); SqlDataReader dr01 = cmd01.ExecuteReader(); dr01.Read(); //Convert.表示强制转换的意思 ToInt32表示类型 类型可以换 int i = Convert.ToInt32(dr01["mory"].ToString().Trim()); //将string类型的文本框强制转换成int类型,思考一下,不用这类强制转换,还有哪些方法可以强制转换 int j = Convert.ToInt32(textBox2.Text); int k = i - j; int txt = Convert.ToInt32(textBox2.Text); dr01.Close(); if (txt > i | txt < 0) { MessageBox.Show("取出来的钱不能大于本金或为负数", "温馨提示"); } else { //原来的本金减去要取出来的金额 //先读取原来的本金为i,再获得取款の为j 即i-j=k 输入数据库 , string NOW = "";//先申明一个空变量来接收时间信息 string count01 = "server=.;database=ox;user=sa;pwd=1;Integrated Security=True;"; string sql = "select * from DATA"; SqlConnection con = new SqlConnection(count); con.Open(); SqlCommand cmd = new SqlCommand(sql, con); SqlDataReader dr = cmd.ExecuteReader(); if (dr.HasRows) { dr.Read(); /*-----------先读取之前转账的信息------------*/ NOW = dr["RECORD"].ToString().Trim(); dr.Close(); con.Close(); } /*------插入储存信息格式------*/ NOW += DateTime.Now.Year + "/" + DateTime.Now.Month + "/" + DateTime.Now.Day + "/" + DateTime.Now.Hour + "/" + DateTime.Now.Minute + "/" + DateTime.Now.Second; NOW += "我方卡号:" + textBox1.Text; NOW += " 金额:" + textBox2.Text + "元 " + "已取出";
string sql02 = "update DATA set mory='" + k + "',RECORD='" + NOW + "'where ID='" + textBox1.Text.Trim() + "'"; SqlConnection con02 = new SqlConnection(count01); con02.Open(); SqlCommand cmd02 = new SqlCommand(sql02, con02); SqlDataReader dr02 = cmd02.ExecuteReader(); dr02.Read(); MessageBox.Show("取款成功"); dr02.Close(); con02.Close();
} } }
|
3, textBox2_KeyPress屏蔽要字母,有这段代码就是文本框里面不能输入字母和符号
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| private void textBox2_KeyPress(object sender, KeyPressEventArgs e) { if (e.KeyChar > 0x20) { try { double.Parse(((TextBox)sender).Text + e.KeyChar.ToString()); } catch { e.KeyChar = (char)0; //处理非法字符 } } }
|
4, textBox3_KeyPress屏蔽要字母,有这段代码就是文本框里面不能输入字母和符号
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| private void textBox3_KeyPress(object sender, KeyPressEventArgs e) { if (e.KeyChar > 0x20) { try { double.Parse(((TextBox)sender).Text + e.KeyChar.ToString()); } catch { e.KeyChar = (char)0; //处理非法字符 } } }
|
5, textBox4_KeyPress屏蔽要字母,有这段代码就是文本框里面不能输入字母和符号
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| private void textBox4_KeyPress(object sender, KeyPressEventArgs e) { if (e.KeyChar > 0x20) { try { double.Parse(((TextBox)sender).Text + e.KeyChar.ToString()); } catch { e.KeyChar = (char)0; //处理非法字符 } } }
|
6, button3存钱功能
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
| private void button3_Click(object sender, EventArgs e) { int txt = Convert.ToInt32(textBox2.Text); if (txt < 0) { MessageBox.Show("不能存小于0的金额"); } else { string NOW=""; string count = "server=.;database=ox;user=sa;pwd=1;Integrated Security=True;"; string sql = "select * from DATA"; SqlConnection con = new SqlConnection(count); con.Open(); SqlCommand cmd = new SqlCommand(sql, con); SqlDataReader dr = cmd.ExecuteReader(); if (dr.HasRows) { dr.Read(); /*-----------先读取之前转账的信息------------*/ NOW = dr["RECORD"].ToString().Trim(); //NOW1 = dr["RECORD"].ToString().Trim();
} dr.Close(); con.Close(); /*------插入储存信息格式------*/ NOW += DateTime.Now.Year + "/" + DateTime.Now.Month + "/" + DateTime.Now.Day + "/" + DateTime.Now.Hour + "/" + DateTime.Now.Minute + "/" + DateTime.Now.Second; NOW += "我方卡号:" + textBox1.Text; NOW += " 金额:" + textBox2.Text + "元 " + "已存入";
/*-----------先判断自己有多少本金再加上要存储的金额------等这些计算好了在存进数据中-------*/ string count01 = "SERVER=.;DATABASE=OX;USER=SA;PWD=1;Integrated Security=True;"; string sql01 = "select * from DATA where ID='" + textBox1.Text.Trim() + "'"; SqlConnection con01 = new SqlConnection(count01); con01.Open(); SqlCommand cmd01 = new SqlCommand(sql01, con01); SqlDataReader dr01 = cmd01.ExecuteReader(); dr01.Read(); int i = Convert.ToInt32(dr01["mory"].ToString().Trim());//读取自己的账户有多少钱 int j = Convert.ToInt32(textBox2.Text);//接受要储存的金额 int k = i + j;//本金加要储存的金额 dr01.Close(); con01.Close(); /*------------------------*/ string sql02 = "update DATA set mory='" + k + "',RECORD='"+NOW+"'where ID='" + textBox1.Text.Trim() + "'"; SqlConnection con02 = new SqlConnection(count); con02.Open(); SqlCommand cmd02 = new SqlCommand(sql02, con02); if (cmd02.ExecuteNonQuery() > 0) { MessageBox.Show("储存成功"); } else { MessageBox.Show("Test"); } con02.Close(); } }
|
7.,button4转账功能
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105
| private void button4_Click(object sender, EventArgs e) { if (textBox4.Text==""&textBox3.Text=="") { MessageBox.Show("请输入转账信息"); } else { textBox3.Visible = true; label3.Visible = true; textBox4.Visible = true; label4.Visible = true; int i2, text2, text4; if (true) { string count = "SERVER=.;USER=SA;PWD=1;DATABASE=OX;Integrated Security=True;"; string sql = "select * from DATA where ID='" + textBox1.Text + "'"; SqlConnection cno = new SqlConnection(count); SqlCommand cmd = new SqlCommand(sql, cno); cno.Open(); SqlDataReader dr = cmd.ExecuteReader(); dr.Read(); //I1-TXT=自己账号的本金更新数据 txt+i2=对方的本金 更新数据 int i1 = Convert.ToInt32(dr["mory"].ToString().Trim());//获取自己的本金 i2 = i1; dr.Close(); cno.Close(); //text4 = textBox4.Text; int txt4 = Convert.ToInt32(textBox4.Text);//要转账的金额 int txt2 = Convert.ToInt32(textBox2.Text); text2 = txt2; text4 = txt4; } if (/*text2 > i2 | text2 < 0 |*/ text4 > i2 | text4 < 0) { MessageBox.Show("取出来的钱不能大于本金或为负数", "温馨提示"); } else { string NOW = ""; string NOW1 = ""; //MessageBox.Show(NOW); string count = "server=.;database=ox;user=sa;pwd=1;Integrated Security=True;"; string sql = "select * from DATA"; SqlConnection con = new SqlConnection(count); con.Open(); SqlCommand cmd = new SqlCommand(sql, con); SqlDataReader dr = cmd.ExecuteReader(); if (dr.HasRows) { dr.Read(); /*-----------先读取之前转账的信息------------*/ NOW = dr["RECORD"].ToString().Trim(); NOW1 = dr["RECORD"].ToString().Trim();
} dr.Close(); con.Close(); //----------储存转账信息------------ NOW += DateTime.Now.Year + "/" + DateTime.Now.Month + "/" + DateTime.Now.Day + "/" + DateTime.Now.Hour + "/" + DateTime.Now.Minute + "/" + DateTime.Now.Second; NOW += "对方卡号:" + textBox3.Text; NOW += "金额:" + text4 + "元 " + "已转入"; /*-----------先判断要存进多少钱,然后自己的账户减多少钱和对方账户加多少钱------等这些计算好了在存进数据中-------*/ string count01 = "SERVER=.;USER=SA;PWD=1;DATABASE=OX;Integrated Security=True;"; string sql01 = "select * from DATA where ID='" + textBox3.Text + "'"; SqlConnection cno01 = new SqlConnection(count01); cno01.Open(); SqlCommand cmd01 = new SqlCommand(sql01, cno01); SqlDataReader dr01 = cmd01.ExecuteReader(); dr01.Read(); int i3 = Convert.ToInt32(dr01["mory"].ToString().Trim());//获取对方账号的本金 dr01.Close(); cno01.Close();
int j = i2 - text4;//减去转账的金额等于剩下自己的本金 string count02 = "SERVER=.;USER=SA;PWD=1;DATABASE=OX;Integrated Security=True;"; string sql02 = "update DATA set mory='" + j + "' ,RECORD='" + NOW + "' where ID='" + textBox1.Text + "'"; //string sql = "select count(*) from yonghu where yname='" + textBox4.Text + "' and ypwd='" + textBox5.Text + "'"; //插入信息 SqlConnection cno02 = new SqlConnection(count02); cno02.Open(); SqlCommand cmd02 = new SqlCommand(sql02, cno02); int n = cmd02.ExecuteNonQuery(); dr01.Close();
NOW1 = DateTime.Now.Year + "/" + DateTime.Now.Month + "/" + DateTime.Now.Day + "/" + DateTime.Now.Hour + "/" + DateTime.Now.Minute + "/" + DateTime.Now.Second; NOW1 += "对方卡号:" + textBox3.Text; NOW1 += "金额:" + text4 + "元 " + "已转出"; int k = i3 + text4;//加上转账过来的金额等于自己的本金 string count03 = "SERVER=.;USER=SA;PWD=1;DATABASE=OX;"; string sql03 = "update DATA set mory='" + k + "', RECORD='" + NOW1 + "' where ID='" + textBox3.Text + "'"; SqlConnection cno03 = new SqlConnection(count03); cno03.Open(); SqlCommand cmd03 = new SqlCommand(sql03, cno03); if (cmd03.ExecuteNonQuery() > 0) { MessageBox.Show("转账成功"); } else { MessageBox.Show("Test"); } } } }
|
8, button5交易明细
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| private void button5_Click(object sender, EventArgs e) { textBox3.Visible = true; label3.Visible = true; textBox4.Visible = true; label4.Visible = true; textBox5.Visible = true; //MessageBox.Show(NOW); string count = "server=.;database=ox;user=sa;pwd=1;Integrated Security=True;"; string sql = "select * from DATA"; SqlConnection con = new SqlConnection(count); con.Open(); SqlCommand cmd = new SqlCommand(sql,con); SqlDataReader dr = cmd.ExecuteReader(); if (dr.HasRows) { dr.Read(); textBox5.Text = dr["RECORD"].ToString().Trim(); dr.Close(); } con.Close(); }
|
9, button6返回登录界面
1 2 3 4 5 6
| private void button6_Click(object sender, EventArgs e) { this.Close(); Form1 f1 = new Form1(); f1.Show(); }
|
csharp_ATM之登录篇
charp_ATM之找回密码篇