一【题目类别】
二【题目难度】
三【题目编号】
四【题目描述】
- 设计实现双端队列。
- 你的实现需要支持以下操作:
- MyCircularDeque(k):构造函数,双端队列的大小为k。
- insertFront():将一个元素添加到双端队列头部。 如果操作成功返回 true。
- insertLast():将一个元素添加到双端队列尾部。如果操作成功返回 true。
- deleteFront():从双端队列头部删除一个元素。 如果操作成功返回 true。
- deleteLast():从双端队列尾部删除一个元素。如果操作成功返回 true。
- getFront():从双端队列头部获得一个元素。如果双端队列为空,返回 -1。
- getRear():获得双端队列的最后一个元素。 如果双端队列为空,返回 -1。
- isEmpty():检查双端队列是否为空。
- isFull():检查双端队列是否满了。
五【题目示例】
- 示例:
MyCircularDeque circularDeque = new MycircularDeque(3); // 设置容量大小为3
circularDeque.insertLast(1); // 返回 true
circularDeque.insertLast(2); // 返回 true
circularDeque.insertFront(3); // 返回 true
circularDeque.insertFront(4); // 已经满了,返回 false
circularDeque.getRear(); // 返回 2
circularDeque.isFull(); // 返回 true
circularDeque.deleteLast(); // 返回 true
circularDeque.insertFront(4); // 返回 true
circularDeque.getFront(); // 返回 4
六【题目提示】
- 所有值的范围为 [1, 1000]
- 操作次数的范围为 [1, 1000]
- 请不要使用内置的双端队列库。
七【解题思路】
- 唯一需要注意一点就是头尾指针的位置问题,做减法时要注意不要越界,如果做减法为-1,取模还是-1,就越界了,解决办法就是加上队列的长度再取模,加法没有这个问题,其余和622.设计循环队列一样
八【时间频度】
九【代码实现】
- Java语言版
package Queue;
public class p641_DesignCircularDeque {
private static int[] queue;
private static int front;
private static int rear;
private static int len;
public static void main(String[] args) {
p641_DesignCircularDeque res1 = new p641_DesignCircularDeque(4);
boolean res2 = insertFront(9);
boolean res3 = deleteFront();
int res4 = getRear();
int res5 = getFront();
int res6 = getFront();
boolean res7 = deleteFront();
boolean res8 = insertFront(6);
boolean res9 = insertLast(5);
boolean res10 = insertFront(9);
int res11 = getFront();
boolean res12 = insertFront(6);
System.out.println(res1 + " " + res2 + " " + res3 + " " + res4 + " " + res5 + " " + res6 + " " + res7 + " " + res8 + " " + res9 + " " + res10 + " " + res11 + " " + res12);
}
public p641_DesignCircularDeque(int k) {
len = k + 1;
queue = new int[len];
front = 0;
rear = 0;
}
public static boolean insertFront(int value) {
if (isFull()) {
return false;
}
front = (front - 1 + len) % len;
queue[front] = value;
return true;
}
public static boolean insertLast(int value) {
if (isFull()) {
return false;
}
queue[rear] = value;
rear = (rear + 1) % len;
return true;
}
public static boolean deleteFront() {
if (isEmpty()) {
return false;
}
front = (front + 1) % len;
return true;
}
public static boolean deleteLast() {
if (isEmpty()) {
return false;
}
rear = (rear - 1 + len) % len;
return true;
}
public static int getFront() {
if (isEmpty()) {
return -1;
}
return queue[(front + len) % len];
}
public static int getRear() {
if (isEmpty()) {
return -1;
}
return queue[(rear - 1 + len) % len];
}
public static boolean isEmpty() {
return rear == front;
}
public static boolean isFull() {
return (rear + 1) % len == front;
}
}
- C语言版
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include<stdbool.h>
typedef struct
{
int *data;
int front;
int rear;
int len;
}MyCircularDeque;
MyCircularDeque* myCircularDequeCreate(int k)
{
MyCircularDeque* queue = (MyCircularDeque *)malloc(sizeof(MyCircularDeque));
queue->len = k + 1;
queue->data = (int *)malloc(queue->len * sizeof(int));
queue->front = 0;
queue->rear = 0;
return queue;
}
bool myCircularDequeIsEmpty(MyCircularDeque* obj)
{
return obj->rear == obj->front;
}
bool myCircularDequeIsFull(MyCircularDeque* obj)
{
return (obj->rear + 1) % obj->len == obj->front;
}
bool myCircularDequeInsertFront(MyCircularDeque* obj, int value)
{
if (myCircularDequeIsFull(obj))
{
return false;
}
obj->front = (obj->front - 1 + obj->len) % obj->len;
obj->data[obj->front] = value;
return true;
}
bool myCircularDequeInsertLast(MyCircularDeque* obj, int value)
{
if (myCircularDequeIsFull(obj))
{
return false;
}
obj->data[obj->rear] = value;
obj->rear = (obj->rear + 1) % obj->len;
return true;
}
bool myCircularDequeDeleteFront(MyCircularDeque* obj)
{
if (myCircularDequeIsEmpty(obj))
{
return false;
}
obj->front = (obj->front + 1) % obj->len;
return true;
}
bool myCircularDequeDeleteLast(MyCircularDeque* obj)
{
if (myCircularDequeIsEmpty(obj))
{
return false;
}
obj->rear = (obj->rear - 1 + obj->len) % obj->len;
return true;
}
int myCircularDequeGetFront(MyCircularDeque* obj)
{
if (myCircularDequeIsEmpty(obj))
{
return -1;
}
return obj->data[(obj->front + obj->len) % obj->len];
}
int myCircularDequeGetRear(MyCircularDeque* obj)
{
if (myCircularDequeIsEmpty(obj))
{
return -1;
}
return obj->data[(obj->rear - 1 + obj->len) % obj->len];
}
void myCircularDequeFree(MyCircularDeque* obj)
{
free(obj->data);
free(obj);
}
十【提交结果】
- Java语言版
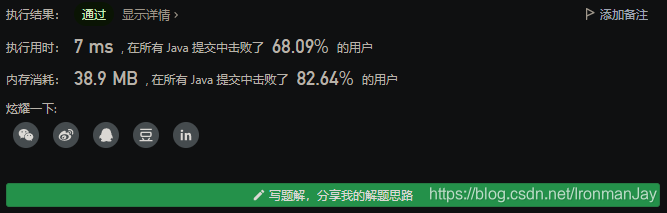
- C语言版
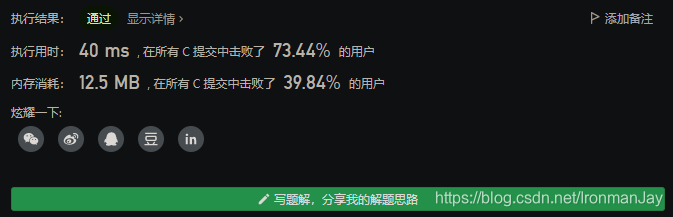