1.Collection 集合概述和使用
//创建集合对象
Collection<String> c = new ArrayList<String>();
2.Collection集合的常用方法
Alt + 7: 打开类的所有窗口,能够看到所有信息
Collection<String> c = new ArrayList<String>();
//boolean add(E e);添加元素
c.add("hello");
//boolean remove(Object o);从集合中移除指定元素
c.remove("hello");
//void clean(); 清空集合中的元素
c.clean();
//bolean contains(Object o); 判断集合中是否存在指定的元素
c.contains("world");
//int size(); 集合的长度,集合中元素个数
c.size();
//boolean isEmpty(); 判断集合是否为空
c.isEmpty();
(5)Coleantion集合的遍历
(6)集合的使用步骤
案例:Collection集合存储学生对象并遍历
package Collection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class CollectionDemo {
public static void main(String[] args) {
//创建集合对象
Collection<Student> c = new ArrayList<Student>();
//创建学生对象
Student s1 = new Student("彭于晏",18);
Student s2 = new Student("李易峰",16);
Student s3 = new Student("白敬亭",17);
//把学生对象添加到集合
c.add(s1);
c.add(s2);
c.add(s3);
//遍历集合(迭代器方法)
Iterator<Student> it = c.iterator();
while(it.hasNext()){
Student s = it.next();
System.out.println(s.getName() + ", " + s.getAge());
}
}
}
public class Student {
//成员变量
private String name;
private int age;
//构造方法
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
//成员方法:get/set方法
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
2.List(需要导包)
(1)概述和特点
(2)List集合的特有方法
List集合带索引,不能越界
/*
for循环遍历List集合
*/
//创建集合对象
List<String> list = new ArrayList<String>();
//添加元素
list.add("hello");
list.add("world");
list.add("java");
//for循环遍历List集合
for(int i = 0; i<list.length; i++){
String s = list.get(i)
System.out.println(s);
}
案例:List集合存储学生对象并遍历
package List;
//导包
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ListDemo {
public static void main(String[] args) {
//创建List集合对象
List<Student> list = new ArrayList<Student>(); //需要Array、List导包、List导包
//创建学生对象
Student s1 = new Student("彭于晏",18);
Student s2 = new Student("李易峰",16);
Student s3 = new Student("白敬亭",17);
//把学生添加到集合
list.add(s1);
list.add(s2);
list.add(s3);
//遍历集合(迭代器/for循环)
//for循环
for(int i=0; i<list.size(); i++){
Student s = list.get(i);
System.out.println(s.getName() + ", " + s.getAge());
}
System.out.println("---------------");
//迭代器循环Iterator
Iterator<Student> it = list.iterator(); //需要Iterator导包
while(it.hasNext()){
Student s = it.next();
System.out.println(s.getName() + ", " + s.getAge());
}
}
}
public class Student {
//定义成员变量
private String name;
private int age;
//构造方法
public Student() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Student(String name, int age) {
this.name = name;
this.age = age;
//成员方法:get/set
}
}
(3)并发修改异常 P229
(4)Listlterator 列表迭代器
(5)增强for循环(简化数组和Collection集合的遍历)
/*
增强for循环
*/
//数组
int[] arr = {1,2,3,4,5};
for(int i: arr){
System.out.println(i);
}
//数组
String[] strArray = {"hello","world","java"};
for(String s : strArray){
Systeem.out.println(s);
}
//集合
List<String> list = new ArrayList<String>();
list.add("hello");
list.add("world");
list.add("java");
for(String s : list){
System.out.println(s);
}
案例:List集合存储学生对象用3种方式遍历
package List2;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class ListDemo {
public static void main(String[] args) {
//创建List集合对象
List<Student> list = new ArrayList<Student>();
//创建学生对象
Student s1 = new Student("彭于晏",18);
Student s2 = new Student("李易峰",16);
Student s3 = new Student("白敬亭",17);
//把学生添加到集合
list.add(s1);
list.add(s2);
list.add(s3);
//遍历集合
//①Iterator迭代器
Iterator<Student> it = list.iterator();
while(it.hasNext()){
Student s = it.next();
System.out.println(s.getName() + ", " + s.getAge());
}
System.out.println("-------------------");
//②普通for循环;带有索引
for(int i=0; i<list.size(); i++){
Student s = list.get(i);
System.out.println(s.getName() + ", " + s.getAge());
}
System.out.println("-------------------");
//③增强for:最方便的
for(Student s : list){
System.out.println(s.getName() + ", " + s.getAge());
}
}
}
//定义学生类
public class Student {
//定义成员变量
private String name;
private int age;
//构造方法
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
//成员方法:get/set
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
(6)数据结构
计算机存储、组织数据的方式;相互之间存在一种或多种特定关系的数据元素的集合
(7)数据结构:栈
压栈/进栈:
压栈/弹栈:栈顶元素先出去
(8)数据结构:队列
(9)数据结构:数组
(10)数据结构:链表
(11)List集合子类特点
List集合子类特点:ArrayList、LinkedList
案例:ArrayList集合存储学生对象用3种方式遍历
package ArrayList;
import java.util.ArrayList;
import java.util.Iterator;
public class ArrayListDemo {
public static void main(String[] args) {
//创建ArrayList集合对象
ArrayList<Student> array = new ArrayList<Student>();
//创建学生对象
Student s1 = new Student("s1",12);
Student s2 = new Student("s2",13);
Student s3 = new Student("s3",14);
//把学生添加到集合
array.add(s1);
array.add(s2);
array.add(s3);
//集合遍历
//①迭代器:集合特有的遍历方式
Iterator<Student> it = array.iterator();
while(it.hasNext()){
Student s = it.next();
System.out.println(s.getName() + ", " + s.getAge());
}
System.out.println("-------------------------------");
//②普通for循环:带有索引的遍历方式
for(int i=0; i<array.size(); i++){
Student s = array.get(i);
System.out.println(s.getName() + ", " + s.getAge());
}
System.out.println("-------------------------------");
//增强for循环;最方便的遍历方式
for(Student s:array){
System.out.println(s.getName() + ", " + s.getAge());
}
}
}
//定义学生类
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
(12)LinkedList集合的特有功能
3 .Set集合
(1)概述和特点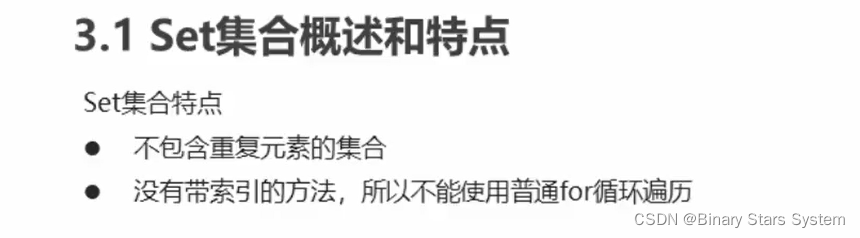
HashSet对集合的迭代顺序不做任何保证
(2)哈希值
(3)HashSet集合概述和特点
(4)HashSet集合保证元素唯一性源码分析
(5)常见数据结构之哈希表
先比较哈希值,哈希值相同再比较内容,如果内容也相同证明重复,不存入
(6)LinkedHashSet集合概述和特点
(7)TreeSet集合概述和特点
//创建集合对象
TreeSet<Integer> ts = new TreeSet<Integer>();
//所有基本类型存储时,用的都是对应的包装类型,如;int ---Integer
(8)自然排序Comparable的使用 P246(再补充)
让类实现该接口,类可以让这个类的对象进行自然排序
package TreeSet;
public class Student implements Comparable<Student>{
//成员变量
private String name;
private int age;
//构造方法
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
//成员方法:get/set方法
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public int compareTo(Student s){
//return 0; //认为s1,s2,s3,s4是重复元素,不会存储
//return 1; //按照升序来存储
//return -1; //按照降序来存储
//按照年龄从小到大排序(this 放在前面)
int num = this.age - s.age;
//年龄按照降序排列(this 放在后面)
//int num = s.age - this.age;
// return num;
//年龄相同时,按照姓名的字母顺序排序
int num2 = num==0?this.name.compareTo(s.name):num;
return num2;
}
}
import java.util.TreeSet;
/*
存储学生对象并遍历,创建集合使用无参构造方法
要求:按照年龄从小到大排序,年龄相同时,按照姓名的字母顺序排列
*/
public class TreeSetDemo02 {
public static void main(String[] args) {
//创建集合对象
TreeSet<Student> ts = new TreeSet<Student>();
//创建学生对象
Student s1 = new Student("d1",11);
Student s2 = new Student("c2",12);
Student s3 = new Student("b3",13);
Student s4 = new Student("a4",14);
//把学生添加到集合
ts.add(s1);
ts.add(s2);
ts.add(s3);
ts.add(s4);
//遍历集合
for(Student s : ts){
System.out.println(s.getName() + ", " + s.getAge());
}
}
}
(9)比较器排序Comparator的使用
3.Set
案例:成绩排序
package TreeSet2;
/*
用TreeSet集合存储多个学生信息(姓名,语文成绩,数学成绩),并遍历该集合
要求:按照总分从高到低出现
思路:1.定义学生类
2.创建TreeSet集合对象,通过比较器进行排序
3.创建学生对象
4.把学生对象添加到集合
5.遍历集合
*/
import java.util.Comparator;
import java.util.TreeSet;
public class TreeSetDemo {
public static void main(String[] args) {
//创建TreeSet集合对象,通过比较器进行排序
TreeSet<Student> ts = new TreeSet<Student>(new Comparator<Student>(){ //接收比较器接口对象,用匿名内部类实现
@Override
public int compare(Student s1,Student s2){
//int num = (s1.getChinese() + s1.getMath()) - (s2.getChinese() + s2.getMath());
//主要条件
int num = s2.getSum() - s1.getSum(); //比较总分:从高到低(从高到低:s2 - s1; 从低到高:s1 - s2)
//次要条件:2个(比较语文成绩,姓名)
int num2 = num==0 ? s1.getChinese() - s2.getChinese() : num; //总分相同的情况下,比较语文成绩
int num3 = num2==0 ? s1.getName().compareTo(s2.getName()) : num2; //语文成绩相同的情况下,比较姓名(用compareTo方法)
return num3;
}
});
//创建学生对象
Student s1 = new Student("彭于晏",98,100);
Student s2 = new Student("李易峰",95,95);
Student s3 = new Student("白敬亭",100,93);
Student s4 = new Student("潘丢丢",100,97);
Student s5 = new Student("不知道",98,98);
Student s6 = new Student("刘以豪",97,99);
Student s7 = new Student("褚克桓",97,99);
//把学生对象添加到集合
ts.add(s1);
ts.add(s2);
ts.add(s3);
ts.add(s4);
ts.add(s5);
ts.add(s6);
ts.add(s7);
//遍历集合:增强for
for(Student s :ts){
System.out.println(s.getName() + ", " + s.getChinese() + " ," + s.getMath() + ", " + s.getSum());
}
}
}
//定义学生类
public class Student {
//定义成员变量
private String name;
private int chinese;
private int math;
//构造方法
public Student() {
}
public Student(String name, int chinese, int math) {
this.name = name;
this.chinese = chinese;
this.math = math;
}
//成员方法:get/set方法
public int getChinese() {
return chinese;
}
public void setChinese(int chinese) {
this.chinese = chinese;
}
public int getMath() {
return math;
}
public void setMath(int math) {
this.math = math;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
//
public int getSum(){
int num = getChinese() + getMath();
return num;
}
}
案例:不重复的随机数
import java.util.*;
public class SetDemo {
public static void main(String[] args) {
//创建Set集合
Set<Integer> set = new HashSet<Integer>(); //无序排列
//set<Integer> set = new TreeSet<Integer>(); //有序排列
//创建随机数对象
Random r = new Random();
//判断集合的长度是不是小于10
while(set.size()<10){
int number = r.nextInt(20) + 1; //生成随机数:1~20
set.add(number); //保证没有添加重复元素
}
//遍历集合
for(Integer i : set){
System.out.println(i);
}
}
}
4.泛型
(1)概述
(2)泛型类
//泛型类
public class Deneric<T>{
/*
public void show(T t){
//方法体
}
*/
//生成get/set方法
}
(3)泛型方法
public class Generic{
public <T> void show(T t){ //泛型方法
//方法体
}
}
(4)泛型接口
//泛型接口
//格式:修饰符 interface 接口名<类型>{ }
public interface Generic<T>{ }
(5)类型通配符
//类型通配符上限: <? extends 类型 Number> 表示本类或者其子类型
List<? extends Number> list1 = new ArrayLIst<Integer>;
//类型通配符下限: <? super 类型 Number> 表示本类或者其父类型
List<? extends Number> list1 = new ArrayLIst<Object>;
(6)可变参数
包含多个参数,可变参数放在后面
(7)可变参数的使用
/*
public static <E> Set<E> of (E... elements):返回一个包含任意数量元素的不可变集合
(不能出现重复元素)
*/
/*
public static <E> List<E> of (E... elements):返回包含任意数量元素的不可变集合
(不能增、删、修改元素)
*/
/*
public static <T> List<T> asList (T... a):返回由指定数组支持的固定大小的列表
(不能增、删元素,可以修改)
*/
5.Map
(1)Map集合的概述和使用
HashMap保证了键的唯一性
(2)Map集合的基本功能
//创建集合对象
Map<String,String> map = new HashMap<String,String>();
(3) Map集合的获取功能
(4)Map集合的遍历(方式1)
(5)Map集合的遍历(方式2)
案例:HashMap 集合存储学生对象并遍历
package HashMap;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class HashMapDemo {
public static void main(String[] args) {
//创建HashMap集合对象
HashMap<String, Student> hm = new HashMap<String, Student>();
//创建学生对象
Student s1 = new Student("彭于晏", 18);
Student s2 = new Student("李易峰", 16);
Student s3 = new Student("褚克桓", 17);
//把学生添加到集合
hm.put("001", s1);
hm.put("002", s2);
hm.put("003", s3);
//方式1:
Set<String> keySet = hm.keySet();
for (String key : keySet) {
Student value = hm.get(key);
System.out.println(key + ", " + value.getName() + ", " + value.getAge());
}
System.out.println("---------------------");
//方式2:
Set<Map.Entry<String, Student>> entrySet = hm.entrySet();
for (Map.Entry<String, Student> me : entrySet) {
String key = me.getKey();
Student value = me.getValue();
System.out.println(key + ", " + value.getName() + ", " + value.getAge());
}
}
}
//定义学生类
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
案例:HashMap集合存储学生对象并遍历
案例:ArrayList集合存储HashMap元素并遍历
package HashMap;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Set;
public class HashMapDemo3 {
public static void main(String[] args) {
//创建ArrayList集合
ArrayList<HashMap<String,String>> array = new ArrayList<HashMap<String, String>>();
//创建HashMap集合,并添加键值对元素
HashMap<String,String> hm1 = new HashMap<String,String>();
hm1.put("孙策","大乔");
hm1.put("周瑜","小乔");
//把HashMap作为元素添加到ArrayList集合
array.add(hm1);
HashMap<String,String> hm2 = new HashMap<String,String>();
hm2.put("郭靖","黄蓉");
hm2.put("杨过","小龙女");
//把HashMap作为元素添加到ArrayList集合
array.add(hm2);
HashMap<String,String> hm3 = new HashMap<String,String>();
hm3.put("令狐冲","任盈盈");
hm3.put("林平之","岳灵珊");
//把HashMap作为元素添加到ArrayList集合
array.add(hm3);
//遍历ArrayList集合
for(HashMap<String,String> hm : array){ //每个hm就是ArrayList中的每个元素
Set<String> keySet = hm.keySet();
for(String key : keySet){
String value = hm.get(key);
System.out.println(key + ", " + value);
}
}
}
}
案例:Hashmap集合存储ArrayList元素并遍历
package HashMap;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Set;
public class HashMapDemo4 {
public static void main(String[] args) {
//创建HashMap集合存储ArrayList对象
HashMap<String,ArrayList<String>> hm = new HashMap<String,ArrayList<String>>();
//创建ArrayList集合,并添加元素
//三国演义
ArrayList<String> sgyy = new ArrayList<String>();
sgyy.add("诸葛亮");
sgyy.add("赵云");
//把arrayList作为元素添加到HashMap集合
hm.put("三国演义",sgyy);
//西游记
ArrayList<String> xyj = new ArrayList<String>();
xyj.add("唐僧");
xyj.add("孙悟空");
//把arrayList作为元素添加到HashMap集合
hm.put("西游记",xyj);
//水浒传
ArrayList<String> shz = new ArrayList<String>();
shz.add("武松");
shz.add("鲁智深");
//把arrayList作为元素添加到HashMap集合
hm.put("水浒传",shz);
//遍历HashMap集合
Set<String> keySet = hm.keySet();
for(String key : keySet){
System.out.println(key);
ArrayList<String> value = hm.get(key);
for(String s : value){
System.out.println("\t" + s);
}
}
}
}
案例:统计字符串中每个字符出现的次数
package HashMap;
import java.util.HashMap;
import java.util.Scanner;
import java.util.Set;
import java.util.TreeMap;
/*
思路:
1.键盘录入一个字符串
2.创建HashMap集合,键是Character ,值是Integer
3.遍历字符串,得到每一个字符
4.拿每一个得到的字符作为键到HashMap集合中去找对应的值,看其返回值
如果返回值是null,说明该字符在HashMap集合中不存在,就把该字符作为键,1作为值存储
如果返回值不是null,说明该字符在HashMap集合中存在,把该值加1,然后重新存储该字符和对应的值
5.遍历HashMap集合,得到键和值,按照要求进行拼接
6.输出结果
*/
public class HashMapDemo5 {
public static void main(String[] args) {
/*
键盘录入一个字符串
*/
//创建Scanner 对象
Scanner input = new Scanner(System.in);
System.out.println("请输入一个字符串:");
String line = input.nextLine();
//创建HashMap集合,键是Character,值是Integer
//HashMap<Character,Integer> hm = new HashMap<Character,Integer>(); //HashMap 无序
TreeMap<Character,Integer> hm = new TreeMap<Character,Integer>(); //TreeSet 有序
//遍历字符串,得到每一个字符
for(int i=0; i<line.length(); i++){
char key = line.charAt(i);
//4.拿每一个得到的字符作为键到HashMap集合中去找对应的值,看其返回值
Integer value = hm.get(key);
// 如果返回值是null,说明该字符在HashMap集合中不存在,就把该字符作为键,1作为值存储
if(value == null){
hm.put(key,1);
}
// 如果返回值不是null,说明该字符在HashMap集合中存在,把该值加1,然后重新存储该字符和对应的值
else{
value++;
hm.put(key,value);
}
}
//5.遍历HashMap集合,得到键和值,按照要求进行拼接
StringBuilder sb = new StringBuilder();
Set<Character> keySet = hm.keySet();
for(Character key : keySet){
Integer value = hm.get(key);
sb.append(key).append("(").append(value).append(")");
}
String result = sb.toString();
//输出结果
System.out.println(result);
}
}
6. Collections
(1)概述和使用
//创建集合对象
List<Integer> list = new ArrayList<Integer>();
//添加元素
list.add(30);
list.add(20);
list.add(50);
list.add(10);
list.add(40);
//升序
Collections.sort(list);
//反转
Collections.reserve(list);
//随机排序
Collections.shuffle(list);
ArrayList存储学生对象并排序
package Collections;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import static java.util.Collections.sort;
public class CollectionsDemo {
public static void main(String[] args) {
//创建ArrayList集合对象
ArrayList<Student> array = new ArrayList<Student>();
//创建学生对象
Student s1 = new Student("pengyuyan",30);
Student s2 = new Student("chukehuan",18);
Student s3 = new Student("liyifeng",19);
Student s4 = new Student("liuyihao",17);
//把学生对象添加到集合
array.add(s1);
array.add(s2);
array.add(s3);
array.add(s4);
//使用Collections对ArrayList集合排序
Collections.sort(array,new Comparator<Student>() {
@Override
public int compare(Student s1,Student s2){
//按照年龄从小到大排序,年龄相同时,按照姓名的字母顺序排序
int num = s1.getAge() - s2.getAge();
int num2 = num == 0 ? s1.getName().compareTo(s2.getName()) : num;
return num2;
}
});
//遍历集合
for(Student s : array){
System.out.println(s.getName() + ", " + s.getAge());
}
}
}
案例:模拟斗地主
package Collections;
import java.util.ArrayList;
import java.util.Collections;
import static java.util.Collections.shuffle;
/*
1.创建一个牌盒,也就是定义一个集合对象,用ArrayList集合实现
2.往牌盒里装牌
3.洗牌,也就是把牌打散,用Collections的shuffle()方法实现
4.发牌,也就是遍历集合,给三个玩家发牌
5。看牌,也就是三个玩家分别遍历自己的牌
*/
public class PokerDemo {
public static void main(String[] args) {
//1.创建一个牌盒,也就是定义一个集合对象,用ArrayList集合实现
ArrayList<String> array = new ArrayList<String>();
//往牌盒里面装牌
/*
♦2,♦3,♦4,♦5,.....,♦K,A
♣2,♣3,♣4,♣5,.....,♣K,A
❤,.....
♠,.....
大王,小王
*/
//定义花色数组 colors
String[] colors = {"♦","♣","❤","♠"};
//定义点数数组 numbers
String[] numbers = {"1","2","3","4","5","6","7","8","9","10","J","Q","K","A"};
//花色和点数拼接(数组array)
for(String color : colors){
for(String number : numbers){
array.add(color+number);
}
}
array.add("大王");
array.add("小王");
//3.洗牌,也就是把牌打散,用Collections的shuffle()方法实现
Collections.shuffle(array);
//4.发牌,也就是遍历集合,给三个玩家发牌(3个集合)
ArrayList array1 = new ArrayList();
ArrayList array2 = new ArrayList();
ArrayList array3 = new ArrayList();
ArrayList array4 = new ArrayList();
for(int i=0; i< array.size(); i++){
//得到牌
String poker = array.get(i);
if(i >= array.size() - 3){
array4.add(poker);
}else if(i % 3 == 0){
array1.add(poker);
}else if(i % 3 == 1){
array2.add(poker);
}
else if(i % 3 == 2){
array3.add(poker);
}
}
//看牌(调用方法)
lookPoker("array1",array1);
lookPoker("array2",array2);
lookPoker("array3",array3);
lookPoker("array4",array4);
System.out.println(array);
}
//看牌的方法
public static void lookPoker(String name,ArrayList<String> array){
System.out.print(name + "的牌是:");
for(String poker : array){
System.out.print(poker + " ");
}
System.out.println(); //显示完每个人的牌之后换行
}
}
案例:模拟斗地主升级版
package Collections;
import java.util.*;
import static java.util.Collections.shuffle;
/*
需求:通过程序实现斗地主过程中的洗牌、发牌、看牌。
要求:对牌进行排序(整副牌处理为编号)
思路:
1.创建HashMap,键是编号,值是牌
2.创建ArrayList,存储编号
3.创建花色数组和点数数组
4.从0开始往HashMap里面存储编号,并存储对应的牌,同时往ArrayList里面存储编号
5.洗牌(洗的是编号),用Collection的Shuffle()方法实现
6.发牌(发的是编号,为保证编号是排序的,创建TreeSet集合接收)
7.定义方法看牌(遍历TreeSet集合,获取编号,到HashSet集合找对应的牌)
8.调用看牌方法
*/
public class PokerDemo2<shuffle> {
public static void main(String[] args) {
//1.创建HashMap,键是编号Integer,值是牌String
HashMap<Integer, String> hm = new HashMap<Integer, String>();
//2.创建ArrayList,存储编号
ArrayList<Integer> array = new ArrayList<Integer>();
//3.创建花色数组和点数数组
/*
♦3,♦4,♦5,.....,♦K,A,♦2
♣2,♣3,♣4,♣5,.....,♣K,A,♣2
❤,.....
♠,.....
大王,小王
*/
//创建花色数组colors
String[] colors = {"♦", "♣", "❤", "♠"};
//创建点数数组numbers
String[] numbers = {"3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A", "2"};
//4.从0开始往HashMap里面存储编号,并存储对应的牌,同时往ArrayList里面存储编号
//拼接花色和点数数组
int index = 0;
for (String color : colors) {
for (String number : numbers) {
hm.put(index, color + number);
array.add(index);
index++;
}
}
hm.put(index, "大王");
array.add(index);
index++;
hm.put(index, "小王");
array.add(index);
//5.洗牌(洗的是编号),用Collection的Shuffle()方法实现
Collections.shuffle(array);
//6.发牌(发的是编号,为保证编号是排序的,创建TreeSet集合接收)
TreeSet<Integer> pyySet = new TreeSet<Integer>(); //彭于晏
TreeSet<Integer> ckhSet = new TreeSet<Integer>(); //褚克桓
TreeSet<Integer> lyfSet = new TreeSet<Integer>(); //李易峰
TreeSet<Integer> lyhSet = new TreeSet<Integer>(); //刘以豪(底牌)
for (int i = 0; i < array.size(); i++) {
int x = array.get(i);
if (i >= array.size() - 3) {
lyhSet.add(x);
} else if (i % 3 == 0) {
pyySet.add(x);
} else if (i % 3 == 1) {
ckhSet.add(x);
} else if (i % 3 == 2) {
lyfSet.add(x);
}
}
//8.调用看牌方法
lookPoker("彭于晏",pyySet,hm);
lookPoker("褚克桓",ckhSet,hm);
lookPoker("李易峰",lyfSet,hm);
lookPoker("刘以豪",lyhSet,hm);
}
// 7.定义方法看牌(遍历TreeSet集合,获取编号,到HashSet集合找对应的牌)
public static void lookPoker(String name, TreeSet<Integer> ts, HashMap<Integer, String> hm){
System.out.print(name + "的牌是:");
for(Integer key : ts){
String poker = hm.get(key);
System.out.print(poker+ " ");
}
System.out.println();
}
}