2.3Python语法基础
**
- 使用缩进来组织代码结构
**
for循环举例
for x in array:
if x < pivot:
less.append(x)
else:
greater.append(x
冒号标志着缩进代码块的开始,冒号之后的所有代码的缩进量必须相同,直到代码
块结束
分号可以用来给同在一行的语句切分:
a = 5; b = 6; c = 7
(Python不建议将多条语句放到一行,这会降低代码的可读性。)
-
万物皆对象
每个数字、字符串、数据结构、函数、类、模块等等,都是在Python解释器的自有“盒子”内,它被认为是Python对象。 -
注释
任何前面带有井号#的文本都会被Python解释器忽略。这通常被用来添加注释。
results = []
for line in file_handle:
# keep the empty lines for now
# if len(line) == 0:
# continue
results.append(line.replace('foo', 'bar'))
也可以在执行过的代码后面添加注释。
print("Reached this line") # Simple status report
- 函数和对象方法调用
你可以用圆括号调用函数,传递零个或几个参数,或者将返回值给一个变量
result = f(x, y, z)
f()
调用访问对象的内容的方法
obj.some_method(x, y, z)
函数可以使用位置和关键词参数的方式:
result = f(a, b, c, d=5, e='foo')
- 变量和参数传递
在等号右边创建了一个对这个变量的引用。
a = [1, 2, 3]
假设将a赋值给一个新变量b:
b = a
在Python中,a和b实际上是同一个对象,即原有列表[1, 2, 3](见下图)。你可以在a中添加一个元素,然后检查b:
a.append(4)
b
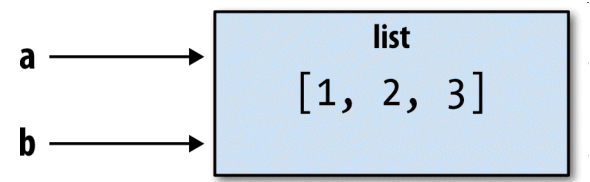
- 属性和方法
Python的对象通常都有属性(其它存储在对象内部的Python对象)和方法(对象的附属函数可以访问对象的内部数据)。可以用obj.attribute_name访问属性和方法:
In [1]: a = 'foo'
In [2]: a.<Press Tab>
a.capitalize a.format a.isupper a.rindex a.strip
a.center a.index a.join a.rjust a.swapcase
a.count a.isalnum a.ljust a.rpartition a.title
a.decode a.isalpha a.lower a.rsplit a.translate
a.encode a.isdigit a.lstrip a.rstrip a.upper
a.endswith a.islower a.partition a.split a.zfill
a.expandtabs a.isspace a.replace a.splitlines
a.find a.istitle a.rfind a.startswith
- 鸭子类型
经常地,你可能不关心对象的类型,只关心对象是否有某些方法或用途。这通常被称为“鸭子类型”,来自“走起来像鸭子、叫起来像鸭子,那么它就是鸭子”的说法。
7.二元运算符和比较运算符
In [32]: 5 - 7
Out[32]: -2
In [33]: 12 + 21.5
Out[33]: 33.5
In [34]: 5 <= 2
Out[34]: False
8.可变与不可变对象
Python中的大多数对象,比如列表、字典、NumPy数组,和用户定义的类型(类),都是可变的。意味着这些对象或包含的值可以被修改
In [43]: a_list = ['foo', 2, [4, 5]]
In [44]: a_list[2] = (3, 4)
In [45]: a_list
Out[45]: ['foo', 2, (3, 4)]
例如字符串和元组,是不可变的
数值类型
Python的主要数值类型是int和float。int可以存储任意大的数,float是浮點數
字符串
你可以用单引号或双引号来写字符串:
a = 'one way of writing a string'
b = "another way"
将两个字符串合并,会产生一个新的字符串:
In [71]: a = 'this is the first half '
In [72]: b = 'and this is the second half'
In [73]: a + b
Out[73]: 'this is the first half and this is the second half'
字符串的模板化或格式化,是另一个重要的主题。Python 3拓展了此类的方法,
这里只介绍一些。字符串对象有format方法,可以替换格式化的参数为字符串,
产生一个新的字符串:
template = '{0:.2f} {1:s} are worth US${2:d}'
在这个字符串中,
{0:.2f}表示格式化第一个参数为带有两位小数的浮点数。
{1:s}表示格式化第二个参数为字符串。
{2:d}表示格式化第三个参数为一个整数。
In [75]: template.format(4.5560, 'Argentine Pesos', 1)
Out[75]: '4.56 Argentine Pesos are worth US$1'
布尔值
Python中的布尔值有两个,True和False。比较和其它条件表达式可以用True和False判断。布尔值可以与and和or结合使用:
In [89]: True and True
Out[89]: True
In [90]: False or True
Out[90]: True
类型转换
In [91]: s = '3.14159'
In [92]: fval = float(s)
In [93]: type(fval)
Out[93]: float
In [94]: int(fval)
Out[94]: 3
In [95]: bool(fval)
Out[95]: True
In [96]: bool(0)
Out[96]: False
None
None是Python的空值类型。如果一个函数没有明确的返回值,就会默认返回None
日期和时间
Python内建的datetime模块提供了datetime、date和time类型。datetime类型结合了date和time,是最常使用的:
In [102]: from datetime import datetime, date, time
In [103]: dt = datetime(2011, 10, 29, 20, 30, 21)
In [104]: dt.day
Out[104]: 29
In [105]: dt.minute
Out[105]: 30
控制流
if、elif和else
if后面可以跟一个或多个elif,所有条件都是False时,还可以添加一个else:
if x < 0:
print('It's negative')
elif x == 0:
print('Equal to zero')
elif 0 < x < 5:
print('Positive but smaller than 5')
else:
print('Positive and larger than or equal to 5')
如果某个条件为True,后面的elif就不会被执行。当使用and和or时,复合条件语句是从左到右执行:
In [117]: a = 5; b = 7
In [118]: c = 8; d = 4
In [119]: if a < b or c > d:
.....: print('Made it')
Made it
for循环
for循环是在一个集合(列表或元组)中进行迭代
for value in collection:
# do something with value
While循环
while循环指定了条件和代码,当条件为False或用break退出循环,代码才会退出:
x = 256
total = 0
while x > 0:
if total > 500:
break
total += x
x = x // 2
pass
pass是Python中的非操作语句。代码块不需要任何动作时可以使用
range
range函数返回一个迭代器,它产生一个均匀分布的整数序列
range的三个参数是(起点,终点,步进)不包括終點:
In [124]: list(range(0, 20, 2))
Out[124]: [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
In [125]: list(range(5, 0, -1))
Out[125]: [5, 4, 3, 2, 1]
三元表达式
Python中的三元表达式可以将if-else语句放到一行里。语法如下:
value = true-expr if condition else false-expr
效果和下列代碼相同
if condition:
value = true-expr
else:
value = false-exp