一、问题描述
- 公司今天招聘了10名员工(A B C D E F G H I J),10名员工进入公司之后,需要指派员工在哪个部门工作
- 员工信息:姓名、工资
- 公司部门:策划、美术、研发
- 随机给10名员工分配部门和工资、工资范围在10k-15k之间
- 通过multimap进行信息的插入<key(部门编号),value(员工)>
- 分部门显示员工信息
二、代码实现
#include <iostream>
#include <multimap>
#include <string>
#include <cstdlib>//随机数
using namespace std;
//员工类
class Staff {
private :
string name;
unsigned int wages;
public :
Staff(string n, int w) : name(n), wages(w) {}
void setName(string n) { this->name = n; }
string getName() { return this->name; }
void setWages(int w) { this->wages = w; }
int getWages() { return this->wages; }
};
//重载<<运算符
ostream& operator<<(ostream& cout, Staff staff) {
cout << staff.getName() << "的工资是" << staff.getWages() << "元";
return cout;
}
//主函数
int main() {
multimap<string, Staff> info;
const string staffNames[10] = { "A", "B", "C", "D", "E", "F", "G", "H", "I", "J"};
const string departments[3] = { "策划", "美术", "研发"};
//设置随机种子,种子根据程序执行时间确定,这样每次执行都可以获取不同的随机数
srand(time(0));
//为员工随机分配工资和部门
for (int i = 0; i < 10; i++) {
//员工工资范围10k-15k
int wages = 10000 + (rand() % 5000);
//根据名字创建员工对象
Staff staff(staffNames[i], wages);
//随机给员工分配一个部门
string dep = departments[rand() % 3];
//将员工和部门插入info中
info.insert(pair<string, Staff>(dep, staff));
}
//使用equal_range()分部门显示员工信息
pair<multimap<string, Staff>::iterator, multimap<string, Staff>::iterator> dt;
cout << "------------------------------------------" << endl;
for (int i = 0; i < 3; i++) {
dt = info.equal_range(departments[i]);
//遍历输出当前部门的所有员工
for (multimap<string, Staff>::iterator it = dt.first; it != dt.second; it++) {
cout << it->first << "部门:" << it->second << endl;
}
cout << "------------------------------------------" << endl;
}
return 0;
}
三、程序执行结果
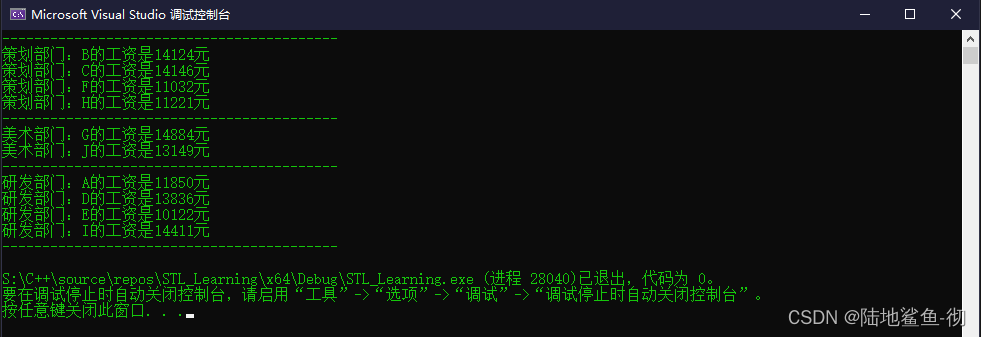