1.常量配置与常用的常量简介
常量配置
Struts2常量,也叫Struts2属性——控制整个Struts2的应用特性。
一个常量名指定一个常量值。
配置方法有3种:
1、在web.xml文件中。
<!-- 通过Filter配置初始化参数来配置Struts2常量 -->
<init-param>
<param-name>常量名字</param-name>
<param-value>常量值</param-value>
</init-param>
2、在struts.xml文件配置。
<constant name="常量名" value="常量值"/>
3、增加一个struts.properties配置文件。
常量名 = 常量值
常用的Struts2常量:
struts.i18n.encoding — 指定Web应用的默认编码集。默认为UTF-8(相当于HttpServletRequest的setCharacterEncoding(“utf-8”))。
struts.multipart.saveDir — 设置文件上传的临时目录。
struts.multipart.maxSize — 设置每次请求上传文件的最大字节数。
struts.action.extension — 设置Action的默认后缀。默认是action。
struts.serve.static.browserCache — 设置浏览器是否缓存静态内容。
struts.enable.DynamicMethodInvocation — 设置是否支持动态方法调用。默认是false。
struts.devMode — 设置是否处于开发模式。默认值为false,设为true时相当于把struts.i18n.reload、struts.configuration.xml.reload都设为true。
struts.i18n.reload — 设置是否为每次请求都重新加载资源文件。默认值为false。
struts.configuration.xml.reload — 设置是否当struts.xml改变后,系统自动重新加载该文件。默认值为false。
struts.custom.i18n.resources — 是否加载国际化资源文件。
常量配置之方法一:web.xml
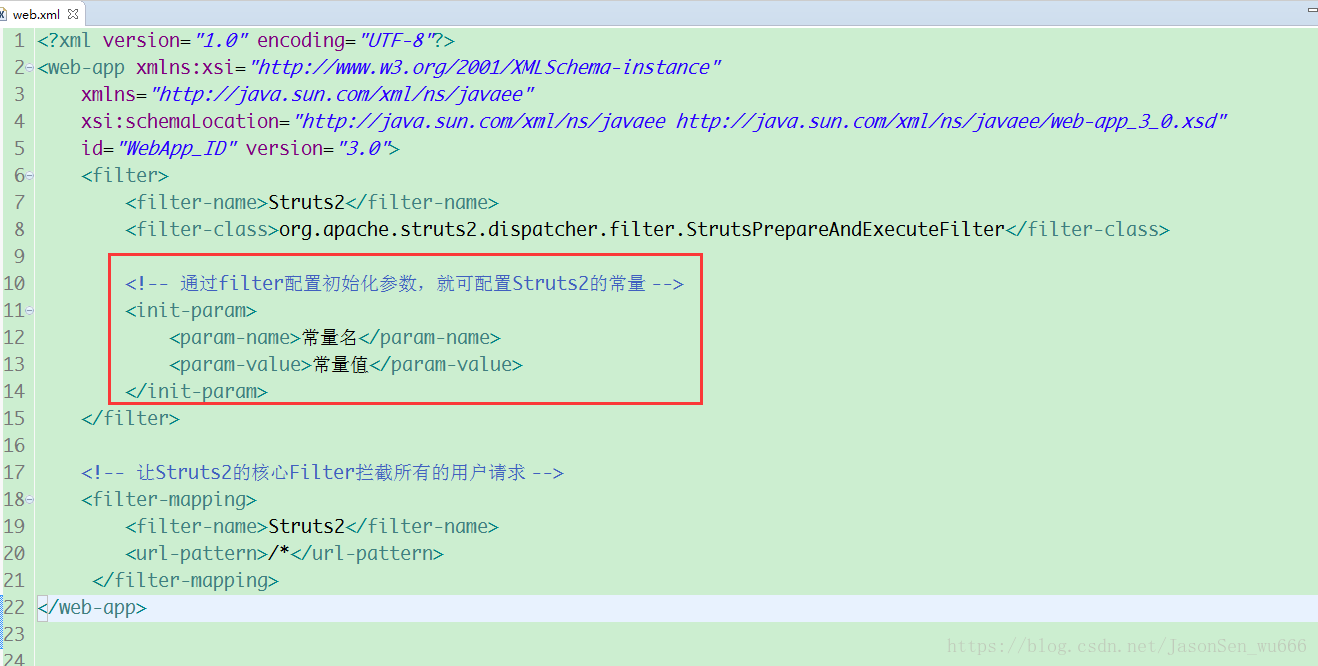
常量配置之方法二:struts.xml
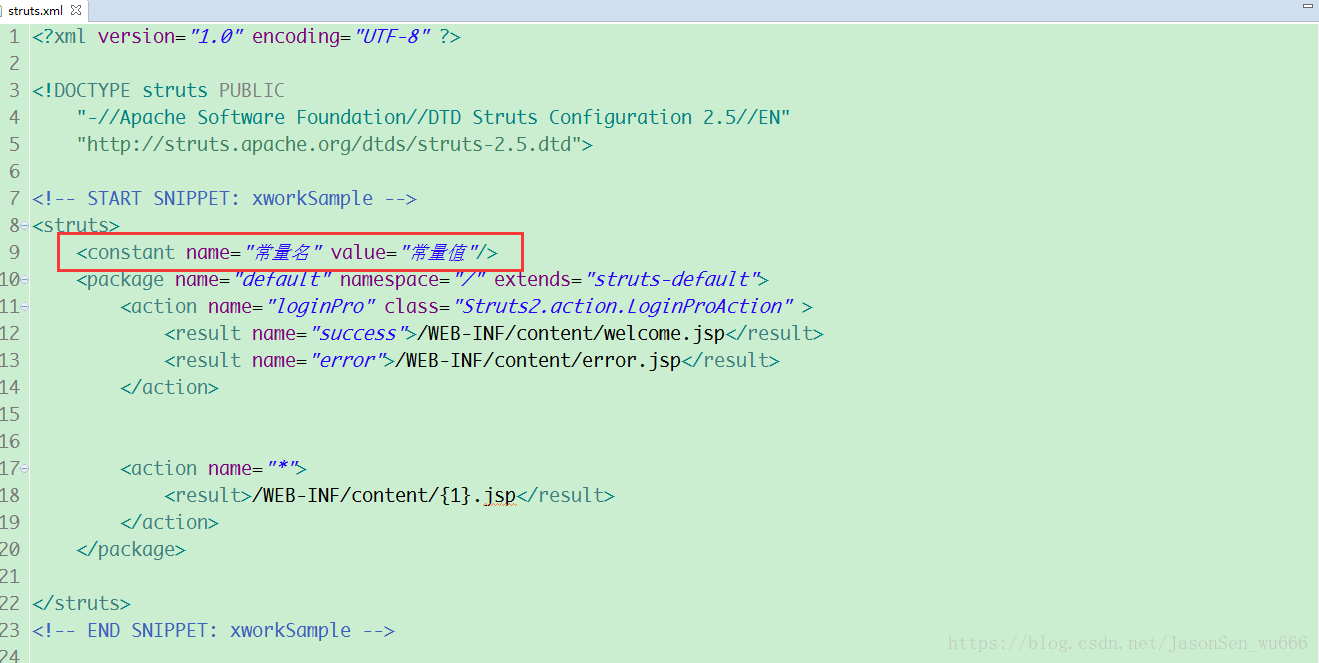
常量配置之方法三:struts.properties
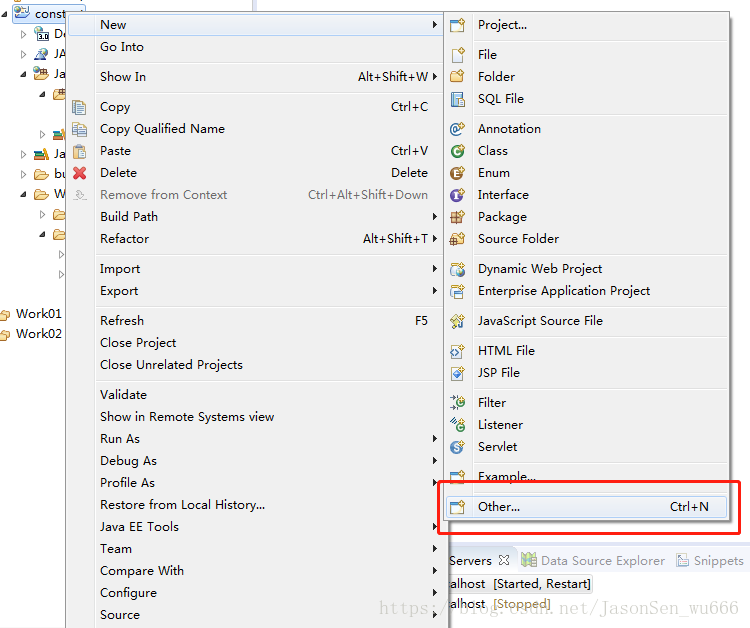
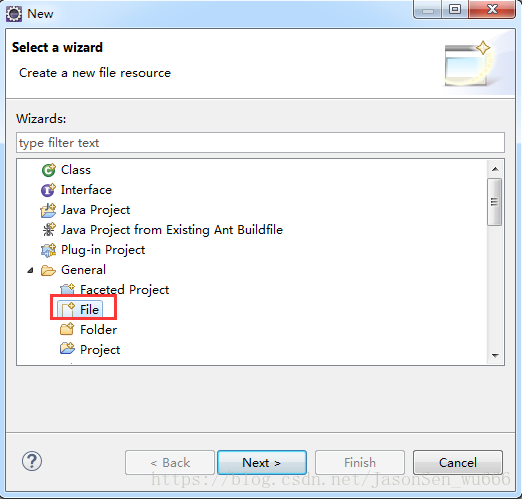
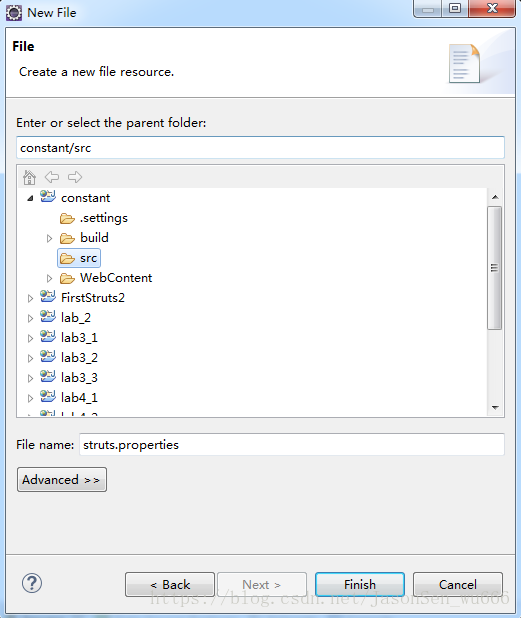
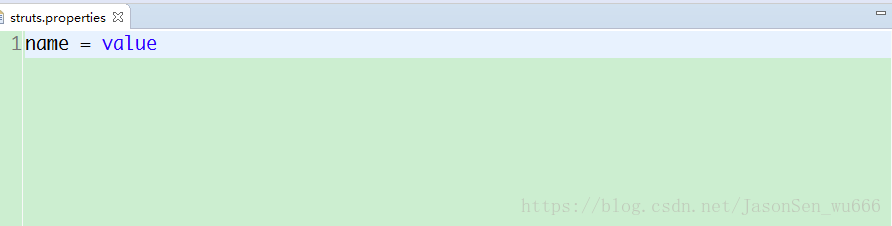
/constant/src/struts.properties
程序代码如下:
struts.devMode=true
#request.srtCharacterEncoding("utf-8")
struts.i18n.encoding=utf-8
/constant/WebContent/WEB-INF/content/LoginForm.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>Insert title here</title>
</head>
<body>
<h1>用户登录</h1>
<form action="loginPro" method="post">
用户名:<input type="text" name="name" /></br>
密码:<input type="text" name="pass" /></br>
<input type="submit" value="登录" /></br>
</form>
</body>
</html>
/constant/WebContent/WEB-INF/content/welcome.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>欢迎</title>
</head>
<body>
登录成功<br>
</body>
</html>
运行项目(保持web服务器开启)
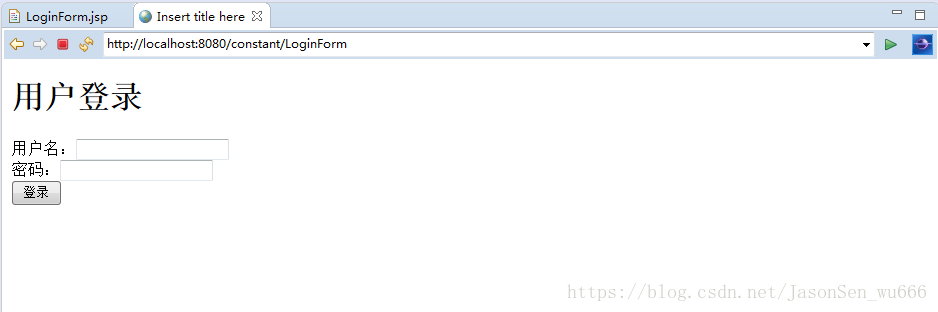
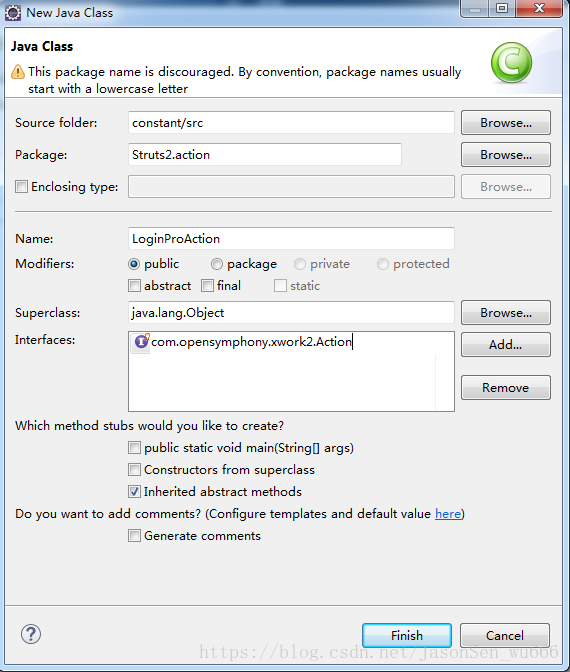
/constant/src/Struts2/action/LoginProAction.java
程序代码如下:
package Struts2.action;
import com.opensymphony.xwork2.Action;
public class LoginProAction implements Action {
@Override
public String execute() throws Exception {
//TODO Auto-generated method stub
return null;
}
}
/constant/src/Struts2/action/LoginProAction.java
程序代码如下:
package Struts2.action;
import com.opensymphony.xwork2.Action;
public class LoginProAction implements Action {
private String name;
private String pass;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPass() {
return pass;
}
public void setPass(String pass) {
this.pass = pass;
}
@Override
public String execute() throws Exception {
if(name.equals("123")&&pass.equals("456")){
return SUCCESS;
}
return ERROR;
}
}
/constant/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<package name="default" namespace="/" extends="struts-default">
<!-- 该action负责处理loginPro的请求,由Struts2.action.LoginProAction的execute方法处理 -->
<action name="loginPro" class="Struts2.action.LoginProAction">
<result>/WEB-INF/content/welcome.jsp</result>
</action>
<action name="*">
<result>/WEB-INF/content/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
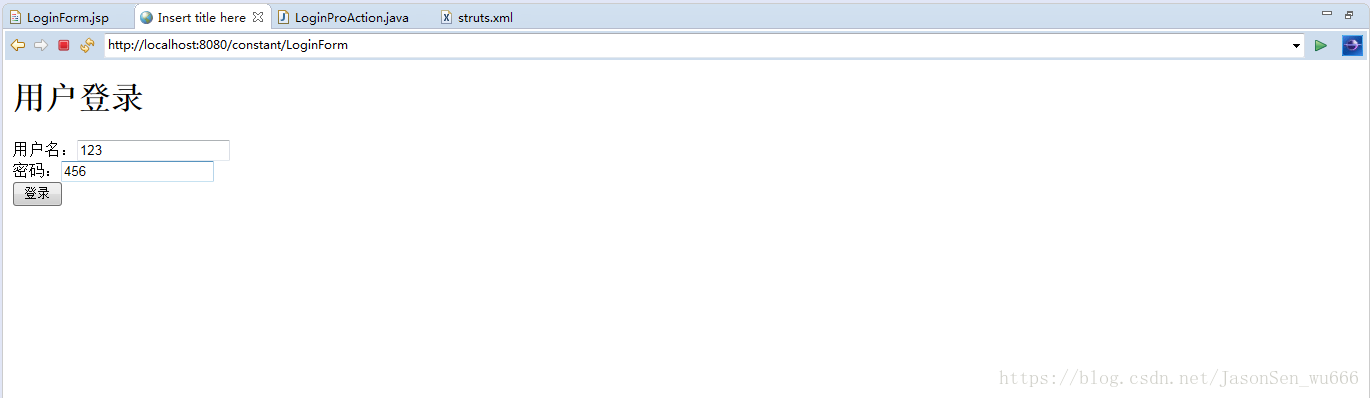

2.包含其他配置文件及包配置和模块化开发示范
配置包:
每个package元素定义了一个包配置,定义package元素时可以指定如下属性:
1、name:这是一个必填属性,指定该包的名字,该名字是该包被其他包引用的key。
2、extends:该属性是一个可选属性,指定该包继承其他包。可以继承其他包中的Action定义、拦截器定义等。
3、namespace:该属性是一个可选属性,定义该包的命名空间。
4、abstract:该属性是一个可选属性,他指定该包是否是一个抽象包,抽象包中不能包含Action定义。
/multiModule/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<constant name="struts.devMode" value="true"></constant>
<include file="struts-books.xml"></include>
<include file="struts-users.xml"></include>
</struts>
<!-- END SNIPPET: xworkSample -->
/multiModule/src/struts-books.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<!-- 指定了namespace为/books,意味着该包下面的所有Action都处于/books空间下 -->
<!-- 即当action的name值若为abc时,实际请求为/books/abc -->
<package name="books" namespace="/books" extends="struts-default">
<action name="*">
<result>/WEB-INF/content/books/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
/multiModule/src/struts-users.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<!-- 指定了namespace为/users,意味着该包下面的所有Action都处于/users空间下 -->
<!-- 即当action的name值若为abc时,实际请求为/users/abc -->
<package name="users" namespace="/users" extends="struts-default">
<action name="*">
<result>/WEB-INF/content/users/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
/multiModule/WebContent/WEB-INF/content/books/addBookForm.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>添加图书</title>
</head>
<body>
<form action="addPro" method="post">
图书名:<input type="text" name="book.name" /></br>
作者:<input type="text" name="book.author" /></br>
<input type="submit" value="添加" /></br>
</form>
</body>
</html>
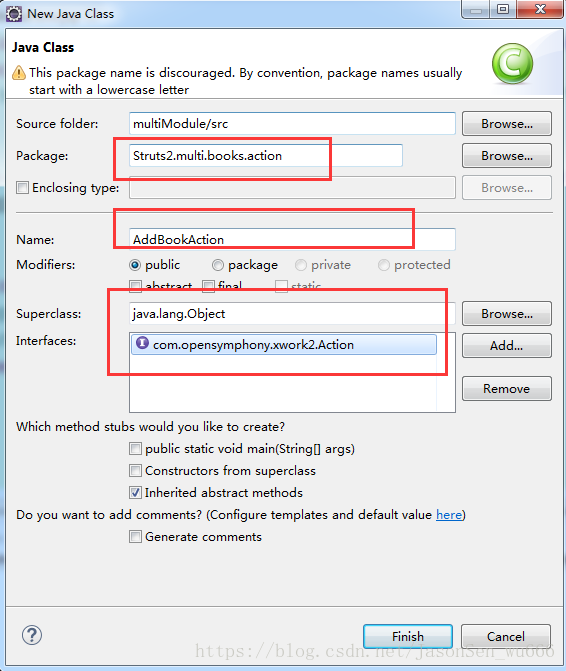
/multiModule/src/Struts2/multi/books/action/AddBookAction.java
程序代码如下:
package Struts2.multi.books.action;
import com.opensymphony.xwork2.Action;
public class AddBookAction implements Action {
private Book book;
public Book getBook() {
return book;
}
public void setBook(Book book) {
this.book = book;
}
@Override
public String execute() throws Exception {
//TODO Auto-generated method stub
return null;
}
}
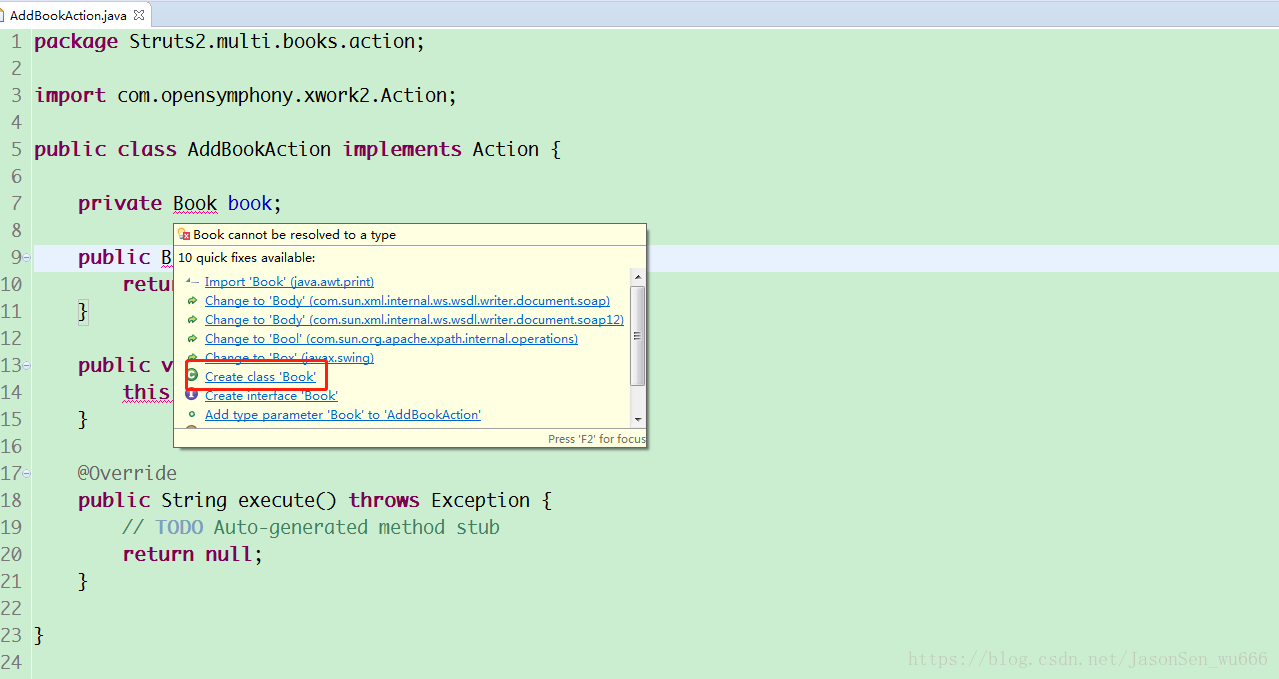
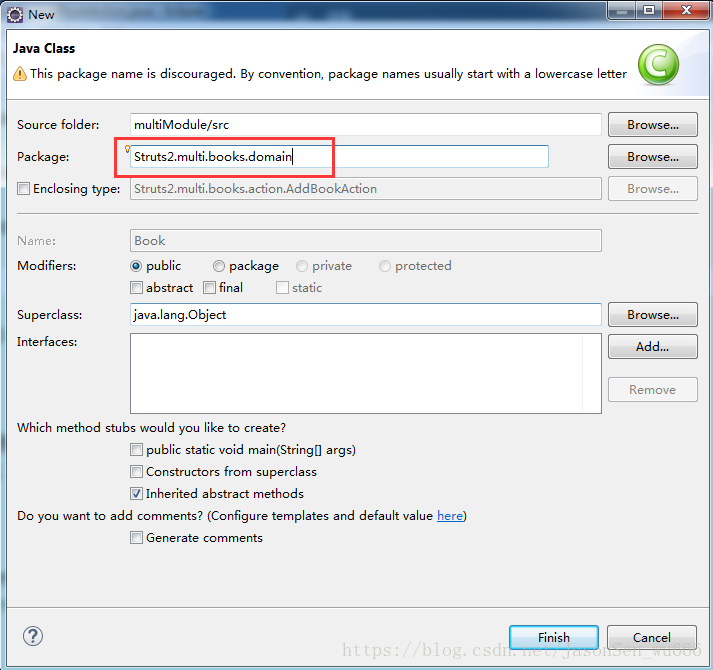
/multiModule/src/Struts2/multi/books/domain/Book.java
程序代码如下:
package Struts2.multi.books.domain;
public class Book {
private Integer id;
private String name;
private String author;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
}
/multiModule/src/Struts2/multi/books/action/AddBookAction.java
程序代码如下:
...
@Override
public String execute() throws Exception {
BookService bs = new BookService();
bs.addBook(book);
return SUCCESS;
}
...
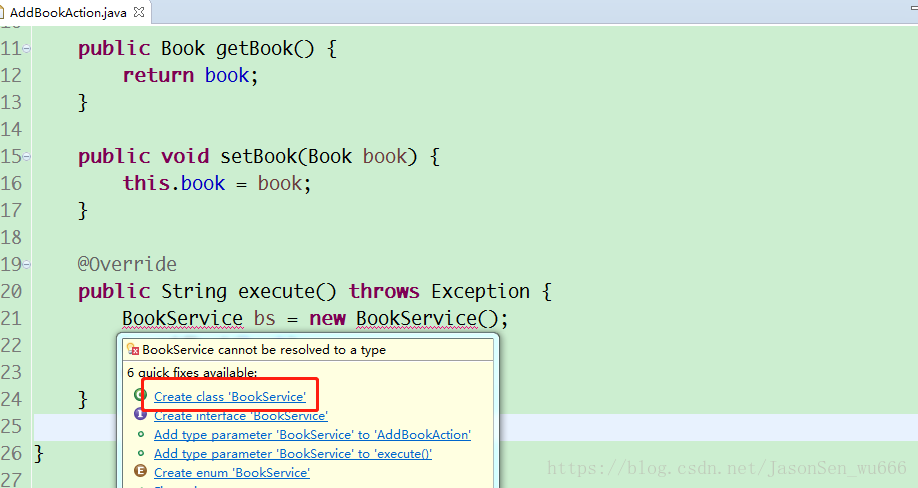
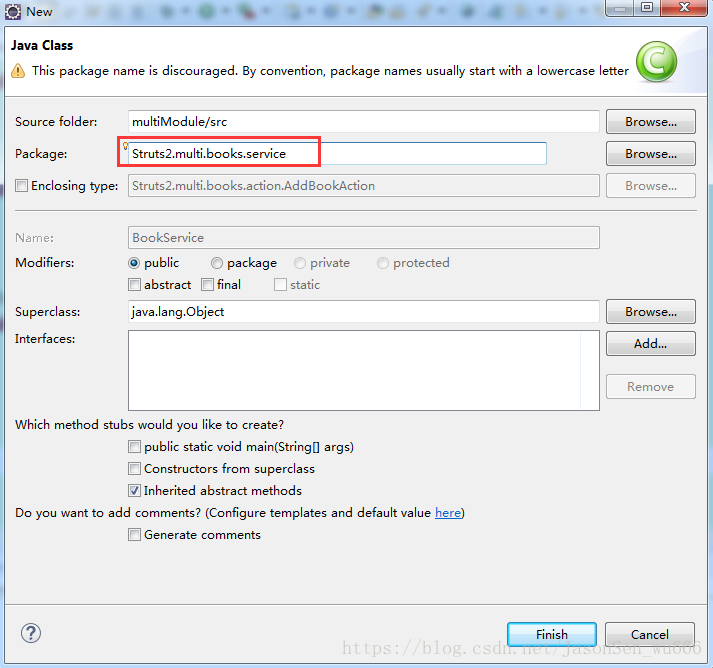
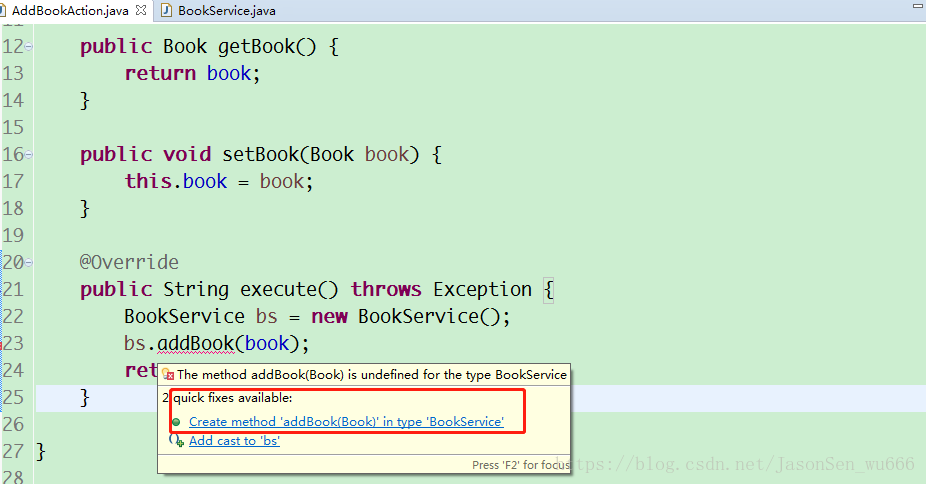
/multiModule/src/Struts2/multi/books/service/BookService.java
程序代码如下:
package Struts2.multi.books.service;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import Struts2.multi.books.domain.Book;
public class BookService {
static List<Book> bookDb = new ArrayList<>();
public void addBook(Book book) {
//为book添加一个随机ID
book.setId(new Random().nextInt(99999));
bookDb.add(book);
}
}
/multiModule/src/struts-books.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<!-- 指定了namespace为/books,意味着该包下面的所有Action都处于/books空间下 -->
<!-- 即当action的name值若为abc时,实际请求为/books/abc -->
<package name="books" namespace="/books" extends="struts-default">
<action name="addPro" class="Struts2.multi.books.action.AddBookAction">
<result>/WEB-INF/content/books/succ.jsp</result>
</action>
<action name="*">
<result>/WEB-INF/content/books/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
/multiModule/WebContent/WEB-INF/content/books/succ.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>添加成功</title>
</head>
<body>
<h3>添加成功</h3>
<a href="viewAllBooks">查看所有图书</a>
</body>
</html>
运行项目http://localhost:8080/multiModule/books/addBookForm


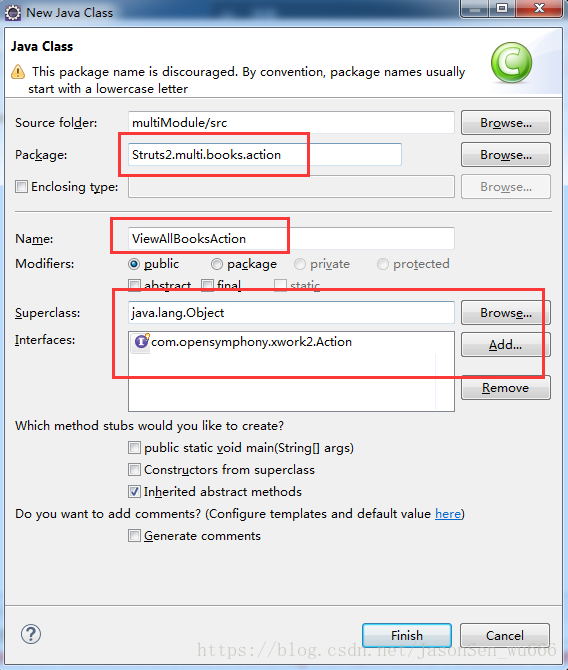
/multiModule/src/Struts2/multi/books/action/ViewAllBooksAction.java
程序代码如下:
package Struts2.multi.books.action;
import java.util.List;
import com.opensymphony.xwork2.Action;
import Struts2.multi.books.domain.Book;
import Struts2.multi.books.service.BookService;
public class ViewAllBooksAction implements Action {
private List<Book> books;
public List<Book> getBooks() {
return books;
}
public void setBooks(List<Book> books) {
this.books = books;
}
@Override
public String execute() throws Exception {
BookService bs = new BookService();
setBooks(bs.getAllBooks());
return SUCCESS;
}
}
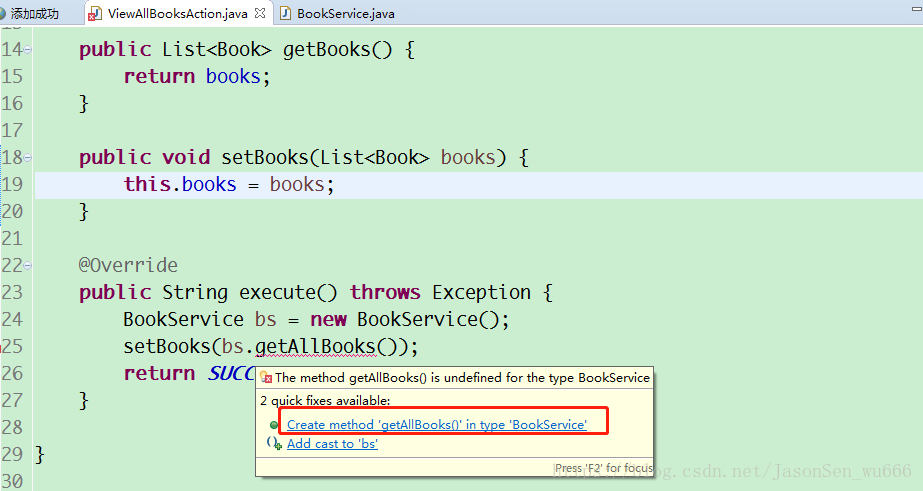
/multiModule/src/Struts2/multi/books/service/BookService.java
程序代码如下:
...
public List<Book> getAllBooks() {
return bookDb;
}
...
/multiModule/src/struts-books.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<!-- 指定了namespace为/books,意味着该包下面的所有Action都处于/books空间下 -->
<!-- 即当action的name值若为abc时,实际请求为/books/abc -->
<package name="books" namespace="/books" extends="struts-default">
<action name="addPro" class="Struts2.multi.books.action.AddBookAction">
<result>/WEB-INF/content/books/succ.jsp</result>
</action>
<action name="viewAllBooks" class="Struts2.multi.books.action.ViewAllBooksAction">
<result>/WEB-INF/content/books/listBooks.jsp</result>
</action>
<action name="*">
<result>/WEB-INF/content/books/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
/multiModule/WebContent/WEB-INF/content/books/listBooks.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<%@ taglib prefix="s" uri="/struts-tags" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>查看所有图书</title>
</head>
<body>
<table width="500" border="1">
<s:iterator value="books" var="b" >
<tr>
<td>${b.id}</td>
<td>${b.name}</td>
<td>${b.author}</td>
</tr>
</s:iterator>
</table>
</body>
</html>




users文件夹下添加loginForm.jsp
/multiModule/WebContent/WEB-INF/content/users/loginForm.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>登录</title>
</head>
<body>
<h1>用户登录</h1>
<form action="loginPro" method="post">
用户名:<input type="text" name="name" /></br>
密码:<input type="text" name="pass" /></br>
<input type="submit" value="登录" /></br>
</form>
</body>
</html>
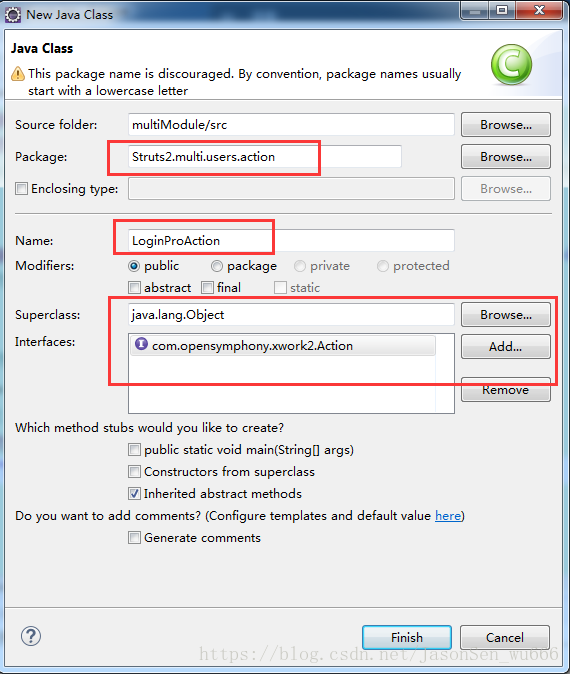
/multiModule/src/Struts2/multi/users/action/LoginProAction.java
程序代码如下:
package Struts2.multi.users.action;
import com.opensymphony.xwork2.Action;
import com.opensymphony.xwork2.ActionContext;
public class LoginProAction implements Action {
private String name;
private String pass;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPass() {
return pass;
}
public void setPass(String pass) {
this.pass = pass;
}
@Override
public String execute() throws Exception {
if(name.equals("123")&&pass.equals("456")){
//把用户登录的用户名设置到Session中
ActionContext.getContext().getSession().put("userName", name);
return SUCCESS;
}
return ERROR;
}
}
/multiModule/src/struts-users.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<!-- 指定了namespace为/users,意味着该包下面的所有Action都处于/users空间下 -->
<!-- 即当action的name值若为abc时,实际请求为/users/abc -->
<package name="users" namespace="/users" extends="struts-default">
<action name="loginPro" class="Struts2.multi.users.action.LoginProAction">
<result>/WEB-INF/content/users/welcome.jsp</result>
<result name="error">/WEB-INF/content/users/fail.jsp</result>
</action>
<action name="*">
<result>/WEB-INF/content/users/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
/multiModule/WebContent/WEB-INF/content/users/welcome.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>登录成功</title>
</head>
<body>
<h1>登陆成功</h1>
欢迎你,${sessionScope.userName}
</body>
</html>
/multiModule/WebContent/WEB-INF/content/users/fail.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>登录失败</title>
</head>
<body>
<h1>登录失败</h1>
</body>
</html>
运行项目http://localhost:8080/multiModule/users/loginForm
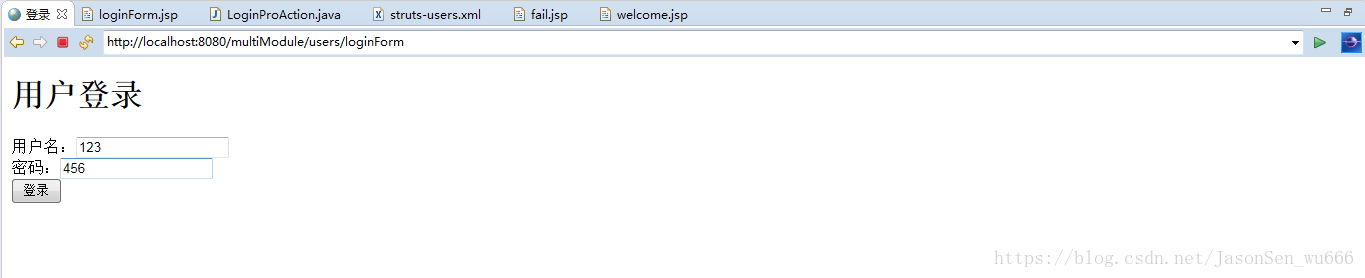

3.Struts2的结果配置
一般的MVC:Servlet(控制器)调用Model处理完用户请求之后,使用reques.getRequestDispatcher(“/abc.jsp”).forward(request,response)
。
缺点:跳转代码已经写写跳转到某个物理视图(硬编码与物理视图资源耦合),在未来的日子里,软件可能要跳转到其他页面(甚至不再使用jsp视图),如果为硬编码耦合,必需手动修改代码。
Struts2做法:Action调用Model处理完用户请求之后,只是返回了一个String类型的逻辑视图名,然后在struts.xml文件中通过<result…/>
元素定义“逻辑视图名”与物理视图之间的对应关系。这样就把原来的硬编码耦合提取到xml配置文件中进行管理。
逻辑视图名与物理视图的关系,是通过<result…/>
元素来管理。
标准的< result >格式:
<result name=“ ” type=“ ”>
<param></param>
<param></param>
…
</result>
支持如下属性
1、name:指定逻辑视图名,默认是success。
2、type:指定所使用的物理视图类型。默认是dispatcher。
如果有如下result配置:
<result name=“逻辑视图名” type=“视图类型”>
<param name=“location”>逻辑视图的位置</param>
<param name=“parse”>true</param> --- 是否解析OGNL表达式的值
</result>
可以简化成如下写法(即默认值可省略):
<result name=“逻辑视图名” type=“视图类型”> 物理视图的位置 </result>
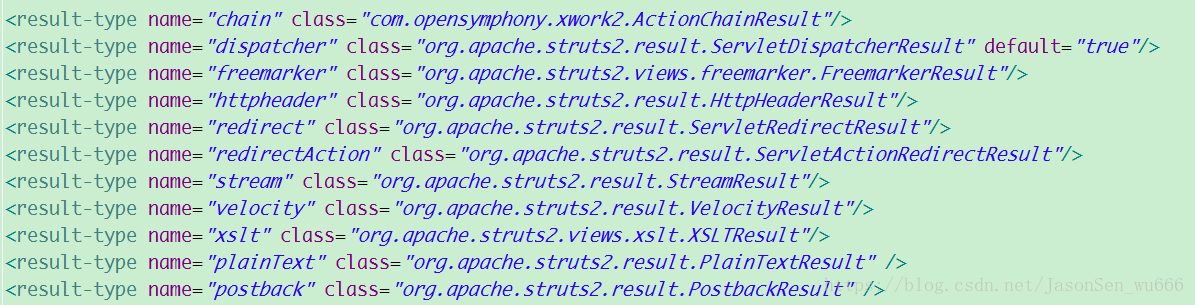
forward依旧是一次请求,地址栏的URL没有变化,请求参数和请求属性都不会丢失。
Redirect重新发送一次新请求(类似于直接在地址栏输入新URL并回车),地址栏的URL会变化,请求参数和请求属性全部都将丢失。
4.常见的集中Result类型及用法示例以及动态结果配置
常见的结果类型:
chain:Action处理完用户请求之后,转发到下一个Action继续处理,行成链式处理。
redirect:直接“重定向”到新的URL,会生成一次新的请求,与默认的dispatch(转发)对应。
dispatch:默认值,转发到jsp页面。
plaintext:直接显示视图的源代码。
stream:直接生成“二进制”流作为响应。
两种result配置:
局部result:将<result…/>
作为<action…/>
元素的子元素配置。
全局result:将<result…/>
作为<global-result…/>
元素的子元素配置。
当全局result配置与局部result配置冲突时,局部result配置会覆盖全局result。尽量少用全局result,只有在多个Action具有某个通用性质的result时,才考虑使用全局result。
chain结果类型案例
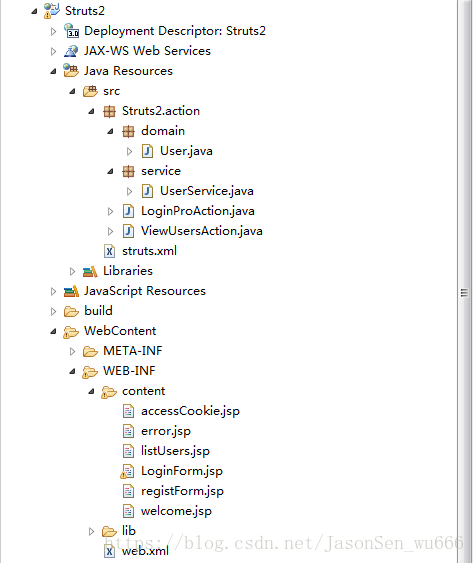
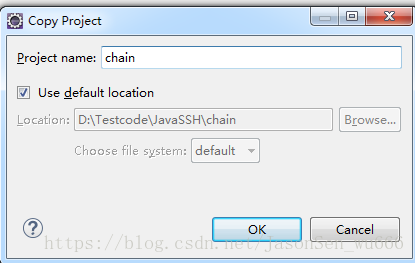
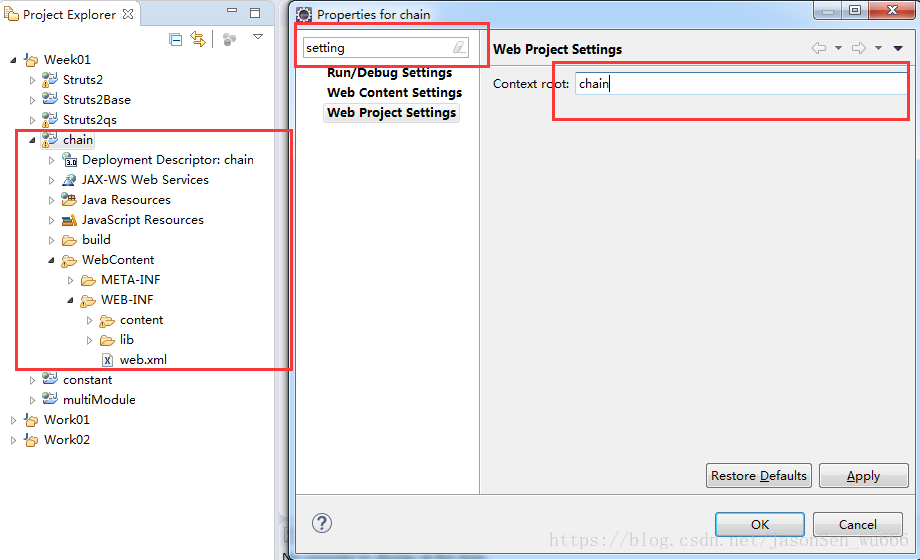
/chain/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<!-- 所有的配置都要放在package元素中,而且包必须继承struts-default -->
<package name="default" namespace="/" extends="struts-default">
<!-- 该action负责处理loginPro的请求,由Struts2.action.LoginProAction的execute(默认)方法处理 -->
<action name="loginPro" class="Struts2.action.LoginProAction" >
<result name="success">/WEB-INF/content/welcome.jsp</result>
<result name="error">/WEB-INF/content/error.jsp</result>
</action>
<!-- 该action负责处理registPro的请求,由Struts2.action.LoginProAction的"regist"方法处理 -->
<action name="registPro" class="Struts2.action.LoginProAction" method="regist">
<!-- 添加完用户之后,我们希望直接跳转到列出所有用户的页面
指定type为chain,就说明处理完之后没转入下一个Action继续进行处理-->
<result type="chain">viewUsers</result>
</action>
<!-- 该action负责处理viewUsers的请求,由Struts2.action.ViewUsersAction.java的execute方法处理 -->
<action name="viewUsers" class="Struts2.action.ViewUsersAction" >
<result name="success">/WEB-INF/content/listUsers.jsp</result>
</action>
<!-- 1.它是一个通用Action,此处的*表明它可以处理任意的请求 -->
<!-- 2.亦可以设为Action*,此处的Action为第一个参数,*号为第二个参数 -->
<action name="*">
<!-- 1.此处{1}代表*号所匹配的字符串 -->
<!-- 2.此处若为{1}.{2}.jsp,1代表第一个参数,2代表*号所匹配的字符串 -->
<result>/WEB-INF/content/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
运行项目http://localhost:8080/chain/registForm
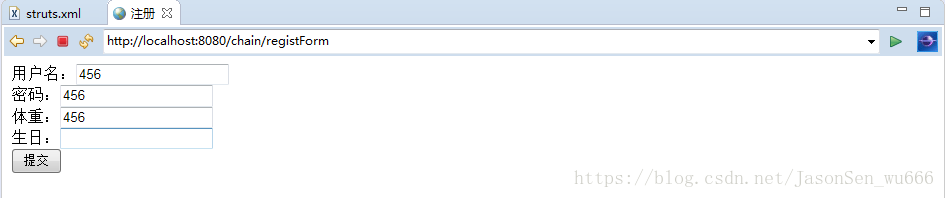
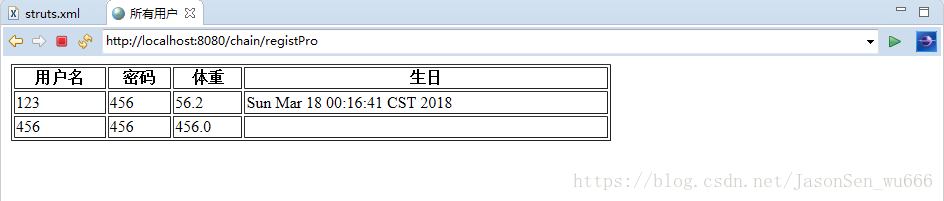
plainText结果类型案例
简单修改welcome.jsp
/plainText/WebContent/WEB-INF/content/welcome.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>欢迎</title>
</head>
<body>
欢迎,${requestScope.name},登录成功<br>
</body>
</html>
修改struts.xml
/plainText/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<package name="default" namespace="/" extends="struts-default">
<!-- 该action负责处理loginPro的请求,由Struts2.action.LoginProAction的execute方法处理 -->
<action name="loginPro" class="Struts2.action.LoginProAction">
<result type="plainText">
<param name="location">/WEB-INF/content/welcome.jsp</param>
</result>
</action>
<action name="*">
<result>/WEB-INF/content/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
运行项目http://localhost:8080/plainText/LoginForm

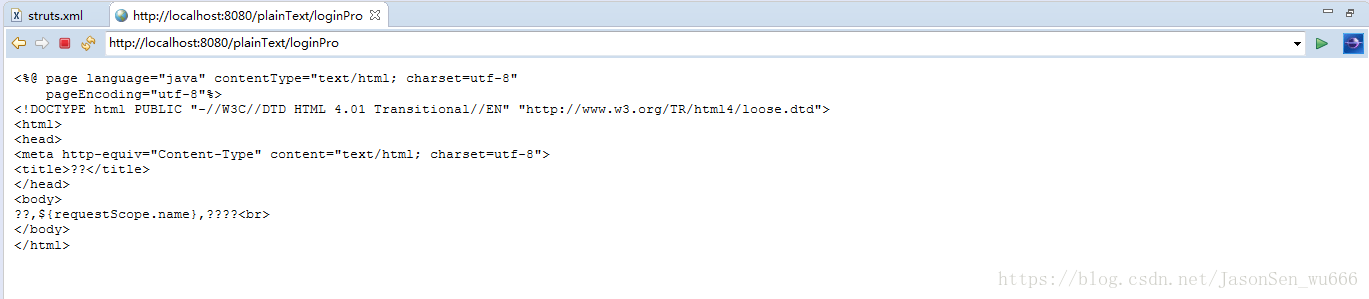
/plainText/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<package name="default" namespace="/" extends="struts-default">
<!-- 该action负责处理loginPro的请求,由Struts2.action.LoginProAction的execute方法处理 -->
<action name="loginPro" class="Struts2.action.LoginProAction">
<result type="plainText">
<param name="location">/WEB-INF/content/welcome.jsp</param>
<!-- 指定物理视图资源编码的字符集 -->
<param name="charSet">utf-8</param>
</result>
</action>
<action name="*">
<result>/WEB-INF/content/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
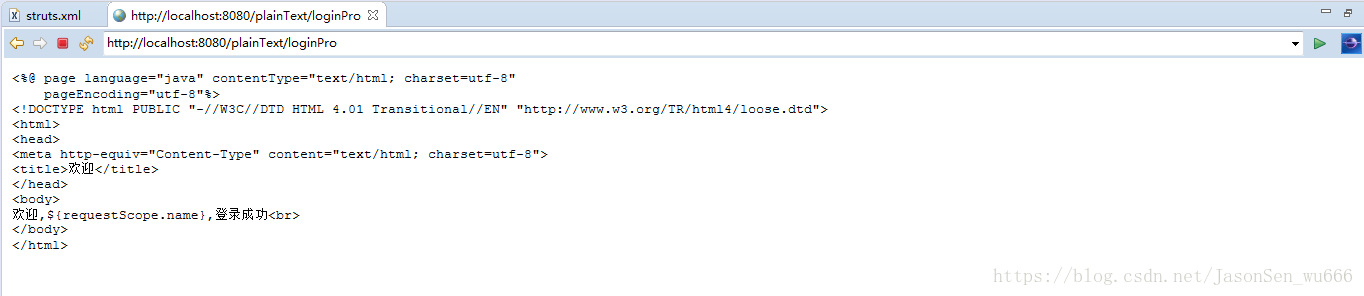
全局结果配置案例
修改struts.xml
/globalResult/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<package name="default" namespace="/" extends="struts-default">
<!-- 在该元素配置result将会对所有Action有效 -->
<global-results>
<result type="plainText">
<param name="location">/WEB-INF/content/welcome.jsp</param>
<!-- 指定物理视图资源编码的字符集 -->
<param name="charSet">utf-8</param>
</result>
</global-results>
<!-- 该action负责处理loginPro的请求,由Struts2.action.LoginProAction的execute方法处理 -->
<action name="loginPro" class="Struts2.action.LoginProAction">
</action>
<action name="*">
<result>/WEB-INF/content/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
动态result案例
新建一个myForm.jsp
/dynaResult/WebContent/WEB-INF/content/myForm.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>起始表单页</title>
</head>
<body>
<form action="myPro" method="post">
参数:<input type="text" name="res" /></br>
<input type="submit" value="提交" /></br>
</form>
</body>
</html>
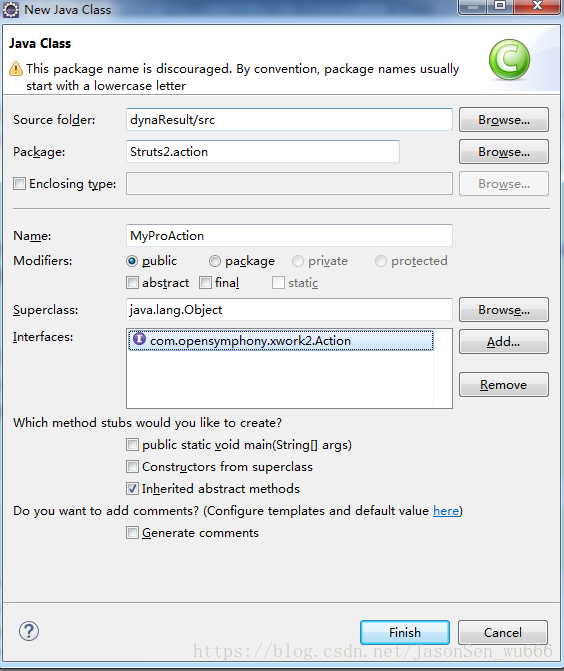
/dynaResult/src/Struts2/action/MyProAction.java
程序代码如下:
package Struts2.action;
import com.opensymphony.xwork2.Action;
public class MyProAction implements Action {
private String res;
public String getRes() {
return res;
}
public void setRes(String res) {
this.res = res;
}
@Override
public String execute() throws Exception {
// TODO Auto-generated method stub
return SUCCESS;
}
}
/dynaResult/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<package name="default" namespace="/" extends="struts-default">
<!--
在定义物理视图资源时,可以直接使用OGNL表达式
所以这个物理视图资源可以动态的改变。
通过OGNL来计算${res}表达式的值,
-->
<action name="myPro" class="Struts2.action.MyProAction">
<result>/WEB-INF/content/${res}.jsp</result>
</action>
<action name="*">
<result>/WEB-INF/content/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
运行项目`http://localhost:8080/dynaResult/myForm
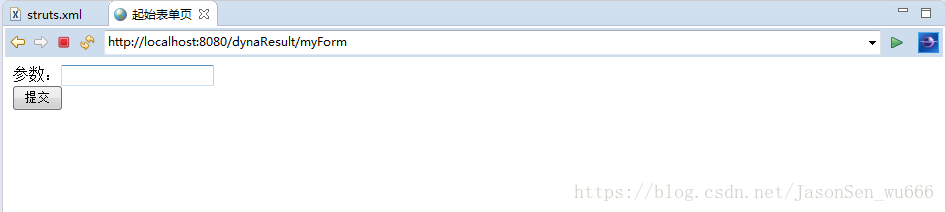
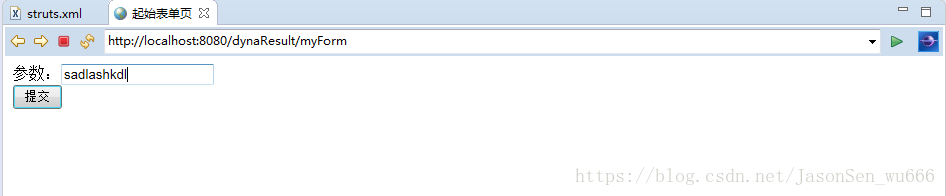
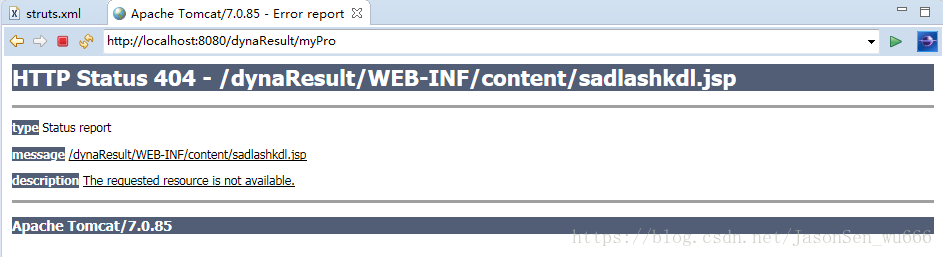
5.动态方法调用(DMI)以及优劣分析
动态方法调用(DMI):可以在提交请求时,直接提交给Action的指定方法进行处理。就在页面上指定请求地址为:action!+方法名。
修改LoginForm.jsp
/dmi/WebContent/WEB-INF/content/LoginForm.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>登录</title>
</head>
<body>
<h1>用户登录</h1>
<!--
如果没有使用动态方法调用,此处只能看出需要提交给哪个Action处理,无法看出提交给哪个方法处理。
如果使用DMI,可以直接在action增加"!方法名",就可以指定提交到某个Actoin的某个方法处理。
-->
<form action="loginRegistPro!login" method="post">
用户名:<input type="text" name="user.name" /></br>
密码:<input type="text" name="user.pass" /></br>
<input type="submit" value="登录" /></br>
</form>
</body>
</html>
修改registForm.jsp
/dmi/WebContent/WEB-INF/content/registForm.jsp
程序代码如下:
<%@ page language="java" contentType="text/html; charset=utf-8"
pageEncoding="utf-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>注册</title>
</head>
<body>
<form action="loginRegistPro!regist" method="post">
用户名:<input type="text" name="user.name"/><br>
密码:<input type="text" name="user.pass"/><br>
体重:<input type="text" name="user.weight"/><br>
生日:<input type="text" name="user.birth"/><br>
<input type="submit" value="提交" />
</form>
</body>
</html>
修改LoginProAction.java
/dmi/src/Struts2/action/LoginProAction.java
程序代码如下:
package Struts2.action;
import java.util.Map;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletResponse;
import org.apache.struts2.ServletActionContext;
import com.opensymphony.xwork2.Action;
import com.opensymphony.xwork2.ActionContext;
import Struts2.action.domain.User;
import Struts2.action.service.UserService;
public class LoginProAction {
// 定义一个User类,封装一个user属性
// 可以通过该user来访问请求参数
private User user;
public User getUser() {
return user;
}
public void setUser(User user) {
this.user = user;
}
//用户请求
public String login() throws Exception {
UserService us = new UserService();
//此时的user已经可以访问请求参数了
//调用业务逻辑组件来处理用户登录
Integer id = us.validateLogin(user);
if(id > 0)
{
ActionContext actCtx = ActionContext.getContext();
//使用了一个Map来模拟HTTP Seesion
Map<String, Object> sess = actCtx.getSession();
//下面代码,表面上只是向Map存入key-value对,但由于该Map模拟HTTP Seesion
//因此下面存入的key-value对,实际会存入HTTP Seesion
sess.put("userId", id);
//此处如果希望用户登录成功,还要添加Cookie
//由于添加Cookie必须用HttpServletResponse对象,所以该Action必须与Servlet耦合
HttpServletResponse response = ServletActionContext.getResponse();
Cookie cookie = new Cookie("userName" , user.getName());
cookie.setMaxAge(300000);
//添加Cookie
response.addCookie(cookie);
return Action.SUCCESS;//SUCCESS是常量名,它的真实值是“success”
}
return Action.ERROR;
}
public String regist(){
UserService us = new UserService();
us.addUser(user);
return Action.SUCCESS;
}
}
修改struts.xml
/dmi/src/struts.xml
程序代码如下:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE struts PUBLIC
"-//Apache Software Foundation//DTD Struts Configuration 2.5//EN"
"http://struts.apache.org/dtds/struts-2.5.dtd">
<!-- START SNIPPET: xworkSample -->
<struts>
<constant name="struts.enable.DynamicMethodInvocation" value="true" />
<!-- 所有的配置都要放在package元素中,而且包必须继承struts-default -->
<package name="default" namespace="/" extends="struts-default">
<global-allowed-methods>regex:.*</global-allowed-methods>
<!-- 该action负责处理loginPro的请求,由Struts2.action.LoginProAction的execute(默认)方法处理 -->
<action name="loginRegistPro" class="Struts2.action.LoginProAction" >
<result name="success">/WEB-INF/content/welcome.jsp</result>
<result name="error">/WEB-INF/content/error.jsp</result>
</action>
<!-- 该action负责处理viewUsers的请求,由Struts2.action.ViewUsersAction.java的execute方法处理 -->
<action name="viewUsers" class="Struts2.action.ViewUsersAction" >
<result name="success">/WEB-INF/content/listUsers.jsp</result>
</action>
<!-- 1.它是一个通用Action,此处的*表明它可以处理任意的请求 -->
<!-- 2.亦可以设为Action*,此处的Action为第一个参数,*号为第二个参数 -->
<action name="*">
<!-- 1.此处{1}代表*号所匹配的字符串 -->
<!-- 2.此处若为{1}.{2}.jsp,1代表第一个参数,2代表*号所匹配的字符串 -->
<result>/WEB-INF/content/{1}.jsp</result>
</action>
</package>
</struts>
<!-- END SNIPPET: xworkSample -->
运行项目
http://localhost:8080/dmi/registForm
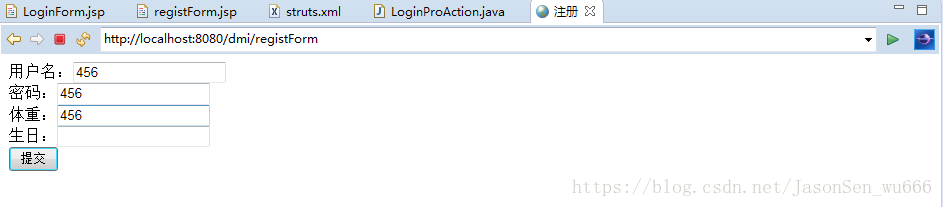

http://localhost:8080/dmi/LoginForm
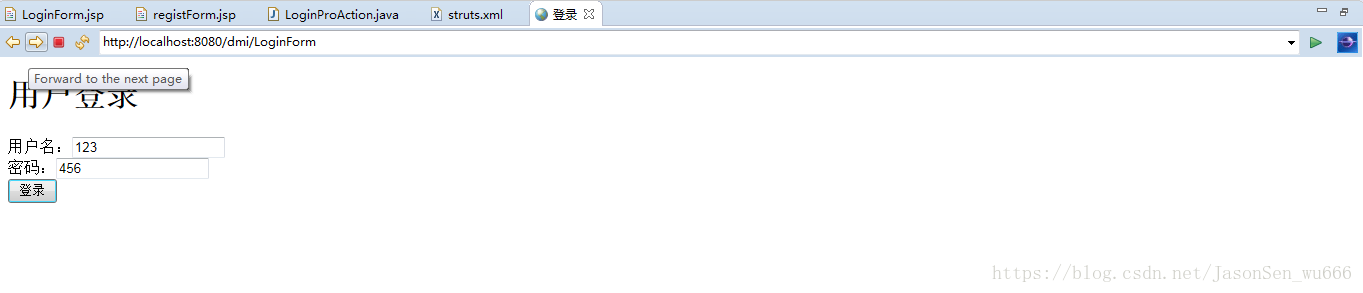
