一:Cooiek
1.首先创建Web项目CooiekDemo01
2.创建login.jsp
3.在login.jsp写入代码制作一个登录界面
代码如下:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8" %>
<html>
<head>
<title>用户登录</title>
</head>
<body>
<h3 style="text-align: center">用户登录</h3>
<form action="do_login.jsp" method="post">
<table border="1" cellpadding="10" style="margin: 0px auto">
<tr>
<td align="center">用户名</td>
<td><input type="text" id = "uname" name="username"/></td>
</tr>
<tr>
<td align="center">密 码</td>
<td><input type="password" id="upwd" name="password"/></td>
</tr>
<tr >
<td colspan="2"><input type="checkbox" name="savepwd" id="savepwd"/>记住密码</td>
</tr>
<tr align="center">
<td colspan="2">
<input type="submit" value="登录"/>
<input type="reset" value="重置"/>
</td>
</tr>
</table>
</form>
4.新建一个名为do_login.jsp作为登录的处理
5.写入代码实现登录的密码校验
代码如下:
<%
//获取登录表单的数据
String username = request.getParameter("username");
String password = request.getParameter("password");
String[] choice = request.getParameterValues("savepwd");
//判断用户是否登录成功
if (username.equals("烧烤") && password.equals("123")) {
//创建cookie对象,写入客户端
Cookie uname = new Cookie("uname",username);
Cookie upwd = new Cookie("upwd",password);
Cookie savepwd = new Cookie("savepwd", "no");
response.addCookie(uname);
//判断是否选择了“记住密码”,如果没选择,则返回空
if (choice != null) {
savepwd = new Cookie("savepwd", "yes");
}
response.addCookie(uname);
response.addCookie(upwd);
response.addCookie(savepwd);
// Cookie check = new Cookie("check_password",password);
// response.addCookie(check);
//采用重定向跳转到登录成功页面
response.sendRedirect("success.jsp");
} else {
//采用重定向跳转到登录页面
response.sendRedirect("login.jsp");
}
%>
6.新建一个success.jsp文件,用作登录成功后的提示页面
在success.jsp中写入登录成功后页面的代码
代码如下:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>登录成功</title>
</head>
<body>
<%
String uname = "";
Cookie[] cookies = request.getCookies();
for (Cookie cookie: cookies) {
if (cookie.getName().equals("uname")) {
uname = cookie.getValue();
break;
}
}
%>
<h3><%= new String (uname.getBytes("iso-8859-1"),"utf-8") %>,登录成功!</h3>
</body>
</html>
7.修改login页面,新增自动添加用户名和密码的功能
代码如下:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8" %>
<html>
<head>
<title>用户登录</title>
</head>
<body>
<h3 style="text-align: center">用户登录</h3>
<form action="do_login.jsp" method="post">
<table border="1" cellpadding="10" style="margin: 0px auto">
<tr>
<td align="center">用户名</td>
<td><input type="text" id = "uname" name="username"/></td>
</tr>
<tr>
<td align="center">密 码</td>
<td><input type="password" id="upwd" name="password"/></td>
</tr>
<tr >
<td colspan="2"><input type="checkbox" name="savepwd" id="savepwd"/>记住密码</td>
</tr>
<tr align="center">
<td colspan="2">
<input type="submit" value="登录"/>
<input type="reset" value="重置"/>
</td>
</tr>
</table>
</form>
<%
String uname = "";
String upwd="";
String savepwd = "";
Cookie[] cookies = request.getCookies();
for (Cookie cookie: cookies) {
if (cookie.getName().equals("uname")) {
uname = cookie.getValue();
}
if (cookie.getName().equals("upwd")) {
upwd = cookie.getValue();
}
if (cookie.getName().equals("savepwd")) {
savepwd = cookie.getValue();
}
}
%>
<script type="text/javascript">
var chkSavepwd = document.getElementById("savepwd");
var savepwd = "<%= savepwd %>";
if (savepwd == "yes") {
chkSavepwd.checked = true;
}
var txtUname = document.getElementById("uname");
txtUname.value = "<%= new String(uname.getBytes("iso-8859-1"),"utf-8") %>";
var savepwd = document.getElementById("savepwd");
if (savepwd.checked) {
var txtUpwd = document.getElementById("upwd");
txtUpwd.value = "<%= upwd %>";
}
</script>
</body>
</html>
最后运行tomcat实现效果
效果如下:
!最后可以加上记住密码和记住用户名,并且设置成:
1.用户名和密码同时记住;
2.用户名记住
3.都不记住
首先在login中修改代码:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8" %>
<html>
<head>
<title>用户登录</title>
</head>
<body>
<h3 style="text-align: center">用户登录</h3>
<form action="do_login.jsp" method="post">
<table border="1" cellpadding="10" style="margin: 0px auto">
<tr>
<td align="center">用户名</td>
<td><input type="text" id = "uname" name="username"/></td>
</tr>
<tr>
<td align="center">密 码</td>
<td><input type="password" id="upwd" name="password"/></td>
</tr>
<tr >
<td colspan="2"><input type="checkbox" name="save" id="savename" onclick="setchkupwd()"/>记住用户名
<input type="checkbox" name="save" id="savepwd" onclick="setchkuname()"/>记住密码</td>
</tr>
<tr align="center">
<td colspan="2">
<input type="submit" value="登录"/>
<input type="reset" value="重置"/>
</td>
</tr>
</table>
</form>
<%
String uname = "";
String upwd="";
String savepwd = "";
String savename = "";
Cookie[] cookies = request.getCookies();
for (Cookie cookie: cookies) {
if (cookie.getName().equals("uname")) {
uname = cookie.getValue();
}
if (cookie.getName().equals("upwd")) {
upwd = cookie.getValue();
}
if (cookie.getName().equals("savepwd")) {
savepwd = cookie.getValue();
}
if (cookie.getName().equals("savename")) {
savename = cookie.getValue();
}
}
%>
<script type="text/javascript">
var chkSavepwd = document.getElementById("savepwd");
var savepwd = "<%= savepwd %>";
if (savepwd == "yes") {
chkSavepwd.checked = true;
}
var chkSavename = document.getElementById("savename");
var savename = "<%= savename %>";
if (savename == "yes") {
chkSavename.checked = true;
}
var savename = document.getElementById("savename");
if (savename.checked) {
var txtUname = document.getElementById("uname");
txtUname.value = "<%= new String(uname.getBytes("iso-8859-1"),"utf-8") %>";
}
var savepwd = document.getElementById("savepwd");
if (savepwd.checked) {
var txtUpwd = document.getElementById("upwd");
txtUpwd.value = "<%= upwd %>";
}
function setchkuname() {
if (chkSavepwd.checked) {
chkSavename.checked = true;
}
}
function setchkupwd() {
if (chkSavepwd.checked) {
chkSavepwd.checked = false;
}
}
</script>
</body>
</html>
然后在do_login中修改代码:
<%
//获取登录表单的数据
String username = request.getParameter("username");
String password = request.getParameter("password");
String[] choice = request.getParameterValues("save");
String[] choiceName = request.getParameterValues("savename");
//判断用户是否登录成功
if (username.equals("烧烤") && password.equals("123")) {
//创建cookie对象,写入客户端
Cookie uname = new Cookie("uname",username);
Cookie upwd = new Cookie("upwd",password);
Cookie savepwd = new Cookie("savepwd", "no");
Cookie savename = new Cookie("savename", "no");
//判断是否选择了“记住密码”,如果没选择,则返回空
//只选择用户名,或者同时选择,或者都不选择
if (choice != null) {
if (choice.length == 2) {
savename.setValue("yes");
savepwd.setValue("yes");
} else {
savename.setValue("yes");
}
}
response.addCookie(uname);
response.addCookie(upwd);
response.addCookie(savepwd);
response.addCookie(savename);
//采用重定向跳转到登录成功页面
response.sendRedirect("success.jsp");
// if ((choice != null && choiceName != null) | (choiceName != null && choice == null ) | (choice == null && choiceName == null)) {
// if (choice != null) {
// savepwd = new Cookie("savepwd", "yes");
// }
// if (choiceName != null) {
// savename = new Cookie("savename", "yes");
// }
//
//
// } else {
//
// //采用重定向跳转到登录页面
// response.sendRedirect("login.jsp");
//
// }
} else {
//采用重定向跳转到登录页面
response.sendRedirect("login.jsp");
}
%>
最后演示结果:
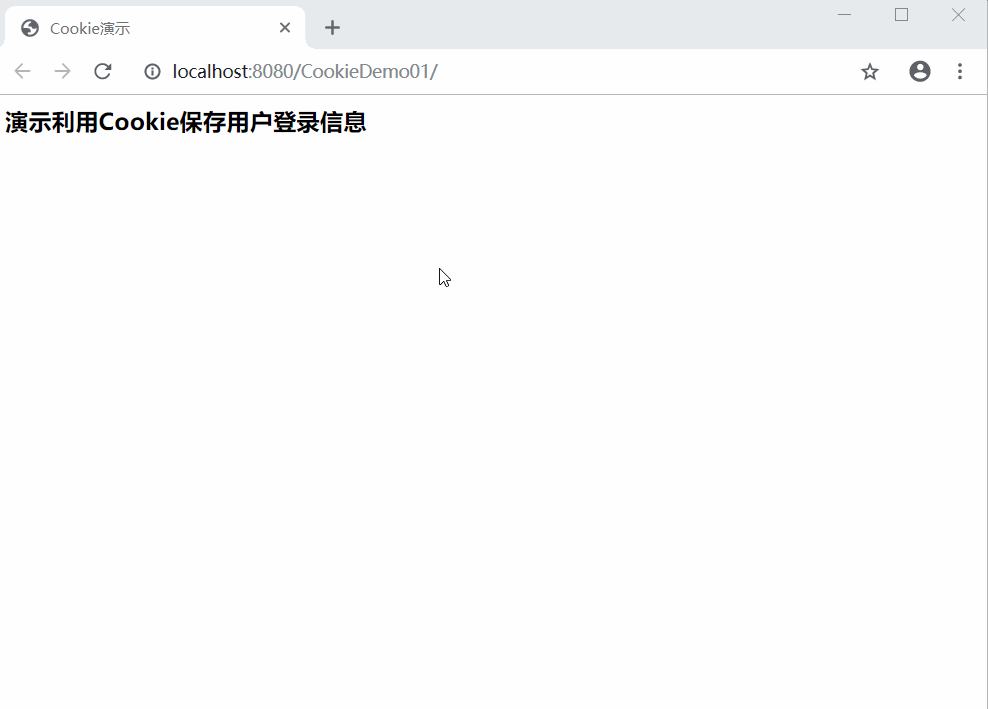
二:Session对象
1.首先创建一个Web项目SessionDemo01
2.在src目录下创建包net.zzk.bean
3.在包下创建User实体类
package net.zzk.bean;
public class User {
private int id;
private String username;
private String password;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
4.创建一个login.jsp文件实现登录窗口
login页面代码如下:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h3 style="text-align: center">登录界面</h3>
<form action="do_login.jsp" method="post">
<table border="1" align="center">
<tr>
<td>用户名</td>
<td><input type="text" name="username"></td>
</tr>
<tr>
<td>密 码</td>
<td><input type="password" name="password"></td>
</tr>
<tr align="center">
<td colspan="2">
<input type="submit" value="登录"><input type="reset" value="重置">
</td>
</tr>
</table>
</form>
<%
String errMsg = (String) session.getAttribute("errMsg");
if (errMsg != null) {
out.print("<script>alert('" + errMsg + "')</script>");
}
%>
</body>
</html>
5.创建index.jsp文件用作新闻建设首页
<%--
Created by IntelliJ IDEA.
User: 23684
Date: 2019/10/14
Time: 10:17
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>首页</title>
</head>
<body>
1.港媒:黎智英儿子在香港的三间餐厅被泼红色油漆<br>
2.普京无惧与美展开军备竞赛:俄已拥有新一代武器<br>
3.超载久治不愈病灶在哪儿 专家:处罚力度明显不足<br>
4.李心草母亲:从媒体获知意外落水消息 打人算醒酒?<br>
5.光头警长刘Sir:不到万不得已 我不会把枪指向他们<br>
6.暖新闻 乘客打到"治愈系"出租车:整座城市都变美好了<br>
7.货船台风天在日本近海沉没 5名中国籍船员遇难<br>
</body>
</html>
6.创建add_news.jsp文件用作添加新闻网页
<%@ page import="net.zzk.bean.User" %><%--
Created by IntelliJ IDEA.
User: 23684
Date: 2019/10/14
Time: 10:36
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>添加新闻</title>
</head>
<body>
<%
User user = (User) session.getAttribute("LOGINED_USER");
if (user == null) {
session.setAttribute("errMsg","要访问添加新闻页面,请先登录!");
response.sendRedirect("login.jsp");
} else {
session.removeAttribute("errMsg");
}
%>
<h3>添加新闻</h3>
此页面还在建设中…………
</body>
</html>
7.将login登录界面的数据返回到do_login中进行处理
<%@ page import="net.zzk.bean.User" %><%
//获取表单数据
String username = request.getParameter("username");
String password = request.getParameter("password");
//判断登录是否成功
if (username.equals("烧烤") && password.equals("123")) {
//创建用户对象
User user = new User();
//设置用户对象属性
user.setUsername(username);
user.setPassword(password);
//保存登录用户信息
session.setAttribute("LOGINED_USER",user);
//采用重定向跳转到首页
response.sendRedirect("index.jsp");
} else {
//采用重定向,跳转到登录页面
response.sendRedirect("login.jsp");
}
%>
总文件:
效果:在未登录的前提下进入add_news网页,提示未登录并且返回到login登录界面让用户进行登录,登录之后方可进入到add_news网页中进行修改查看。
运行效果如下:
!对未登录的用户进行拒绝访问网页,登录之后才可进入,对网页进行访问控制
三:Application
1.创建一个ApplicationDemo01的Web项目
2.在Web-INF中创建lib包,添加数据库驱动程序jar包
3.在Web里创建index.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>演示Application对象</title>
</head>
<body>
<h3>演示Application对象</h3>
Application类似于系统的“全局变量”,用于实现用户之间的数据共享
</body>
</html>
4.在Web目录里创建login.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>登录界面</title>
</head>
<body>
<h3 style="text-align: center">登录界面</h3>
<form action="login_do.jsp" method="post">
<table border="1" align="center">
<tr>
<td>用户名</td>
<td><input type="text" name="username"></td>
</tr>
<tr>
<td>密 码</td>
<td><input type="password" name="password"></td>
</tr>
<tr align="center">
<td colspan="2">
<input type="submit" value="登录"><input type="reset" value="重置">
</td>
</tr>
</table>
</form>
<%
String errMsg = (String) session.getAttribute("errMsg");
if (errMsg != null) {
out.println("<p style='text-align:center;color:red'>" + new String(errMsg.getBytes("ISO-8859-1"),"utf-8") + "</p>");
}
%>
</body>
</html>
5.创建数据库
6.