一.首先来看android-async-http的一段介绍:
An asynchronous callback-based Http client for Android built on top of Apache’s HttpClient libraries. All requests are made outside of your app’s main UI thread, but any callback logic will be executed on the same thread as the callback was created using Android’s Handler message passing. You can also use it in Service or background thread, library will automatically recognize in which context is ran.
1.移动端网络请求是个再普遍不过的东西,使用原生的请求API会书写大量网络请求代码,导致繁琐和大量重复性逻辑。关于一些第三方的网络请求框架其实也很多,并且在Git上开源,比如:xUtil,Volley等都是非常强大的网络请求框架。
xUtil与Volley比较:
Volley:2013年Google I/O大会上推出了一个新的网络通信框架——Volley。Volley可是说是把AsyncHttpClient和Universal-Image-Loader的优点集于了一身,既可以像AsyncHttpClient一样非常简单地进行HTTP通信,也可以像Universal-Image-Loader一样轻松加载网络上的图片。除了简单易用之外,Volley在性能方面也进行了大幅度的调整,它的设计目标就是非常适合去进行数据量不大,但通信频繁的网络操作,而对于大数据量的网络操作,比如说下载文件等,Volley的表现就会非常糟糕。
这一点可以通过其官方介绍的图片可以看出: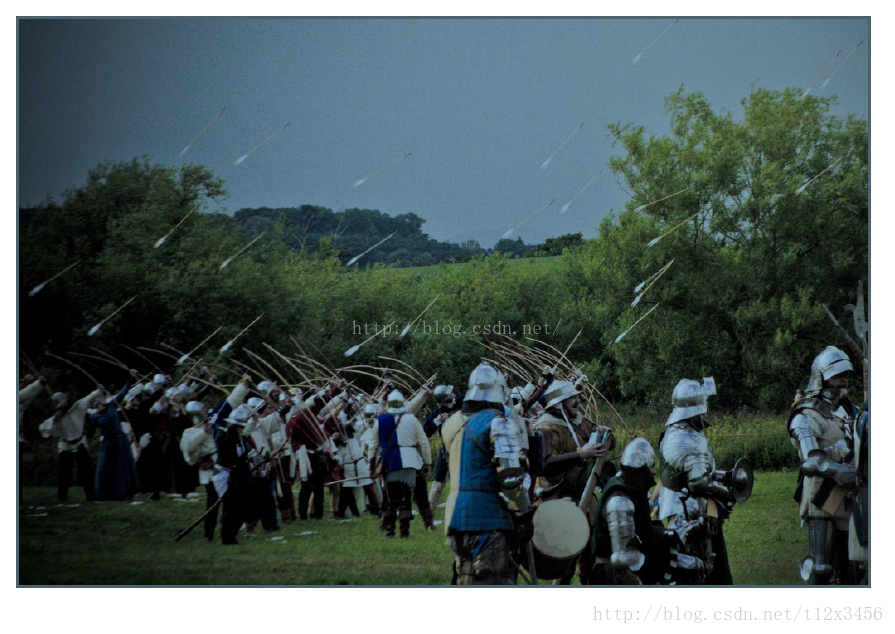
从图中可以看出Volley非常适合通信量不大但是高并发的场景,这张图很准确的表示出Volley的特性。
可以通过该地址获取到最新的源码: https://android.googlesource.com/platform/frameworks/volley
xUtil:目前它已经进化到xUtil3,
- xUtils 包含了很多实用的android工具。
- xUtils 最初源于Afinal框架,进行了大量重构,使得xUtils支持大文件上传,更全面的http请求协议支持(10种谓词),拥有更加灵活的ORM,更多的事件注解支持且不受混淆影响...
- xUitls最低兼容android 2.2 (api level 8)
共有四大模块:{DbUtils, ViewUtils,HttpUtils,BitmapUtils}
关于它的介绍网上的资料非常多,并且很详细,缺点是它比起其它相关的框架有些偏大。
可以通过该地址获取到最新的源码:
2.其实网络请求框架根本实现原理都是一致的,只是为了方便开发者使用,在原有的基础上做了层抽象,使的每一个网络请求的书写不在那么繁琐,使调用者使用几行代码通过实现其回调方法等完成一次网络请求任务。从上文官方介绍中可以了解到android-async-http基于异步回调的Android内置的Apache HttpClient库HTTP客户端。所有请求都是在main thread之外的(UI线程),但任何回调逻辑都会在同一个线程上执行,回调使用android内置的消息机制。
二.android-async-http有哪些特性: 如果使用android studio通过:获得最新
1.它具有很小的开销。只有90KB
2.独立与UI线程之外
3.使用线程池并发
4.持久性cookie存储,保存cookie到你的应用程序的SharedPreferences
等,有兴趣的话可以通过可以了解详情:http://loopj.com/android-async-http/
三.使用非常简单,向前面所说只需几行代码实现其接口回调即可完成一次网络请求
1.创建一个新的asynchttpclient实例并提出要求: get方式
2.建议用法:做静态的HTTP客户端:
import com.loopj.android.http.*;
public class TwitterRestClient {
private static final String BASE_URL = "https://api.twitter.com/1/";
private static AsyncHttpClient client = new AsyncHttpClient();
public static void get(String url, RequestParams params, AsyncHttpResponseHandler responseHandler) {
client.get(getAbsoluteUrl(url), params, responseHandler);
}
public static void post(String url, RequestParams params, AsyncHttpResponseHandler responseHandler) {
client.post(getAbsoluteUrl(url), params, responseHandler);
}
private static String getAbsoluteUrl(String relativeUrl) {
return BASE_URL + relativeUrl;
}
}
3.这使得它很容易与你的代码中的代码:
import org.json.*;
import com.loopj.android.http.*;
class TwitterRestClientUsage {
public void getPublicTimeline() throws JSONException {
TwitterRestClient.get("statuses/public_timeline.json", null, new JsonHttpResponseHandler() {
@Override
public void onSuccess(int statusCode, Header[] headers, JSONObject response) {
// If the response is JSONObject instead of expected JSONArray
}
@Override
public void onSuccess(int statusCode, Header[] headers, JSONArray timeline) {
// Pull out the first event on the public timeline
JSONObject firstEvent = timeline.get(0);
String tweetText = firstEvent.getString("text");
// Do something with the response
System.out.println(tweetText);
}
});
}
}
4.与persistentcookiestore持久性cookie存储:
首先创建AsyncHttpClient实例:
AsyncHttpClient myClient = new AsyncHttpClient();
现在这个客户端的cookie存储是persistentcookiestore新实例,PersistentCookieStore myCookieStore = new PersistentCookieStore(this); myClient.setCookieStore(myCookieStore);
服务器接收到的任何的饼干都将被储存在持久性的饼干存储中:
BasicClientCookie newCookie = new BasicClientCookie("cookiesare", "awesome");
newCookie.setVersion(1);
newCookie.setDomain("mydomain.com");
newCookie.setPath("/");
myCookieStore.addCookie(newCookie);
5.使用post请求方式:
构建请求实体:RequestParams
创建一个单参数的RequestParams实例
使用现有的Map获取key,value
6.Requestparams上传文件,支持多文件上传,如下:添加一个InputStream的Requestparams上传:
添加一个文件对象的requestparams上传:
添加一个字节数组的requestparams上传:
7.下载的二进制数据FileasyncHttpresponseHandler:byte[] myByteArray = blah; RequestParams params = new RequestParams(); params.put("soundtrack", new ByteArrayInputStream(myByteArray), "she-wolf.mp3");
AsyncHttpClient client = new AsyncHttpClient(); client.get("https://example.com/file.png", new FileAsyncHttpResponseHandler(/* Context */ this) { @Override public void onSuccess(int statusCode, Header[] headers, File response) { // Do something with the file `response` } });
先介绍这么多,感兴趣的朋友可以深入了解:http://loopj.com/android-async-http/