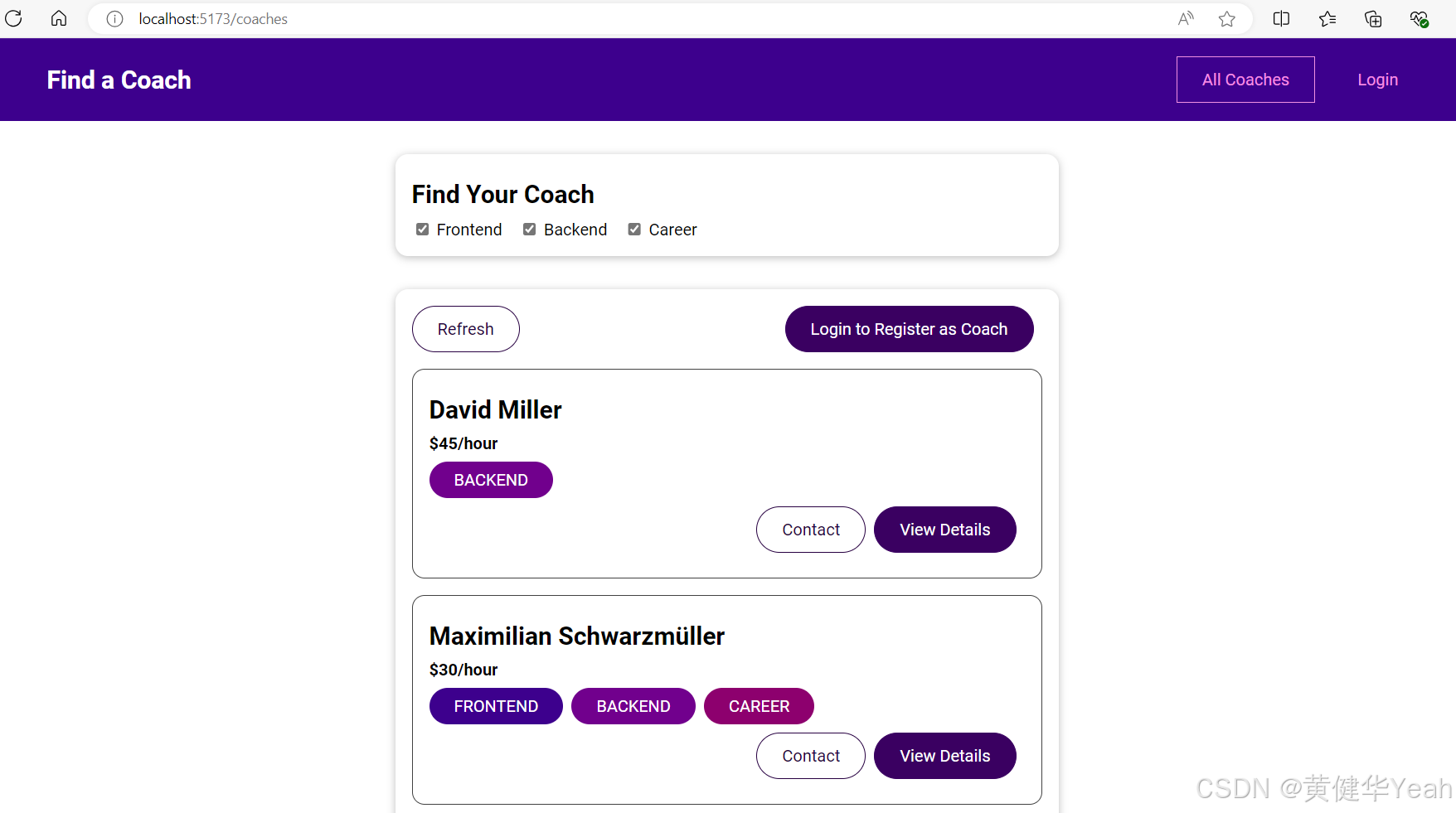
步骤
1、UserAuth.vue中登录成功后跳转路由到注册页面或者Coaches页面
async submitForm() {
this.formIsValid = true;
if (
this.email === '' ||
!this.email.includes('@') ||
this.password.length < 6
) {
this.formIsValid = false;
return;
}
this.isLoading = true;
const actionPayload = { email: this.email, password: this.password };
try {
// send http request...
if (this.mode === 'login') {
console.log('Logging in...');
await this.$store.dispatch('login', actionPayload);
console.log('Logged in successfully.');
} else {
console.log('Signing up...');
await this.$store.dispatch('signup', actionPayload);
console.log('Signed up successfully.');
}
const redirectUrl = '/' + (this.$route.query.redirect || 'coaches');
this.$router.replace(redirectUrl);
} catch (error) {
this.error = error.message || 'Failed to authenticate, try later.';
}
this.isLoading = false;
},
2、TheHeader.vue
logout() {
this.$store.dispatch("logout");
this.$router.replace("/coaches");
}
3、登录成功后重定向到注册页面CoachesList.vue
<base-button link to="/auth?redirect=register" v-if="!isLoggedIn">Login to Register as Coach</base-button>
4、router.js添加Guard
import { createRouter, createWebHistory } from "vue-router";
import CoachDetail from "./pages/coaches/CoachDetail.vue";
import CoachesList from "./pages/coaches/CoachesList.vue";
import CoachRegistration from "./pages/coaches/CoachRegistration.vue";
import ContactCoach from "./pages/requests/ContactCoach.vue";
import RequestsReceived from "./pages/requests/RequestsReceived.vue";
import UserAuth from "./pages/auth/UserAuth.vue";
import NotFound from "./pages/NotFound.vue";
import store from "./stores/index.js";
const router = createRouter({
history: createWebHistory(),
routes: [
{ path: '/', redirect: '/coaches' },
{ path: '/coaches', component: CoachesList },
{
path: '/coaches/:id',
component: CoachDetail,
props: true, // make id available as a prop
children: [
{ path: 'contact', component: ContactCoach }, // /coaches/1234567890/contact
]
},
{ path: '/register', component: CoachRegistration, meta: { requiresAuth: true } },
{ path: '/requests', component: RequestsReceived, meta: { requiresAuth: true } },
{ path: '/auth', component: UserAuth, meta: { requiresUnauth: true } },
{ path: '/:notFound(.*)', component: NotFound },
],
});
//Guard against unauthorized access
router.beforeEach((to, from, next) => {
if (to.meta.requiresAuth && !store.getters.isAuthenticated) {
next('/auth');
} else if (to.meta.requiresUnauth && store.getters.isAuthenticated) {
next('/coaches');
} else {
next();
}
});
export default router;