class类的使用
/**
* class类的使用
*/
public class demo1 {
public static void main(String[] args) throws IllegalAccessException, InstantiationException {
ProductService service = new ProductService();
Class<ProductService> class1 = ProductService.class;
Class<? extends ProductService> class2 = service.getClass();
Class<?> class3 = null;
try {
class3 = Class.forName("com.example.demo.MyAOp.service.ProductService");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
/**
* class1,class2,class3表示ProductService类的类类型
* 万事万物皆对象
* 类也是对象,是class类的实例对象
* 这种对象我们称为该类的类类型
* 一个类只可能是Class类的一个实例对象,class1=class2=class3
*/
System.out.println(class1==class2);
System.out.println(class1==class3);
System.out.println(class2==class3);
try {
ProductService productService1 = class1.newInstance();
ProductService productService2 = class2.newInstance();
ProductService productService3 = (ProductService)class3.newInstance();
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
获取方法信息
/**
* 获取方法信息
*/
public class MehtedMseeage {
public static void main(String[] args) {
getClassMessage(new ProductService());
}
/**
* 获取方法返回类型,方法名,参数类型
* @param obj
*/
public static void getClassMessage(Object obj) {
Class<?> c = obj.getClass();
String name = c.getName();
String simpleName = c.getSimpleName();
Method[] methods = c.getMethods();
Method[] declaredMethods = c.getDeclaredMethods();
for (Method declaredMethod:declaredMethods){
Class<?> returnType = declaredMethod.getReturnType();
System.out.print(returnType.getSimpleName()+ " ");
String name1 = declaredMethod.getName();
System.out.print(name1 + "(");
Class<?>[] parameterTypes = declaredMethod.getParameterTypes();
for (Class parameterType:parameterTypes) {
System.out.print(parameterType.getSimpleName() + ",");
}
System.out.println(")");
}
}
}
成员变量信息
/**
* 获取成员变量
* 成员变量也是对象
* 是java.lang.reflect.Filed对象
* Filed封装了关于成员变量的操作
*/
public class MemberVariables {
public static void main(String[] args) {
getMethodFiledMessage(new ProductService());
}
/**
* 成员变量信息
* @param obj
*/
public static void getMethodFiledMessage(Object obj) {
Class<?> c = obj.getClass();
Field[] fields = c.getFields();
Field[] declaredFields = c.getDeclaredFields();
for (Field field : declaredFields) {
Class<?> type = field.getType();
System.out.print(type.getSimpleName() + " ");
String name = field.getName();
System.out.println(name);
}
}
}
构造函数
/**
* 构造函数信息
* java.lang.Constructors种封装了构造函数信息
* @param obj
*/
public static void getMethodConMessage(Object obj) {
Class<?> c = obj.getClass();
Constructor<?>[] constructors = c.getConstructors();
Constructor<?>[] declaredConstructors = c.getDeclaredConstructors();
for (Constructor constructor:declaredConstructors){
String name = constructor.getName();
System.out.print(name + "(");
Class[] parameterTypes = constructor.getParameterTypes();
for (Class parameterType:parameterTypes) {
System.out.print(parameterType.getSimpleName() + ",");
}
System.out.println(")");
}
}
方法的反射
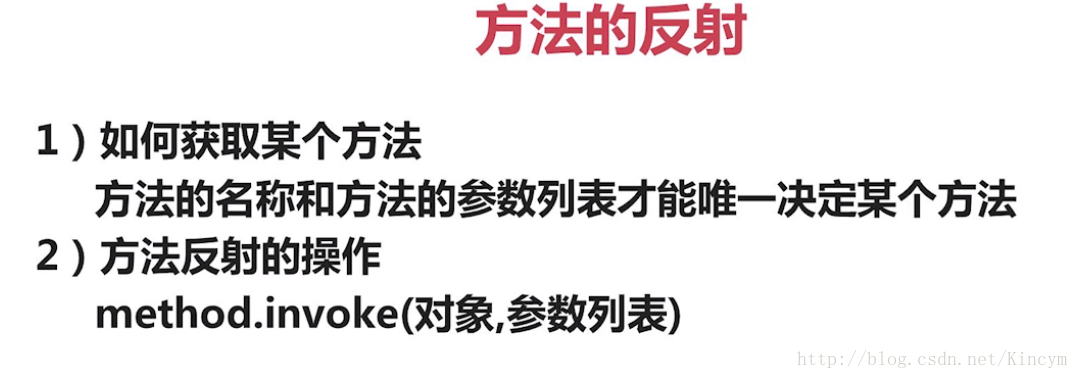
public class FanShe {
public static void main(String[] args) {
try {
A a = new A();
Class<? extends A> c = a.getClass();
Method method = c.getDeclaredMethod("print",new Class[]{int.class,int.class});
Object invoke = method.invoke(a, 1, 2);
System.out.println("======------------------------===============");
Method method2 = c.getDeclaredMethod("print",String.class,String.class);
Object invoke2 = method2.invoke(a, new Object[]{"av","aa"});
} catch (NoSuchMethodException e) {
e.printStackTrace();
System.out.println("======");
} catch (Exception e) {
e.printStackTrace();
System.out.println("======");
}
}
}
class A {
public void print(int a,int b) {
System.out.println(a+b);
}
public void print(String a,String b) {
System.out.println(a.toUpperCase()+b.toLowerCase());
}
}