数据结构学习了以下内容:顺序表,链表,顺序栈,链栈,顺序队列,链式队列,数结构,二叉树,图,,快速排序,哈希;
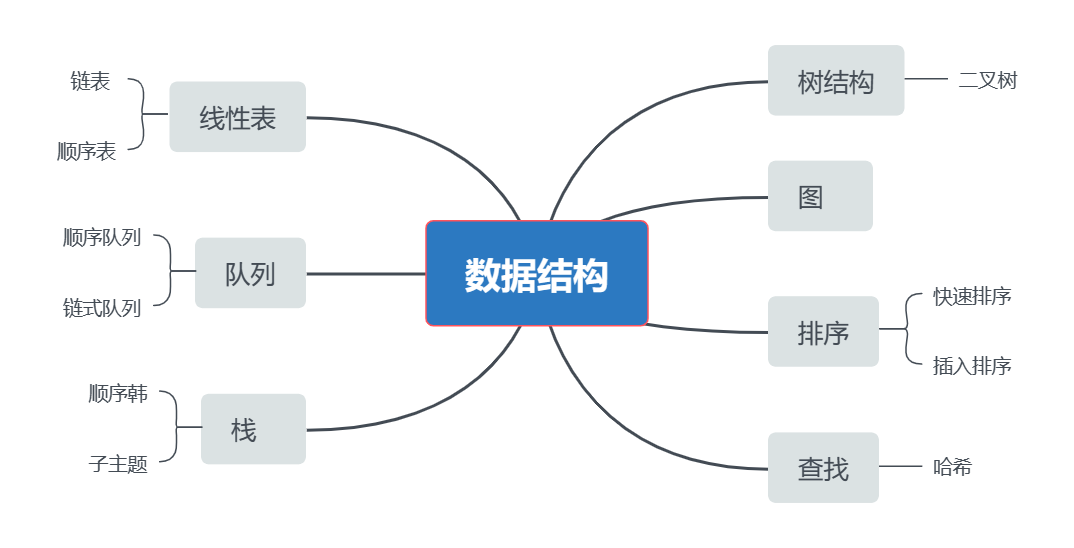
数据与数据之间满足一对一的关系:线性关系
对于线性关系的存储结构主要从如下三个方面展开
一、线性表
线性表定义:线性表中数据元素之间满足线性关系,线性表是一种基础的,简单的数据结构类型
线性表的特征:
对于长度为n的非空线性表,A0为表头,无前驱
An-1 为表尾,无后继
其他元素Ai都有唯一一个直接前驱(Ai-1)和一个直接后继(Ai+1)
线性表的顺序存储:顺序表
顺序表是线性表的顺序存储,需要开辟一块连续的空间,所以使用数组。为了方便访问顺序表,我们需要定义一个变量last,存储最后一个数据的位置,后续代码(用last = -1编写)
sqlist.h头文件
#ifndef _seqlist_
#define _seqlist_
#define N 64
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
typedef int datatype;
typedef struct{
datatype data[N];
int last;
}seqlist;
seqlist* seqlistCreate();
void seqlistPrint(seqlist *s);
void seqlistInsert(seqlist *s,datatype value);
bool seqlistIsEmpty(seqlist *s);
bool seqlistIsFull(seqlist *s);
void seqlistModifyDataByPos(seqlist *s, datatype value, int pos);
void seqlistModifyDataByData(seqlist *s, datatype oldvalue, datatype newvalue);
int seqlistSearchPosByData(seqlist *s, datatype value);
void seqlistIsertDataByPos(seqlist *s,datatype value, int pos);
void seqlistDeleteDataByPos(seqlist *s,int pos);
void seqlistDedup(seqlist *s);
void seqlistMerge(seqlist *s1, seqlist *s2);
void seqlistSortSimple(seqlist *s);
datatype seqlistDelete(seqlist *s);
#endif
sqlist.c
#include "sqlist.h"
seqlist* seqlistCreate(){
seqlist *s;
if ((s = (seqlist *)malloc(sizeof(seqlist))) == NULL){
printf("malloc failed\n");
return NULL;
}
memset(s->data,0,sizeof(s->data));
s->last = -1;
return s;
}
void seqlistPrint(seqlist *s){
if (seqlistIsEmpty(s) == true){
printf("empty\n");
return;
}
int i = 0;
for ( i = 0; i <= s->last; i++){
printf("%d ",s->data[i]);
}
puts("");
}
void seqlistInsert(seqlist *s,datatype value){
if (seqlistIsFull(s) == true){
printf("full\n");
return;
}
s->last++;
s->data[s->last] = value;
}
bool seqlistIsEmpty(seqlist *s){
return s->last < 0 ? true:false;
}
bool seqlistIsFull(seqlist *s){
return s->last > N-1? true:false;
}
datatype seqlistDelete(seqlist *s){
if (seqlistIsEmpty(s) == true){
printf("empty\n");
return (datatype)-1;
}
s->last--;
return s->data[s->last+1];
}
void seqlistModifyDataByPos(seqlist *s, datatype value, int pos){
if (seqlistIsEmpty(s) == true){
printf("empty\n");
return;
}
if (pos > s->last || pos < 0){
printf("pos error\n");
return;
}
s->data[pos] = value;
}
void seqlistModifyDataByData(seqlist *s, datatype oldvalue, datatype newvalue){
int i;
if (seqlistIsEmpty(s) == true){
printf("empty\n");
return;
}
for (i = 0; i <= s->last; i++){
if (s->data[i] == oldvalue){
s->data[i] = newvalue;
}
}
}
int seqlistSearchPosByData(seqlist *s, datatype value){
int i;
for ( i = 0; i <= s->last; i++){
if (s->data[i] == value){
break;
}
}
return i;
}
void seqlistIsertDataByPos(seqlist *s,datatype value, int pos){
if (seqlistIsEmpty(s) == true){
printf("empty\n");
return;
}
if (pos > s->last || pos < 0){
printf("pos error\n");
return;
}
printf("data:%d\n",s->data[pos]);
}
void seqlistDeleteDataByPos(seqlist *s,int pos){
int i;
if (seqlistIsEmpty(s) == true){
printf("empty\n");
return;
}
if (pos > s->last || pos < 0){
printf("pos error\n");
return;
}
for (i = pos + 1; i <= s->last; i++){
s->data[i-1] = s->data[i];
}
s->last--;
}
void seqlistDedup(seqlist *s){
int i = 0, j = 0;
if (seqlistIsEmpty(s)){
printf("empty\n");
return;
}
if (s->last == 0){
printf("Data only one\n");
return;
}
for (i = 0; i < s->last; i++){
for(j = i+1; j <= s->last; j++){
if (s->data[j] == s->data[i]){
seqlistDeleteDataByPos(s,j);
j--;
}
}
}
}
void seqlistMerge(seqlist *s1, seqlist *s2){
int i = 0, j = 0, k = s1->last;
bool iFind;
for (i = 0; i <= s2->last; i++){
iFind = false;
for (j = 0; j <= k; j++){
if (s2->data[i] == s1->data[j]){
iFind = true;
break;
}
}
if (!iFind){
seqlistInsert(s1,s2->data[i]);
if (s1->last > N-1){
printf("overflow\n");
return;
}
}
}
}
void seqlistSortSimple(seqlist *s){
int i, j;
int temp = s->data[0];
for (i = 0; i <s->last; i++){
for(j = i+1; j <= s->last; j++){
if (s->data[i] < s->data[j]){
temp = s->data[i];
s->data[i] = s->data[j];
s->data[j] = temp;
}
}
}
}
main.c
#include "sqlist.h"
int main(){
seqlist *s1= seqlistCreate();
seqlist *s2= seqlistCreate();
seqlistInsert(s1,10);
seqlistInsert(s1,20);
seqlistInsert(s1,50);
seqlistInsert(s1,70);
seqlistInsert(s2,10);
seqlistInsert(s2,40);
seqlistInsert(s2,30);
seqlistInsert(s2,40);
seqlistInsert(s2,30);
seqlistInsert(s2,40);
seqlistInsert(s2,30);
seqlistInsert(s2,40);
seqlistInsert(s2,20);
printf("s1: ");
seqlistPrint(s1);
printf("s2: ");
seqlistPrint(s2);
printf("Merge: ");
seqlistMerge(s1,s2);
seqlistPrint(s1);
puts("111");
seqlistSortSimple(s1);
// seqlistModifyDataByPos(s,111,2);
// seqlistIsertDataByPos(s,111,1);
// seqlistDeleteDataByPos(s,1);
// printf("pos is %d\n",seqlistSearchPosByData(s,100));
seqlistPrint(s1);
/*seqlistDelete(s);
seqlistDelete(s);
seqlistDelete(s);
seqlistDelete(s);
seqlistDelete(s);
seqlistDelete(s);
seqlistDelete(s);*/
return 0;
}
Makefile
sqlist:sqlist.o main.o
sqlist.o: sqlist.c
main.o:main.c
.PHONY:clean
clean:
${RM} sqlist *.o
线性表的链式存储:链表
单链表不需要开辟连续的空间保存数据,为了让数据与数据之间建立联系,我们把每一个数据称之为结点,结点有两部分组成:数据域和指针域,数据域保存数据,指针域保存后一个结点的地址。
sqlin.h头文件
#ifndef _linklist_
#define _linklist_
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
typedef int datatype;
typedef struct node{
datatype data;
struct node *next;
}linklist;
linklist *linklistCreate();
void linklistHeadInsert(linklist *head, datatype value);
void linklistTailInsert(linklist *head, datatype value);
void linklistPrint(linklist *head);
datatype linklistHeadDelete(linklist *head);
bool linklistIsEmpty(linklist *head);
datatype linklistTailDelete(linklist *head);
void linklistModifyValueByPos(linklist *head, int pos, datatype value);
void linklistModifyValueByValue(linklist *head, datatype oldvalue, datatype newvalue);
void linklistIsertsort(linklist *head, datatype value);
void linklistReversal(linklist *head);
void linklistsort(linklist *head);
datatype linklistSearchValueByPos(linklist *head,int pos);
int linklistSearchPosByValue(linklist *head, datatype value);
void linklistInsertValueByPos(linklist *head,datatype value, int pos);
#endif
sqlink.c
#include "sqlink.h"
linklist *linklistCreate(){
linklist *head = (linklist*)malloc(sizeof(linklist));
if (head == NULL){
printf("malloc failed\n");
return NULL;
}
head->data = 0;
head->next = NULL;
return head;
}
void linklistHeadInsert(linklist *head, datatype value){
linklist *temp = (linklist *)malloc(sizeof(linklist));
temp->data = value;
temp->next = head->next;
head->next = temp;
}
void linklistTailInsert(linklist *head, datatype value){
linklist *temp = (linklist *)malloc(sizeof(linklist));
temp->data = value;
temp->next = NULL;
while(head->next != NULL){
head = head->next;
}
head->next = temp;
}
void linklistPrint(linklist *head){
if(linklistIsEmpty(head) == true){
printf("empty\n");
return;
}
while(head->next !=NULL){
head = head->next;
printf("%d ",head->data);
}
puts("");
}
datatype linklistHeadDelete(linklist *head){
linklist *temp;
datatype t;
temp = head->next;
t = temp->data;
head->next = temp->next;
free(temp);
return t;
}
bool linklistIsEmpty(linklist *head){
return head->next ==NULL ? true:false;
}
datatype linklistTailDelete(linklist *head){
linklist *temp;
datatype t;
if(linklistIsEmpty(head) == true){
printf("empty\n");
return (datatype)-1;
}
while(head->next->next != NULL){
head = head->next;
}
temp = head->next;
head->next = NULL;
t = temp->data;
free(temp);
return t;
}
void linklistModifyValueByPos(linklist *head, int pos, datatype value){
int i = 0;
if(linklistIsEmpty(head) == true){
printf("empty\n");
return;
}
while(i < pos && head->next != NULL){
head = head->next;
i++;
}
if (i != pos){
printf("pos error\n");
return;
}
head->data = value;
}
void linklistModifyValueByValue(linklist *head, datatype oldvalue, datatype newvalue){
if(linklistIsEmpty(head) == true){
printf("empty\n");
return;
}
while (head->next != NULL){
head = head->next;
if (head->data = oldvalue){
head->data = newvalue;
return;
}
}
printf("none\n");
}
void linklistIsertsort(linklist *head, datatype value){
linklist *temp = (linklist *)malloc(sizeof(linklist));
temp->data = value;
temp->next = NULL;
while (head->next != NULL && value > head->next->data){
head = head->next;
}
temp->next = head->next;
head->next = temp;
}
void linklistReversal(linklist *head){
linklist *temp1, *temp2;
temp1 = head->next;
head->next = NULL;
while(temp1 != NULL){
temp2 = temp1;
temp1 = temp1->next;
temp2 = head->next;
head->next = temp2;
}
}
void linklistsort(linklist *head){
linklist *temp = NULL, *tail = NULL, *p = NULL;
tail = head->next;
head->next = NULL;
while(tail != NULL){
p = head;
temp = tail;
tail = tail->next;
while(p->next != NULL && temp->data > p->next->data){
p = p->next;
}
temp->next = p->next;
p->next = temp;
}
}
datatype linklistSearchValueByPos(linklist *head,int pos){
int i = 0;
if (linklistIsEmpty(head)){
printf("the linklist is empty\n");
return (datatype)-1;
}
while(head->next != NULL && i < pos){
head = head->next;
i++;
}
if (i != pos){
printf("pos error\n");
return (datatype)-1;
}
return head->data;
}
int linklistSearchPosByValue(linklist *head, datatype value){
int i = 0;
if (linklistIsEmpty(head)){
printf("the linklist is empty\n");
return (datatype)-1;
}
while(head->next != NULL){
head = head->next;
i++;
if (head->data == value){
return i;
}
}
printf("none\n");
return -1;
}
void linklistInsertValueByPos(linklist *head,datatype value, int pos){
int i = 1;
linklist *temp = (linklist *)malloc(sizeof(linklist));
if (linklistIsEmpty(head)){
printf("the linklist is empty\n");
return ;
}
while(head->next != NULL && i < pos){
head = head->next;
i++;
}
if (i != pos){
printf("pos error\n");
free(temp);
return ;
}
temp->next = head->next;
temp->data = value;
head->next = temp;
}
main.c
#include "sqlink.h"
int main(){
linklist *head = linklistCreate();
linklistIsertsort(head,1);
linklistIsertsort(head,7);
linklistIsertsort(head,9);
linklistIsertsort(head,5);
linklistIsertsort(head,6);
linklistIsertsort(head,2);
linklistIsertsort(head,10);
linklistIsertsort(head,4);
linklistIsertsort(head,3);
linklistIsertsort(head,8);
linklistPrint(head);
puts("");
linklistReversal(head);
linklistPrint(head);
puts("");
linklistHeadInsert(head,1);
linklistHeadInsert(head,2);
linklistTailInsert(head,1);
linklistTailInsert(head,2);
linklistTailInsert(head,3);
linklistPrint(head);
puts("");
linklistModifyValueByPos(head,3,5);
linklistModifyValueByPos(head,8,5);
linklistPrint(head);
puts("");
printf("pos 2 value:%d\n",linklistSearchValueByPos(head,2));
printf("value 2 pos:%d\n",linklistSearchPosByValue(head,2));
printf("value 3 pos:%d\n",linklistSearchPosByValue(head,3));
printf("value 8 pos:%d\n",linklistSearchPosByValue(head,8));
linklistInsertValueByPos(head,100,6);
linklistPrint(head);
puts("");
linklistInsertValueByPos(head,100,8);
linklistPrint(head);
puts("");
linklistsort(head);
puts("111");
linklistPrint(head);
puts("");
return 0;
}
单向循环链表
loop.h
#ifndef _LOOPLIST_H_
#define _LOOPLIST_H_
#include <stdio.h>
#include <stdlib.h>
typedef int datatype;
typedef struct looplist{
datatype data;
struct looplist *next;
}loop;
loop *loopCreate();
void loopHeadInsert(loop * head,datatype data);
void loopprint(loop *head);
loop *loopDelHead(loop *head);
void loopNoHeadPrint(loop *head);
#endif
loop.c
#include "looplist.h"
loop *loopCreate(){
loop *head = (loop *)malloc(sizeof(loop));
head->next = head;
return head;
}
void loopHeadInsert(loop * head,datatype data){
loop *temp = (loop *)malloc(sizeof(loop));
temp->next = head->next;
head->next = temp;
temp->data = data;
}
void loopprint(loop *head){
loop *temp = head;
while(temp->next != head){
temp = temp->next;
printf("%d ",temp->data);
}
puts("");
}
loop *loopDelHead(loop *head){
loop *temp = head;
while (temp->next != head){
temp = temp->next;
}
temp->next = head->next;
temp = head->next;
free(head);
return temp;
}
void loopNoHeadPrint(loop *head){
loop *temp = head;
while (temp->next != head){
printf("%d ",temp->data);
temp = temp->next;
}
printf("%d\n",temp->data);
}
main.c
#include "looplist.h"
int main(){
loop *head = loopCreate();
loopHeadInsert(head,100);
loopHeadInsert(head,200);
loopHeadInsert(head,300);
loopHeadInsert(head,400);
loopHeadInsert(head,500);
loopHeadInsert(head,600);
loopprint(head);
head = loopDelHead(head);
printf("after del head\n");
loopNoHeadPrint(head);
}
双向循环链表
doublelooplist.h
#ifndef _LOOPLIST_H_
#define _LOOPLIST_H_
#include <stdio.h>
#include <stdlib.h>
typedef int datatype;
typedef struct looplist{
datatype data;
struct looplist *next;
}loop;
loop *loopCreate();
void loopHeadInsert(loop * head,datatype data);
void loopprint(loop *head);
loop *loopDelHead(loop *head);
void loopNoHeadPrint(loop *head);
#endif
doublelooplist.c
#include "looplist.h"
loop *loopCreate(){
loop *head = (loop *)malloc(sizeof(loop));
head->next = head;
return head;
}
void loopHeadInsert(loop * head,datatype data){
loop *temp = (loop *)malloc(sizeof(loop));
temp->next = head->next;
head->next = temp;
temp->data = data;
}
void loopprint(loop *head){
loop *temp = head;
while(temp->next != head){
temp = temp->next;
printf("%d ",temp->data);
}
puts("");
}
loop *loopDelHead(loop *head){
loop *temp = head;
while (temp->next != head){
temp = temp->next;
}
temp->next = head->next;
temp = head->next;
free(head);
return temp;
}
void loopNoHeadPrint(loop *head){
loop *temp = head;
while (temp->next != head){
printf("%d ",temp->data);
temp = temp->next;
}
printf("%d\n",temp->data);
}
main.c
#include "looplist.h"
int main(){
loop *head = loopCreate();
loopHeadInsert(head,100);
loopHeadInsert(head,200);
loopHeadInsert(head,300);
loopHeadInsert(head,400);
loopHeadInsert(head,500);
loopHeadInsert(head,600);
loopprint(head);
head = loopDelHead(head);
printf("after del head\n");
loopNoHeadPrint(head);
}
二、栈
栈是限制在一端进行插入操作和删除的操作的线性表,允许进行操作的一端称作“栈顶”,另一个固定端称之为“栈底”,当栈中没有元素时称为“空栈”。特点:先进后出。
顺序栈
seqstack.h
#ifndef _stack_
#define _stack_
#define N 8
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
typedef int datatype;
typedef struct
{
datatype data[N];
int top;
}stack;
stack *stackCreate();
void stackPush(stack *s,datatype value);
datatype stackPop(stack *s);
bool stackIsEmpty(stack *s);
bool stackIsFull(stack *s);
#endif
seqstack.c
#include "stack.h"
stack *stackCreate(){
stack *s = (stack *)malloc(sizeof(stack));
s->top = -1;
return s;
}
void stackPush(stack *s,datatype value){
if (stackIsFull(s)){
puts("full");
return ;
}
s->top++;
s->data[s->top] = value;
}
datatype stackPop(stack *s){
if (stackIsEmpty(s)){
puts("empty");
return -1;
}
s->top--;
return s->data[s->top+1];
}
bool stackIsEmpty(stack *s){
return (s->top == -1) ? true:false;
}
bool stackIsFull(stack *s){
return (s->top == N-1) ? true:false;
}
main.c
#include "stack.h"
int main(){
stack *s = (stack *)malloc(sizeof(stack));
stackPush(s,100);
stackPush(s,200);
stackPush(s,300);
stackPush(s,400);
stackPush(s,500);
stackPush(s,600);
stackPush(s,700);
stackPush(s,800);
stackPush(s,900);
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
printf("pop:%d\n",stackPop(s));
}
链式栈
linkstack.h头文件
#ifndef _linkstack_
#define _linkstack_
#include<stdio.h>
#include<stdlib.h>
#include<stdbool.h>
typedef int datatype;
typedef struct node{
datatype data;
struct node *next;
}stack;
void linkstackpush(stack **s, datatype data);
datatype linkstackpop(stack **s);
bool linkstackIsEmpty(stack *s);
#endif
linkstack.c
#include "linkstack.h"
void linkstackpush(stack **s, datatype data){
stack *temp = (stack *)malloc(sizeof(stack));
temp->next = *s;
temp->data = data;
*s = temp;
}
datatype linkstackpop(stack **s){
if(linkstackIsEmpty(*s)){
puts("empty");
return (datatype)-1;
}
stack *temp = *s;
datatype t = temp->data;
*s = (*s)->next;
free(temp);
temp = NULL;
return t;
}
bool linkstackIsEmpty(stack *s){
return (s == NULL) ? true : false;
}
main.c
#include "linkstack.h"
int main(){
stack *s = NULL;
printf("pop :%d\n",linkstackpop(&s));
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
linkstackpush(&s,100);
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
printf("pop :%d\n",linkstackpop(&s));
}
三、队列
顺序队列
queue.h
#ifndef _squeue_
#define _squeue_
#include<stdio.h>
#include<stdlib.h>
#include<stdbool.h>
#define N 8
typedef int datatype;
typedef struct{
datatype data[N];
int head;
int tail;
}squeue;
squeue *squeueCreate();
void squeueInput(squeue *sq,datatype data);
datatype squeueOutput(squeue *sq);
bool squeueEmpty(squeue *sq);
bool squeueFull(squeue *sq);
void squeueprint(squeue *sq);
#endif
queue.c
#ifndef _squeue_
#define _squeue_
#include<stdio.h>
#include<stdlib.h>
#include<stdbool.h>
#define N 8
typedef int datatype;
typedef struct{
datatype data[N];
int head;
int tail;
}squeue;
squeue *squeueCreate();
void squeueInput(squeue *sq,datatype data);
datatype squeueOutput(squeue *sq);
bool squeueEmpty(squeue *sq);
bool squeueFull(squeue *sq);
void squeueprint(squeue *sq);
#endif
main.c
#include "squeue.h"
int main(){
squeue *sq = squeueCreate();
squeueInput(sq,1);
squeueInput(sq,2);
squeueInput(sq,3);
squeueInput(sq,4);
squeueInput(sq,5);
squeueInput(sq,6);
squeueInput(sq,7);
squeueInput(sq,8);
squeueInput(sq,9);
squeueprint(sq);
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
squeueprint(sq);
squeueInput(sq,5);
printf("tail: %d data: %d\n",sq->tail,sq->data[7]);
squeueInput(sq,6);
printf("tail: %d data: %d\n",sq->tail,sq->data[sq->tail-1]);
squeueInput(sq,7);
printf("tail: %d data: %d\n",sq->tail,sq->data[sq->tail-1]);
squeueInput(sq,8);
printf("tail: %d data: %d\n",sq->tail,sq->data[sq->tail-1]);
squeueInput(sq,9);
printf("tail: %d data: %d\n",sq->tail,sq->data[sq->tail-1]);
squeueprint(sq);
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
printf("%d\n",squeueOutput(sq));
}
链式队列
linkqueue
#ifndef _LINKQUEUE_
#define _LINKQUEUE_
#include<stdio.h>
#include<stdlib.h>
#include<stdbool.h>
#include<unistd.h>
typedef int datatype;
typedef struct linkqueue{
datatype data;
struct linkqueue *next;
}ln;
typedef struct{
ln *head;
ln *tail;
}lq;
lq *linkqueueCreate(void);
void linkqueueInsert(lq *q,datatype data);
datatype linkqueueOut(lq *q);
bool linkqueueEmpty(lq *q);
void linkqueuePrint(lq *q);
#endif
linkqueue.c
#include "linkqueue.h"
lq *linkqueueCreate(){
lq *q = (lq *)malloc(sizeof(lq));
q->head = q->tail = (ln *)malloc(sizeof(ln));
q->head->next = NULL;
return q;
}
void linkqueueInsert(lq *q, datatype data){
ln *temp = (ln *)malloc(sizeof(ln));
temp->data = data;
temp->next = NULL;
q->tail->next = temp;
q->tail = temp;
}
datatype linkqueueOut(lq *q){
if(linkqueueEmpty(q)){
printf("empty\n");
return -1;
}
ln * temp = q->head;
q->head = q->head->next;
if (linkqueueEmpty(q)){
q->tail = q->head;
}
free(temp);
return q->head->data;
}
bool linkqueueEmpty(lq *q){
return (q->head == q->tail&&q->head->next == NULL) ? true : false;
}
void linkqueuePrint(lq *q){
ln *temp = q->head;
while(temp->next != NULL){
temp = temp->next;
printf("%d ",temp->data);
}
puts("");
}
main.c
#include "linkqueue.h"
int main(){
lq *q = linkqueueCreate();
linkqueueInsert(q,1) ;
linkqueueInsert(q,2) ;
linkqueueInsert(q,3) ;
linkqueueInsert(q,4) ;
linkqueueInsert(q,5) ;
linkqueueInsert(q,6) ;
linkqueueInsert(q,7) ;
linkqueueInsert(q,8) ;
linkqueuePrint(q);
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
printf("%d\n",linkqueueOut(q));
linkqueuePrint(q);
}
四、快排
#include<stdio.h>
#include<unistd.h>
void quicksort(int *arr,int low, int high){
if(low >= high){
return;
}
int temp = arr[low];
int i = low;
int j = high;
while(i < j){
while (arr[j]>=temp && i < j){
j--;
}
arr[i] = arr[j];
if(arr[i] <= temp && i < j){
i++;
}
arr[j] = arr[i];
}
arr[i] = temp;
quicksort(arr,low,i-1);
quicksort(arr,i+1,high);
}
int main(){
int arr[20] = {11,45,3,6,8,94,7,9,65,3,15,45,96,45,98,89,3,1,44,92};
quicksort(arr,0,19);
int i = 0;
while(i<20){
printf("%d ",arr[i]);
usleep(100000);
fflush(stdout);
i++;
}
puts("");
return 0;
}
五、树
树的概念:
树(Tree)是由n(n>=0)个节点的有限集合T
它满足两个条件:
有且仅有一个特定的节点,被称作根(Root)
其余的节点可以分为m个互不相交的有限集合,其中每一个集合又是一棵树,并称之为根的子树。
度树:
一个节点的子树的个数称之为节点的度数
一棵树的度数是指该树中节点的最大度数
层数:
节点的层数等于父节点的层数加一,根节点的层数定义为一
树中节点层数的最大值称为该树的高度或深度
边数:
从根节点K1遍历到此结点Kj,并满足Ki是Ki+1的父节点,就称之为一条从K1到Kj的路径,路径的长度为j-1,即为路径的边数。
叶子(叶节点):
没有子节点的节点
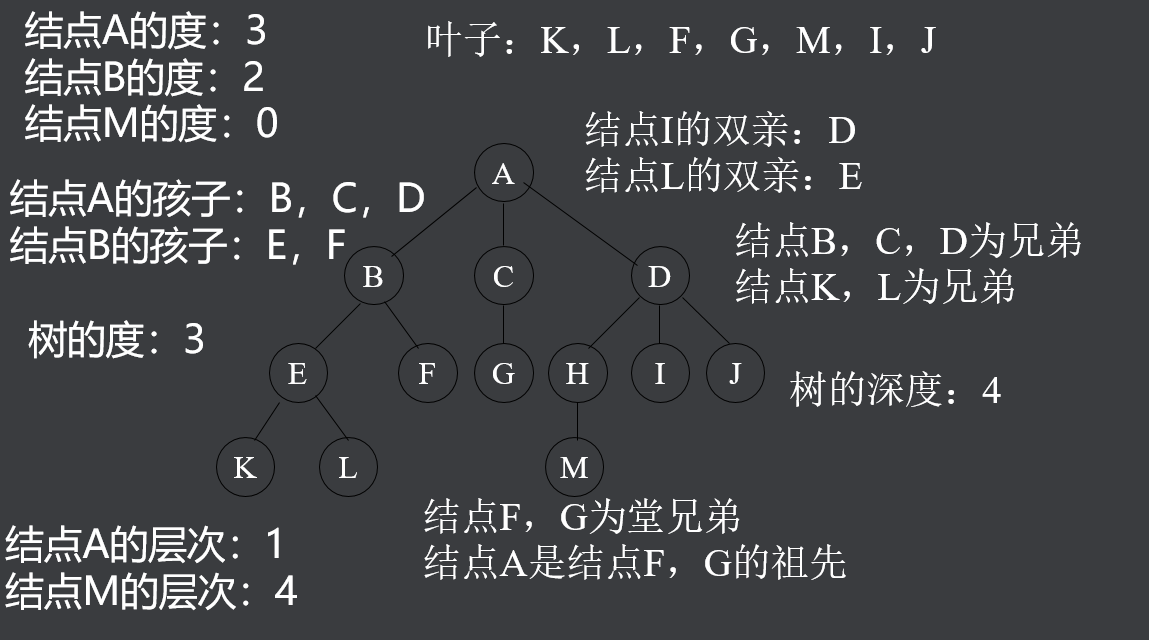
如果根节点的编号为1,则之后的每个节点从上到下、从左到右依次递增编号,如果节点总数为n,
某一个节点的编号为i,则可以判断这个节点是否存在左右子树且可以得到左右子树的编号。
如果2 * i <= n,则这个节点存在左子树,并且编号为 2 * i
如果2 * i + 1 <= n,则这个节点存在右子树,并且编号为2 * i + 1
先序遍历:
先遍历根节点,再遍历左节点,再遍历右节点 —— 根左右
中序遍历:
先遍历左节点,再遍历根节点,再遍历右节点 —— 左根右
后续遍历:
先遍历左节点,再遍历右节点,再遍历根节点 —— 左右根
六、图
1.1 图的相关概念
任意的两个元素都可能相关,即图中任一元素可以有若干个直接前驱和直接后继,属于网状结构类型,我们用“关系”来表示图中数据与数据之间的路径。
1.2 图的分类
有向图(Digraph)
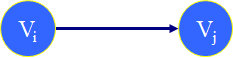
设 Vi、Vj为图中的两个顶点,若 Vi,Vj 存在方向性,即:
从Vi到Vj的路径不等同与从Vj到Vi的路径,则用<>表示,称为关系<Vi, Vj>,Vi为弧头,Vj为弧尾
无向图(Undigraph)
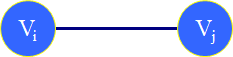
设 Vi、Vj为图中的两个顶点,若 Vi,Vj 不存在方向性,即
从Vi到Vj的路径等同与从Vj到Vi的路径,则用()表示,称为关系(Vi,Vj)或(Vj,Vi)
1.3 图的存储方式
1.3.1 图的链式存储
邻接表表示法:创建多个链表,每个链表的结点存储与当前顶点直接相邻的顶点
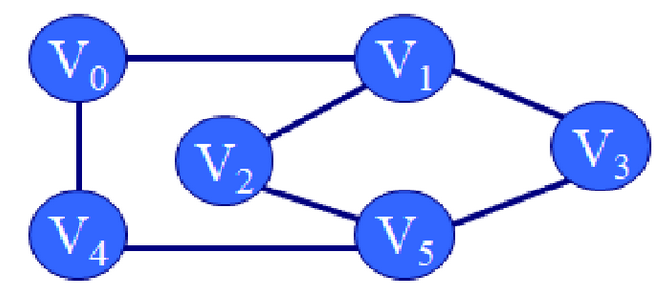
0->1->4->NULL
1->0->3->NULL
2->1->5->NULL
3->1->5->NULL
4->0->5->NULL
5->2->3->NULL